Getting Started with Go: A Beginner's Guide
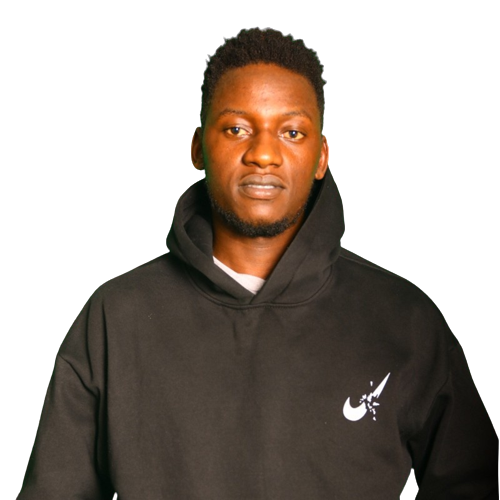
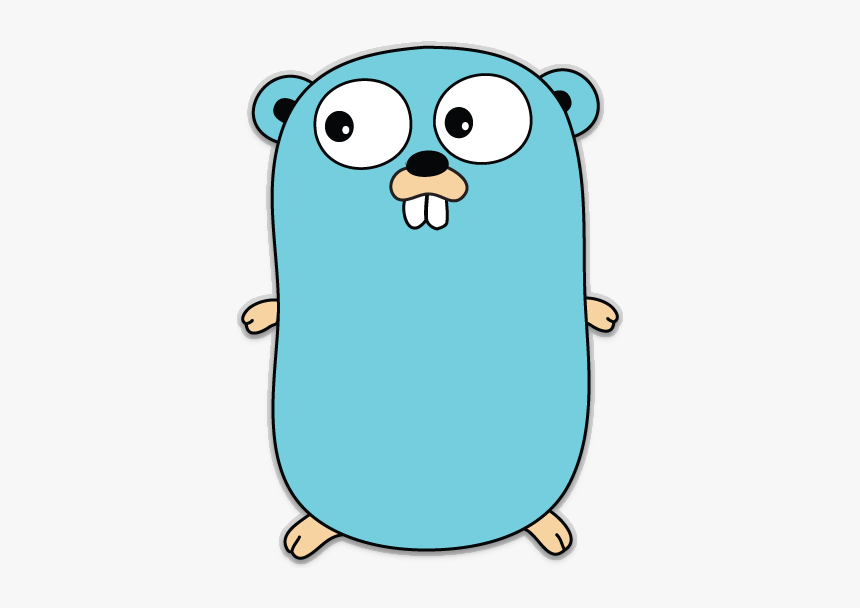
Into
Go (or Golang) has gained immense popularity for its simplicity, strong performance, and excellent support for concurrent programming. Let's dive into the basics of this powerful language.
Setting Up Your Environment
First, download Go from the official website (golang.org) and install it. To verify your installation, open a terminal and run:
go version
Your First Go Program
Let's start with the classic "Hello, World!" program. Create a file named hello.go
:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Let's break down the key elements:
package main
: Every Go file starts with a package declarationimport "fmt"
: Imports the formatting package for input/outputfunc main()
: The entry point of our program
Variables and Data Types
Go offers several ways to declare variables:
// Explicit type declaration
var name string = "John"
// Type inference
age := 25
// Multiple declarations
var (
firstName string = "Jane"
lastName string = "Doe"
isActive bool = true
)
Control Structures
Go's if statements and loops are straightforward:
// If statement
if age >= 18 {
fmt.Println("Adult")
} else {
fmt.Println("Minor")
}
// For loop (Go's only loop construct)
for i := 0; i < 5; i++ {
fmt.Println(i)
}
// While-style loop
count := 0
for count < 5 {
fmt.Println(count)
count++
}
Functions
Functions in Go can return multiple values, which is quite unique:
func calculateStats(numbers []int) (min int, max int) {
min = numbers[0]
max = numbers[0]
for _, num := range numbers {
if num < min {
min = num
}
if num > max {
max = num
}
}
return min, max
}
func main() {
nums := []int{2, 4, 6, 8, 10}
minimum, maximum := calculateStats(nums)
fmt.Printf("Min: %d, Max: %d\n", minimum, maximum)
}
Working with Collections
Go provides several built-in collection types:
// Arrays (fixed length)
var colors [3]string
colors[0] = "Red"
colors[1] = "Green"
colors[2] = "Blue"
// Slices (dynamic length)
fruits := []string{"Apple", "Banana", "Orange"}
fruits = append(fruits, "Mango")
// Maps
ages := map[string]int{
"Alice": 25,
"Bob": 30,
"Carol": 35,
}
Next Steps
You can read more about Go here
If you need a more hands-on approach, check out the Tour of Go
Subscribe to my newsletter
Read articles from Enock Omondi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
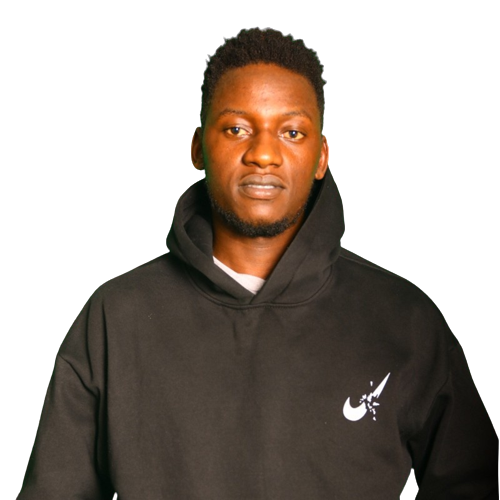
Enock Omondi
Enock Omondi
Software Engineer