React Query: Your New Best Friend in Data Management
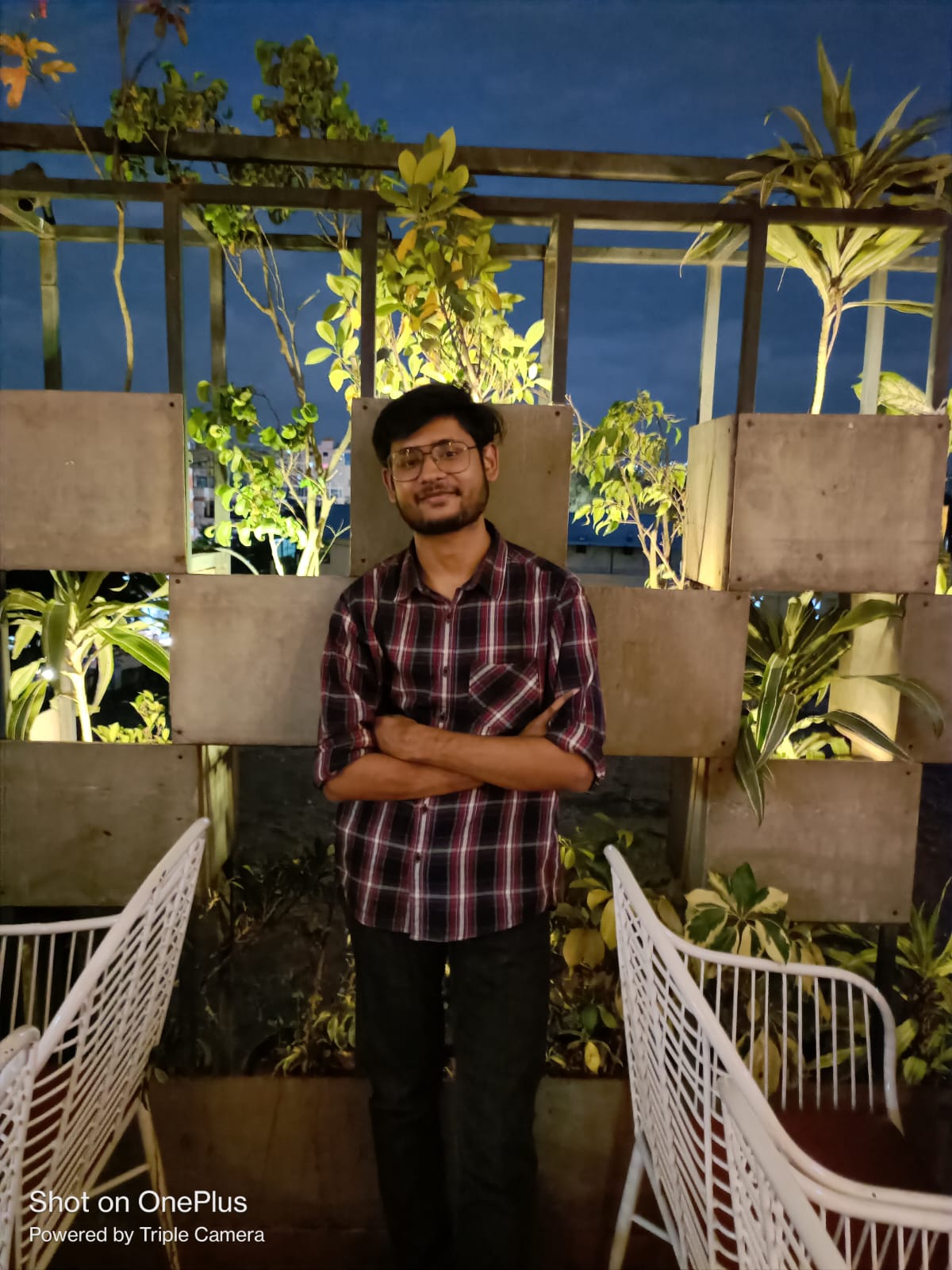
If you’ve ever built a React application, you know how crucial it is to manage data efficiently. Fetching data from an API, updating it, and keeping your UI in sync can be a headache. Enter React Query, a library that takes the pain out of data fetching and state management. Let’s explore how this tool can become your new best friend in the world of React development.
