What is Express.js, How it works?
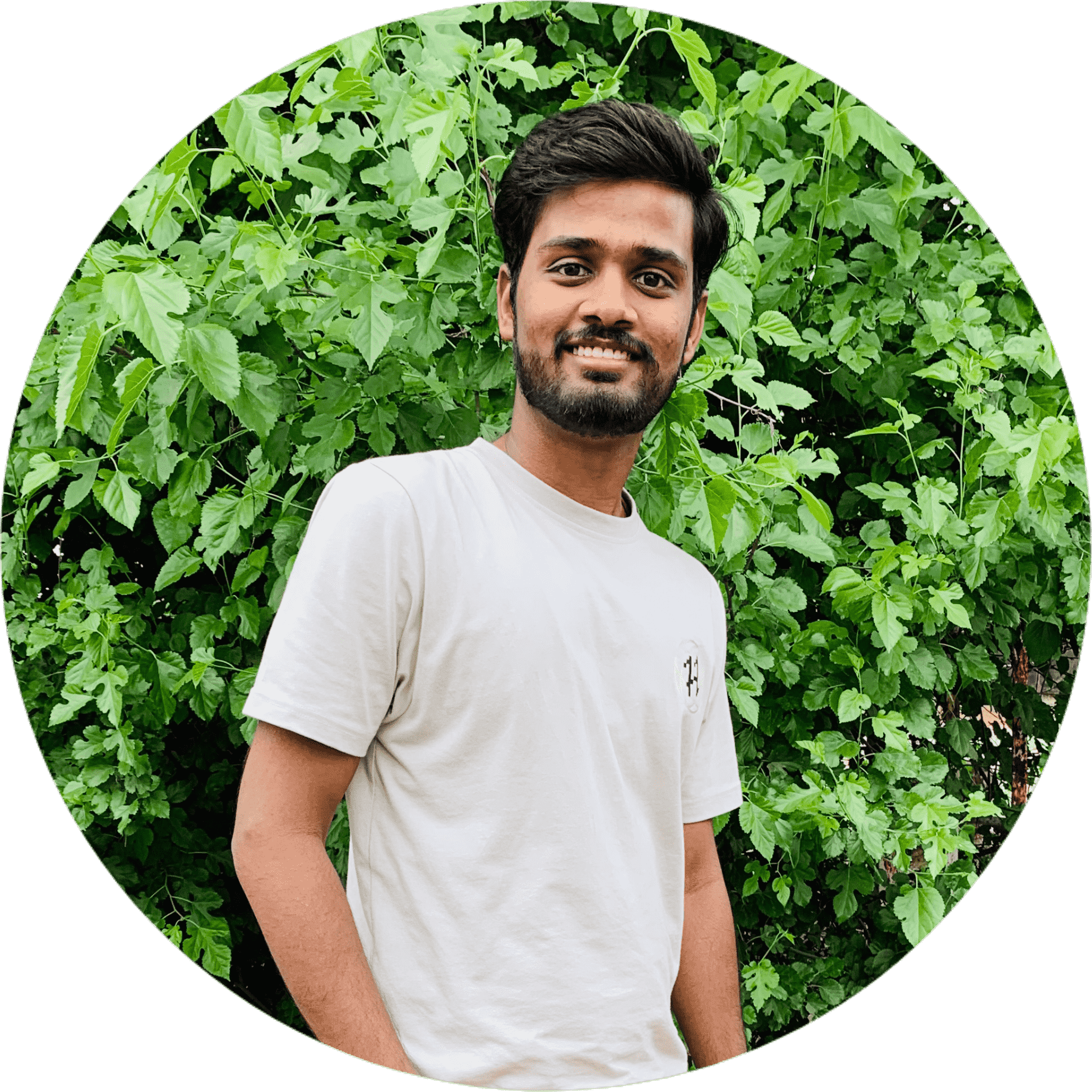
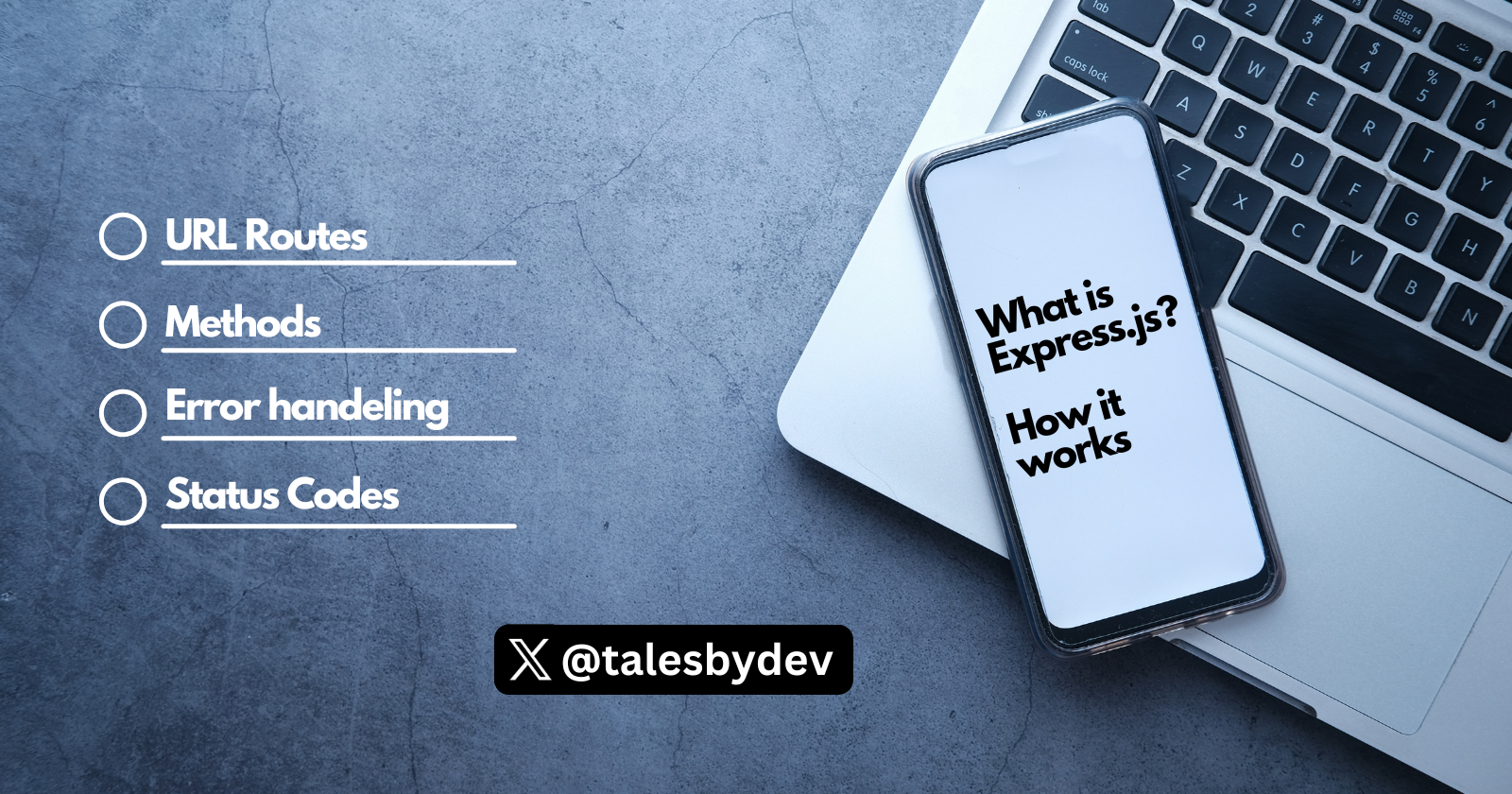
Hey, Everyone! Welcome back to my blog. đź‘‹ Today, we're going to know about Express.js, how it helps to build web applications and API. How to including routing, middleware support, and templating engines.
Introduction to Express.js
Express.js is a popular Node.js web application framework that simplifies the process of building web applications and APIs.
Features of Express.js
Routing:
Express provides a powerful routing system that allows you to define routes for different HTTP methods (GET, POST, PUT, DELETE, etc.) and map them to specific handler functions.
