"Data Types and Operators in Python🐍"

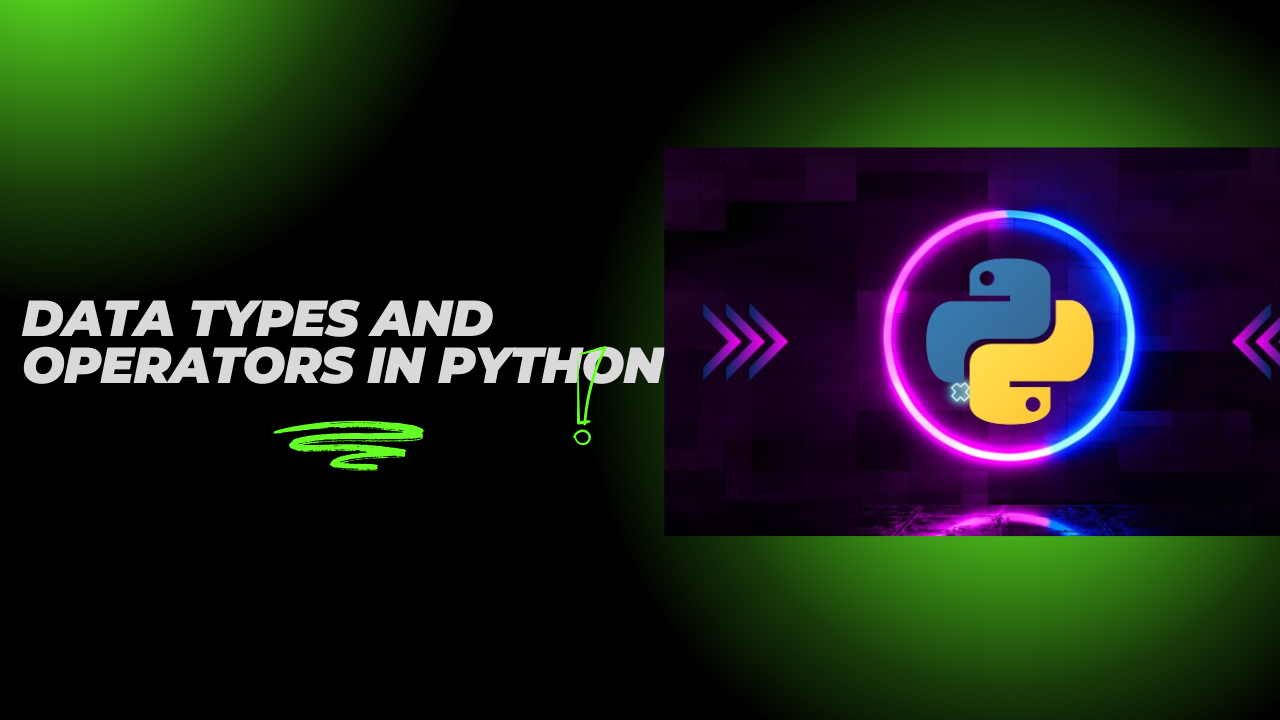
What Are Data Types in Python?
Data types tell the interpreter what kind of data is being used in the program. Python automatically assigns the correct type to variables when they are created.(dynamically typed)
Now we will look into the most important Python data types. Python provides us with several data types to store and process data. choosing the right data type matters for solving a problem and having an idea about each data type functionalities & what they are capable of ,will make easier to pick right data type.
type()
function is used to determine the type of data
Basic data types:
The basic data types in Python store a single value, like a number or a piece of text.
