Mastering the Dependency Inversion Principle in Laravel: A Practical Guide for Beginners

Table of contents
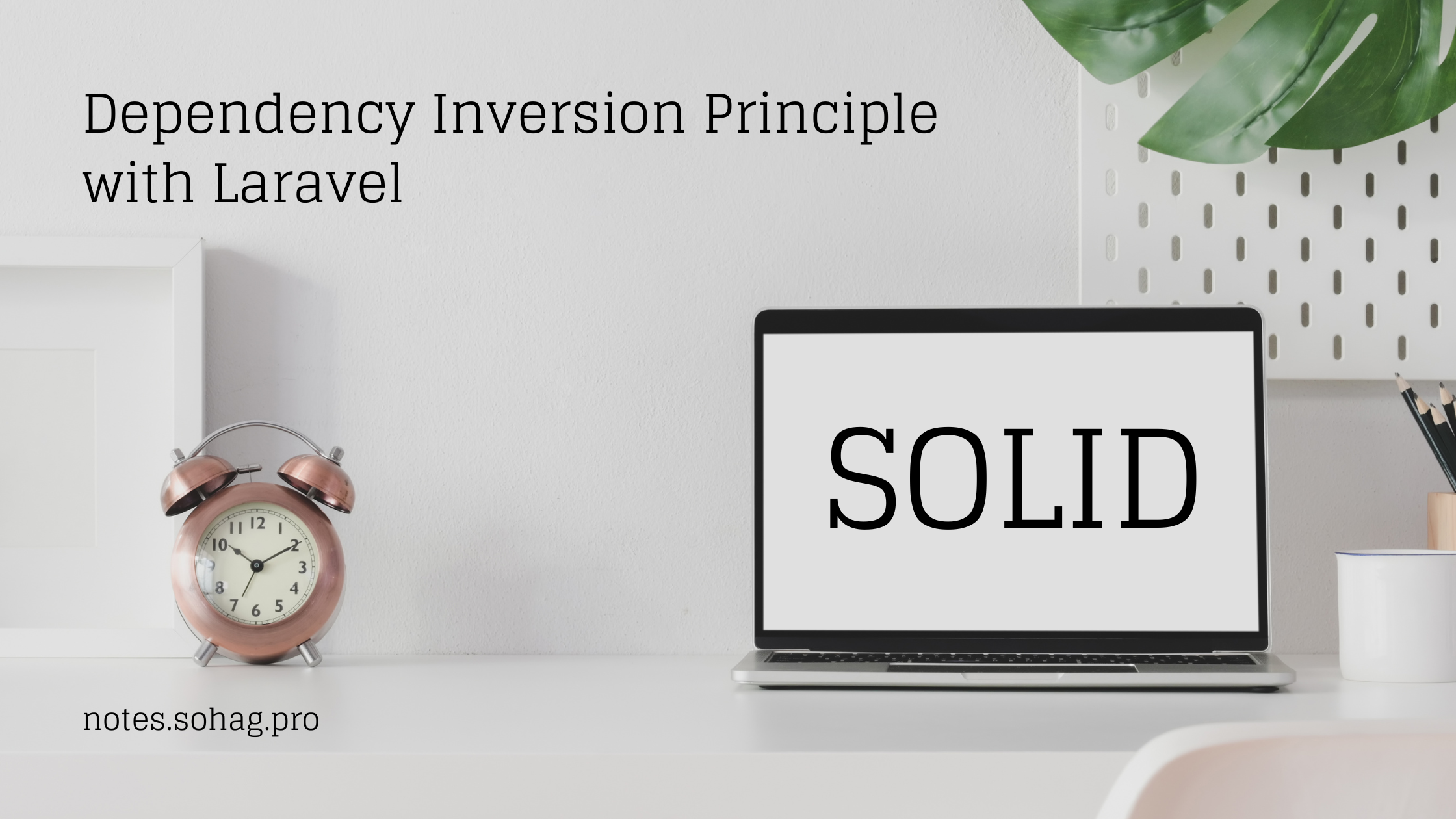
Dependency Inversion Principle in Laravel
Have you ever struggled with tightly coupled code that's difficult to maintain and test? The Dependency Inversion Principle (DIP) is here to save the day! In this guide, we'll explore how DIP can make your Laravel applications more flexible and maintainable using real-world examples.
What is the Dependency Inversion Principle?
The Dependency Inversion Principle is one of the SOLID principles of object-oriented design. It states that:
High-level modules should not depend on low-level modules. Both should depend on abstractions.
Abstractions should not depend on details. Details should depend on abstractions.
Sounds complicated? Let's break it down with a real-world example!
Real-World Analogy: The Coffee Shop
Imagine you're running a coffee shop. Initially, you might have code that looks like this:
class CoffeeShop
{
private $traditionalCoffeeMaker;
public function __construct()
{
$this->traditionalCoffeeMaker = new TraditionalCoffeeMaker();
}
public function brewCoffee()
{
return $this->traditionalCoffeeMaker->brew();
}
}
class TraditionalCoffeeMaker
{
public function brew()
{
return 'Brewing traditional coffee...';
}
}
This code violates DIP because our CoffeeShop
class is tightly coupled to the TraditionalCoffeeMaker
. What happens when we want to add an espresso machine or a cold brew maker?
