Supercharge Your State Management with Redux Toolkit

Table of contents
- What is Redux Toolkit?
- Why You’ll Love Redux Toolkit
- 1. Less Boilerplate, More Productivity
- 2. Built-In Asynchronous Handling with createAsyncThunk
- 3. configureStore for Hassle-Free Store Setup
- 4. Mutability without Mutation (Thanks, Immer)
- 5. Better Code Structure & Readability
- Key Advantages Redux Toolkit Brings
- In Summary
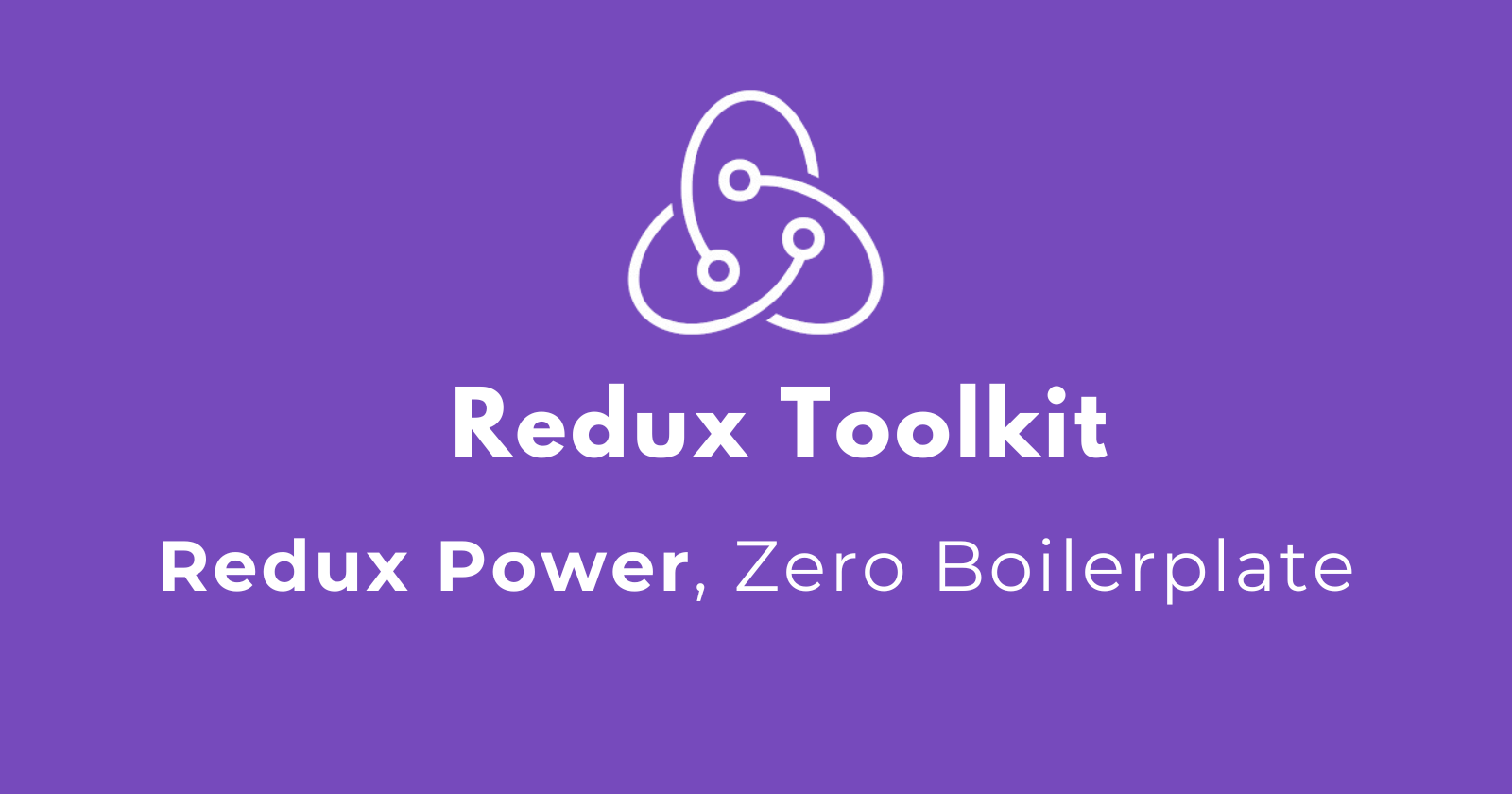
State management is a term every React developer hears sooner or later, and the journey often starts with Redux. But as many have discovered, while Redux is powerful, it also used to be known for its verbosity and complexity. Enter Redux Toolkit (RTK), the official, streamlined approach to using Redux! This tool is so well-designed it’s hard to imagine Redux without it. Let's dive into what Redux Toolkit is and how it brings superpowers to state management in React.
What is Redux Toolkit?
