5-Minute Coffee Tip #1: Convert a PNG to ICO with Pillow
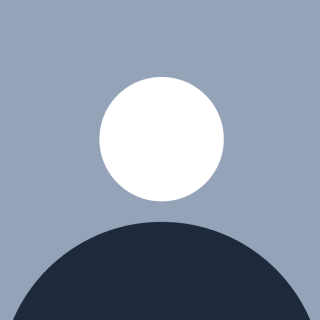
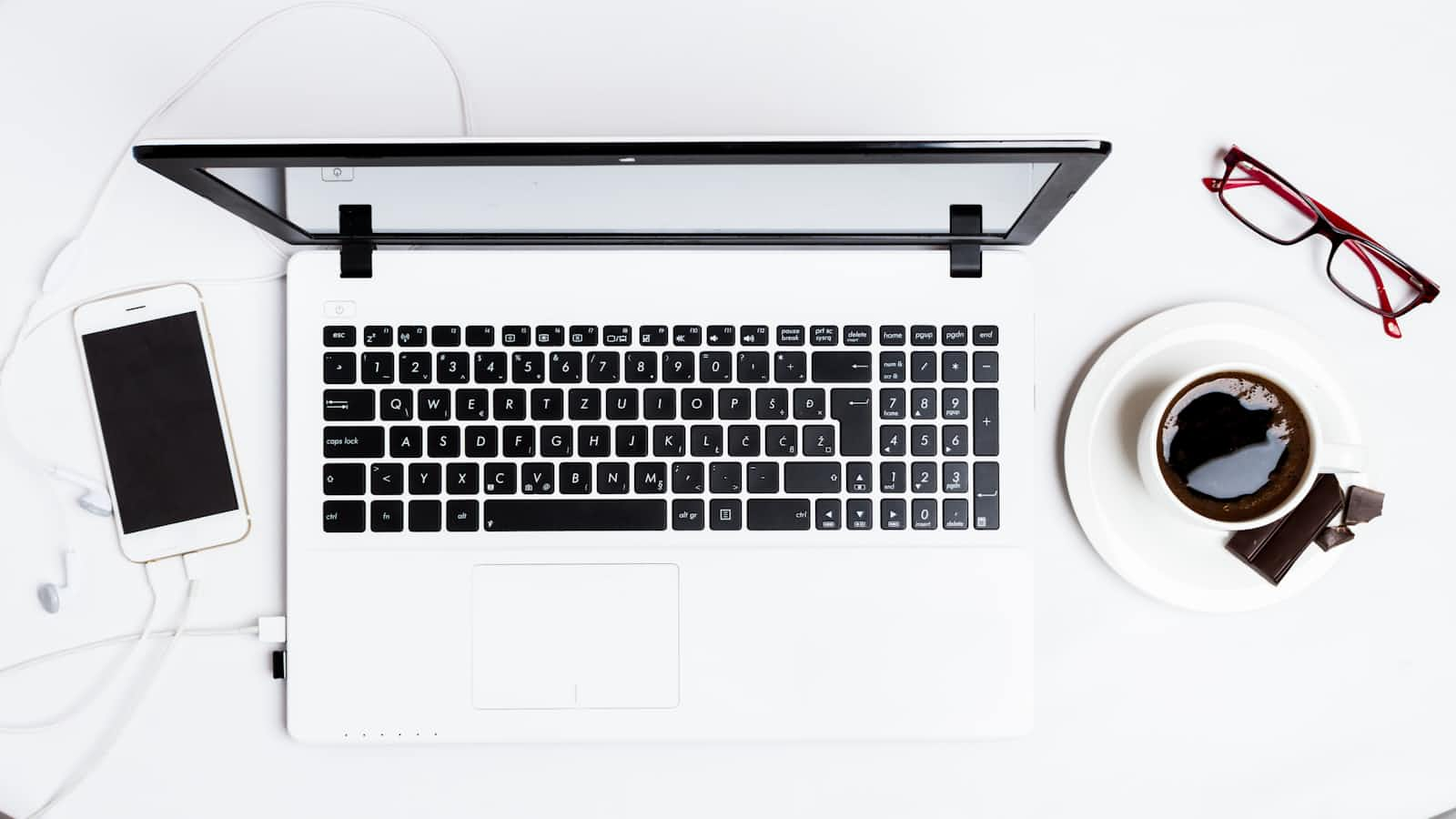
In the world of digital design, icons are super important for making user interfaces look great and for sharing information quickly.
