Unlocking the Power of JSON: Tips and Tricks for Developers

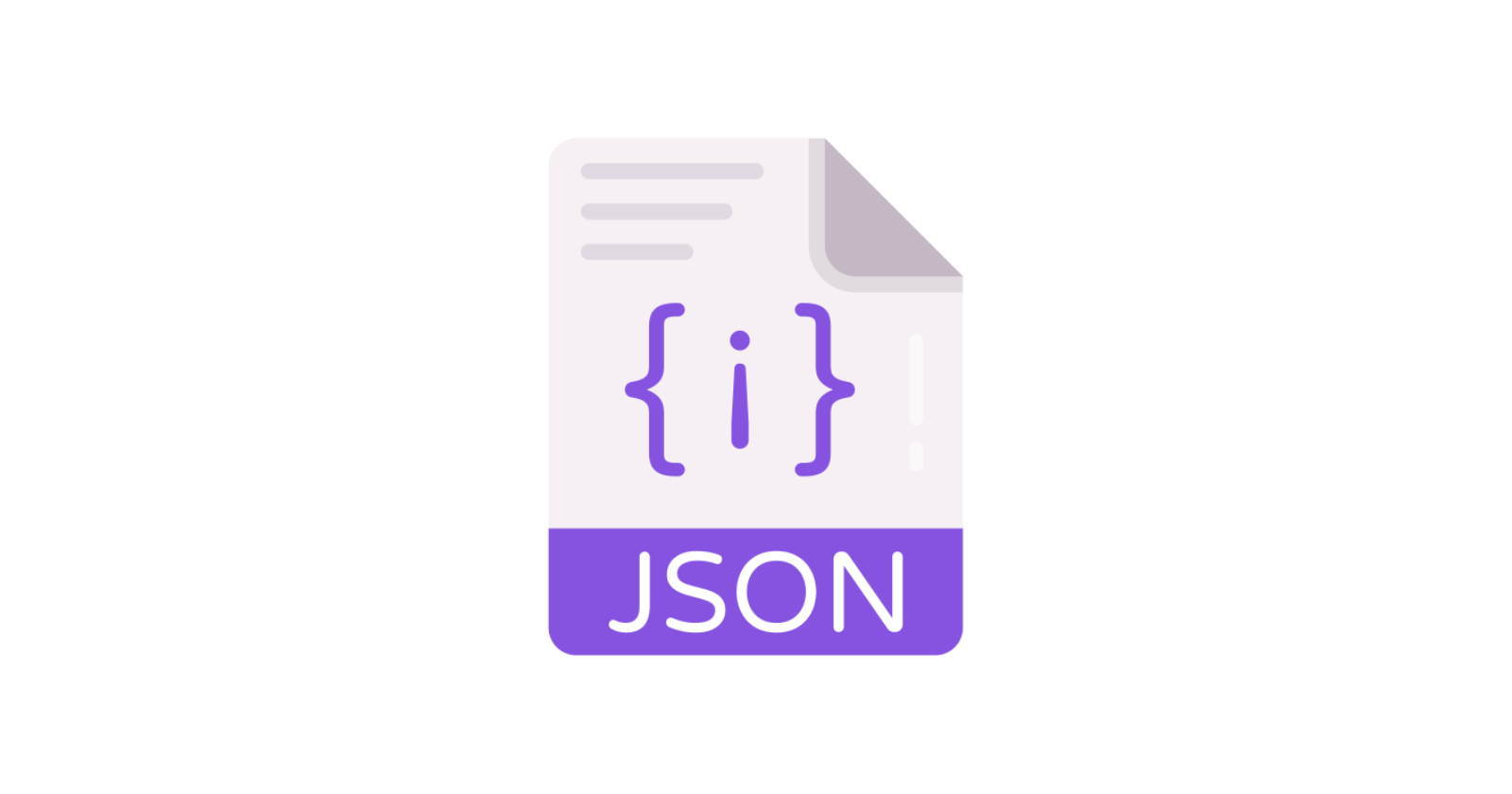
What is JSON?
Definition: JSON is a format for representing structured data as text, using key-value pairs.
Origins: Derived from JavaScript, but now language-independent.
File Extension:
.json
Media Type:
application/json
History of JSON
American computer programmer Douglas Crockford created JSON in the early 2000s. The format is derived from the JavaScript programming language standard and follows JavaScript object syntax.
JSON Syntax Rules
Keys must always be strings, enclosed in double quotes.
Values can be strings, numbers, objects, arrays, booleans, or null.
Commas separate key-value pairs or array elements.
