Keep It Simple, Keep It Separate: A Beginner's Guide to Interface Segregation in Laravel

Table of contents
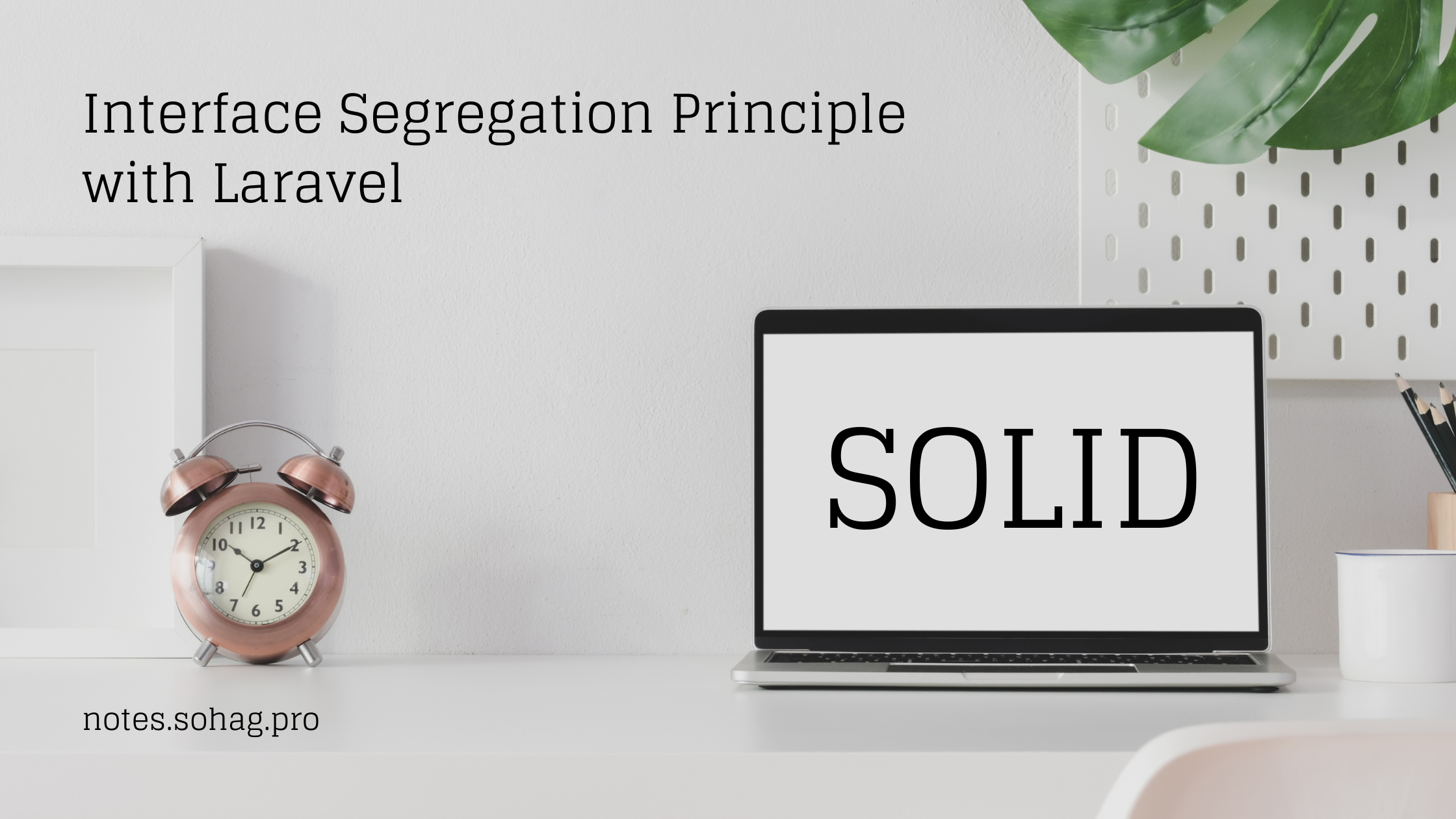
Interface Segregation Principle in Laravel
Have you ever been to a restaurant where the menu is so huge that it takes forever to find what you want? Or used a TV remote with 50 buttons when you only need 5? That's exactly what the Interface Segregation Principle (ISP) helps us avoid in programming. Let's dive into how we can apply this principle in Laravel applications with real-world examples.
What is Interface Segregation Principle?
The Interface Segregation Principle states that "clients should not be forced to depend upon interfaces they do not use." In simpler terms, it's better to have multiple smaller, specific interfaces than one large, general-purpose interface.
Think of it like this: Instead of having one super-employee who needs to know everything, we create specialized roles where each person excels at their specific tasks.
