118. Pascal's Triangle
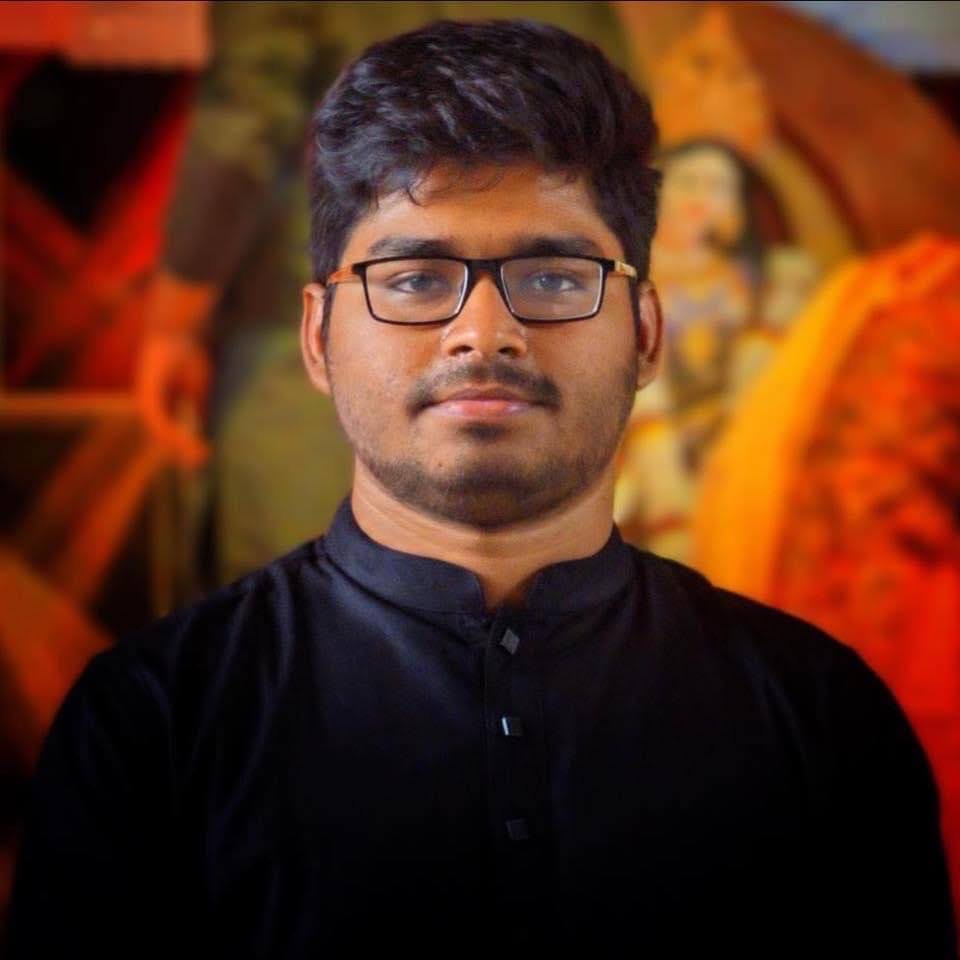
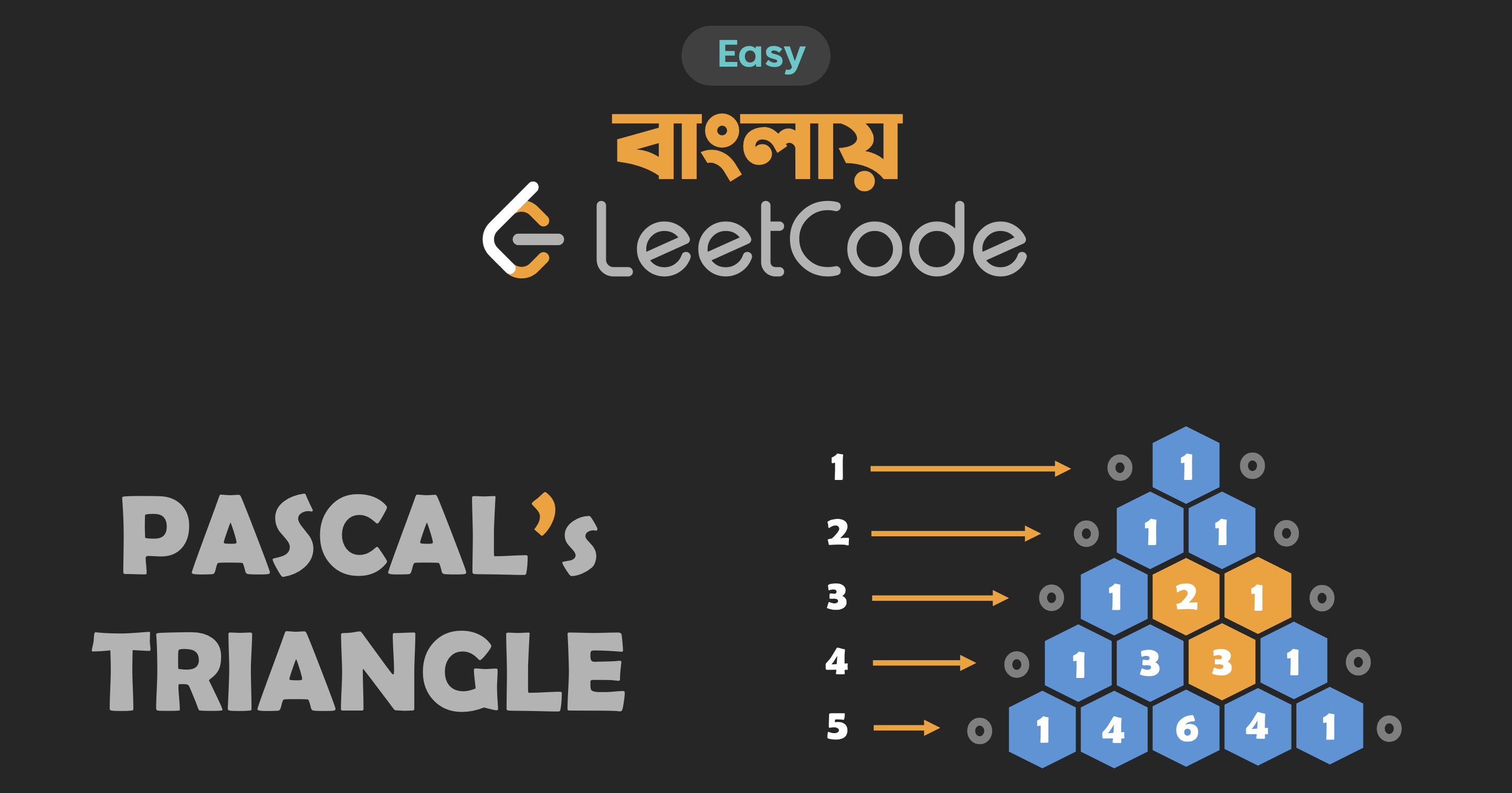
[A1] - Brute Force
Time: O(n^2)
Space: O(n^2)
class Solution:
def generate(self, numRows: int) -> List[List[int]]:
# Initialize the triangle with the first row
pascal_triangle = []
for i in range(numRows):
# Start each row with a list
row = [1] * (i + 1) # Every row starts with 1s
# Fill in the interior values
for j in range(1, i):
row[j] = pascal_triangle[i - 1][j - 1] + pascal_triangle[i - 1][j]
# Append the completed row to the triangle
pascal_triangle.append(row)
return pascal_triangle
What this code does:
Solution class: It defines a class that co ntains a method for generating Pascal’s Triangle.
