A Complete Guide to React Hooks: Everything You Need to Know

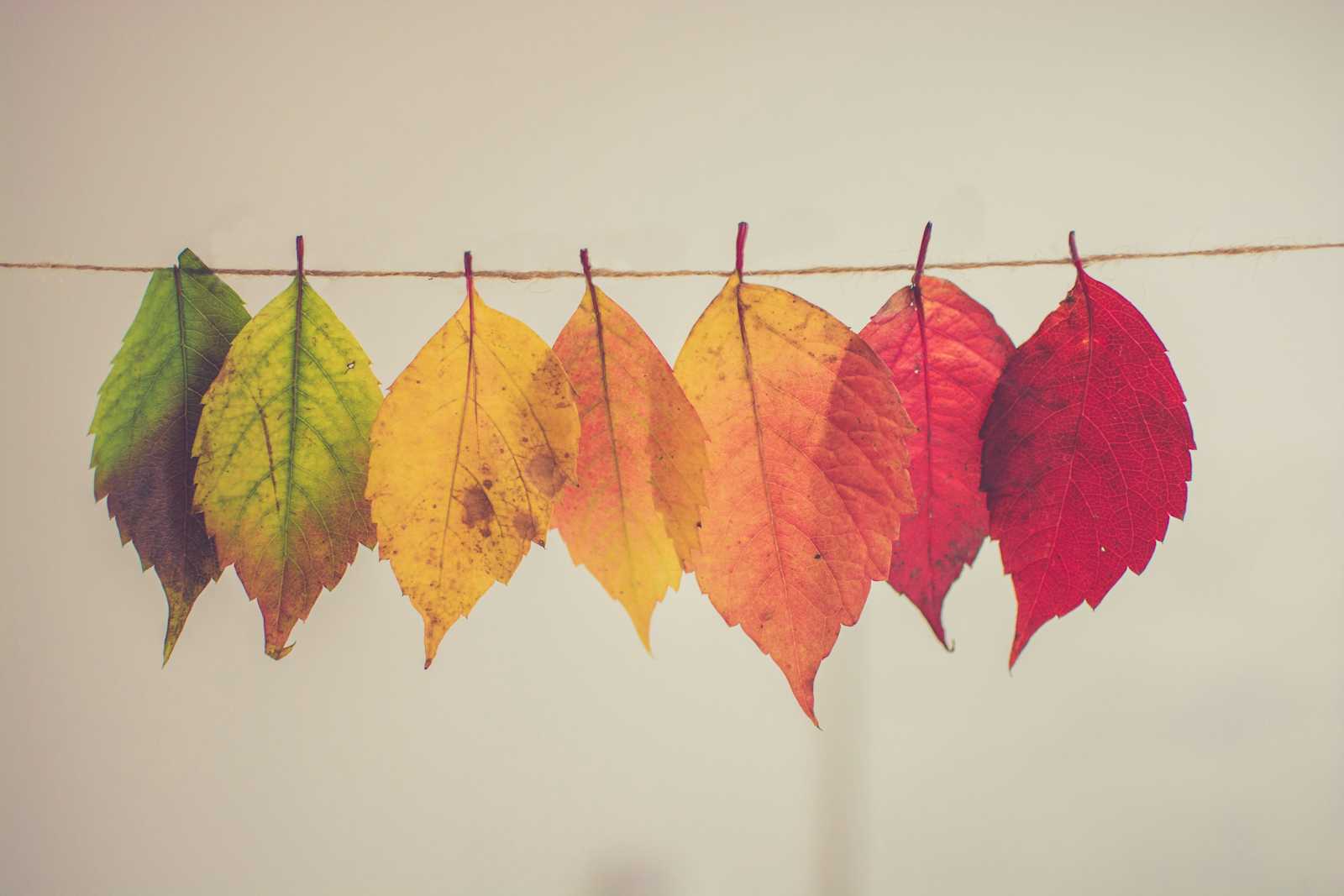
Introduction to React Hooks
React Hooks, introduced in version 16.8, transformed the way we manage state and lifecycle in functional components. Before their arrival, class components were the go-to for handling these features, often resulting in more complex and less reusable code. Hooks changed that by enabling state management, side effects, and more within functional components, making code cleaner and easier to maintain.
This guide will walk you through all the essential React hooks, from the basics like useState and useEffect to more advanced hooks like useReducer and useMemo, as well as how to create custom hooks. Whether you're just starting with React or looking to enhance your knowledge, this article will show you how to leverage hooks for more efficient and modular code.
Why React Hooks?
Before hooks, React relied heavily on class components for managing state and lifecycle methods. While class components were effective, they came with several challenges
1. Complexity: Class components often became difficult to manage, especially as state and logic grew more complex. Developers had to deal with issues like this binding, and logic was sometimes split between different lifecycle methods, making the code harder to follow.
2. Code Reusability: Sharing logic between components required using patterns like Higher-Order Components (HOCs) or render props, which could add unnecessary complexity and boilerplate code. Reusing stateful logic across components wasn’t straightforward.
3. Verbose Syntax: Class components introduced more boilerplate and syntax, like constructors and lifecycle methods, which made the code verbose and harder to read, especially for beginners.
React hooks solve these problems by:
Simplifying State Management: Hooks like useState allow you to easily manage state in functional components without needing class-based components.
Handling Side Effects: The useEffect hook streamlines how you manage side effects, replacing several lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount with a single, more intuitive API.
Encouraging Code Reusability: Custom hooks allow you to extract and reuse logic across multiple components, making your code more modular and maintainable.
Improving Readability: With hooks, functional components are concise and easier to read, making it simpler for developers to reason about their code.
By introducing hooks, React allowed developers to write cleaner, more efficient code, which in turn has made building and scaling applications much easier. Let’s now dive into the most commonly used hooks, starting with the basics.
Core Hooks
1. useState – Manages state in functional components.
2. useEffect – Handles side effects like fetching data or subscriptions.
3. useContext – Consumes context values without needing Consumer components.
Additional Hooks
4. useReducer – Manages more complex state logic and actions, similar to Redux.
5. useCallback – Memoizes functions to prevent unnecessary re-creations.
6. useMemo – Memoizes computed values to optimize performance.
7. useRef – Provides a way to access and persist DOM elements or values between renders.
8. useImperativeHandle – Customizes the instance value that is exposed to parent components when using ref.
9. useLayoutEffect – Similar to useEffect, but fires synchronously after all DOM mutations (used for measurements).
10. useDebugValue – Used for displaying custom hook values in React DevTools.
Special Hooks for Concurrent Mode
11. useTransition – Allows marking updates as non-urgent, improving UI responsiveness.
12. useDeferredValue – Defers updating a value until there’s less urgency, useful for delaying non-critical renders.
13. useId – Generates unique IDs for accessibility and server-side rendering purposes.
14. useSyncExternalStore – Allows subscribing to external stores, ensuring consistent state between renders.
Subscribe to my newsletter
Read articles from harshad dhongade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