Integer:
Represents whole numbers (positive, negative, or zero) without decimal points, there's no fixed size.
we can convert string and float to integer by using int()
function
Float:
Represents numbers with decimal points or exponent notation.
we can convert int and string to float by using float()
function
Boolean:
Represents logical values: True or False. Internally, True is equivalent to 1 and False is equivalent to 0
None:
Represents the absence of a value, similar to null in other languages. None is often used to indicate that a variable has no value or an uninitialized state
String:
Represents sequences of characters ,immutable (they cannot be changed after creation).Can use single (' ') or double (" ") or triple (“““ “““) quotes to create strings
The nice thing about triple quotes is that you can use both single and double quotes within them. So you can use triple quotes to cleanly create strings that contain both single and double quotes.
we can convert int and float to string by using str()
function
.ord(character)
: gives ASCII (or Unicode) integer value of a given character. .chr(integer)
: gives character associated with the specified ASCII (or Unicode) integer value.
# Using ord() to get ASCII/Unicode values
ascii_value = ord('A')
print(ascii_value) # Output: 65
# Using chr() to get characters from ASCII/Unicode values
character = chr(65)
print(character) # Output: A
BASIC STRING OPERATIONS:
concatenation: we can add two strings (+ brings Python strings together)
repetitions: multiplication operator repeats the string given number of times.
indexing: to access specific character in a string (+ve and -ve indexing)
slicing: to access specific part(sub string) in a string
# Concatenation
str1 = "Hello"
str2 = "World"
str = str1 + " " + str2
print(str) # Output: Hello World
# Repetition
str = str1 * 3
print(str) # Output: HelloHelloHello
# Indexing
# Positive indexing
char1 = str1[0] # First character
char2 = str1[1] # Second character
print(char1) # Output: H
print(char2) # Output: e
# Negative indexing
char1 = str1[-1] # Last character
char2 = str1[-2] # Second to last character
print(char1) # Output: o
print(char2) # Output: l
# Slicing:
# Syntax: string[start:end] (end index is exclusive)
substring = str1[1:4] # Characters from index 1 to 3
print(substring) # Output: ell
# Slicing with negative indices
negative_slice = str1[-4:-1] # Characters from index -4 to -2
print(negative_slice) # Output: ell
1. Case Modification
capitalize()
: Capitalizes the first letter.upper()
: Converts all characters to uppercase.lower()
: Converts all characters to lowercase.swapcase()
: Swaps uppercase to lowercase and vice versa.title()
: Capitalizes the first letter of each word.casefold()
: Converts the string to lowercase in a way that’s more aggressive thanlower()
, suitable for case-insensitive comparisonsmystring = "Hello world" print(mystring.capitalize()) # Output: "Hello world" print(mystring.upper()) # Output: "HELLO WORLD" print(mystring.lower()) # Output: "hello world" print(mystring.swapcase()) # Output: "hELLO WORLD" print(mystring.title()) # Output: "Hello World"
2. Searching and Counting
find("world")
: Returns the index of the first occurrence of "world" or-1
if not found.index("world")
: Similar tofind()
but raises an error if not found.count("o")
: Counts occurrences of "o".mystring = "Hello world" print(mystring.find("world")) # Output: 6 print(mystring.index("world")) # Output: 6 print(mystring.count("o")) # Output: 2
3. Checking String Properties
isalpha()
: Checks if all characters are alphabetic.isdigit()
: Checks if all characters are digits.isascii()
: Checks if all characters are ASCII.isalnum()
: Checks if all characters are alphanumeric.isspace()
: Checks if all characters are whitespace.islower()
: Checks if all characters are lowercase.isupper()
: Checks if all characters are uppercase.istitle()
: Checks if string is in title case.
4. Modifying String Content
replace("world", "Python")
: Replaces "world" with "Python".strip()
: Removes whitespace from both ends.lstrip()
: Removes whitespace from the left.rstrip()
: Removes whitespace from the right.print(mystring.replace("world", "Python")) # Output: "Hello Python" print(mystring.strip()) # Output: "Hello world"
5. Splitting and Joining
split(separator)
: Splits the string into a list of substrings based on the specified separator.
join(["Hello", "world"])
: Joins elements of a list with mystring
as a separator.
print(mystring.split()) # Output: ['Hello', 'world']
print(", ".join(["Hello", "world"])) # Output: "Hello, world"
python string formatting with f-strings: f-strings (formatted string literals) used to embed expressions directly within strings, using curly braces {}
name = "tom"
age = 20
print(f"Hello, {name}. You are {age} years old.")
