Day 5 Task: Advanced Linux Shell Scripting for DevOps Engineers with User Management
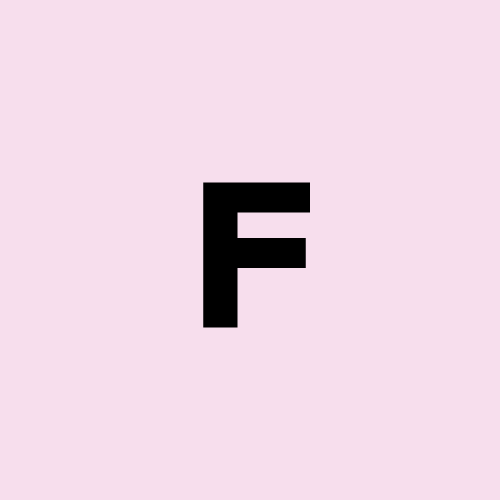
Table of contents
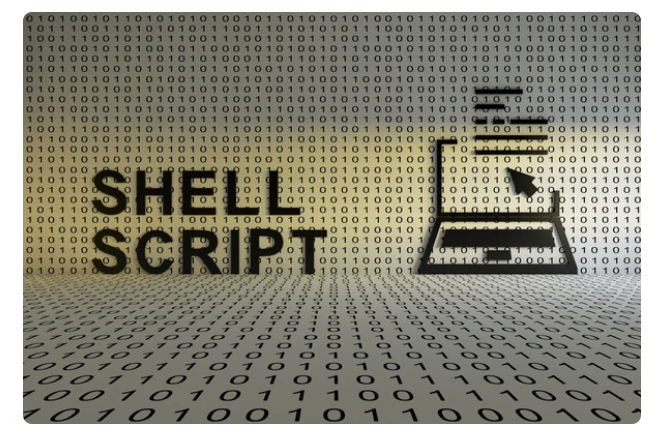
Create Directories Using Shell Script
Create a Script to Backup All Your Work
Read About Cron and Crontab to Automate the Backup Script
Read About User Management
1. Create Directories Using Shell Script
You need a script called createDirectories.sh
that takes three arguments: a directory name prefix, a starting number, and an ending number. Here’s how you can write that script:
#!/bin/bash
# Check if the correct number of arguments is provided
if [ "$#" -ne 3 ]; then
echo "Usage: $0 <directory_name> <start_number> <end_number>"
exit 1
fi
dir_name=$1
start_num=$2
end_num=$3
# Create directories using a loop
for (( i=start_num; i<=end_num; i++ ))
do
mkdir "${dir_name}${i}"
done
echo "Directories created from ${dir_name}${start_num} to ${dir_name}${end_num}."
Usage:
Make it executable chmod +x createDirectories.sh
To create directories from day1 to day90:
./createDirectories.sh day 1 90
To create directories from Movie20 to Movie50:
./createDirectories.sh Movie 20 50
2. Create a Script to Backup All Your Work
Here's an example backup script named backup.sh
. This script creates a backup of a specified directory to a backup location:
#!/bin/bash
# Variables
SOURCE_DIR="$1" # Directory to backup
BACKUP_DIR="$2" # Backup destination
# Check if the correct number of arguments is provided
if [ "$#" -ne 2 ]; then
echo "Usage: $0 <source_directory> <backup_directory>"
exit 1
fi
# Create backup directory if it doesn't exist
mkdir -p "$BACKUP_DIR"
# Create a timestamp
TIMESTAMP=$(date +"%Y%m%d_%H%M%S")
# Backup using tar
tar -czf "$BACKUP_DIR/backup_$TIMESTAMP.tar.gz" "$SOURCE_DIR"
echo "Backup of $SOURCE_DIR completed at $BACKUP_DIR/backup_$TIMESTAMP.tar.gz."
Usage: To run the backup script:
./backup.sh /path/to/source /path/to/backup
To automate the backup script using cron, you would do the following:
A crontab command allows users to submit, edit, or delete entries to cron. A crontab file is a user file that holds the scheduling information.
Cron is a job scheduler utility, In simple terms it executes described commands/scripts at a particular predefined interval.
crontab is a table in which we maintain all the cron jobs that we need to execute in our system.
command to check the crontab list:
crontab -l
create a new cron job and when you run this command the first time it will ask you to select an editor like nano, vim, etc, and then select the editor as per your choice and add a new line in the file:
Open the crontab configuration for editing:
crontab -e
Add a cron job entry. For example, to run the backup script every day at 2 AM:
0 2 * * * /path/to/backup.sh /path/to/source /path/to/backup
4. Create 2 Users and Display Their Usernames
You can create users using the useradd
command. Here’s how you can create a simple script createUsers.sh
to create two users:
#!/bin/bash
# Creating two users
useradd user1
useradd user2
# Display the usernames
echo "Created users:"
echo "User 1: user1"
echo "User 2: user2"
Usage: To create the users, run:
./createUsers.sh
Summary
createDirectories.sh
: Creates directories with a specified prefix and range.backup.sh
: Backups a specified directory to a designated backup location.Cron: Automates the backup process by scheduling it using crontab.
createUsers.sh
: Creates two users and displays their usernames
We appreciate❤️ you taking the time to read and connect with us! Your engagement means a lot to us, and we look forward to hearing more from you📝
Subscribe to my newsletter
Read articles from Fauzeya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
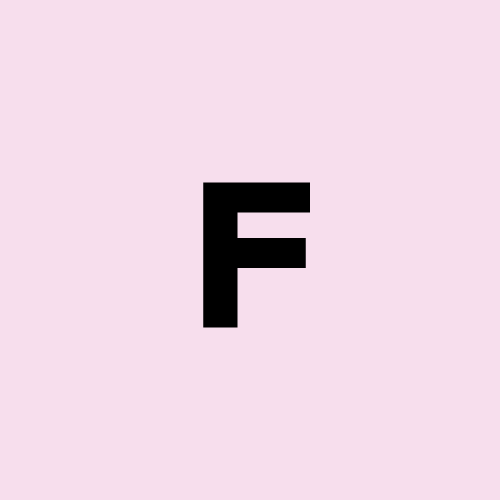
Fauzeya
Fauzeya
Hi there! I'm Fauzeya 👩💻, a passionate DevOps Engineer with a background in Computer Science Engineering🎓. I’m committed to enhancing security🔒, efficiency⚙️, and effectiveness in software development and deployment processes. With extensive knowledge in cloud computing☁️, containerization📦, and automation🤖, I aim to stay updated with the latest tools and methodologies in the DevOps field. Currently, I’m on a journey to deepen my understanding of DevOps I enjoy sharing my learning experiences and insights through my blog, 📝where I cover topics related to DevOps practices, tutorials, and challenges. I believe in continuous growth and learning and am excited to connect with fellow tech enthusiasts and professionals🤝. Let’s embark on this journey together!🚀