Redux Toolkit is the official way to write Redux logic. Think of it as Redux on a diet — it’s slimmed down, less boilerplate, and focused on making Redux easier and faster to implement. RTK brings new tools and practices to streamline configuration, reduce boilerplate, and improve code readability. It’s packed with intuitive features that make Redux feel, well, friendlier.
Why You’ll Love Redux Toolkit
With Redux Toolkit, you no longer have to write mountains of code just to set up a simple store. It makes Redux’s benefits (like centralized state, predictability, and great debugging) more accessible, especially for those who were put off by the traditional Redux setup. Here’s why Redux Toolkit is such a game-changer:
1. Less Boilerplate, More Productivity
Redux used to require defining action types, action creators, and reducers — separately. All this boilerplate could make even the most straightforward feature feel like a daunting task. Redux Toolkit drastically reduces this by combining these into slices.
With createSlice
, you can define the reducer logic and action creators all in one go. Each slice of state has its own actions and reducers, making it easy to manage and understand.
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: { value: 0 },
reducers: {
increment: (state) => { state.value += 1 },
decrement: (state) => { state.value -= 1 }
}
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
This small code block sets up the entire counter logic, complete with action creators and a reducer. Say goodbye to lengthy action files!
2. Built-In Asynchronous Handling with createAsyncThunk
Async operations used to be a headache in Redux, often requiring complex middleware setups like Thunk or Saga. But RTK includes createAsyncThunk
for handling asynchronous logic right out of the box. This means fetching data, updating state, and handling loading/error states are all easier.
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit';
export const fetchUser = createAsyncThunk('user/fetchUser', async (userId) => {
const response = await fetch(`/api/user/${userId}`);
return response.json();
});
const userSlice = createSlice({
name: 'user',
initialState: { data: null, status: 'idle' },
extraReducers: (builder) => {
builder
.addCase(fetchUser.pending, (state) => { state.status = 'loading' })
.addCase(fetchUser.fulfilled, (state, action) => {
state.data = action.payload; state.status = 'succeeded'
})
.addCase(fetchUser.rejected, (state) => { state.status = 'failed' });
}
});
export default userSlice.reducer;
Now you can handle async actions without having to worry about multiple action types for loading, success, or failure. This feature alone makes async logic in Redux so much simpler.
3. configureStore
for Hassle-Free Store Setup
In classic Redux, configuring the store could feel like setting up a new project in itself, requiring you to import reducers, middleware, and dev tools configuration manually. Redux Toolkit’s configureStore
wraps all that up in a single function call.
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './counterSlice';
import userReducer from './userSlice';
const store = configureStore({
reducer: {
counter: counterReducer,
user: userReducer
}
});
export default store;
Why is this awesome? configureStore
automatically sets up the Redux DevTools extension and includes the popular redux-thunk
middleware by default. You’re instantly ready to start managing state and handling async actions without needing extra setup.
4. Mutability without Mutation (Thanks, Immer)
Redux Toolkit uses Immer under the hood, allowing you to write “mutative” code that’s still safe for Redux. In plain Redux, state updates are strictly immutable, so you’re often writing cumbersome code just to update deeply nested properties. With RTK, you can update state directly:
reducers: {
addToCart: (state, action) => {
state.cart.push(action.payload);
}
}
Underneath, Immer converts this into an immutable update, so you get the ease of direct mutation without sacrificing Redux’s core principles.
5. Better Code Structure & Readability
Redux Toolkit encourages a “feature-first” approach by organizing slices and related logic together. This improves readability and keeps files more organized, especially in large applications. Instead of managing separate folders for actions, reducers, and constants, RTK recommends keeping slices of state (and their reducers, actions, and async thunks) in their own files.
This approach keeps everything related to a specific feature in one place, making it easier to manage as the app grows.
Key Advantages Redux Toolkit Brings
1. Simplicity: Say goodbye to boilerplate and endless configuration. RTK makes Redux simpler and faster to set up.
2. Async Made Easy: With createAsyncThunk
, handling API calls and async logic is straightforward and built-in.
3. Improved Dev Experience: Automatic DevTools setup, easy store configuration, and Immer for seamless immutability — all these features enhance the developer experience significantly.
4. Scalability: RTK’s feature-based organization means it scales beautifully, keeping related logic bundled together for easier maintenance in large applications.
5. Performance: Built-in support for efficient updates and async handling means RTK performs well, even with complex state management requirements.
In Summary
Redux Toolkit modernizes and simplifies Redux, making it a go-to choice for state management in React apps. It’s not just Redux with a facelift; it’s Redux re-imagined for productivity and scalability. With Redux Toolkit, you get to enjoy the benefits of Redux without the hassle of excessive boilerplate or complex configurations. It’s the best of both worlds!
So, if you’re still hesitant about using Redux, give Redux Toolkit a try. It just might change the way you think about state management in React.
Subscribe to my newsletter
Read articles from Madhan Mohan T directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Madhan Mohan T
Madhan Mohan T
Crafting snappy UIs with React & JavaScript | Frontend Dev | Code, coffee, and clean design