Mastering File System Operations in NodeJs: Reading and Writing to Files
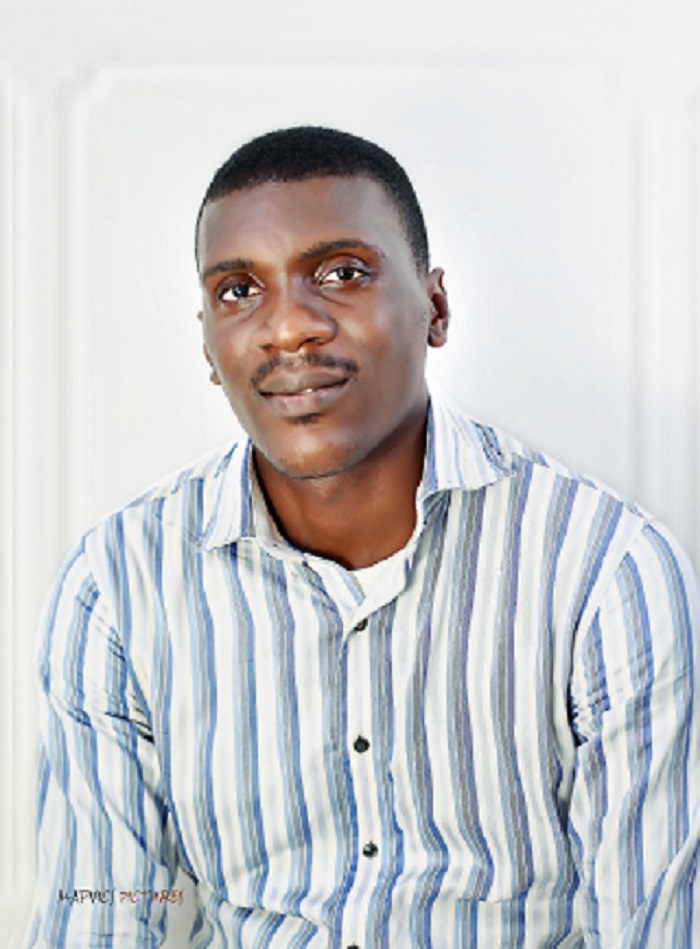
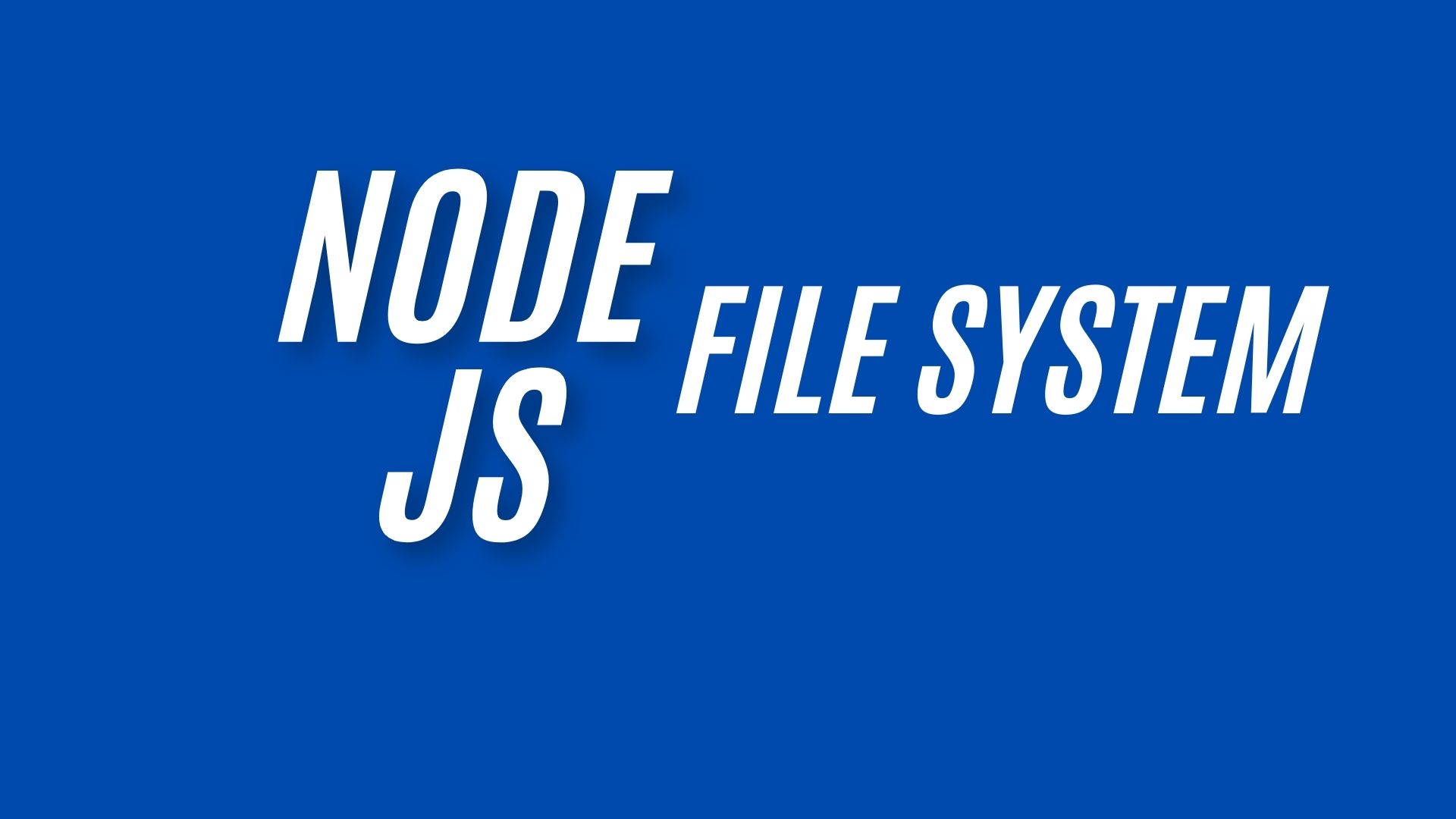
Introduction
Reading and writing to files in Nodejs can be done in two ways: asynchronous and synchronous. Nodejs is asynchronous by default. A file system is a strategy that an operating system uses to organize and handle files on storage devices in a computer. It helps to determine the storage of data, how it is named, and how it can be accessed. A file system helps to structure data into files and directories.
In this article, I will discuss these two major processes of carrying out file system operations in Nodejs and demonstrate some examples. In the end, you will be able to understand the difference between each of these methods and how to use them.
Reading a file asynchronously
When you open a file, you immediately have access to its location and file statistics, such as size and extension, and you can conveniently get its content. One of the major pointers in reading files asynchronously is that the asynchronous method follows the nonblocking pattern in reading files and uses a callback. This function only gets executed after the main function.
const fs = require(“fs”)
const path = require(“path”)
const filePath = path.join(__dirname, “files”, “filename.txt”)
fs.open(filePath, ‘r’, (err, fileDescriptor) => {
if(err) {
console.log(`An error occurred while opening the file: ${err}`)
return
}
fs.readFile(fileDescriptor, ‘utf8’, (err, data) => {
if(err) {
console.log(`An error occurred while opening the file: ${err}`)
return
}
console.log(data)
})
})
In the above code sample, the file system module and the path module were imported, which enables access to file directories.
Calling the open method will request the file path and the mode in which the file should be opened. After the main function is executed, a callback function will be executed. The open method returns a file handler or descriptor that is passed to the read function to get the file's content and an encoding scheme for how the file is saved.
