Day 7: Mastering JavaScript DOM
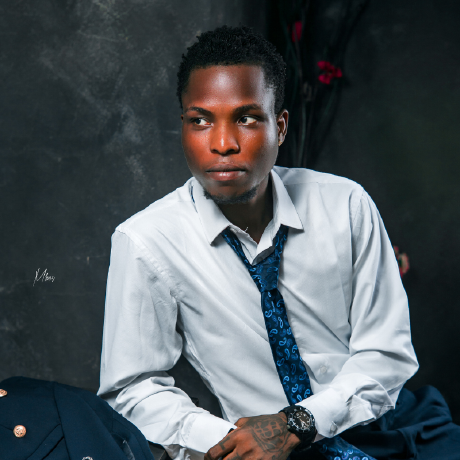
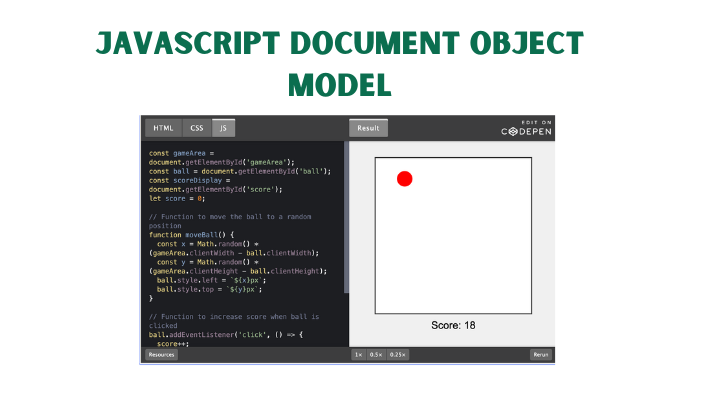
The Document Object Model (DOM) bridges JavaScript and HTML, allowing developers to manipulate the webpage dynamically. It represents the structure of a webpage as a tree of objects, where each HTML element is a node, making it possible to update the content, structure, and styles of a webpage without reloading.
Why Understanding the DOM is Essential for JavaScript Developers
Mastering the DOM gives developers the power to create interactive web applications. From responding to user actions to dynamically updating content, a solid understanding of the DOM is vital for building responsive and engaging user interfaces.
DOM Basics: Key Methods and Their Uses
1. Selecting Elements in the DOM
To manipulate elements, we need ways to select them. Here are some core methods:
getElementById
: Finds an element by its ID.const header = document.getElementById("header");
getElementsByClassName
: Retrieves a list of elements that share the same class.const items = document.getElementsByClassName("item");
querySelector
andquerySelectorAll
: Select elements using CSS selectors.querySelector
finds the first match, whilequerySelectorAll
finds all matches.const mainTitle = document.querySelector(".title"); const buttons = document.querySelectorAll("button");
- Explore DOM Selection Methods more to deepen your understanding.
2. Modifying Elements
Once selected, elements can be modified in various ways:
Changing text content:
mainTitle.textContent = "Welcome to JavaScript DOM!";
Updating styles:
mainTitle.style.color = "blue";
Adding or removing classes:
mainTitle.classList.add("highlight");
- Read more about modifying elements to see how you can transform web pages dynamically.
3. Event Handling in the DOM
JavaScript lets us add event listeners to DOM elements to trigger actions based on user interactions, such as clicks or mouse movements.
const button = document.querySelector("button"); button.addEventListener("click", () => alert("Button clicked!"));
Interactive Example: Building a "Catch the Ball" Game Using the DOM
Here’s a fun way to apply the DOM principles we've learned! Below is our "Catch the Ball" game where you can test your skills. Click on the ball to "catch" it as it moves around the screen. This interactive game is a hands-on example of DOM manipulation, demonstrating element selection, event handling, and dynamic style changes.
Types of Loops in JavaScript DOM Manipulation
When working with collections of DOM elements, JavaScript loops can be invaluable. Here’s a quick overview of the most common loops for DOM manipulation:
forEach Loop: Often used to loop over a list of elements selected by
querySelectorAll
.document.querySelectorAll(".item").forEach(item => { item.style.backgroundColor = "yellow"; });
for Loop: Traditional and allows for more control over the iteration.
const items = document.querySelectorAll(".item"); for (let i = 0; i < items.length; i++) { items[i].style.color = "green"; }
JavaScript Event Loop in the DOM
JavaScript’s event loop is essential for understanding asynchronous behavior. When users interact with the DOM, JavaScript places these interactions in a queue, processing them in order. This keeps the UI responsive and allows JavaScript to handle multiple interactions without freezing.
Conclusion and Key Takeaways
Understanding the DOM is critical for creating dynamic web applications. By learning to select, modify, and respond to elements on a page, developers unlock the full potential of JavaScript to build engaging experiences.
Further Resources on JavaScript DOM
MDN Web Docs: Introduction to the DOM – Comprehensive introduction to DOM concepts.
W3Schools: JavaScript HTML DOM – Guide on various DOM methods and properties.
JavaScript.info: The DOM – In-depth look into the DOM tree and traversal.
Happy Coding !!
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
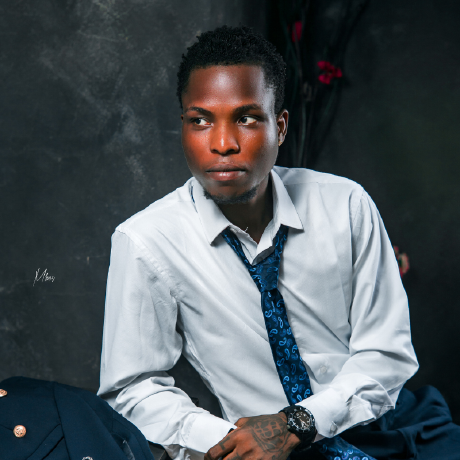
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com