What is React Query?
At its core, React Query is a data-fetching library that helps you manage server state in your applications. Whether you need to fetch, cache, or synchronize data, React Query has got you covered. It’s like a Swiss Army knife for data management, offering tools that make your life as a developer easier and your applications faster.
Why React Query is a Game Changer
Automatic Caching: One of the standout features of React Query is its automatic caching. When you fetch data, it gets stored in a cache. If you need to access the same data again, React Query will serve it from the cache instead of making another network request. This speeds up your application and reduces load times.
Simplified API: React Query’s API is intuitive and easy to use. You can fetch data with just a few lines of code. This means less boilerplate and more focus on building your app’s features.
Background Synchronization: Have you ever looked at your app and wondered, “Is this data up to date?” With React Query, you don’t have to worry. It automatically refetches data in the background, ensuring users always see the latest information.
Built-in Error Handling: Error handling can be a pain, but React Query makes it simple. You can easily manage error states and display error messages to users when something goes wrong.
Optimistic Updates: Want to make your app feel snappy? With optimistic updates, you can immediately update the UI before the server responds. This gives users a more responsive experience, making your app feel faster and more interactive.
Pagination and Infinite Scrolling: If your app needs to display lots of data, React Query has built-in support for pagination and infinite scrolling. This makes it a breeze to implement these features without reinventing the wheel.
Getting Started with React Query
To start using React Query, you’ll first need to install it. You can do this easily with npm or yarn:
npm install react-query
or
yarn add react-query
After installing, you need to set up a QueryClient and wrap your application in a QueryClientProvider. Here’s a quick setup:
import React from 'react';
import { QueryClient, QueryClientProvider } from 'react-query';
import App from './App';
const queryClient = new QueryClient();
const Root = () => (
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>
);
export default Root;
Example: Fetching and Mutating Data
Let’s see how React Query works with a practical example. Imagine we’re building a simple app to manage users. We’ll fetch a list of users and also add a new user using mutations.
Here’s how you can fetch users:
import React from 'react';
import { useQuery, useMutation } from 'react-query';
// Fetch users
const fetchUsers = async () => {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
};
// Create user
const createUser = async (newUser) => {
const response = await fetch('https://jsonplaceholder.typicode.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(newUser),
});
if (!response.ok) {
throw new Error('Error creating user');
}
return response.json();
};
const UsersList = () => {
const { data, error, isLoading } = useQuery('users', fetchUsers);
const mutation = useMutation(createUser, {
onSuccess: () => {
// Invalidate and refetch
queryClient.invalidateQueries('users');
},
});
const handleAddUser = () => {
const newUser = { name: 'New User' };
mutation.mutate(newUser);
};
if (isLoading) return <div>Loading...</div>;
if (error) return <div>Error fetching users: {error.message}</div>;
return (
<div>
<button onClick={handleAddUser} disabled={mutation.isLoading}>
Add User
</button>
{mutation.isLoading && <div>Adding user...</div>}
{mutation.isError && <div>Error: {mutation.error.message}</div>}
<ul>
{data.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default UsersList;
Breaking Down the Example
Fetching Data: We use
useQuery
to fetch the list of users. The hook automatically handles loading states and errors, so we can focus on rendering the UI.Mutating Data: The
useMutation
hook allows us to add a new user. When the mutation is successful, we invalidate theusers
query to ensure the user list is up to date.User Experience: The UI provides immediate feedback to users, such as loading indicators and error messages, making for a smooth experience.
Conclusion
React Query is a powerful ally for any React developer. It simplifies data fetching and state management, allowing you to build faster and more responsive applications. With features like automatic caching, background synchronization, and built-in error handling, you can focus on what truly matters: creating amazing user experiences. So why not give React Query a try? Your future self will thank you!
