C# Beginner's Guide: Create a Console Message Program for HNDIT Students

Table of contents
- Explanation:
- 1. Print Hello World
- 2. Print a Simple Math Result
- 3. Print a Multiplication Result
- 4. Print a Simple Message Multiple Times
- 5. Print a Simple Shape
- 6. Print the Result of the Division
- 7. Print Your Name and Age
- 8.Print a List of Fruits
- 9. Print Simple Shapes Using Asterisks
- 10. Print Multiple Lines of Text
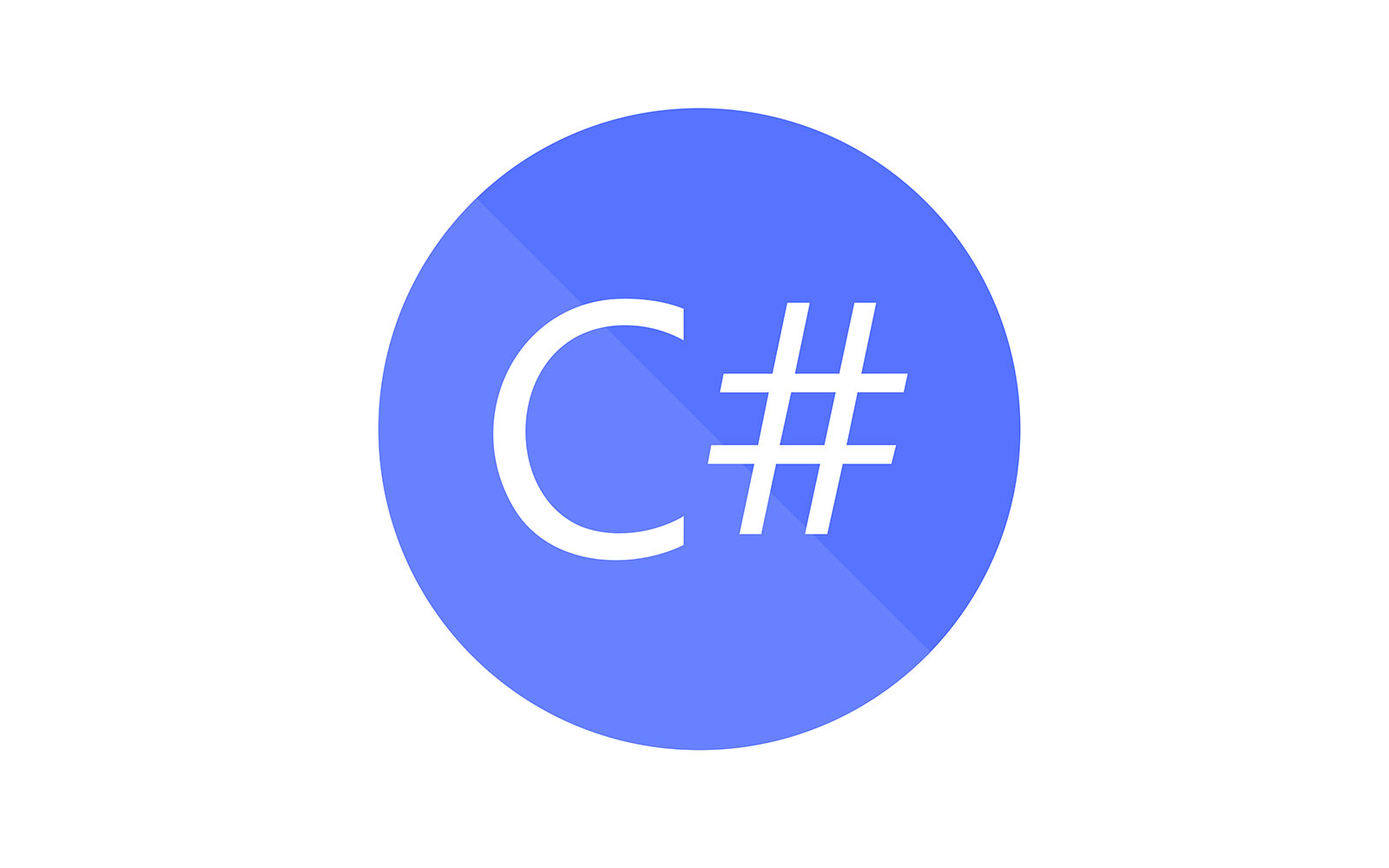
C# program for beginners that prints a message to the console:
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
Console.WriteLine("Welcome to C# programming!");
Console.ReadKey();
}
}
Explanation:
using System;
– This includes theSystem
namespace, which contains basic classes likeConsole
that we need for input/output.class Program
– Defines a class namedProgram
.static void Main(string[] args)
– TheMain
method is the entry point of a C# console application.Console.WriteLine("Hello, World!");
– Prints "Hello, World!" and moves to the next line.Console.ReadKey();
– Waits for the user to press a key before closing the console window.
1. Print Hello World
Write a simple program that prints "Hello, World!" to the console.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
2. Print a Simple Math Result
Write a program that directly prints the result of adding two numbers.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(10 + 20); // Prints the sum of 10 and 20
}
}
3. Print a Multiplication Result
Print the result of multiplying two numbers directly.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(5 * 3); // Prints the result of 5 multiplied by 3
}
}
4. Print a Simple Message Multiple Times
Print a message multiple times using Console.WriteLine
.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("I am learning C#!");
Console.WriteLine("I am learning C#!");
Console.WriteLine("I am learning C#!");
}
}
5. Print a Simple Shape
Print a square shape using asterisks (*
).
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("*****");
Console.WriteLine("*****");
Console.WriteLine("*****");
Console.WriteLine("*****");
Console.WriteLine("*****");
}
}
6. Print the Result of the Division
Write a program that prints the result of dividing two numbers directly.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(100 / 5); // Prints the result of 100 divided by 5
}
}
7. Print Your Name and Age
Write a program that prints your name and age to the console.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("My name is [Your Name].");
Console.WriteLine("I am [Your Age] years old.");
Console.ReadKey();
}
}
Task: Replace [Your Name]
and [Your Age]
with your actual name and age.
8.Print a List of Fruits
Print a list of fruits in separate lines.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("List of fruits:");
Console.WriteLine("1. Apple");
Console.WriteLine("2. Banana");
Console.WriteLine("3. Mango");
Console.WriteLine("4. Orange");
Console.WriteLine("5. Pineapple");
}
}
9. Print Simple Shapes Using Asterisks
Print a triangle shape using asterisks (*
).
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(" * ");
Console.WriteLine(" *** ");
Console.WriteLine("*****");
}
}
10. Print Multiple Lines of Text
Print a few sentences in separate lines.
csharpCopy codeusing System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("C# is a powerful programming language.");
Console.WriteLine("It is widely used for developing desktop and web applications.");
Console.WriteLine("Let's keep practicing and improving!");
}
}
break;
Subscribe to my newsletter
Read articles from Arzath Areeff directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arzath Areeff
Arzath Areeff
I co-founded digizen.lk to promote online safety and critical thinking. Currently, I’m developing an AI app to fight misinformation. As Founder and CEO of ideaGeek.net, I help turn startup dreams into reality, and I share tech insights and travel stories on my YouTube channels, TechNomad and Rz Omar.