Chapter 41: Dynamic Programming (Part 2)
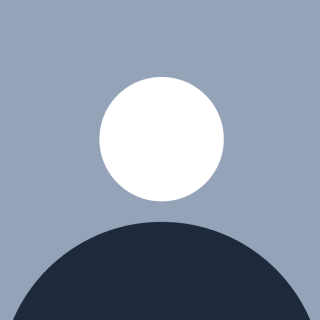
Introduction
In this chapter, we dive deeper into Dynamic Programming, focusing on several classic problems and strategies associated with Knapsack-type problems. These problems, widely known for their use of recursion, memoization, and tabulation, highlight essential DP techniques that can be applied to many real-world optimization scenarios. By the end of this chapter, you’ll understand how to solve and implement various types of Knapsack problems, including the 0-1 Knapsack problem, Target Sum Subset, and Unbounded Knapsack. Each section introduces a new concept, gradually building up from recursive solutions to optimized tabulation techniques.
Let’s break down each problem type and approach step-by-step to master the dynamic programming approach to Knapsack problems.
1. Types of Knapsack Problems
The Knapsack problems are categorized based on the restrictions placed on item usage and the goal we aim to achieve. Here are the most commonly encountered types:
0-1 Knapsack: Each item can either be included or excluded from the knapsack, hence the term 0-1 (include = 1, exclude = 0).
Target Sum Subset: Given a set of numbers, this problem focuses on determining if a subset of numbers can sum to a target value.
Unbounded Knapsack: Unlike the 0-1 Knapsack, each item can be included multiple times to maximize the value of the knapsack.
These problem types have distinct applications and vary in approach based on constraints, making them a core study area in dynamic programming.
2. 0-1 Knapsack Problem (Recursion)
The 0-1 Knapsack problem is a combinatorial optimization problem where we try to maximize the total value of items in a knapsack, given a weight limit. This problem, in its recursive form, can be solved by considering each item to either include it in the knapsack or exclude it.
Recursive Approach Explanation
Base Case: If there are no items left or the knapsack's capacity is zero, the maximum profit is zero.
Recursive Case:
Include the item: Add its value to the total profit and reduce the capacity by the item's weight.
Exclude the item: Move to the next item without changing the capacity.
The final answer will be the maximum of these two options.
public int knapsackRecursive(int[] weights, int[] values, int capacity, int n) {
if (n == 0 || capacity == 0) {
return 0;
}
if (weights[n - 1] > capacity) {
return knapsackRecursive(weights, values, capacity, n - 1);
} else {
return Math.max(values[n - 1] + knapsackRecursive(weights, values, capacity - weights[n - 1], n - 1),
knapsackRecursive(weights, values, capacity, n - 1));
}
}
3. 0-1 Knapsack Problem (Memoization)
The recursive solution for the 0-1 Knapsack problem can lead to redundant calculations. Memoization helps by storing the results of subproblems in a cache to avoid recomputation, improving the time complexity.
Memoization Code Explanation
Initialize a DP Array: Use a 2D array where each entry
dp[i][w]
stores the maximum value that can be achieved withi
items and weightw
.Store Results: As you solve subproblems, save results in the
dp
array.Return Saved Results: If a subproblem is already solved, retrieve the result instead of recalculating.
public int knapsackMemoization(int[] weights, int[] values, int capacity, int n, int[][] dp) {
if (n == 0 || capacity == 0) {
return 0;
}
if (dp[n][capacity] != -1) {
return dp[n][capacity];
}
if (weights[n - 1] > capacity) {
dp[n][capacity] = knapsackMemoization(weights, values, capacity, n - 1, dp);
} else {
dp[n][capacity] = Math.max(values[n - 1] + knapsackMemoization(weights, values, capacity - weights[n - 1], n - 1, dp),
knapsackMemoization(weights, values, capacity, n - 1, dp));
}
return dp[n][capacity];
}
4. 0-1 Knapsack Problem (Tabulation)
The tabulation approach fills up a DP table iteratively, avoiding recursion altogether. This method iteratively calculates the solution by breaking it down into manageable subproblems, building the solution from the ground up.
Tabulation Code Explanation
Table Setup: A 2D array
dp[i][j]
wherei
represents items considered andj
represents the knapsack's capacity.Fill Table: Iterate over items and capacities, updating each cell with the maximum achievable value.
Result Extraction: The final solution is at
dp[n][capacity]
.
public int knapsackTabulation(int[] weights, int[] values, int capacity, int n) {
int[][] dp = new int[n + 1][capacity + 1];
for (int i = 1; i <= n; i++) {
for (int w = 1; w <= capacity; w++) {
if (weights[i - 1] <= w) {
dp[i][w] = Math.max(values[i - 1] + dp[i - 1][w - weights[i - 1]], dp[i - 1][w]);
} else {
dp[i][w] = dp[i - 1][w];
}
}
}
return dp[n][capacity];
}
5. Target Sum Subset (Tabulation)
The Target Sum Subset problem asks whether a subset of numbers can achieve a given target sum. This problem can be solved using a tabulation approach similar to the Knapsack problem by breaking it down into subproblems.
Explanation and Code
Initialize a Table:
dp[i][j]
wherei
is the subset size, andj
is the target sum.Filling the Table:
If
dp[i-1][j]
is true, thendp[i][j]
is also true.If
j >= arr[i-1]
, then check if adding this element achievesj
.
public boolean targetSumSubset(int[] arr, int target) {
int n = arr.length;
boolean[][] dp = new boolean[n + 1][target + 1];
for (int i = 0; i <= n; i++) {
dp[i][0] = true;
}
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= target; j++) {
if (arr[i - 1] <= j) {
dp[i][j] = dp[i - 1][j] || dp[i - 1][j - arr[i - 1]];
} else {
dp[i][j] = dp[i - 1][j];
}
}
}
return dp[n][target];
}
6. Target Sum Subset Code
The code above provides a solution for finding a subset with a sum equal to the target. It is efficient with a time complexity of (O(n \times target)) and gives clear insight into the subset-sum dynamics in the context of dynamic programming.
7. Unbounded Knapsack (Tabulation)
In the Unbounded Knapsack problem, each item can be chosen multiple times. This version requires a slightly modified approach from the 0-1 Knapsack.
Code Explanation
Initialize Table: Set up a DP array of size
capacity + 1
.Iterative Solution: For each capacity, consider including items multiple times to achieve the maximum value.
public int unboundedKnapsack(int[] weights, int[] values, int capacity) {
int[] dp = new int[capacity + 1];
for (int i = 0; i < weights.length; i++) {
for (int j = weights[i]; j <= capacity; j++) {
dp[j] = Math.max(dp[j], dp[j - weights[i]] + values[i]);
}
}
return dp[capacity];
}
Conclusion
In this chapter, we explored various types of Knapsack problems and their solutions using recursion, memoization, and tabulation techniques. These approaches allow for efficient problem-solving by leveraging the DP framework. By understanding these techniques, you are now equipped to tackle many related optimization problems in dynamic programming.
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