Single Responsibility - Clean Mobile Architecture by Petros Efthymiou
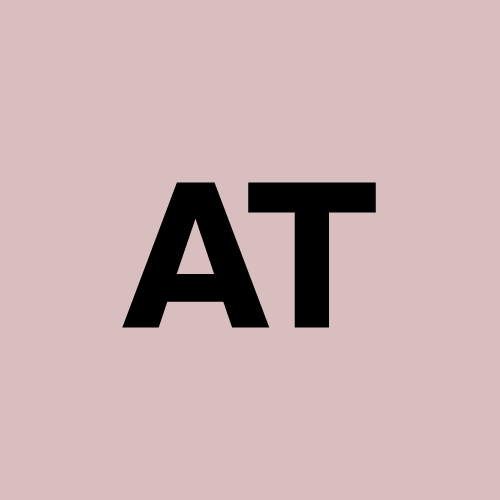
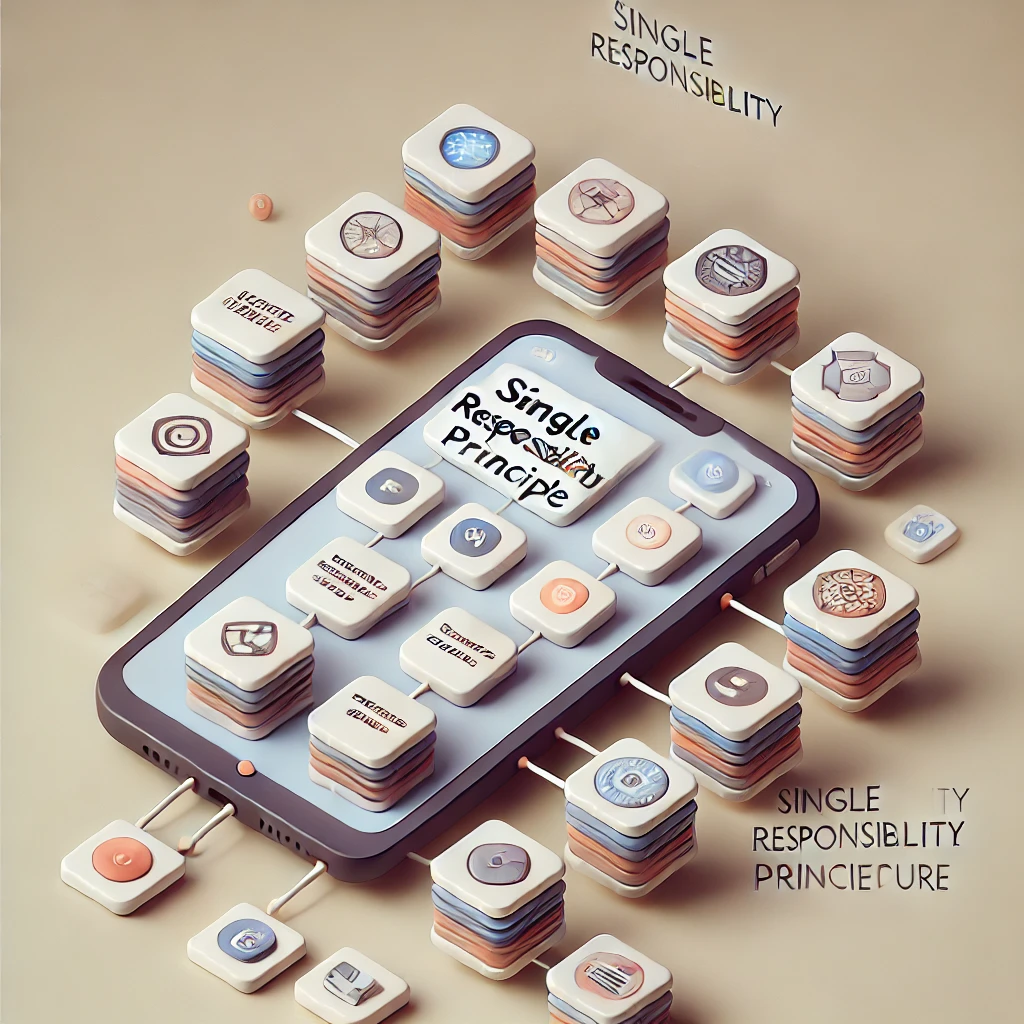
The Single Responsibility Principle (SRP) is a core concept in software design, emphasizing that every class or module should have only one reason to change. In mobile development, this translates to having components with focused responsibilities, making the code easier to understand, maintain, and test.
What is the Single Responsibility Principle (SRP)?
Definition: The Single Responsibility Principle states that a class or module should only have one reason to change, meaning it should have one job or responsibility.
By following SRP, you ensure that each part of your application is modular and performs a specific function, avoiding code that is too complex or does too many things at once.
Problem Scenario
Imagine you are developing a mobile app that handles user authentication, data storage, and network communication. If all these responsibilities are handled by a single class, the code would be difficult to maintain, modify, or test. For example, changes to how you manage user data storage could affect network communication if they're coupled together, leading to bugs and unpredictable behavior.
To improve the design, you can apply SRP and separate these responsibilities into distinct classes or modules.
