Day 34 Guide: Mastering Object-Oriented Programming in Python

Table of contents
- Introduction :
- Python's Object Oriented Programming System (OOPs)
- What is Object:
- Syntax to create object:
- What is Reference Variable:
- Program:
- Write a Python program to create a Student class and Creates an object to it. Call the method talk() to display student details.
- Self variable:
- Note:
- Challenges :
- Resources :
- Goals for Tomorrow :
- Conclusion :
- Connect with me :
- Join the conversation :
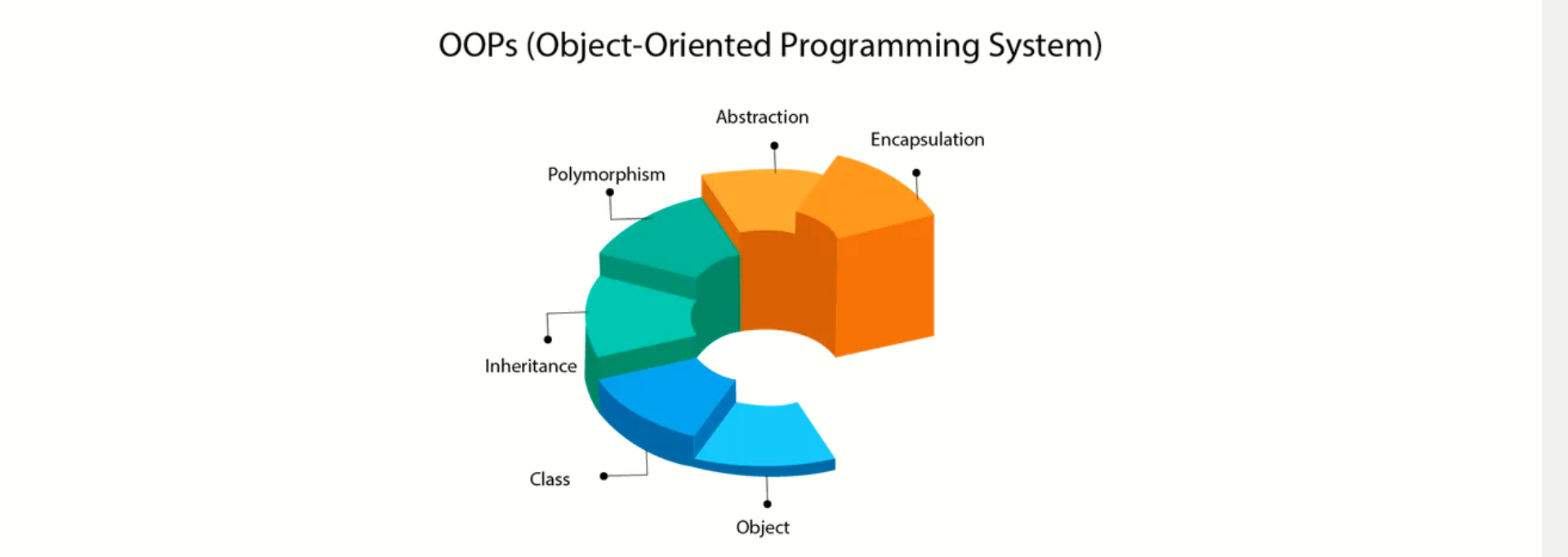
Introduction :
Welcome back to my Python journey! Yesterday, I laid the foundation with MongoDB database in python.
Today, I dove into OOPs concept in python, Let's explore what I learned!
Python's Object Oriented Programming System (OOPs)
What is Class:
In Python every thing is an object.
To create objects we required some Model or Plan or Blue print, which is nothing but class.
We can write a class to represent attributes and behaviour of object.
Attributes can be represented by variables Behaviour can be represented by Methods.
Hence class contains both variables and method
How to Define a class ?
We can define a class by using class keyword.
Syntax:
class className:
''' documentation string '''
variables: instance variables, static and local variables
methods: instance methods, static methods, class methods
Note:
Documentation string represents description of the class. Within the class doc string is always optional.
We can get doc string by using the following 2 ways.
1. print(classname.__doc__) (double underscore)
2. help(classname)
Example :
class Student:
''''' This is student class with required data'''
print(Student.__doc__) (double underscore)
help(Student)
Within the Python class we can represent data by using variables.
There are 3 types of variables are allowed.
1. Instance Variables (Object Level Variables)
2. Static Variables (Class Level Variables)
3. Local variables (Method Level Variables)
Within the Python class, we can represent operations by using methods.
The following are various types of allowed methods.
1. Instance Methods
2. Class Methods
3. Static Methods
Example for class:
class Student:
'''''Developed by jithon for python demo'''
def __init__(self):
self.name='jithon'
self.age=30
self.marks=80
def talk(self):
print("Hello I am :",self.name)
print("My Age is:",self.age)
print("My Marks are:",self.marks)
What is Object:
Physical existence of a class is nothing but object.
We can create any number of objects for a class.
Syntax to create object:
referencevariable = classname()
Example: s = Student()
What is Reference Variable:
The variable which can be used to refer object is called reference variable.
By using reference variable, we can access properties and methods of object.
Program:
Write a Python program to create a Student class and Creates an object to it. Call the method talk() to display student details.
class Student:
def __init__(self,name,rollno,marks):
self.name=name
self.rollno=rollno
self.marks=marks
def talk(self):
print("Hello My Name is:",self.name)
print("My Rollno is:",self.rollno)
print("My Marks are:",self.marks)
s1=Student("jithon",101,80)
s1.talk()
Self variable:
self is the default variable which is always pointing to current object (like this keyword in Java)
By using self we can access instance variables and instance methods of object.
Note:
1. self should be first parameter inside constructor
def __init__(self):
2. self should be first parameter inside instance methods
def talk(self):
Challenges :
Understanding python databases.
Handling errors.
Resources :
Official Python Documentation: python OOPs concept
W3Schools' Python Tutorial: python OOPs concept
Scaler's Python Courses : python OOPs concept
Goals for Tomorrow :
- Explore something more on OOPs.
Conclusion :
Day 34’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ linkedin.com/in/archana-prusty-4aa0b827a ]
twitter : [ https://x.com/_archana77 ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.