Middleware:
Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application's request-response cycle. They can be used to perform various tasks like:
Parsing request bodies
Handling authentication and authorization
Logging
Serving static files
Templating Engines:
Express supports various templating engines like EJS, Pug, Handlebars, etc., which allow you to generate dynamic HTML content.
Error Handling:
Express has built-in error handling mechanisms that allow you to catch and handle errors gracefully.
Basic example of Express.js
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
This app starts a server and listens on port 3000 for connections. The app responds with “Hello World!” for requests to the root URL (/
) or route. For every other path, it will respond with a 404 Not Found.
URL Routes
In Express.js, routing is the mechanism by which your application responds to different client requests based on the URL and HTTP method. It determines how your application handles incoming requests and directs them to the appropriate code to execute.
const express = require('express')
const app = express()
const port = 3000
// Define a route for the home page (GET request)
app.get('/', (req, res) => {
res.send('Hello from the home page!');
});
// Define a route for handling user data (POST request)
app.post('/users', (req, res) => {
const userData = req.body; // Access data sent in the request body
// Process the user data and send a response
res.send('User data received!');
});
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
Methods in Express.js
In Express.js, methods refer to the functions that handle different HTTP request types for specific routes. These methods correspond to the standard HTTP verbs like GET, POST, PUT, DELETE, and more.
Here's a breakdown of common Express.js methods and their usage:
app.get(path, callback)
: Handles GET requests to the specified path.app.post
(path, callback)
: Handles POST requests to the specified path.app.put(path, callback)
: Handles PUT requests to the specified path.app.delete(path, callback)
: Handles DELETE requests to the specified path.app.all(path, callback)
: Handles all HTTP request methods for the specified path.app.use(middleware)
: Mounts middleware functions to the app. Middleware functions can access the request and response objects, and can perform tasks like logging, authentication, or data manipulation.
Error handeling in Express.js
In Express.js, error handling is achieved using middleware functions with four arguments: (err, req, res, next)
. Here's how it works:
1. Error Handling Middleware:
Create middleware functions specifically designed to handle errors. These functions have four arguments:
(err, req, res, next)
.Place these error handling middleware functions at the end of your middleware stack.
2. Triggering Error Handling:
- When an error occurs in your application, pass it to the
next()
function
app.get('/', (req, res, next) => {
if (someErrorCondition) {
const err = new Error('Something went wrong');
next(err);
}
// ...
});
3. Handling Errors:
Inside the error handling middleware, you can:
Log the error for debugging purposes.
Send an appropriate error response to the client
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Status codes
In Express.js, status codes are three-digit numbers that indicate the outcome of an HTTP request. They provide information about whether the request was successful, encountered an error, or requires further action.
Common Status Codes:
200 (OK): The request was successful.
201 (Created): A new resource was created.
400 (Bad Request): The server cannot or will not process the request due to an apparent client error.
401 (Unauthorized): The client must authenticate itself to get the requested response.
403 (Forbidden): The client does not have access rights to the content.
404 (Not Found): The server cannot find the requested resource.
500 (Internal Server Error): The server encountered an unexpected condition that prevented it from fulfilling the request.
Setting Status Codes in Express:
You can set status codes in your Express.js responses using the res.status()
method
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.status(200).send('Success!');
});
app.get('/not-found', (req, res) => {
res.status(404).send('Not Found');
});
app.listen(3000);
Conclusion
In this blog post, we’ve taken a deep dive into Express.js, a powerful framework that streamlines the process of building web applications and APIs with Node.js. From understanding its core features—like routing, middleware support, and error handling—to setting up a basic server, you now have a solid foundation to start your journey with Express.js.
We explored how routing allows your application to respond to different client requests based on URLs and HTTP methods, making it easy to define clear and efficient pathways for data handling. Middleware functions enhance the framework’s flexibility, enabling you to perform tasks like parsing request bodies, logging, and managing authentication seamlessly.
Additionally, we covered the importance of error handling and how to implement it effectively in your applications. Understanding status codes is crucial for communicating the outcome of requests, and Express.js provides a straightforward way to manage these responses.
As you continue to experiment with Express.js, consider integrating it with various templating engines to generate dynamic content or connecting it to databases for more complex applications. The possibilities are endless!
