Mastering Memory Management in JavaScript: A Deep Dive into Garbage Collection

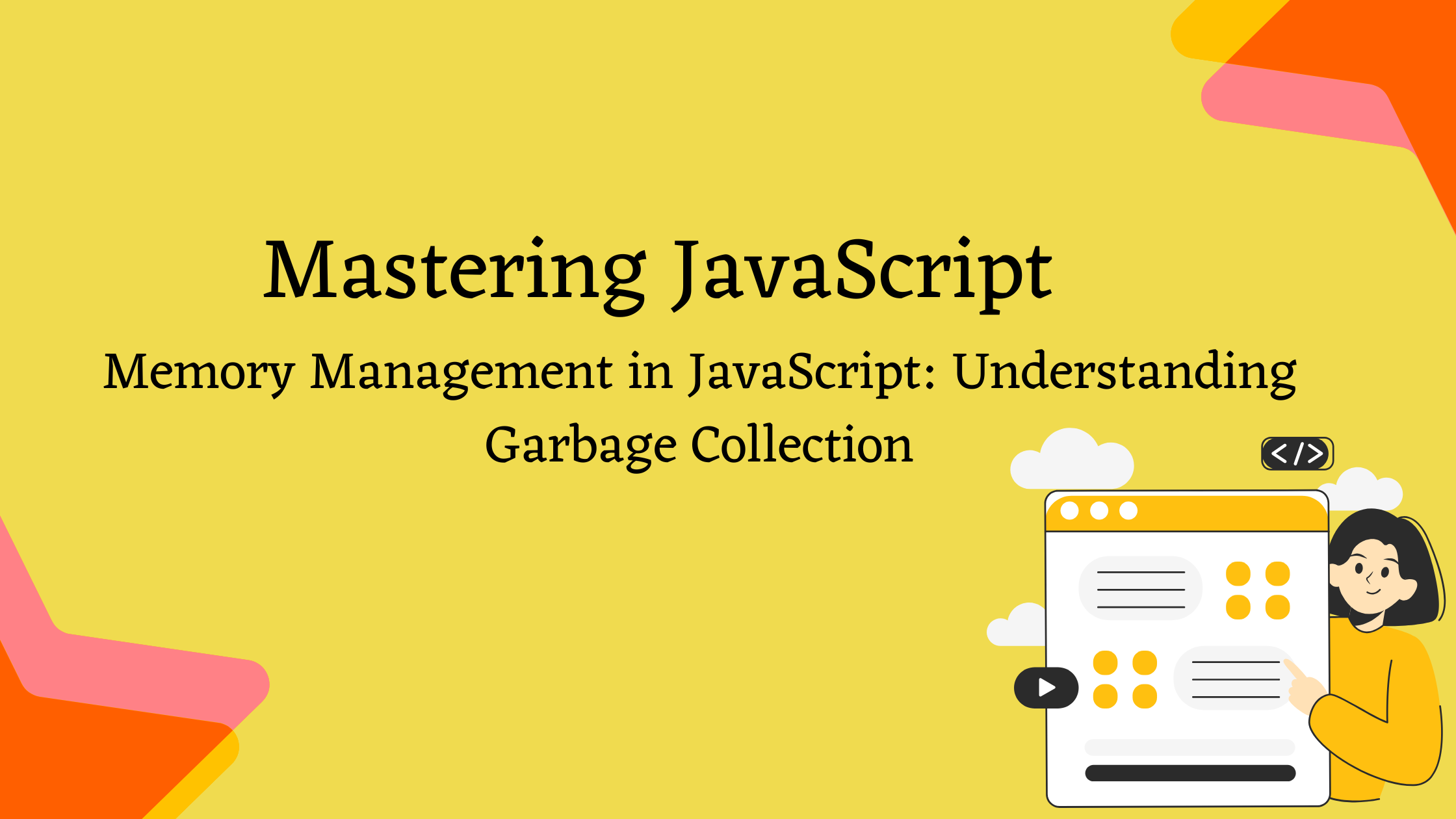
Introduction
In any programming language, managing memory efficiently is crucial to building high-performance applications. JavaScript, despite being a high-level language, handles memory management for you through a process called Garbage Collection. This means developers don’t need to manually allocate or free memory, but that doesn’t mean we should ignore how memory management works. Understanding Garbage Collection in JavaScript helps developers optimize their code, avoid memory leaks, and build applications that scale well.
In this article, we’ll explore how JavaScript manages memory, what Garbage Collection is, why it's essential for production environments, and how poor memory management can affect databases and applications running at scale.
Why We Need to Care About Memory Management in Production
JavaScript’s automatic memory management might lead you to believe that you don’t have to worry about memory. While the JavaScript engine does a great job most of the time, it can still lead to performance issues in specific cases, particularly in production. If your application is inefficient with memory, you could face:
Memory Leaks: If your app holds onto memory it no longer needs, memory usage can grow uncontrollably.
