Creating an Unbeatable Tic Tac Toe Game Using Minimax Algorithm with Alpha-Beta Pruning in Flutter Development

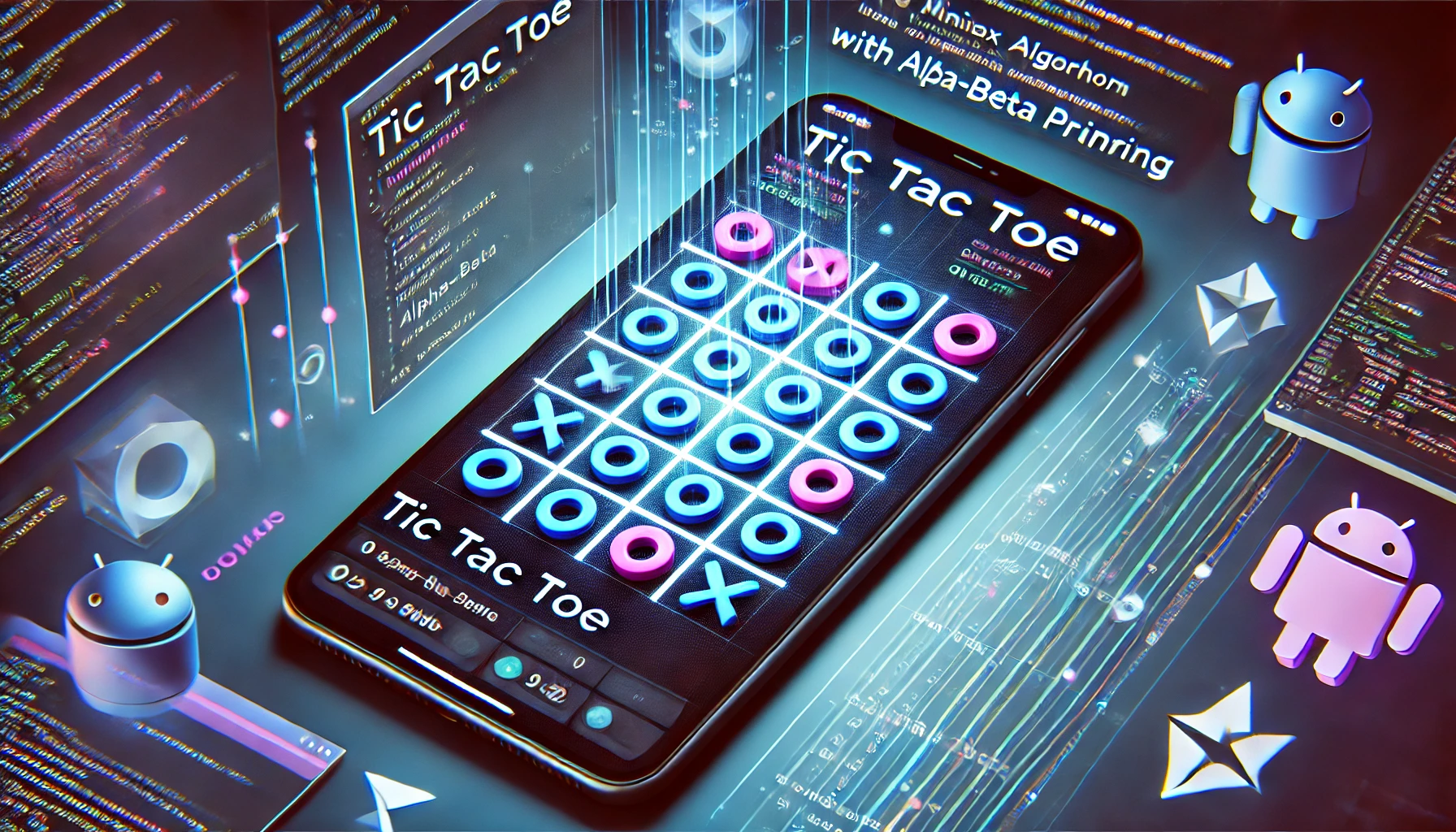
Tic Tac Toe is a simple game, but making an unbeatable AI requires an intelligent algorithm. One such solution is the Minimax Algorithm combined with Alpha-Beta Pruning, which reduces the number of possibilities the AI needs to evaluate. In this article, we will explore how to implement this unbeatable Tic Tac Toe game in Flutter using these advanced techniques.
Introduction to Minimax Algorithm
The Minimax Algorithm is a decision-making algorithm used in turn-based games to minimize the possible loss for a worst-case scenario. It assumes that the opponent will always play optimally. In a Tic Tac Toe game, the algorithm considers all possible moves, assigns a score to each possible outcome, and chooses the move that maximizes the AI's chances of winning or minimizing losses.
How Minimax Works in Tic Tac Toe
Maximizing Player (AI): The AI aims to maximize its score.
Minimizing Player (Human): The human aims to minimize the AI’s score.
The algorithm recursively explores all possible moves, and at the end of the game tree, it assigns a score:
+10 for a win,
0 for a draw,
-10 for a loss.
What is Alpha-Beta Pruning?
Alpha-Beta Pruning is an optimization technique for the Minimax Algorithm. It reduces the number of nodes evaluated by the algorithm in the search tree, cutting off branches that won’t lead to better results than previously examined branches. This makes the algorithm faster and more efficient without affecting the result.
Benefits of Alpha-Beta Pruning
Reduces the number of possible game states evaluated.
Improves performance, especially in games with larger state spaces.