Whitespace is allowed but ignored.
Example of JSON
{
"name": "Alice",
"age": 25,
"isStudent": false,
"hobbies": ["reading", "music"],
"address": {
"city": "San Francisco",
"state": "CA"
},
"middleName": null
}
Advantages of JSON
Human-Readable:
Easy to understand and write.
Facilitates debugging and troubleshooting.
Lightweight:
Smaller file sizes.
Faster transmission and reduced network bandwidth.
Improved application performance.
Versatile:
Widely supported by various programming languages and platforms.
Suitable for data exchange, configuration files, and data storage.
Easy to Parse and Generate:
Efficient parsing and generation.
Simplifies data manipulation and processing.
Platform-Independent:
- Compatible across different systems and environments.
Flexible Structure:
Adaptable to changing data requirements.
Supports dynamic and evolving data models.
Secure:
Minimal security vulnerabilities.
Suitable for transmitting sensitive data.
Supported Data Types
JSON supports the following data types:
Number
: Integers and floating-point numbers.String
: Sequences of characters enclosed in double quotes"
.Boolean
:true
orfalse
.Array
: Ordered collections of values enclosed in square brackets.Object
: Unordered collections of key-value pairs enclosed in curly braces.null
: Represents the absence of a value.
Parsing JSON
To work with JSON data, you typically need to parse it into a data structure that your programming language can understand. Most programming languages provide libraries or built-in functions for parsing JSON.
Build-in Method in languages:
JSON.parse()
⇒ JavaScriptjson.loads()
⇒ Pythonjson_decode()
⇒ PHP// Parsing JSON string to JavaScript object const jsonString = '{"name": "John", "age": 30}'; const obj = JSON.parse(jsonString);
Generating or Creating JSON
You can create JSON objects in your programming language by constructing data structures and then converting them to JSON using appropriate methods.
Build-in Method in languages:
JSON.stringify()
⇒ JavaScriptjson.dumps()
⇒ Pythonjson_encode()
⇒ PHPconst obj = { name:"John", age : 30 } // Stringifying JavaScript object to JSON string const jsonStringified = JSON.stringify(obj);
this method doesn't work with non-serializable data (like functions, undefined, or circular references).
Common Use Cases of JSON
API Communication: JSON is widely used for exchanging data between web applications and APIs.
Data Storage: JSON can be used to store data in files or databases.
Configuration Files: JSON is often used for storing configuration settings in applications.
Logging: Many applications log data in JSON format for easy parsing and analysis.
JSON in NoSQL Databases
Many NoSQL databases, such as MongoDB and CouchDB, use JSON (or its binary equivalent, BSON) as their primary data storage format. Understanding JSON is crucial when working with these databases, as it defines the way data is stored and queried.
JSON vs XML
Feature | JSON | XML |
Syntax | Simpler, lightweight | Verbose, uses start/end tags |
Data types | Supports string, number, boolean | Everything is text |
Readability | Easier for humans to read | More difficult to read |
Performance | Typically faster to parse | Slower to parse |
Schemas | JSON Schema | XML Schema |
Security Concerns
Injection attacks: Be careful when parsing JSON, as malformed JSON could potentially be used for injection attacks.
Cross-Site Scripting (XSS): If not properly handled, JSON data can be a vector for XSS attacks.
Mitigation: Always validate and sanitize JSON data before use, especially when dealing with external inputs.
JSON Formatter/Validator
Tools like jsonlint.com can help validate and format JSON.
JSON Schema
JSON Schema is a way to describe the structure of a JSON document, enabling validation of data. It provides a way to validate JSON documents against a predefined set of rules, ensuring data consistency, integrity, and interoperability.
{
"$schema": "http://json-schema.org/draft-07/schema#",
"title": "Person",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"age": {
"type": "integer",
"minimum": 0
}
},
"required": ["name", "age"]
}
In conclusion, mastering JSON is a valuable skill for any developer. By understanding its syntax, parsing and generating JSON data, and applying advanced techniques, you can efficiently handle data interchange in your projects. Now that you have these tools at your disposal, start experimenting with JSON in your own applications. If you found this guide helpful, don’t forget to share it with your fellow developers and leave a comment below with your thoughts or questions!
Subscribe to my newsletter
Read articles from Md.Rejoyan Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Md.Rejoyan Islam
Md.Rejoyan Islam
I’m Md Rejoyan Islam, a full-stack web developer with a passion for creating impactful digital experiences. Proficient in JavaScript, Python, React.js, Next.js, Node.js, and both SQL and NoSQL databases. Always eager to learn and adapt to new technologies.