Building a RESTful API with Rails
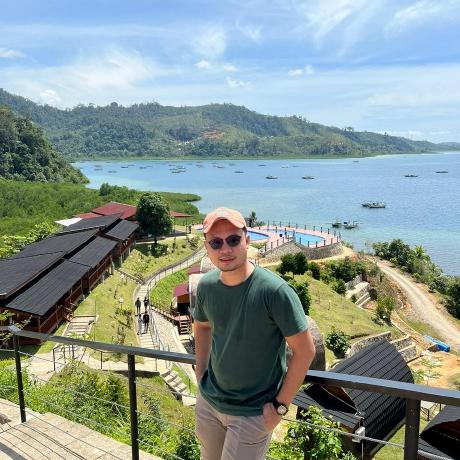
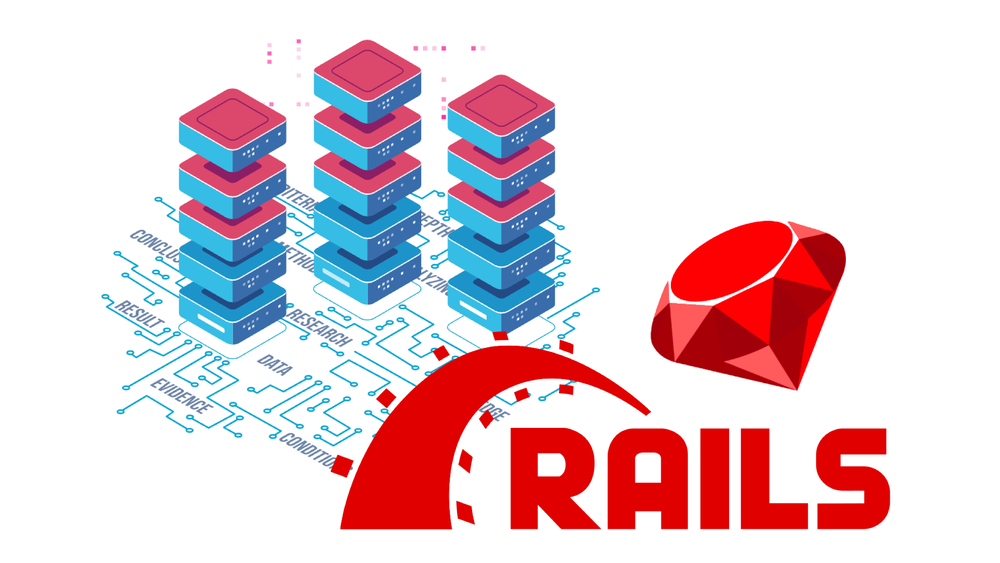
Every time I create a new Rails API with a PostgreSQL database, I find myself googling “create a new Rails API with a PostgreSQL database”. So I’m leaving these notes here for myself, and anyone else who finds this helpful.
For the purpose of this tutorial, let’s imagine we’re creating a super awesome app, and we’re going to call it Super Awesome App.
Installation of Ruby and Rails
The first step to building a RESTful CRUD API with Ruby on Rails is to verify that both Ruby and Rails are installed. (macOS Steps)
Open the terminal and run the following command:
ruby -v
This command verifies the version of Ruby installed. If Ruby is not installed, run the following command:
rvm install 2.7.4 --default
# replace 2.7.4 with the desired Ruby version
To install Rails, run the following command:
gem install bundler
gem install rails
Installation of PostgreSQL
Download the latest version of Postgres from Postgres.app or install using brew:
brew install postgresql
brew services start postgresql
Create a Rails Application
Open the terminal or command prompt, navigate to the desired directory, and run the following command:
rails new redwhite_project --api --minimal --database=postgresql
This command sets the application to be an API with minimal dependencies and sets the database to PostgreSQL.
Generating a Resource
The next step is to create a migration and model for the API. This can be accomplished by using a Rails generator
.
Open the terminal or command prompt and run the following command:
rails g resource Minister first_name last_name genre
# This is also be written as:
# rails g resource Minister first_name:string last_name:string genre:string
This command generates a new model, controller, and migration file for the Minister resource.