Implementing the Single Responsibility Principle
Let’s break down how to apply SRP using an example from Clean Mobile Architecture.
Step 1: Identify Responsibilities
Start by identifying the various responsibilities in your application. For example, let's say you have an app that performs user authentication and data storage. In this case, you can split these responsibilities:
User Authentication: Handles logging in and logging out users.
Data Storage: Manages storing and retrieving user data.
Network Communication: Takes care of sending and receiving network requests.
Step 2: Create Separate Classes
For each identified responsibility, create a dedicated class or module. Here's an example:
// Handles user authentication tasks
class AuthenticationService {
fun login(username: String, password: String): Boolean {
// Code to handle user login
}
fun logout() {
// Code to handle user logout
}
}
// Handles data storage tasks
class UserDataStorage {
fun saveUserData(data: UserData) {
// Code to save user data
}
fun retrieveUserData(): UserData {
// Code to retrieve user data
}
}
// Handles network communication tasks
class NetworkService {
fun sendData(data: Any) {
// Code to send data over the network
}
fun fetchData(url: String): Any {
// Code to fetch data from the network
}
}
In this example, each class has a single responsibility, making it easier to maintain and test.
Step 3: Use Dependency Injection
To make your components even more modular and testable, consider using Dependency Injection (DI) to manage dependencies between them.
class UserRepository(
private val authService: AuthenticationService,
private val userDataStorage: UserDataStorage
) {
fun authenticateAndStoreUser(username: String, password: String) {
if (authService.login(username, password)) {
val userData = // fetch user data from network or other sources
userDataStorage.saveUserData(userData)
}
}
}
In this example, UserRepository
depends on AuthenticationService
and UserDataStorage
, but each class maintains its single responsibility.
Benefits of Single Responsibility Principle
Easier Maintenance
By ensuring that each class has a single responsibility, you make it easier to maintain and modify the code. Changes to one aspect of the application don't ripple through unrelated parts.
Better Testability
With SRP, each class can be tested independently. You can create unit tests for individual classes without worrying about how they interact with other parts of the system.
Improved Readability
Smaller, focused classes are easier to understand. Developers can quickly grasp what each part of the code does, leading to better collaboration and faster onboarding of new developers.
Example in Mobile Architecture
In the Clean Mobile Architecture book by Petros Efthymiou, SRP is a key principle in creating maintainable mobile apps. For example, in an MVVM (Model-View-ViewModel) architecture, SRP can be applied by dividing the code into distinct layers:
Model: Manages the data and business logic.
View: Represents the UI and interacts with the user.
ViewModel: Acts as a middle layer that communicates between the View and Model.
By keeping these components separate, each has its clear responsibility, reducing complexity.
Violations of SRP
Signs of SRP Violation
Fat Classes: If a class has too many responsibilities or methods, it’s a clear sign that SRP is being violated.
Multiple Reasons to Change: If you identify more than one reason for a class to change, it’s doing too much.
Difficult Testing: If testing a class requires setting up unrelated components, it's likely breaking SRP.
Consequences of Violating SRP
Violating SRP can lead to tightly coupled code, where changes in one part can inadvertently affect other areas. It also makes the code harder to read and maintain, leading to longer development cycles and potential bugs.
When to Use the Single Responsibility Principle
Improving Testability: SRP makes unit testing straightforward by isolating each class's functionality.
Reducing Complexity: Use SRP when you notice a class becoming too large or handling unrelated tasks.
Increasing Flexibility: SRP makes it easier to swap components or refactor code without causing cascading changes.
BulletMastering Code Simplicity with Single Responsibility Principle Points
The Single Responsibility Principle ensures that each class or module has only one reason to change.
It’s essential for creating maintainable, testable, and readable code.
SRP can be applied at different levels, from classes to entire modules or layers in an architecture.
Violations of SRP can lead to complex, tightly coupled code that’s difficult to manage.
Conclusion
The Single Responsibility Principle is foundational in software development, especially in mobile architecture. By adhering to SRP, you build components that are easier to manage, test, and extend. This principle aligns with the goals of Clean Mobile Architecture—to create robust and scalable mobile applications with clean, maintainable code.
Subscribe to my newsletter
Read articles from Alyaa Talaat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
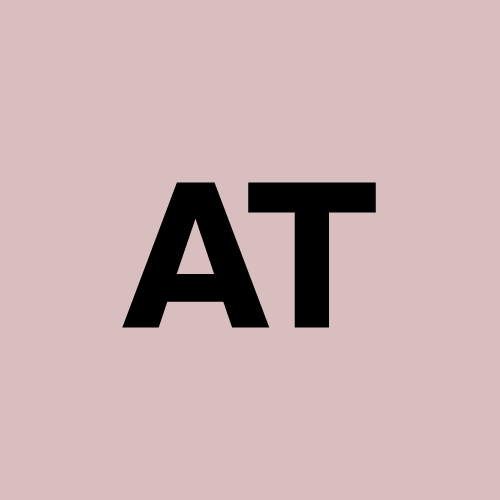
Alyaa Talaat
Alyaa Talaat
As a continuous learner, I’m always exploring new technologies and best practices to enhance my skills in software development. I enjoy tackling complex coding challenges, whether it's optimizing performance, implementing new features, or debugging intricate issues.