How to Use the Spread Operator (...) in JavaScript to Simplify Your Code

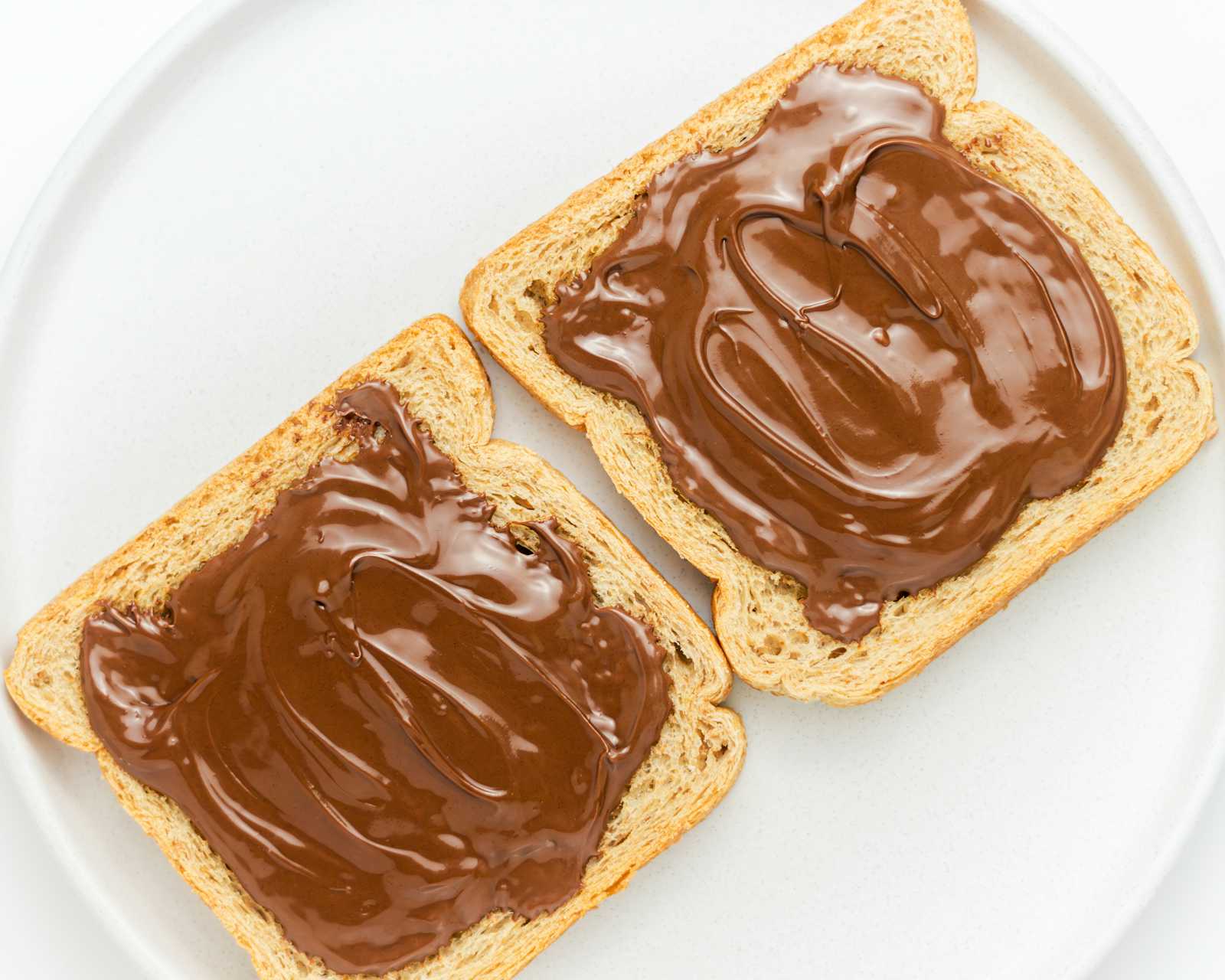
JavaScript is full of powerful tools, and one versatile feature that often goes unnoticed is the spread operator (...), introduced in ES6 that can simplify your code in ways that may not be immediately obvious. Whether you're copying arrays, merging objects, or passing arguments to functions, the spread operator helps make your code more readable and efficient. In this post, we'll explore how to use the spread operator in different scenarios and why it's such a valuable tool for modern JavaScript developers. Although simple, the spread operator is versatile and capable of transforming the way you work with arrays, objects, and even functions.
What is the Spread Operator?
The spread operator (...) is a feature introduced in ES6 that allows you to expand an iterable (such as an array or object) into individual elements. Its primary purpose is to help simplify tasks like merging arrays or objects, copying data, or passing arguments to functions.
But what exactly does it do, and why is it so useful? Let's dive into some examples to see the power of the spread operator in action.
const arr1 = [1, 2];
const arr2 = [3, 4];
const mergedArray = [...arr1, ...arr2];
console.log(mergedArray); // Output: [1, 2, 3, 4]
So, what’s happening here?