Applying DIP to Our Coffee Shop
Let's refactor this code following DIP:
interface CoffeeMakerInterface
{
public function brew(): string;
}
class TraditionalCoffeeMaker implements CoffeeMakerInterface
{
public function brew(): string
{
return 'Brewing traditional coffee...';
}
}
class EspressoMachine implements CoffeeMakerInterface
{
public function brew(): string
{
return 'Brewing espresso...';
}
}
class ColdBrewMaker implements CoffeeMakerInterface
{
public function brew(): string
{
return 'Preparing cold brew...';
}
}
class CoffeeShop
{
private $coffeeMaker;
public function __construct(CoffeeMakerInterface $coffeeMaker)
{
$this->coffeeMaker = $coffeeMaker;
}
public function brewCoffee(): string
{
return $this->coffeeMaker->brew();
}
}
Implementing in Laravel with Service Container
Laravel makes it easy to implement DIP using its powerful Service Container. Here's how:
// AppServiceProvider.php
namespace App\Providers;
use App\Services\Coffee\CoffeeMakerInterface;
use App\Services\Coffee\TraditionalCoffeeMaker;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->bind(CoffeeMakerInterface::class, function ($app) {
return new TraditionalCoffeeMaker();
});
}
}
Now you can use dependency injection in your controllers:
class CoffeeController extends Controller
{
private $coffeeShop;
public function __construct(CoffeeShop $coffeeShop)
{
$this->coffeeShop = $coffeeShop;
}
public function brew()
{
return response()->json([
'message' => $this->coffeeShop->brewCoffee()
]);
}
}
A More Complex Example: Order Processing System
Let's look at a more practical example involving order processing:
interface OrderProcessorInterface
{
public function process(Order $order): bool;
}
interface PaymentGatewayInterface
{
public function charge(float $amount, string $currency): bool;
}
class StripePaymentGateway implements PaymentGatewayInterface
{
public function charge(float $amount, string $currency): bool
{
// Stripe-specific implementation
return true;
}
}
class PayPalPaymentGateway implements PaymentGatewayInterface
{
public function charge(float $amount, string $currency): bool
{
// PayPal-specific implementation
return true;
}
}
class OrderProcessor implements OrderProcessorInterface
{
private $paymentGateway;
private $logger;
public function __construct(
PaymentGatewayInterface $paymentGateway,
LoggerInterface $logger
) {
$this->paymentGateway = $paymentGateway;
$this->logger = $logger;
}
public function process(Order $order): bool
{
try {
$success = $this->paymentGateway->charge(
$order->total_amount,
$order->currency
);
if ($success) {
$this->logger->info("Order {$order->id} processed successfully");
return true;
}
$this->logger->error("Failed to process order {$order->id}");
return false;
} catch (\Exception $e) {
$this->logger->error("Error processing order: " . $e->getMessage());
return false;
}
}
}
Pros of Using DIP
Flexibility: Easy to swap implementations without changing the core business logic
Testability: Makes unit testing easier with mock objects
Maintainability: Reduces coupling between modules
Scalability: New implementations can be added without modifying existing code
Code Organization: Clear separation of concerns and better architecture
Cons and Challenges
Initial Complexity: More interfaces and classes to manage
Learning Curve: Takes time to understand and implement properly
Over-abstraction: Risk of creating unnecessary abstractions
Setup Time: Requires more initial setup and planning
Best Practices
Keep Interfaces Simple: Design interfaces with the minimum necessary methods
Use Meaningful Names: Make your abstractions and implementations clear and descriptive
Don't Over-abstract: Only create abstractions when you expect multiple implementations
Use Laravel's Service Container: Take advantage of Laravel's built-in DI container
Testing with DIP
DIP makes testing much easier. Here's an example:
class OrderProcessorTest extends TestCase
{
public function test_order_processing_with_successful_payment()
{
// Create mock payment gateway
$paymentGateway = $this->createMock(PaymentGatewayInterface::class);
$paymentGateway->method('charge')->willReturn(true);
// Create mock logger
$logger = $this->createMock(LoggerInterface::class);
// Create order processor with mocks
$processor = new OrderProcessor($paymentGateway, $logger);
// Create test order
$order = new Order([
'total_amount' => 100.00,
'currency' => 'USD'
]);
// Assert
$this->assertTrue($processor->process($order));
}
}
Conclusion
The Dependency Inversion Principle might seem complex at first, but it's a powerful tool for creating maintainable and flexible Laravel applications. By depending on abstractions rather than concrete implementations, we can create code that's easier to test, maintain, and extend.
Remember: Start small, identify clear use cases for abstraction, and gradually incorporate DIP into your projects. Your future self (and your team) will thank you for it!
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author