Understanding the Encoder-Decoder Architecture

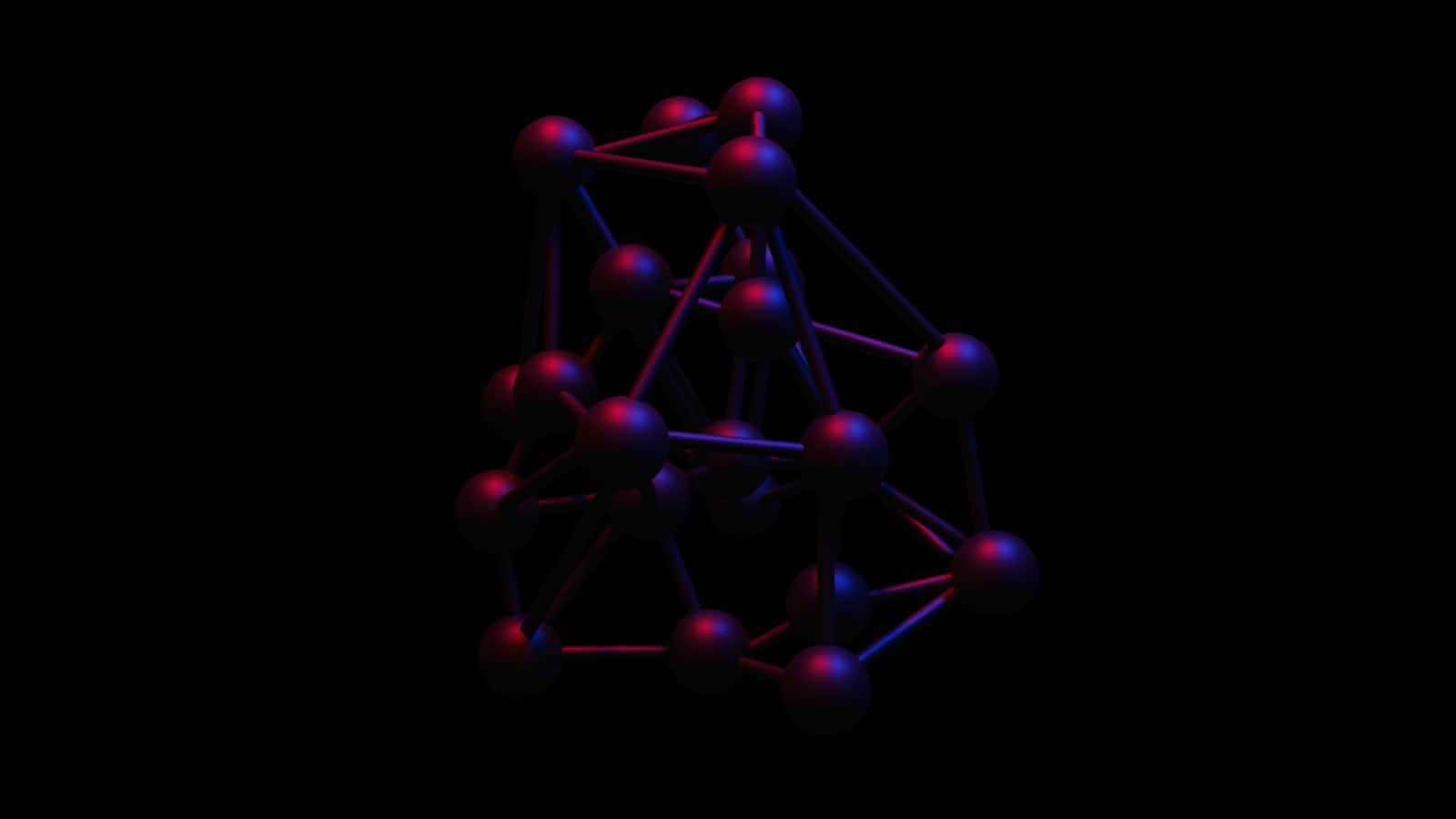
Introduction
The Encoder-Decoder architecture is a foundational neural network design pattern used extensively in sequence-to-sequence (Seq2Seq) tasks. It enables the transformation of an input sequence into an output sequence, which can be of different lengths and modalities. This architecture is pivotal in applications such as machine translation, text summarization, speech recognition, and conversational modeling.
Key Components
1. Encoder
Purpose: Processes the input sequence and encodes it into a fixed-size context vector or a sequence of context vectors.
Functionality:
Captures the semantic and syntactic information of the input data.
Handles variable-length input sequences.
2. Decoder
Purpose: Generates the output sequence using the encoded information from the encoder.
Functionality:
Produces one element at a time.
Utilizes previous outputs and the context vector(s) to inform the next output.
Can incorporate attention mechanisms to focus on relevant parts of the input.
How the Encoder-Decoder Architecture Works
Workflow:
Encoding Phase:
Input: A sequence of elements (e.g., words in a sentence).
Process:
Each element is converted into an embedding vector.
The embeddings are passed through the encoder network (e.g., RNN, LSTM, GRU, Transformer encoder).
The encoder processes the sequence and outputs a context vector or a sequence of vectors.
Output: Encoded representation of the input sequence.
Decoding Phase:
Input: The context vector(s) from the encoder and an initial token (e.g., a start-of-sequence symbol).
Process:
At each timestep, the decoder predicts the next element in the output sequence.
The prediction is based on the context vector(s) and the decoder's previous outputs.
Attention mechanisms can be used to focus on specific parts of the input.
Output: Generated output sequence.
Example: Machine Translation
Let's consider translating the English sentence "I love programming" into French.
Steps:
Encoder:
Input: "I love programming"
Embedding: Each word is converted into a numerical vector.