ICO or Icon files are popular for displaying icons on Windows and are also used to add a logo to a website's browser tab, known as a favicon.
They can hold several images at different resolutions, so icons always look sharp on any screen. In this blog post, we'll dive into how to create ICO files from PNG images using the Python Pillow package.
Introduction to Pillow
Pillow is a super handy fork of the Python Imaging Library (PIL) that makes it easy to open, tweak, and save different image file formats.
It's a go-to tool for image stuff like resizing, cropping, and adding filters. Plus, it has this cool feature where you can create ICO files without any hassle.
Step 0: Install Pillow
Before we begin, make sure that you have Pillow installed. You can install it using pip (in your virtual environment, of course):
pip install pillow
Step 1: Import Pillow
First, let’s import the necessary module from Pillow:
from PIL import Image
Step 2: Load the PNG Image
To create an ICO file, load the PNG image using Pillow’s Image.open
()
method. Suppose you have a PNG file named "icon.png" in the same directory as your script. You can load it like this:
img_to_convert = Image.open("icon.png")
or if you would like to pass the raw path:
image_to_convert = Image.open(r"path_to_the_file\icon.png")
Step 3: Create the ICO File
Now that we've got our image ready, let's go ahead and create an ICO file. We'll use the Image.save
()
method for this and set the file format to ".ico". We can also include a list of sizes (in pixels) to cover different icon resolutions.
# Use the default
image_to_convert.save("favicon.ico")
# OR use some more fine-grained options for your icon
img.save('favicon.ico', sizes=[(48, 48)], format="ICO", quality=90)
Step 4: Verify the Result
To make sure the ICO file was created without any hiccups, you can open it with Pillow and take a look at its properties. Here's how you do it:
ico_image = Image.open("favicon.ico")
print("ICO Image Format:", ico_image.format)
Conclusion
In this tutorial, we learned how to create ICO files from PNG images using the Pillow package in Python.
Pillow makes it super easy to convert a PNG image and save it as an ICO file.
Here’s the full code as a function:
from PIL import Image
# Optionally, you may specify the icon sizes you want
icon_sizes = [(16, 16), (32, 32), (48, 48), (64, 64), (128, 128), (255, 255)]
# Otherwise, the default is 48x48 pixels
def convert_png_to_ico(image_filename, sizes=[(48, 48)]):
img = Image.open(image_filename)
img.save('icon.ico', sizes=sizes, format="ICO",quality=90)
convert_png_to_ico('Logo_500x500.png')
Whether you're a graphic designer or a developer, knowing how to turn PNGs into ICO files with Pillow is a handy skill.
It lets you customize and create icons that fit your app's or website’s needs, boosting its look and user experience.
Now that you've got the basics of making ICO files with Pillow down, feel free to dive deeper by trying out different image sizes, adding transparency, or crafting more complex icons.
With Pillow's wide range of features, the sky's the limit, and you can really elevate your icon design game while you tinker with Python.
Now… take that well deserved, self-satisfied sip of coffee. ☕️
Subscribe to my newsletter
Read articles from Shani Rivers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shani Rivers
Shani Rivers
I'm a data enthusiast with web development and graphic design experience. I know how to build a website from scratch, but I sometimes work with entrepreneurs and small businesses with their Wix or Squarespace sites, so basically I help them to establish their online presence. When I'm not doing that, I'm studying and blogging about data engineering, data science or web development. Oh, and I'm wife and a mom to a wee tot.