Reading a file synchronously
The readFileSync is a blocking operation that waits for the file to be read.
const fs = require(“fs”)
const path = require(“path”)
const filePath = path.join(__dirname, “files”, “filename.txt”)
const newFile = fs.openSync(filePath, ‘r’ )
const newFileData = fs.readFileSync(newFile, ‘utf8’ )
console.log(newFileData)
Writing to files
When writing to files, you are either adding to an already existing file or writing new content into the file. When an entirely new content is written into an existing file, it will overwrite the content initially present. If the file path does not exist for this new content, it will be created. The append mode is used to add more text to the new lines of a file.
Synchronous method
const fs = require(“fs”)
const path = require(“path”)
const textFilePath = path.join(__dirname, “files”, “newFile.txt”)
const content = “File is written in a synchronous method”
fs.writeFileSync(textFilePath, content)
console.log(“File is written successfully”)
Asynchronous method
const fs = require(“fs”)
const path = require(“path”)
const textFilePath = path.join(__dirname, “files”, “newFile.txt”)
const content = “New text is written into this new file”
fs.writeFile(textFilePath, content ,(err) => {
if(err) {
console.log(err)
return
}
console.log(“File is successfully written”)
} )
Appending to a file
const fs = require(“fs”)
const path = require(“path”)
const textFilePath = path.join(__dirname, “files”, “newFile.txt”)
const newContent = “\nThis new text is written into this file and added to a new of the file”
fs.appendFile(textFilePath, newContent ,(err) => {
if(err) {
console.log(err)
return
}
console.log(“File is appended successfully”)
} )
Conclusion
In summary, this article briefly discusses how the file system and path module are used in order to read and write a file in Nodejs, the two processes of reading and writing to a file, which are synchronous and asynchronous. The asynchronous uses a callback and it is nonblocking while the synchronous is a blocking method and hence does not use a callback.
Subscribe to my newsletter
Read articles from Adebayo Otomuola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
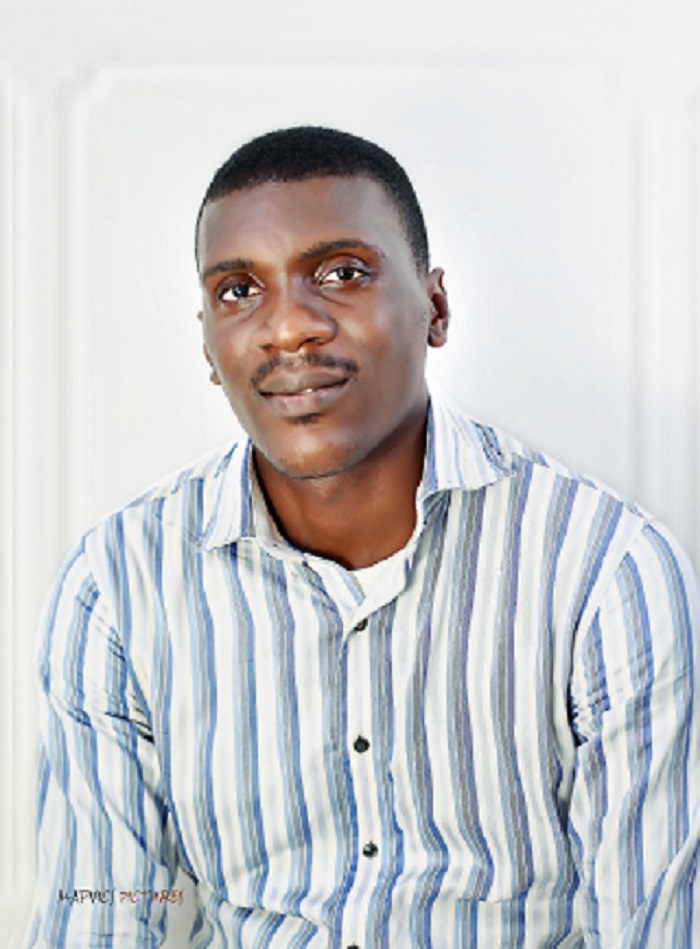
Adebayo Otomuola
Adebayo Otomuola
I'm a backend engineer and technical writer.