Performance Degradation: The Garbage Collector works in cycles, and inefficient memory usage can trigger more frequent and longer Garbage Collection pauses, slowing down your app.
Out of Memory Errors: Especially in long-running applications or services that handle many concurrent requests, failing to release unused memory can lead to crashes.
For large-scale, production-ready applications, avoiding these issues is critical to maintaining performance, especially when handling large datasets, managing real-time updates, or providing services to millions of users.
How Garbage Collection Works in JavaScript
Garbage Collection in JavaScript is based on a concept called reachability. Simply put, objects in memory that can still be reached or accessed by the program are considered live, while objects that are no longer reachable are considered garbage and can be collected and freed.
The Two Phases of Garbage Collection:
Marking: The Garbage Collector starts from the root objects (like global variables or objects in the call stack) and marks everything that is still reachable. If an object isn’t marked, it’s considered unnecessary and can be collected.
Sweeping: After marking, the collector “sweeps” through memory and frees up space used by the unmarked objects.
This process happens automatically, but developers can unintentionally hold onto references that prevent objects from being cleaned up, leading to memory issues.
How Memory Management Affects Connected Databases
Memory management isn’t just about the internal workings of JavaScript—poor memory management can also impact connected databases. Here’s how:
Caching Data: In web applications, data is often cached in memory to reduce database queries. While caching improves performance, improperly managed memory can lead to an overgrown cache that consumes more memory than necessary. If the Garbage Collector doesn’t clean up unused cache items, it will eventually increase memory pressure and slow down the application.
Database Connections: Applications often maintain database connections in memory to keep communication efficient. However, if these connections aren’t closed properly or if too many connections remain open due to poor memory management, it could overwhelm the database, leading to performance bottlenecks or even downtime.
Data Handling: In applications where large datasets are fetched from a database, inefficient memory usage can slow down Garbage Collection, as more data needs to be marked and swept. This could cause slow queries, delayed responses, and server crashes under heavy load.
Production Use-Case: Millions of Users in Action
Let’s say you have a real-time messaging app with millions of users. Your app stores user data, chat histories, and notifications in memory, and it frequently connects to a database to retrieve and update user information. Here’s how memory management and Garbage Collection can become a problem at scale:
Handling Large Objects: With millions of users sending messages and sharing files, your app will likely have many large objects stored in memory. If not properly managed, these large objects could remain in memory even when they’re no longer needed, leading to memory bloat. As the memory fills up, the Garbage Collector will need to work harder, leading to longer pauses and reduced application responsiveness.
User Sessions: Each user session might maintain an open connection to the server. Without careful memory management, old, inactive sessions could stay in memory longer than necessary, consuming resources and slowing down the application.
Increased Traffic: When millions of users are online simultaneously, the load on both your server and your database increases. If the server struggles with memory issues, this could lead to more frequent Garbage Collection cycles, causing delays in response times, higher latency, and even server crashes.
Database Querying and Memory Leaks: When handling queries to the database, your app may store results in memory for quick access. But if these results aren’t properly released after use, they’ll accumulate, consuming more memory over time. This creates a memory leak that could eventually crash the app, especially during high-traffic periods.
Best Practices for Memory Management in JavaScript
To avoid memory-related issues in production, here are some best practices:
Avoid Memory Leaks: Ensure that references to objects, event listeners, and intervals are properly cleaned up when they’re no longer needed.
Use Efficient Data Structures: Choose the right data structures for your use case. For example, use
WeakMap
orWeakSet
for storing temporary objects that can be garbage collected when they’re no longer needed.Limit Object Lifetimes: Use functions like closures to limit the lifetime of objects, ensuring they’re freed when they go out of scope.
Profiling Memory Usage: Use tools like Chrome DevTools to profile and monitor your application’s memory usage, helping you identify potential memory leaks or areas of inefficiency.
Optimize Garbage Collection Pauses: Design your code in a way that minimizes the frequency and duration of Garbage Collection pauses, such as splitting large tasks into smaller chunks to avoid blocking the event loop.
Conclusion
Although JavaScript handles memory management for you with Garbage Collection, it’s crucial to understand how it works, especially in production environments where performance is key. Poor memory management can lead to serious performance issues, especially when your app connects to a database or serves millions of users.
By following best practices and keeping an eye on memory usage, you can build robust and scalable applications that avoid the pitfalls of inefficient memory management.
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
I’m a problem solver at heart, driven by the idea of building solutions that genuinely make a difference in people’s everyday lives. I’m always curious, always learning, and always looking for ways to improve the world around me through thoughtful, impactful work. Beyond building, I love giving back to the community — whether it’s by sharing what I’ve learned through blogs, tutorials, or helpful insights. My goal is simple: to make technology a little more accessible and useful for everyone. Let’s learn, build, and grow together.