Makes the Minimax Algorithm feasible for real-time applications like Tic Tac Toe in Flutter.
Why Use Flutter for Tic Tac Toe?
Flutter is an excellent framework for building cross-platform mobile and web applications. Here’s why Flutter is perfect for this Tic Tac Toe game:
Cross-platform development: You can deploy your game on both Android and iOS with a single codebase.
Efficient performance: Flutter development services ensures smooth and fast user experiences, even in real-time gameplay scenarios.
Easy UI design: Creating the game interface is simple and intuitive with Flutter’s widget system.
Setting Up a Flutter Project
To get started, you’ll need to set up a Flutter project. Follow these steps:
Install Flutter SDK from the official Flutter website.
Create a new Flutter project by running:
flutter create tic_tac_toe
Navigate to the project folder:
cd tic_tac_toe
Open the project in your favorite IDE (VS Code, Android Studio, etc.).
Basic Dependencies
To manage the game state, we will be using Flutter’s built-in StatefulWidget.
dependencies:
flutter:
sdk: flutter
Implementing the Tic Tac Toe Game Logic
Start by setting up a simple UI for the Tic Tac Toe board using a 3x3 grid. Here’s an example of how to create the grid:
import 'package:flutter/material.dart';
void main() => runApp(TicTacToe());
class TicTacToe extends StatefulWidget {
@override
_TicTacToeState createState() => _TicTacToeState();
}
class _TicTacToeState extends State<TicTacToe> {
// Define variables for the board and the current player
List<List<String>> board;
String currentPlayer;
@override
void initState() {
super.initState();
_initializeBoard();
}
void _initializeBoard() {
board = List.generate(3, (_) => List.filled(3, ''));
currentPlayer = 'X';
}
// UI implementation
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Tic Tac Toe with Minimax'),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
for (int i = 0; i < 3; i++)
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
for (int j = 0; j < 3; j++)
_buildCell(i, j),
],
),
],
),
);
}
Widget _buildCell(int row, int col) {
return GestureDetector(
onTap: () {
_handleMove(row, col);
},
child: Container(
width: 100,
height: 100,
margin: EdgeInsets.all(4.0),
decoration: BoxDecoration(
border: Border.all(),
),
child: Center(
child: Text(
board[row][col],
style: TextStyle(fontSize: 48.0),
),
),
),
);
}
void _handleMove(int row, int col) {
// Handle move and check for win
}
}
Game Logic
Initialize a 2D array to represent the board.
Implement the
handleMove()
function to alternate between player and AI moves.
Using Minimax Algorithm with Alpha-Beta Pruning
The core part of the unbeatable Tic Tac Toe AI is the Minimax Algorithm with Alpha-Beta Pruning. Let’s dive into the implementation.
int minimax(List<List<String>> board, int depth, bool isMax, int alpha, int beta) {
int score = evaluateBoard(board);
// Base case: return score if game is over
if (score == 10 || score == -10 || isDraw(board)) {
return score;
}
if (isMax) {
int best = -1000;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (board[i][j] == '') {
board[i][j] = 'X';
best = max(best, minimax(board, depth + 1, false, alpha, beta));
board[i][j] = '';
alpha = max(alpha, best);
if (beta <= alpha) break;
}
}
}
return best;
} else {
int best = 1000;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (board[i][j] == '') {
board[i][j] = 'O';
best = min(best, minimax(board, depth + 1, true, alpha, beta));
board[i][j] = '';
beta = min(beta, best);
if (beta <= alpha) break;
}
}
}
return best;
}
}
Explanation of the Code
The AI calculates the best possible move using Minimax and reduces the number of evaluations with Alpha-Beta Pruning.
If the board is in a winning or losing state, the function returns a score.
The algorithm recursively simulates all possible moves and assigns a score based on outcomes.
Optimizing the Game for Performance
Here are a few tips to optimize the game for performance:
Cache Results: Store game states that have already been evaluated to avoid redundant calculations.
Move Ordering: Consider evaluating likely winning moves first to allow Alpha-Beta Pruning to cut off unneeded branches faster.
User Interface Optimization: Make sure the UI is updated efficiently by using Flutter's built-in mechanisms like
setState()
andAnimatedBuilder
for smooth transitions.
Conclusion
Developing an unbeatable Tic Tac Toe game using the Minimax Algorithm with Alpha-Beta Pruning is a fantastic way to explore game development and AI in Flutter. The inclusion of Alpha-Beta Pruning ensures the game runs quickly and efficiently, making it perfect for real-time gameplay. By implementing this, you'll create an AI that is impossible to defeat while enhancing your knowledge of algorithms and Flutter development.
Ready to take on the challenge and build your unbeatable Tic Tac Toe game? If you're looking for expert assistance, be sure to hire Flutter developers to bring your game to life!
Subscribe to my newsletter
Read articles from AddWeb Solution directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

AddWeb Solution
AddWeb Solution
Outcome-driven IT development, consulting, and outsourcing company, specializing in Web/Mobile App Development. For the past 10+ years, we have thrived by ‘adding’ value to the ‘web’ world with our timely and quality ‘solutions’. Our IT solutions help startups grow, improve the reach of medium-sized businesses, and help larger ventures make deeper connections. AddWeb Solution is consistently sloping upwards, providing flawless solutions, timely deliveries, and boosting overall productivity by ensuring maximum ROI. We are really proud of building great products for world-class brands. We are a professional and friendly team with experience ranging from 2 to 16 years. With more than 500+ successful projects delivered, we are ready to take it to the next height.