# Output: "Hello, tom. You are 20 years old."
Advanced data types:
They can store many items, like lists of items or key-value pairs
List:
An ordered collection of items (of any data type).Lists are mutable, meaning you can change their contents after creation. Items are indexed, starting from 0.
l=['apple','banana',2,9]
print(l) #['apple','banana',2,9]
print(type(l)) #class <'list'>
# Empty
print([]) #[]
# 1D
print([1,2,3,4,5]) #[1,2,3,4,5]
# 2D
print([1,2,3,[4,5]]) #[1, 2, 3, [4, 5]]
# 3D
print([[[1,2],[3,4]],[[5,6],[7,8]]])
# Hetrogenous
print([1,True,5.6,5+6j,'Hello'])
# Using Type conversion
print(list('hello')) #['h', 'e', 'l', 'l', 'o']
accessing elements of list:
my_list = [10, 20, 30, 40, 50]
# Access elements
print(my_list[0]) # Output: 10 (first element)
print(my_list[-1]) # Output: 50 (last element)
print(len(my_list)) #5
# Slice the list
print(my_list[1:3]) # Output: [20, 30]
print(my_list[:3]) # Output: [10, 20, 30] (first 3 elements)
list methods:
my_list = [10, 20, 30, 40]
# Modify an element
my_list[2] = 100
print(my_list) # Output: [10, 20, 100, 40]
#`list.append(element)`:Adds a single element to the end of the list
my_list = [1, 2, 3]
my_list.append(4)
my_list.append([8,7,90])
print(my_list) # Output: [1, 2, 3, 4, [8, 7, 90]]
#`list.extend(iterable)`:
#Extends the list by appending elements from an iterable (like another list).
my_list = [1, 2, 3]
my_list.extend([4, 5, 6])
my_list.extend("higft")
print(my_list) # Output: [1, 2, 3, 4, 5, 6, 'h', 'i', 'g', 'f', 't']
my_list = [1, 2, 3]
my_list.insert(1, "apple") # Inserts "apple" at index 1
print(my_list) # Output: [1, 'apple', 2, 3]
#`list.remove(value)`:Removes first occurrence of a specified value from the list.and Returns None
my_list = [1, 2, 3, 2]
my_list.remove(2) # Removes the first occurrence of 2
print(my_list) # Output: [1, 3, 2]
# If the value is not found, it raises a `ValueError`.
#`list.pop(index)`: Removes and returns the item at the specified index (or the last item if no index is provided).
# Returns: The removed item
my_list = [1, 2, 3]
item = my_list.pop() # Removes and returns the last item
print(item) # Output: 3
print(my_list) # Output: [1, 2]
# If the specified index is out of range, it raises an `IndexError`.
# del list[index]` or `del list`: Deletes an element at a specified index or the entire list.and Returns: None
my_list = [1, 2, 3]
del my_list[1] # Deletes the item at index 1
print(my_list) # Output: [1, 3]
#You can also use `del my_list` to delete the entire list, which frees up the memory.
Tuples:
Similar to lists but immutable .Once a tuple is created, its elements cannot be changed.
t=('apple','banana',2,9)
print(t) #['apple','banana',2,9]
print(type(t)) #class <'tuple'>
t=(1)
print(type(t)) #class <'int'>
t=(1,)
print(type(t)) #class <'tuple'>
#Accessing Tuple Elements
#Like lists, you can access tuple elements using indexing and slicing.
my_tuple = (10, 20, 30, 40)
print(my_tuple[0]) # Output: 10 (first element)
print(my_tuple[-1]) # Output: 40 (last element)
# Slice a tuple
print(my_tuple[1:3]) # Output: (20, 30)
nested_tuple = ((1, 2), (3, 4), (5, 6))
# Access nested elements
print(nested_tuple[0]) # Output: (1, 2) (first tuple)
print(nested_tuple[0][1]) # Output: 2 (second element of the first tuple)
Range:
range
is a data type in Python. It represents an immutable sequence of numbers and is commonly used for generating a sequence of integers.