For Detailed Information check Documentation
Thank you for joining me on this exploration of Express.js! If you have any questions, experiences, or tips to share, please leave a comment below. Happy coding, and I look forward to seeing what you create with Express.js!
Subscribe to my newsletter
Read articles from Nitish singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
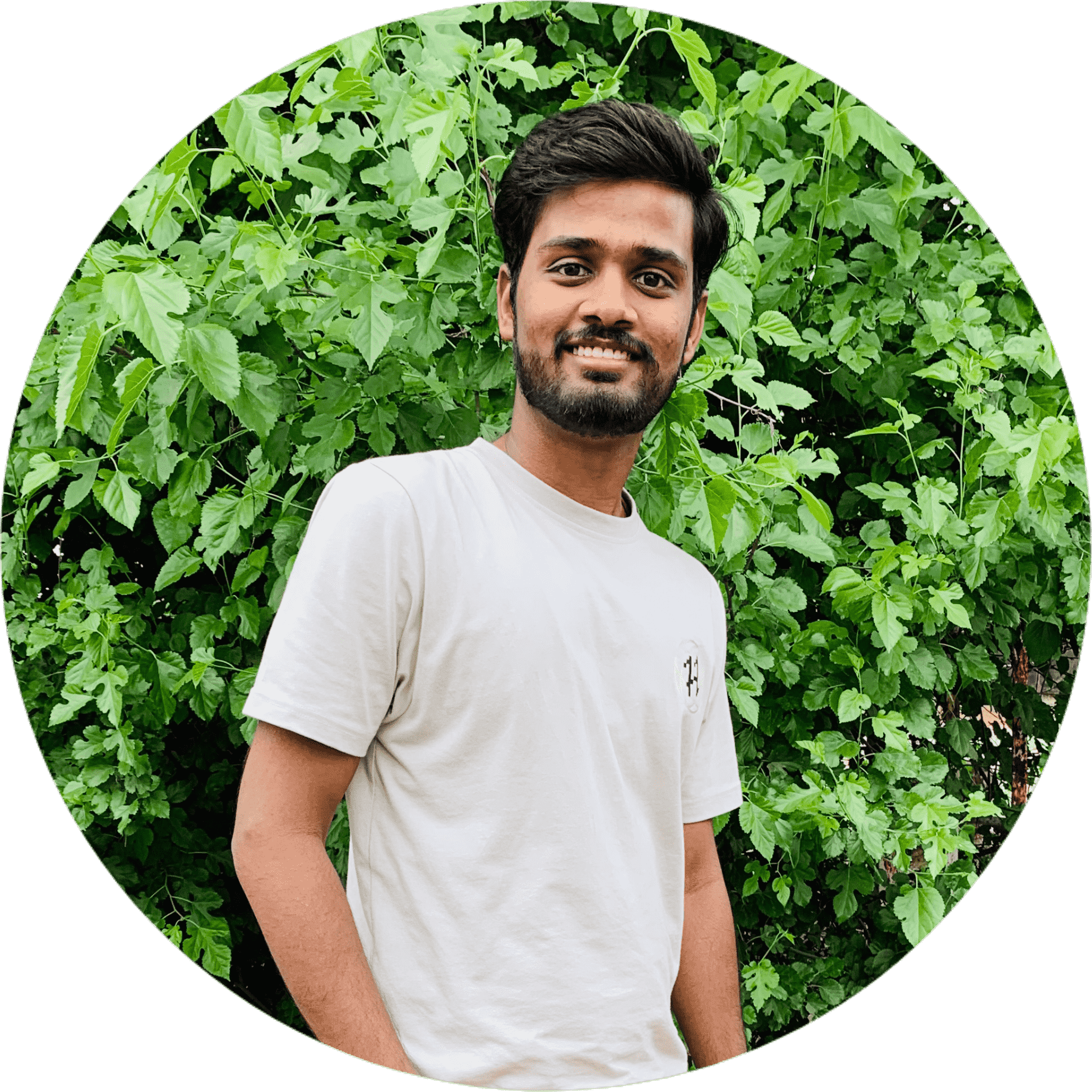
Nitish singh
Nitish singh
A Software Engineer, Frontend Developer looking for opportunities to build myself