Implementing Dynamic Routes in React with react-router-dom: A Practical Guide.
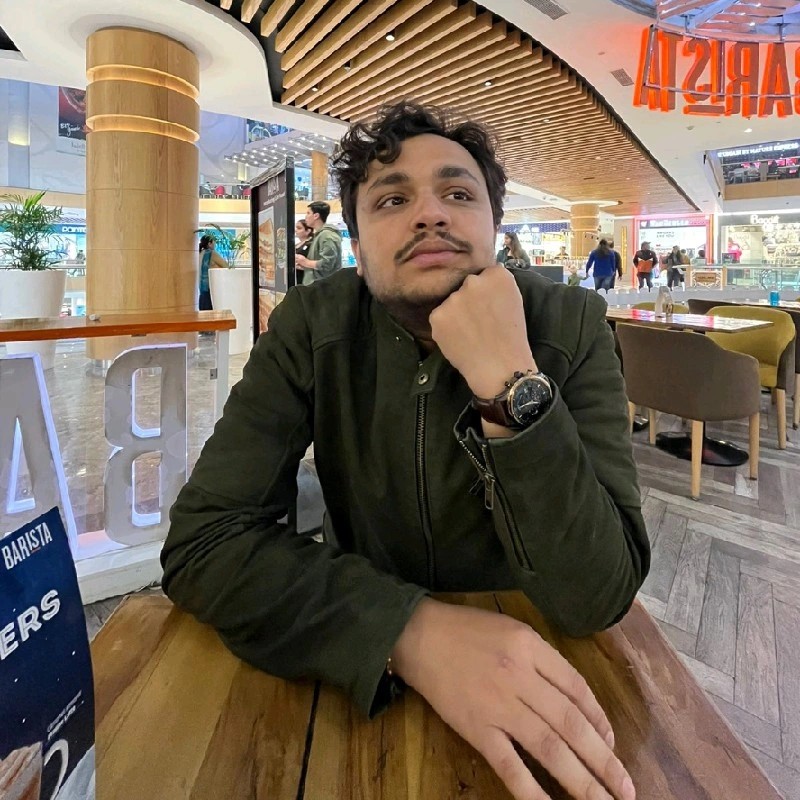
Introduction to react-router-dom
When building web applications with React, navigating between different pages or views within a single-page application (SPA) is crucial. React Router DOM, an essential library in the React ecosystem, allows developers to implement client-side routing seamlessly. This library provides the tools to handle routing within an SPA, enabling dynamic URL management and allowing users to navigate without needing a full page reload.
Why Use react-router-dom
?
SPA Navigation: Without
react-router-dom
, SPAs would require custom implementations for navigating and displaying different views, which can be inefficient and complex.User Experience:
react-router-dom
ensures that pages load faster by handling the routes directly on the client side, enhancing the user experience with smooth transitions.URL Management: It allows developers to build meaningful and descriptive URLs, which can improve accessibility, sharing, and SEO in some cases.
Dynamic Routing: Enables the use of dynamic URLs to handle various resources, like displaying a specific user profile or blog post without hard-coding each route.
When to Use react-router-dom
?
Use react-router-dom
whenever you need to build a multi-page application or require navigation between different sections of an app. This library is ideal for:
E-commerce platforms where you need to navigate between product listings and product details.
Blogs or news apps where each article or category has its own page.
Social media applications where user profiles, posts, and interactions are accessible via distinct URLs.
Implementing Dynamic Routes with react-router-dom
In my recent project, I implemented a dynamic routing system using react-router-dom
to manage comments based on their unique commentId
. Here’s how I set it up:
Step 1: Setting Up the Route
To create a dynamic route for comments, I used the <Route>
component with a path that includes a parameter, :commentId
. The colon (:
) before commentId
specifies that this part of the URL will be dynamic. Here’s how I defined the route:
<Route path="/comment/:commentId" element={<CommentComponent />} />
In this setup:
/comment/:commentId
is the path, wherecommentId
can be any unique identifier.<CommentComponent />
is the component that renders whenever this route is matched.
With this route configuration, whenever a user navigates to a URL like /comment/123
, CommentComponent
is rendered, and 123
is passed as the commentId
parameter.