A Real-World Example: Online Bookstore
Let's say we're building an online bookstore. Without ISP, we might create something like this:
interface BookRepositoryInterface
{
public function findById(int $id): Book;
public function save(Book $book): void;
public function delete(int $id): void;
public function processPayment(float $amount): bool;
public function sendEmail(string $to, string $subject, string $body): void;
public function generatePDF(Book $book): string;
public function calculateDiscount(float $price): float;
}
This is problematic because any class implementing this interface must implement ALL methods, even if it only needs a few. It's like hiring a bookstore clerk and requiring them to be an accountant, marketing specialist, and delivery person all at once!
Better Approach: Segregated Interfaces
Let's break this down into smaller, focused interfaces:
interface BookRepositoryInterface
{
public function findById(int $id): Book;
public function save(Book $book): void;
public function delete(int $id): void;
}
interface PaymentProcessorInterface
{
public function processPayment(float $amount): bool;
}
interface EmailServiceInterface
{
public function sendEmail(string $to, string $subject, string $body): void;
}
interface DocumentGeneratorInterface
{
public function generatePDF(Book $book): string;
}
interface DiscountCalculatorInterface
{
public function calculateDiscount(float $price): float;
}
Practical Implementation in Laravel
Let's see how we can implement these interfaces in a real Laravel application:
class MySQLBookRepository implements BookRepositoryInterface
{
public function findById(int $id): Book
{
return Book::findOrFail($id);
}
public function save(Book $book): void
{
$book->save();
}
public function delete(int $id): void
{
Book::destroy($id);
}
}
class StripePaymentProcessor implements PaymentProcessorInterface
{
public function processPayment(float $amount): bool
{
// Stripe payment processing logic
return true;
}
}
class OrderService
{
private $bookRepository;
private $paymentProcessor;
public function __construct(
BookRepositoryInterface $bookRepository,
PaymentProcessorInterface $paymentProcessor
) {
$this->bookRepository = $bookRepository;
$this->paymentProcessor = $paymentProcessor;
}
public function purchaseBook(int $bookId, float $amount): bool
{
$book = $this->bookRepository->findById($bookId);
if ($this->paymentProcessor->processPayment($amount)) {
// Process successful purchase
return true;
}
return false;
}
}
Service Provider Registration
In Laravel, we can bind these interfaces to their implementations in a service provider:
class BookstoreServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind(BookRepositoryInterface::class, MySQLBookRepository::class);
$this->app->bind(PaymentProcessorInterface::class, StripePaymentProcessor::class);
}
}
Benefits of Using ISP in Laravel
Maintainability: Smaller interfaces are easier to implement and maintain
Flexibility: Easy to swap implementations (e.g., switching from Stripe to PayPal)
Testing: Simpler to mock small interfaces for unit tests
Clarity: Clear separation of concerns makes code more understandable
Scalability: Easier to add new features without modifying existing code
Potential Drawbacks
More Files: You'll have more interface files to manage
Initial Complexity: Might seem overwhelming for very small projects
Over-segregation: Risk of creating too many tiny interfaces
Real-World Scenario: Adding a New Payment Method
Let's say we want to add PayPal as a payment option. With ISP, it's as simple as creating a new implementation:
class PayPalPaymentProcessor implements PaymentProcessorInterface
{
public function processPayment(float $amount): bool
{
// PayPal payment processing logic
return true;
}
}
Then update the service provider:
class BookstoreServiceProvider extends ServiceProvider
{
public function register()
{
// ... other bindings ...
// Use environment variable to determine payment processor
$this->app->bind(PaymentProcessorInterface::class, function ($app) {
return config('payment.processor') === 'paypal'
? new PayPalPaymentProcessor()
: new StripePaymentProcessor();
});
}
}
Best Practices for Using ISP in Laravel
Keep Interfaces Focused: Each interface should serve a single purpose
Follow Laravel's Conventions: Use Laravel's service container and dependency injection
Use Type-Hinting: Always type-hint interfaces in your constructors and methods
Document Your Interfaces: Use PHPDoc to clearly document interface methods
Consider Package Development: When creating Laravel packages, use ISP to make them more flexible
Conclusion
The Interface Segregation Principle might seem like extra work at first, but it's like organizing your toolbox โ everything has its place, and you can find exactly what you need when you need it. In Laravel applications, ISP helps create more maintainable, testable, and flexible code that can grow with your project.
Remember: Start with larger interfaces if you're unsure, and refactor to smaller ones as patterns emerge in your code. It's better to have a working system that violates ISP than a perfectly segregated system that doesn't work at all!
Happy coding! ๐
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author