Subscribe to my newsletter
Read articles from Divyesh Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
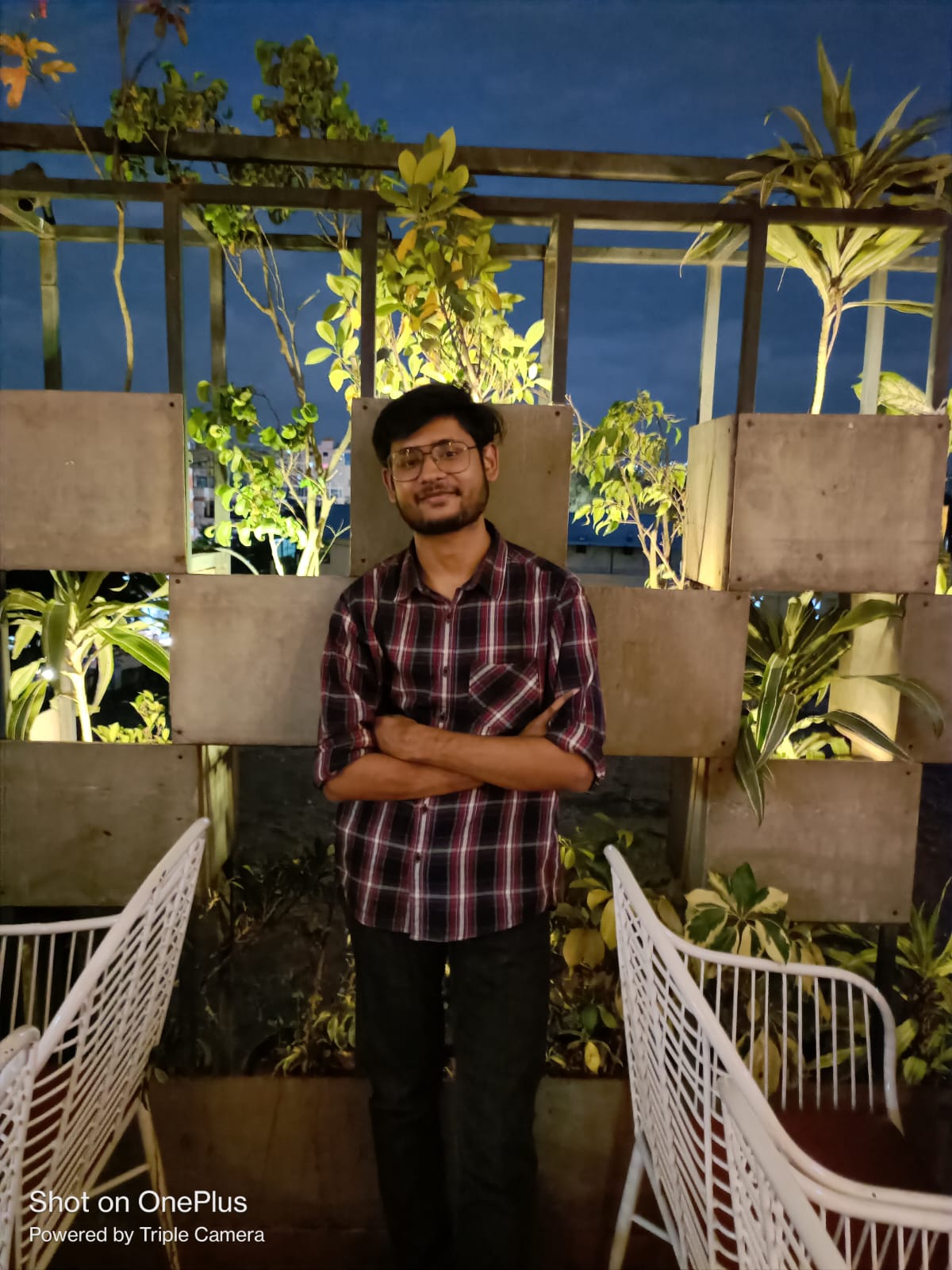
Divyesh Jain
Divyesh Jain
I'm a quick learner, turning complex ideas into seamless user experiences.