Shell-Basics
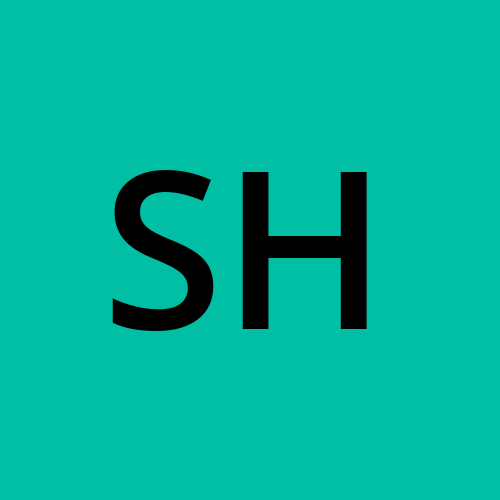

What is a shell? A shell is a program on our computer that lets you interact with your operating system. Shell is of two types one is command line which uses human readable commands and the other is graphical user interface. They’re many different types of shells out there like BASH(Bourne Again SHell), KornShell, command-line etc. To use shell on our computer, we would need a terminal installed on the computer which mostly will already be installed if not it can be downloaded and installed as well.
When you first launch your terminal, you will see a prompt like the below:
This means that the userprofile of the computer sharo and the computer name given is SharonEarnest and the MINGW64 is the version of Git that I’m using. ~ this tells us about the current directory which is the home. $ tells us that I’m not a root user.
Echo executes the arguments we pass after it. We can use ‘ or “ to have it print a word or argument that has space between them. You can even use escape \ as well.
Now how does the shell know how to execute those commands? How does it know that when I type echo followed by something, it should print those arguments? Shell is itself a programming language like Python and it has its own variables, loops and functions. When type a command it means that we are writing a line of code that the shell needs to understand and take action. Which means when I write a command, it checks the $PATH variable which is called the environment variable. This variable has all the directories listed in it for the shell to look for the programs and run it. So when I type echo it checks the $PATH variable for the command and then executes that program. If it doesn’t find the command then throws an error saying command not found.
These are all the directories that the PATH variable has so when you type a command the shell checks in all of these directories and executes the program to give the output.
We can also find from which directory the command was executed using the command which. Also, we can skip for the shell to search the $PATH variable by entering the directory of the command itself.
On Linux and macOS the path is separated by a ‘/’ while in windows it is ‘\’
In Linux and macOS / means that all the files and folders are under this root file system.
In Windows every disk has its own root for an example the C:\There are two types of paths: absolute and relative paths.
Absolute: These start from the root of the systemRelative: They’re relevant to the current directory
When we enter cd . means it refers to the current directory but when we enter cd . . means it refers to the parent directory.
cd . doesn’t change your location, it lets you stay in the current location only.
ls to see what the directory contains.
cat to see the contents of the file.
Many commands use flags and options that start with - that is used to modify their behaviour.
To see the flags and options that can be used for a particular command we can use the command followed by -h or - -help command.
If you notice when I entered the command ls in the current directory it gave me the list of all the files and folders in that directory but when I used ls -l command it gave me a long list of the files and directories with their time and date and the permissions as well. Also, we use the command ls -a command to view the hidden files and folders.
Let’s talk about permissions now. For example when we see drwxer-xr-x 1 for a file or folder. This means that the first letter d indicates that it is a folder and the next 3 set of letters rwx talks about the permissions the owner, group and other users have on that particular folder.
If the first letter is - then it is a regular file and there are many more indicators like this.
In the above example, it is a directory. The next 3 sets of letters indicate that rwx, which means the owner of the file has all the access to read(r), write(w) and execute (x) the file. When it comes to r-x this means that the users who are part of the same group as the file or directory have read and execute access only but not write to the file or folder. r-x means all other users have read and execute access the same as the group members.
mv command is used to move the files or rename the files.
cp command is used to copy a file.
mkdir command is used to create a new directory.
man command gives more information about a command.
**Redirections:
**> you send the output to a file instead of printing it to the terminal. If the file doesn’t exist, the shell creates it. If it does, the shell overwrites it.
\>> using this also you send the output to a file instead of printing it to the terminal.
However, the shell doesn’t overwrite it but appends the output to the last line of the file.
< with this you have the input read from a file.
