Supercharge Your React Apps: Dynamic Routing with "useParams"
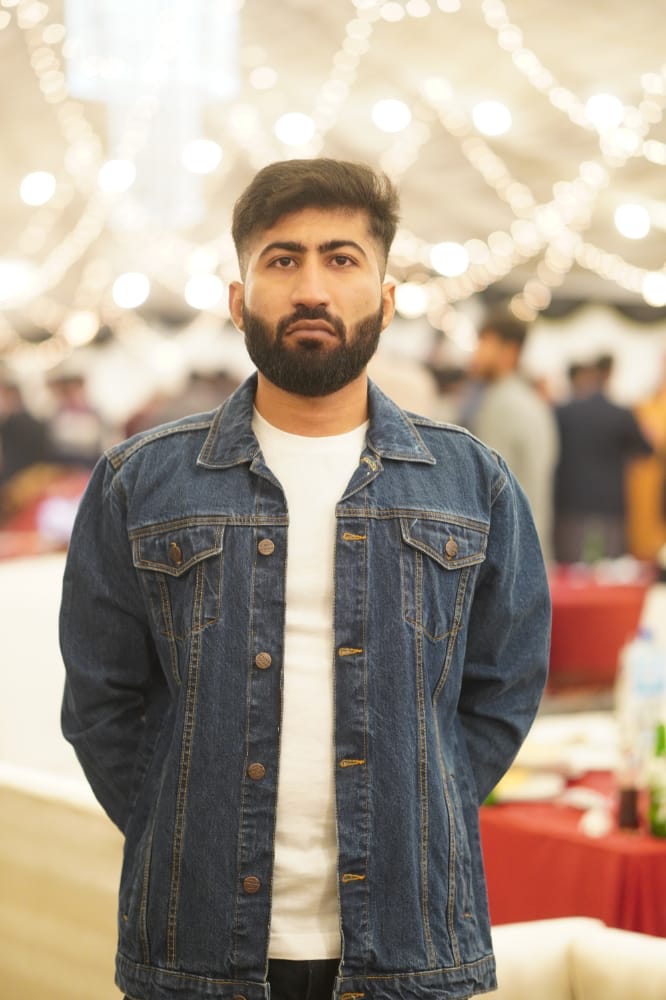
The useParams
hook is a part of React Router and is used to access URL parameters in your application. It's very useful when you have dynamic routes—URLs that include variables, such as user IDs or post IDs, and you want to access those parameters in your component.
How useParams
Works
useParams
returns an object of key-value pairs from your route parameters. For example, if you have a route with /:id
, the id
parameter in the URL can be accessed using useParams
.
Example: User Profile Page
Suppose you have a route where each user has a unique profile page with a URL structure like /profile/:userId
. The userId
is a dynamic parameter in the URL that identifies which user's profile to display.
Here’s a complete example of how to use useParams
to get the userId
and fetch user data based on it.
Step 1: Set Up Routes with Dynamic Parameters
First, define your routes, including a dynamic route for the user profile.
// App.js
import React from 'react';
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import Home from './Home';
import UserProfile from './UserProfile';
function App() {
return (
<Router>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/profile/:userId" element={<UserProfile />} />
</Routes>
</Router>
);
}
export default App;
In this setup:
"/"
is the home route."/profile/:userId"
is the dynamic route where:userId
will vary based on the user.
Step 2: Access URL Parameters with useParams
Now, inside the UserProfile
component, use useParams
to access userId
and display data accordingly.
// UserProfile.js
import React, { useEffect, useState } from 'react';
import { useParams } from 'react-router-dom';
const UserProfile = () => {
const { userId } = useParams(); // Access the dynamic parameter userId
const [user, setUser] = useState(null);
useEffect(() => {
// Fetch user data based on userId
const fetchUser = async () => {
try {
const response = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`);
const data = await response.json();
setUser(data);
} catch (error) {
console.error("Error fetching user data:", error);
}
};
fetchUser();
}, [userId]); // Dependency array ensures fetch happens on userId change
if (!user) return <p>Loading user information...</p>;
return (
<div>
<h1>User Profile</h1>
<h2>{user.name}</h2>
<p>Email: {user.email}</p>
<p>Username: {user.username}</p>
</div>
);
};
export default UserProfile;
Explanation of the Code
useParams()
: This hook extracts theuserId
from the URL.Fetching Data: Inside
useEffect
, we fetch user data when theuserId
changes, ensuring it re-fetches if a differentuserId
is provided.Displaying Data: Once data is loaded,
user
information is displayed.
How It Works in Action
When the user navigates to
/profile/1
,useParams
returns{ userId: "1" }
.userId
is used to fetch data for user1
from the API.The
UserProfile
component renders details for that specific user.
Benefits of useParams
Dynamic Routing: Essential for apps with unique pages based on URL parameters.
Reactiveness: When the parameter changes, the component re-renders, enabling dynamic content updates.
Subscribe to my newsletter
Read articles from Asawer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
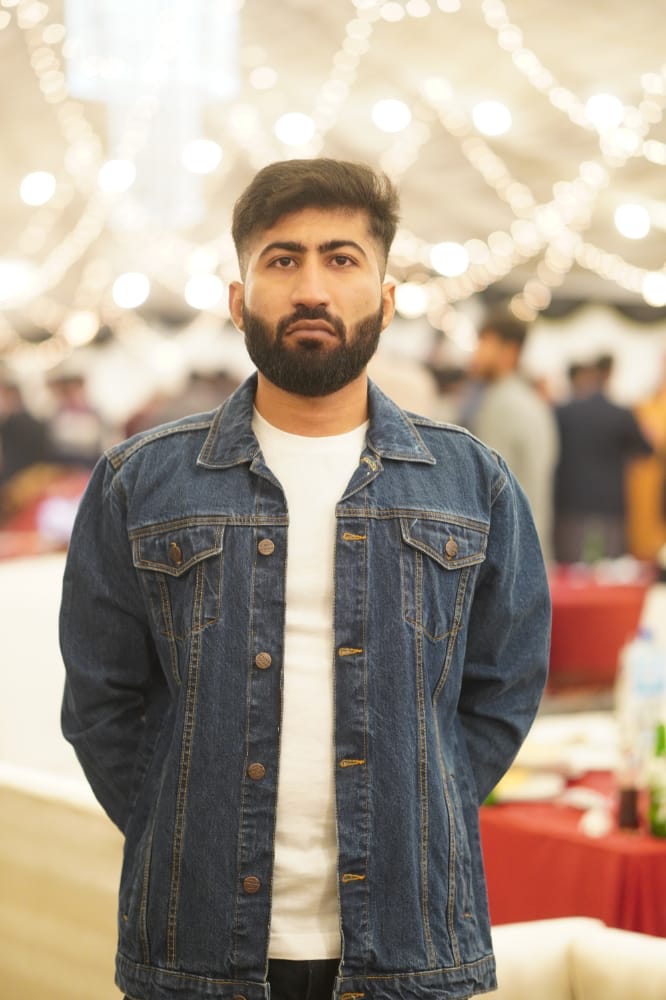