Processing: The encoder network processes the embeddings and produces context vectors encapsulating the sentence's meaning.
Output: Context vectors representing the input sentence.
Decoder:
Initial Input: Start-of-sequence token
<SOS>
.Processing:
First Timestep:
- Uses the context vectors and
<SOS>
to predict the first word, e.g., "J'".
- Uses the context vectors and
Subsequent Timesteps:
Feeds the previous word into the decoder to predict the next word.
Continues until an end-of-sequence token
<EOS>
is generated.
Output: "J'aime programmer"
Incorporating Attention Mechanism
The basic Encoder-Decoder architecture compresses the entire input sequence into a fixed-size context vector. This can lead to information bottlenecks, especially with long sequences. The attention mechanism addresses this by allowing the decoder to access all encoder outputs and weigh their importance dynamically at each decoding step.
Benefits of Attention:
Enhanced Performance: Improves the model's ability to handle long input sequences.
Dynamic Focus: Allows the model to focus on relevant parts of the input when generating each output element.
Interpretability: Provides insights into which parts of the input the model is attending to during decoding.
Code Example: Encoder-Decoder with Attention in TensorFlow Keras
Below is a simplified implementation of the Encoder-Decoder architecture with attention using TensorFlow Keras.
Setup
import tensorflow as tf
from tensorflow.keras.layers import Input, LSTM, Dense, Embedding, Concatenate
from tensorflow.keras.models import Model
Parameters
# Define parameters
latent_dim = 256 # Dimensionality of the encoding space
embedding_dim = 100 # Dimension of the embedding space
num_encoder_tokens = 10000 # Size of the input vocabulary
num_decoder_tokens = 10000 # Size of the output vocabulary
Encoder
# Encoder
encoder_inputs = Input(shape=(None,), name='encoder_inputs')
encoder_embedding = Embedding(input_dim=num_encoder_tokens, output_dim=embedding_dim, name='encoder_embedding')(encoder_inputs)
encoder_lstm = LSTM(latent_dim, return_sequences=True, return_state=True, name='encoder_lstm')
encoder_outputs, state_h, state_c = encoder_lstm(encoder_embedding)
encoder_states = [state_h, state_c]
Explanation:
encoder_inputs
: Placeholder for input sequences.Embedding
: Converts input tokens to embeddings.LSTM
: Processes embeddings, returns sequences for attention and final states for initialization of the decoder.
Decoder
# Decoder
decoder_inputs = Input(shape=(None,), name='decoder_inputs')
decoder_embedding = Embedding(input_dim=num_decoder_tokens, output_dim=embedding_dim, name='decoder_embedding')(decoder_inputs)
decoder_lstm = LSTM(latent_dim, return_sequences=True, return_state=True, name='decoder_lstm')
decoder_outputs, _, _ = decoder_lstm(decoder_embedding, initial_state=encoder_states)
Explanation:
decoder_inputs
: Placeholder for target sequences.Embedding
: Converts target tokens to embeddings.LSTM
: Processes embeddings, initialized with encoder states.
Attention Mechanism
# Attention Mechanism
from tensorflow.keras.layers import Attention
attention_layer = Attention(name='attention_layer')
attention_outputs = attention_layer([decoder_outputs, encoder_outputs])
# Concatenate attention output and decoder LSTM output
decoder_concat_input = Concatenate(axis=-1, name='concat_layer')([decoder_outputs, attention_outputs])
Explanation:
Attention
: Computes attention scores and applies them to encoder outputs.Concatenate
: Merges decoder outputs with attention context vectors.
Output Layer
# Output layer
decoder_dense = Dense(num_decoder_tokens, activation='softmax', name='output_layer')
decoder_outputs = decoder_dense(decoder_concat_input)
Explanation:
Dense
: Produces output probabilities over the target vocabulary.
Define and Compile the Model
# Define the model
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
# Compile the model
model.compile(optimizer='rmsprop', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# View the model summary
model.summary()
Practical Example: Summarization
Task:
Summarize the sentence:
"The rapid advancement of artificial intelligence and machine learning technologies is transforming the landscape of many industries."
Using the Encoder-Decoder with Attention:
Encoder:
Encodes the input sentence into context vectors.
Captures key information such as "rapid advancement," "artificial intelligence," "transforming industries."
Decoder with Attention:
At each decoding step, attends to relevant parts of the input.
Generates a concise summary by focusing on important phrases.
Generated Summary:
"AI and ML advancements are transforming industries."
Additional Notes
Handling Variable-Length Sequences
Padding: Shorter sequences are padded with special tokens to match the length of the longest sequence in a batch.
Masking: The model can be instructed to ignore padding tokens during training.
Training Considerations
Teacher Forcing: During training, the decoder uses the actual target output from the previous timestep as input, rather than its own previous prediction.
Loss Function: Use
sparse_categorical_crossentropy
when target data is integer-encoded, andcategorical_crossentropy
when one-hot encoded.
Quick Revision Notes
Encoder-Decoder Architecture:
Encoder: Encodes input sequences into context vectors.
Decoder: Generates output sequences using the context vectors.
Attention Mechanism:
Allows the decoder to focus on specific parts of the input sequence.
Improves model performance on longer sequences.
Applications:
Machine Translation
Text Summarization
Speech Recognition
Conversational Agents
Key Advantages:
Handles variable-length input and output sequences.
Provides a flexible framework for many Seq2Seq tasks.
Enhances interpretability through attention weights.
Conclusion
The Encoder-Decoder architecture, especially when combined with attention mechanisms, is a powerful tool in modern deep learning. It allows models to effectively process and generate sequences, making it indispensable in tasks that require understanding and producing human language or other sequential data.
Subscribe to my newsletter
Read articles from Sai Prasanna Maharana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