< > we can combine both input and output redirects which read the contents from one file and add it to the other file. Which basically works like copy and paste.
Root User: Root user is a superuser which means they’ve access to all the files to read, write and execute. Since this has a high level of access, logging in as root is not recommended. Instead of performing executions on root directly, we can use sudo command to execute the commands as root user.
/sys: It is a special folder that has kernel settings as files. Like we can adjust the brightness of the computer using this directory. For this, that is to make changes to any kernel settings available in this directory when we execute the commands using sudo, even then it will say **permission is denied.
**
This is because the redirection operators are executed by the shell itself and not the commands which we use as in the example echo. So, since the shell itself doesn’t run in the superuser mode, it throws the permission denied error when trying to read the brightness file. To resolve this issue we use the command tee that is used to open the file to write to it and since we will add sudo to it as well, it will execute the command without any error.
GREP: It stands for Global Regular Expression Print. It is used to search through text or files for any specific patterns and returns the lines that match.
Example : when we type grep “hello “ hello.txt. This will search for the word hello in the entire file hello.txt and will print each line that contains that word.
This command is case sensitive, if we type the above command then it will only print the lines with the word hello in it and not HELLO.
However,using the option -i we can print the Hello irrespective of being case sensitive. Meaning grep -i “hello” hello.txt will print hello, HELLO, Hello etc.
Option -c will print the number of lines that contain the word hello in the text file.
Option -n will show each line with the line number where the pattern is found.
Option -v will show all the lines except for the pattern you gave as input in the command.
Option -r will search for the pattern in all the files and directories in the path that you mentioned and displays the file name and the line where the match is found. If we don’t want to specify the path we can simply type the dot(.) symbol which will indicate it to search in the current directory.
Option -o will only show the parts of the lines that match the pattern entered and not the entire line.
Option -w will match the entire word in the file and line.
Egrep will provide all the lines that match either of the pattern entered after the pipe or before the pipe from the file.
Option -I ignores binary files to prevent unreadable characters from being displayed.
-A any number shows lines after the pattern match. As in if word hello is in the first line, it will show the lines after the line match according to the number u pass after the option -A.
-B any number shows lines before the pattern match. As in if word hello is in the third line, it will show the lines before the line match according to the number you have after the option -B.
-C any number shows lines before and after the pattern match. As in if word hello is in the third line, it shows the lines before the line match and after the line match according to the number you enter after the option -C.
**Regular expressions in grep
**1)Dot(.) - matches any single character except the new line.
2)Asterisk (*): Matches zero or more of the preceding character.
3)Caret (^): Matches the beginning of a line.
4)Dollar ($): Matches the end with the pattern you enter.
5)Brackets ([]): Matches any one of the characters in brackets.
6)Escape Character (\): Use this to escape special characters.
Grep with pipes: We can combine grep with other commands using pipes |
Example: ls -l | grep "^d" which will show all the directories in the current directory.
You can search all multiple files by mentioning the names of it or also just using the asterisk followed by the file type.
Important
Executing a script directly with ./example.sh requires execute permission.
Running a script using an interpreter like sh example.sh or bash example.sh only requires read permission, as the shell is responsible for executing the commands in the script.
Subscribe to my newsletter
Read articles from Sharon Jonallagadda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
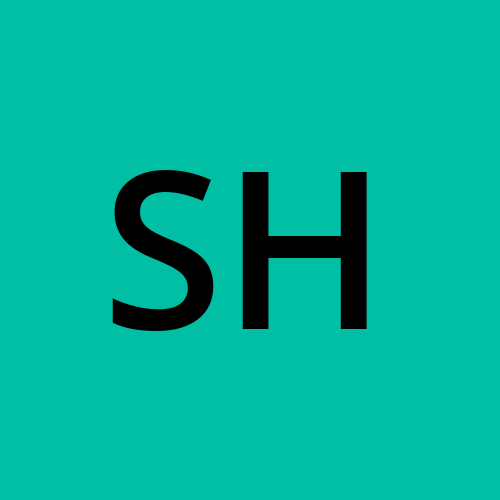
Sharon Jonallagadda
Sharon Jonallagadda
Pursuing my Master’s in IT. Documenting my journey as I work towards securing an internship and a full-time role. Sharing the knowledge and experiences I gain along the way.