The ... takes each element from arr1
and arr2
and spreads them into a new array we call mergedArray. Under the hood, this is almost like copying each element from arr1
and arr2
and pasting them into a new array, in the same order. This is why the final array includes all elements, resulting in [1, 2, 3, 4]
. Both arrays remain independent; modifying one will not affect the other.
One of the benefits of the spread operator is that it provides concise code and improved readability. Prior to the spread operator, merging arrays used to involve the concat method. Let’s take a look at some comparison examples;
Using concat: Inserting an element between two arrays would require chaining concat()
calls or using additional steps,
const result = arr1.concat(5).concat(arr2);
// result: [1, 2, 5, 3, 4]
With spread operator: You can insert elements naturally between arrays:
const result = [...arr1, 5, ...arr2];
// result: [1, 2, 5, 3, 4]
This makes it more readable when adding or inserting elements at different points in the array.
The spread operator can easily be combined with other JavaScript operations, such as destructuring or mapping, making it more versatile. When used with destructuring (unpack values from arrays or properties from objects into distinct variables.) as shown below,
const arr = [1, 2, 3, 4];
const [first, ...rest] = arr;
console.log(rest); // [2, 3, 4]
what we are doing is telling JavaScript to:
Assign the first element of the array
arr
to the variable first.Assign the rest of the elements to a new array, and store that array in the variable rest.
So, first
will be assigned the value 1, and rest
will be assigned [2, 3, 4]
. The variables are declared and used at the same time. The concat() method doesn’t offer this kind of flexibility.
Spreading Elements into Function Arguments
The spread operator can be used to pass the elements of an array as individual arguments to a function. This is especially helpful when you don't know the number of elements beforehand, or when you have them stored in an array.
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // Output: 6
Instead of manually passing each argument, the spread operator expands the array numbers and passes each value as a separate argument to the sum function.
Copying and Merging Objects
The spread operator also works with objects, making it a great way to copy or merge object properties.
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const mergedObj = { ...obj1, ...obj2 };
console.log(mergedObj); // Output: { a: 1, b: 3, c: 4 }
Notice that the property ‘b’ from obj2
overwrites the ‘b’ property from obj1
. When merging, later properties take precedence over earlier ones. The spread operator ... copies the key-value pairs from obj1
and obj2
into a new object. It does not create duplicates of keys. Instead, it treats object keys as unique identifiers.
Converting Iterables to Arrays
The spread operator can also be used to convert iterable objects, like strings or NodeLists, into arrays. This makes it easy to work with iterable data structures in situations where you need array-like methods.
const str = "hello";
const chars = [...str];
console.log(chars); // Output: ['h', 'e', 'l', 'l', 'o']
This technique is particularly useful when working with DOM manipulation, where NodeList objects (e.g., from document.querySelectorAll) don’t have array methods. You can use the spread operator to convert them into arrays quickly.
A Real-World Use Case.
The spread operator is much more than just a fancy syntax for copying or merging arrays and objects. Its true power lies in the way it enables you to work with immutable data and clean up objects without modifying the original structure. Let’s explore a key use case: working with immutable data with the spread operator to target specific properties. This is widely used, especially in frameworks like React, and can help you write cleaner, more efficient code.
Working with Immutable Data: Use Case 1
When working with JavaScript, and particularly with state management in frameworks like React, immutability is a core principle. This means you should never directly modify the original data (like state). Instead, you create a copy, modify the copy, and then use the modified version.
Let’s look at a common example in React,
const [state, setState] = useState({ name: 'John', age: 30 });
// To update the 'age' without mutating the original state:
setState({ ...state, age: 31 });
So, what's happening here?
The spread operator (...state) creates a shallow copy of the current state object.
When the copy is created, we update the age property to 31.
The setState function then updates the state with this new object, leaving the original state object unmodified.
This approach is critical in React because direct mutation of state can lead to bugs and unexpected behaviour. The spread operator makes it easy to update only specific properties of an object while preserving the rest.
In this case, we only change the age property, but the name property remains unchanged, even though it's part of the same state object. Without the spread operator, you would need more verbose code or a manual copy of each property, which is error-prone and inefficient.
Removing Properties: Use Case 2.
const user = { id: 1, name: 'John', password: 'secret' };
const { password, ...safeUser } = user;
console.log(safeUser); // Output: { id: 1, name: 'John' }
Here, the password property is removed using destructuring and the spread operator, ensuring that only safe data is passed along.
In conclusion, the spread operator is a small but powerful feature that can greatly simplify your code. Whether you’re working with arrays, objects, or functions, this operator can help you write more concise, readable, and efficient JavaScript. Next time you’re handling arrays or objects, give the spread operator a try, it could save you time and prevent errors.
Feel free to share your own experiences and additional tips in the comments section and remember to subscribe to my FREE newsletter. Until next time, Happy programming!
Subscribe to my newsletter
Read articles from Mandla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mandla
Mandla
Junior Developer and Tech Enthusiast with a Drive to Transform Industries through Innovation.