generate method: This method takes an integer numRows as input, representing the number of rows in Pascal’s Triangle to generate.
Initializes an empty list called
pascal_triangle
that will hold all rows of Pascal’s Triangle.Uses a loop to iterate over the range of numRows to generate each row of the triangle.
Each row is initialized with 1s using
[1] * (i + 1)
.For rows beyond the second, the interior values (non-1s) are calculated using values from the previous row:
pascal_triangle[i - 1][j - 1] + pascal_triangle[i - 1][j]
.After completing a row, it appends the row to the
pascal_triangle
list.Finally, the
pascal_triangle
is returned.
Subscribe to my newsletter
Read articles from Debjoty Mitra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
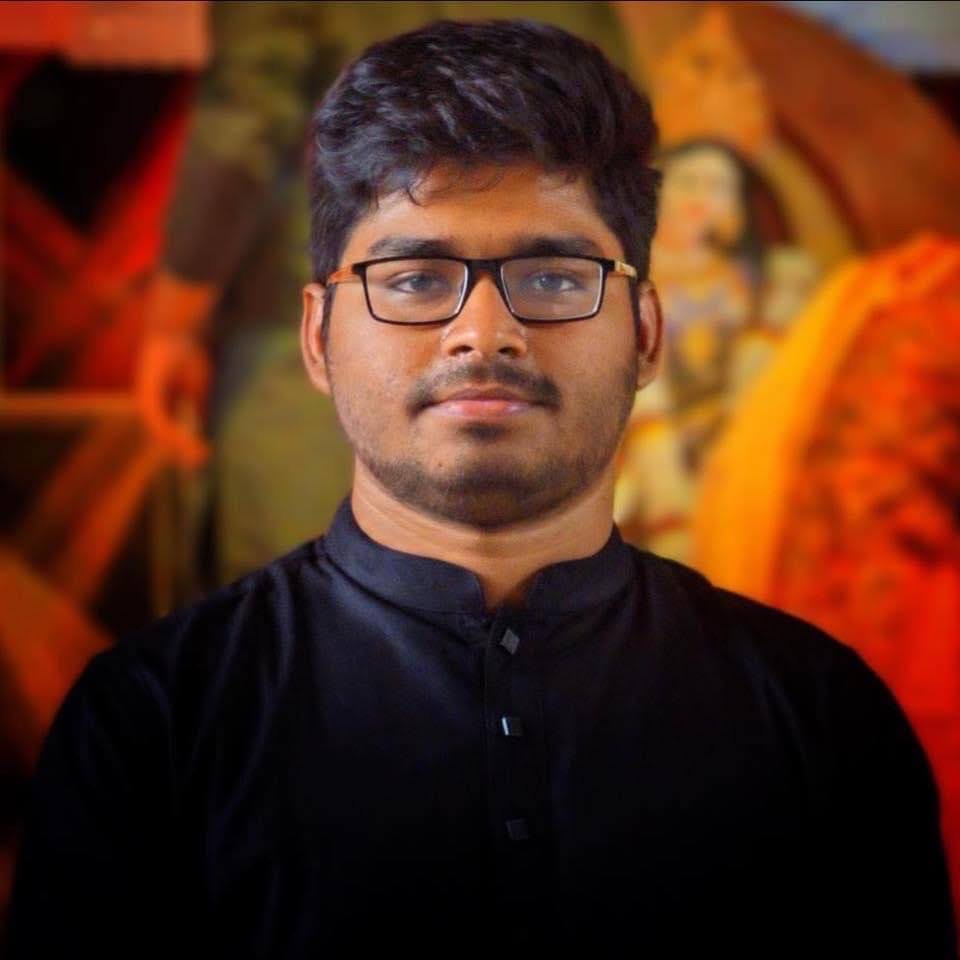