Optional: Create Seed Data
Open the seeds file located at db/seeds.rb and input the following:
Minister.create!(first_name: 'xam', last_name: 'Txhomas', genre: 'rock')
Minister.create!(first_name: 'xarah', last_name: 'Jxones', genre: 'pop')
Minister.create!(first_name: 'xoe', last_name: 'Sxmith', genre: 'country')
Minister.create!(first_name: 'xen', last_name: 'Axdams', genre: 'folk')
Perform Migration
Open the terminal or command prompt and run the following command:
rails db:create db:migrate
# If using seed data, run:
# rails db:create db:migrate db:seed
when finding error just do 2 this optional
rake db:create:all
rake db:migrate
Define the Config Routes
Navigate to config/routes.rb and define the routes using one of the following methods:
Rails.application.routes.draw do
# Option 1 - Allow all controller actions
resources : minister
# Option 2 - Allow specific controller actions
resources :minister, only: [:index, :create, :destroy]
# Option 3 - Define specific controller action
get '/minister', to: 'minister#index'
end
Define Controller Actions
Suggested Active Record Rescues
The rescue_from
method is an important feature in Rails that enables centralized error handling, thereby preventing duplication of error handling logic across multiple controller actions. For example, the ActiveRecord::RecordNotFound
exception occurs when a record is not found in the database and is handled by rendering a 404 Record Not Found response to the user. On the other hand, the ActiveRecord::RecordInvalid
exception is raised when a record fails to save to the database due to validation errors and is handled by rendering a 422 Unprocessable Entity response along with the validation errors.
class Minister < ApplicationController
rescue_from ActiveRecord::RecordNotFound, with: :render_not_found_response
rescue_from ActiveRecord::RecordInvalid, with: :render_unprocessable_entity_response
private
def render_not_found_response
render json: { error: "Minister Not Found" }, status: :not_found
end
def render_unprocessable_entity_response(invalid)
render json: { errors: invalid.record.errors.full_messages }, status: :unprocessable_entity
end
end
**Index Action: GET /**minister
This route is used to retrieve a collection of resources. Typically returns a list of all the resources in the database.
def index
render json: Minister.all
end
Show Action: GET /minister/:id
This route is used to retrieve a single resource by its unique identifier or primary key. Typically returns a single resource.
def show
render json: Minister.find(params[:id])
end
**Create Action: POST /**minister
This route is used to create a new resource. Typically returns the newly created resource.
def create
minister = Minister.create!(minister_params)
render json: minister, status: :created
end
private
def minister_params
params.permit(:first_name, :last_name, :genre)
end
Update Action: PATCH or PUT /minister/:id
This route is used to update an existing resource by its unique identifier or primary key. Typically returns the updated resource.
def update
minister = Minister.find(params[:id])
minister.update!(minister_params)
render json: minister
end
Destroy Action: DELETE /minister/:id
This route is used to delete a resource by its unique identifier or primary key. Typically returns a response with a status code of 204 (No Content) to indicate that the resource has been deleted.
def destroy
Minister.find(params[:id]).destroy
head :no_content
end
Closing
Thank-you for reading this guide to building a RESTful CRUD API with Ruby on Rails and PostgreSQL.
References;
https://github.com/rokhimin/ruby-CRUD
https://medium.com/@hanpersonality/basic-crud-operations-of-rdbms-with-postgresql-d613c6c4d00c
https://github.com/nejdetkadir/simple-rails-crud
https://dev.to/justahmed99/crud-rest-api-with-nodejs-expressjs-and-postgresql-57b2
https://medium.com/@ethanryan/creating-a-new-rails-api-with-a-postgresql-database-488ffce649d9
https://github.com/JamesHuangUC/Ruby-on-Rails-CRUD
https://dev.to/nemwelboniface/api-with-rails-7-ngh
https://startup-house.com/blog/creating-a-project-with-ruby-on-rails
https://www.protonshub.com/blogs/power-up-your-development-with-ruby-on-rails
https://github.com/bensheldon/good_job
https://www.protonshub.com/blogs/power-up-your-development-with-ruby-on-rails
https://www.monterail.com/blog/how-to-build-restful-apis-with-ruby-on-rails
Subscribe to my newsletter
Read articles from Redha Bayu Anggara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
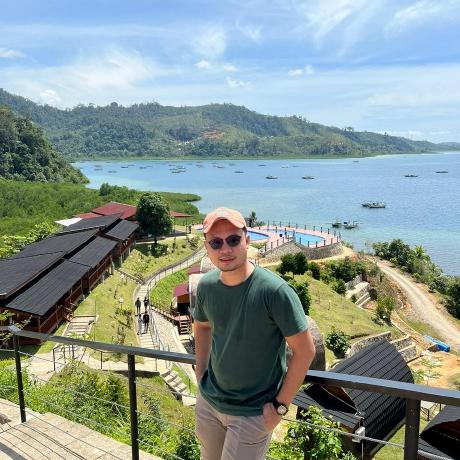
Redha Bayu Anggara
Redha Bayu Anggara
Software Engineer