Mastering JavaScript Loops: A Complete Guide for Software Engineers
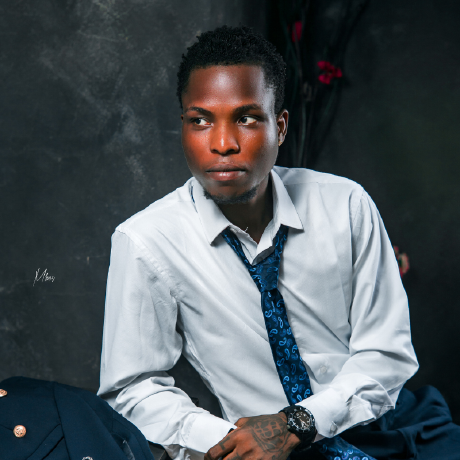
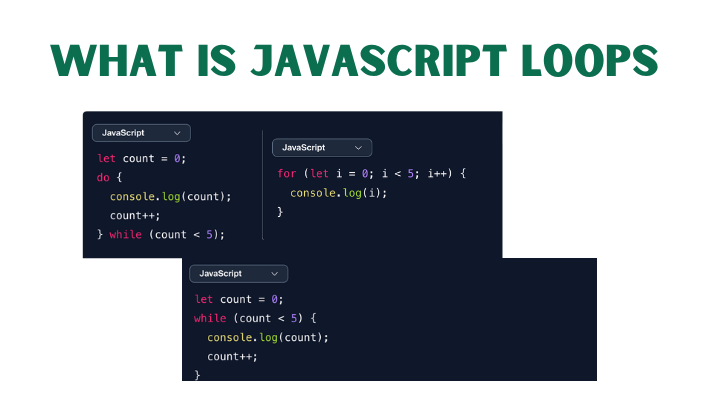
Loops are essential tools in JavaScript that enable developers to efficiently perform repetitive tasks. Understanding how loops operate and when to use them is vital for any software engineer. In this article, we will explore the different types of loops, their syntax, and practical applications.
1. What Are JavaScript Loops?
Loops in JavaScript allow you to execute a block of code repeatedly, either a set number of times or until a condition is met. Loops help in situations where you need to run the same operation multiple times, such as iterating through an array or executing a function until a condition becomes false.
2. Types of Loops in JavaScript
JavaScript supports several types of loops, each suited for different scenarios:
for
Loop: Ideal when you know the number of iterations in advance.while
Loop: Useful when the number of iterations is unknown, but the loop should continue until a certain condition is false.do...while
Loop: Similar towhile
, but guarantees at least one iteration.for...in
Loop: Used for iterating over the properties of an object.for...of
Loop: Used for iterating over iterable objects like arrays, strings, and more.
3. Understanding the for
Loop
The for
loop is one of the most common loops in JavaScript. It allows you to iterate over a block of code a set number of times.
Syntax:
for (let i = 0; i < 5; i++) {
console.log(i);
}
This loop prints numbers from 0 to 4. It's best used when the number of iterations is predetermined.
4. Using the while
Loop
The while
loop runs a block of code as long as a specified condition is true. It checks the condition before each iteration, making it suitable when you don’t know how many times the loop will run.
Syntax:
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
5. Exploring the do...while
Loop
The do...while
loop is similar to the while
loop but with one key difference: it ensures that the loop body runs at least once before checking the condition.
Syntax:
let count = 0;
do {
console.log(count);
count++;
} while (count < 5);
6. for...in
and for...of
Loops
for...in
Loop:
The for...in
loop is used to iterate over the properties of an object.
Example:
let person = { name: "John", age: 30 };
for (let key in person) {
console.log(key + ": " + person[key]);
}
for...of
Loop:
The for...of
loop is used to iterate over iterable objects like arrays or strings.
Example:
let arr = [1, 2, 3];
for (let value of arr) {
console.log(value);
}
7. The JavaScript Event Loop
JavaScript is single-threaded, meaning it can only execute one operation at a time. The event loop is responsible for managing asynchronous operations, such as setTimeout
, promises, and event handlers. It continuously checks the call stack and callback queue, ensuring non-blocking behavior in JavaScript.
8. Common Use Cases for Loops in JavaScript
Loops are essential for:
Iterating through arrays or objects.
Automating repetitive tasks.
Manipulating data structures.
Handling asynchronous operations using loops and callbacks.
9. Looping Through Arrays Using forEach
The forEach
method is a built-in array method in JavaScript that allows you to loop through the elements of an array and execute a function for each element.
Example:
let arr = [1, 2, 3];
arr.forEach(function(item) {
console.log(item);
});
forEach
is particularly useful when you need to apply a function to each element of an array.
10. Looping in Real Projects
In real-world projects, loops are used in various scenarios, from rendering lists of items in UI to processing data and making repeated API calls. Understanding loops and their variants is key to writing efficient and maintainable code.
Conclusion
JavaScript loops are a powerful tool for automating repetitive tasks and handling data efficiently. Whether you're working with arrays, objects, or asynchronous operations, mastering loops is an essential skill for any software engineer.
Further Resources on JavaScript Loops
W3Schools: JavaScript Loops – A detailed guide on loops and their applications.
MDN Web Docs: Loops and Iteration – Comprehensive documentation on JavaScript loops.
FreeCodeCamp: JavaScript Loops – Free resources to learn and practice loops in JavaScript.
Day 3 Blog Post: JavaScript Arrays – Learn how to work with arrays, another core concept in JavaScript.
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
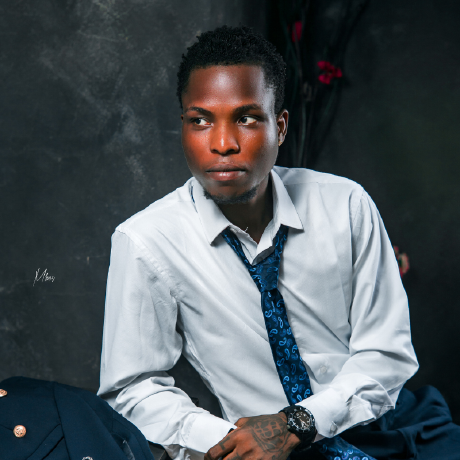
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com