The range
function returns a range
object, which is its own data type
a=range(3)
print(a) #range(0,3)
print(type(range(a))) # Output: <class 'range'>
list ,tuple and range are Python’s three built-in sequence data types
set:
An unordered collection of unique items .Mutable, but items must be immutable (e.g., integers, strings, tuples).Useful for membership tests and removing duplicates.
animals = { "cat", "monkey", "donkey" }
# Mixed types are allowed
mixed_set = { 'a2', 15, (1.7, 2) }
Dictionaries:
A collection of key-value pairs. Each key must be unique, and the value can be of any type. Dictionaries are mutable.
std_details = { 'Nick': 12,'Jopy': 24 ,'reko':18}
print(std_details('reko')) # output 18
Operators in Python:
Operators are special symbols used to perform operations on variables and values.
Arithmetic Operators(+,-,*,/,//,%,**):perform mathematical operations.
Comparison (Relational) Operators: used for comparing values.
Logical Operators: combine conditional expressions
Assignment Operators: assign values to variables, and some perform arithmetic or bitwise operations as well.
Bitwise Operators: used to perform operations on bits (binary numbers).
Membership Operators: test whether a value is a member of a sequence (such as a string, list, or tuple).
Identity Operators: check if two variables point to the same object These operators compare the memory location of two objects (i.e., if they refer to the same object).
Arithmetic Operators(+,-,*,/,//,%,**)
x = 10
y = 3
# Addition
print(x + y) # Output: 13
# Subtraction
print(x - y) # Output: 7
# Multiplication
print(x * y) # Output: 30
# Division (result is float)
print(x / y) # Output: 3.333...
# Floor Division (result is integer)
print(x // y) # Output: 3
# Modulus (remainder of division)
print(x % y) # Output: 1
# Exponentiation (x raised to the power of y)
print(x ** y) # Output: 1000
Comparison (Relational) Operators
x = 10
y = 3
print(x == y) # Output: False
print(x != y) # Output: True
print(x > y) # Output: True
print(x < y) # Output: False
print(x >= y) # Output: True
print(x <= y) # Output: False
Logical Operators
x = 10
y = 3
# Logical AND
print(x > 5 and y < 10) # Output: True
# Logical OR
print(x > 5 or y > 10) # Output: True
# Logical NOT
print(not(x > 5)) # Output: False
Assignment Operators
x = 10
x += 5 # x = x + 5
print(x) # Output: 15
x *= 2 # x = x * 2
print(x) # Output: 30
Bitwise Operators
x = 5 # Binary: 0101
y = 3 # Binary: 0011
# Bitwise AND
print(x & y) # Output: 1 (Binary: 0001)
# Bitwise OR
print(x | y) # Output: 7 (Binary: 0111)
# Bitwise XOR
print(x ^ y) # Output: 6 (Binary: 0110)
# Bitwise NOT
print(~x) # Output: -6 (Binary: -(0101 + 1))
# Left Shift
print(x << 1) # Output: 10 (Binary: 1010)
# Right Shift
print(x >> 1) # Output: 2 (Binary: 0010)
Membership Operators
# List membership
my_list = [1, 2, 3, 4]
print(3 in my_list) # Output: True
print(5 not in my_list) # Output: True
# String membership
print("a" in "apple") # Output: True
print("z" not in "apple") # Output: True
Identity Operators
x = [1, 2, 3]
y = [1, 2, 3]
z = x
print(x is z) # Output: True (z refers to the same object as x)
print(x is y) # Output: False (y is a different object)
print(x == y) # Output: True (their contents are the same)
print(x is not y) # Output: True
Subscribe to my newsletter
Read articles from Sandeep directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