Step 2: Navigating with the Link
Component
To navigate to this dynamic route, I used the Link
component from react-router-dom
. This is preferable over traditional anchor tags (<a>
) because Link
provides smooth, client-side navigation without refreshing the page. Here’s how I set up the link to navigate to a specific comment:
<Link to={`/comment/${_id}`}>View Comment</Link>
In this code:
to={
/comment/${_id}}
dynamically inserts the comment’s unique ID, allowing users to navigate directly to the appropriate comment page.Wrapping the element inside
Link
makes it clickable, so when users click "View Comment," they’re taken to/comment/:commentId
.
Step 3: Accessing the Dynamic Route Parameter with useParams
Once on the CommentComponent
page, I needed to access the commentId
to fetch or display relevant information about the specific comment. The useParams
hook provided by react-router-dom
is perfect for this.
Here’s how I used useParams
to retrieve commentId
within CommentComponent
:
import { useParams } from 'react-router-dom';
const CommentComponent = () => {
const { commentId } = useParams();
return (
<div>
<h2>Comment Details for ID: {commentId}</h2>
</div>
);
};
With useParams
, I accessed the dynamic parameter directly within CommentComponent
, enabling me to use commentId
to fetch or display data unique to that specific comment. This approach helps in building highly dynamic and flexible applications without the need for hardcoding multiple routes.
Summary
Implementing dynamic routes with react-router-dom
is a powerful technique in React for building scalable applications. Here’s a quick recap of the steps we covered:
Defining a Dynamic Route using
<Route>
with a parameter (e.g.,:commentId
).Navigating with
Link
to dynamically create paths based on unique IDs.Accessing the Dynamic Parameter within the target component using
useParams
.
By leveraging these tools, you can easily build applications that are not only efficient but also highly adaptable to user-specific data and page navigation.
Using react-router-dom
in this way has helped streamline my project’s structure, ensuring a smooth and interactive user experience. Give it a try in your next React project!
Subscribe to my newsletter
Read articles from Ravi Shankar Pratihast directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
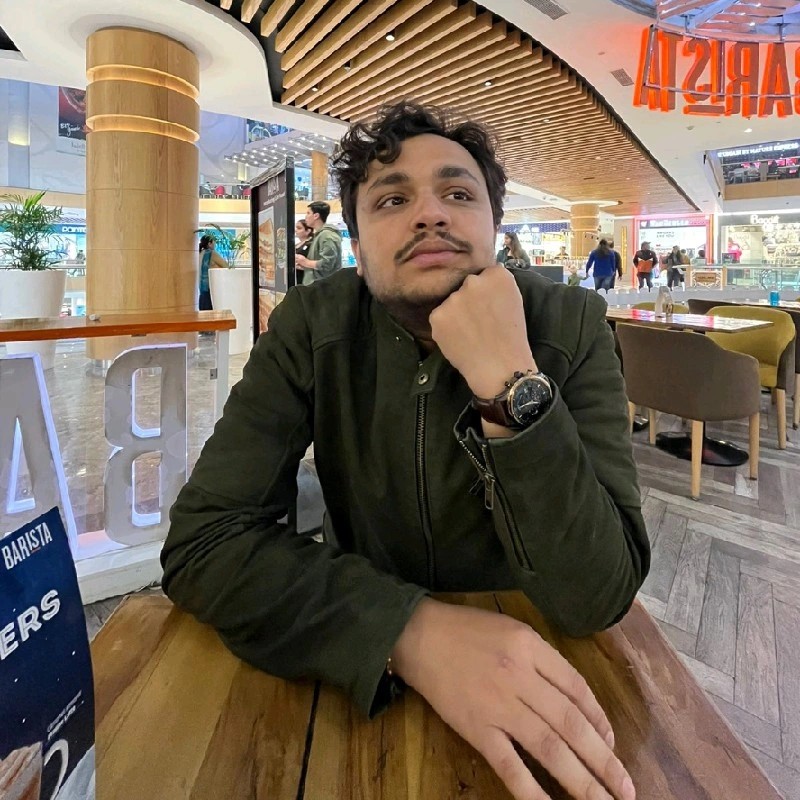
Ravi Shankar Pratihast
Ravi Shankar Pratihast
I am a dedicated frontend engineer with 1.5 years of experience specializing in React.js. My expertise includes developing user-friendly interfaces, efficiently managing application state with Redux, and ensuring seamless integration of front-end components with back-end systems through RESTful APIs. I am adept at implementing responsive design principles to create applications that perform optimally across various devices and browsers. I am also committed to continuous learning, staying updated on industry trends, and optimizing applications for speed and scalability.