Understanding Higher-Order Functions in JavaScript
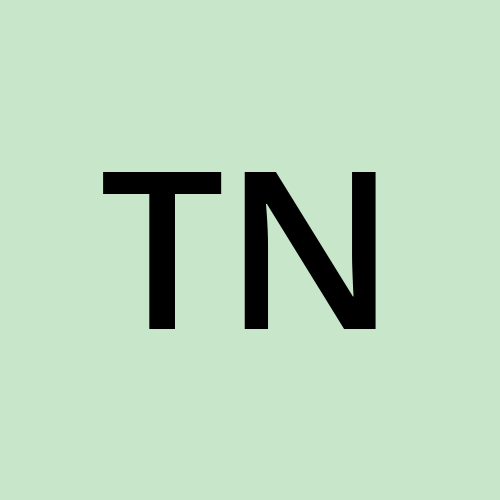
This blog is inspired by a conversation I had with ChatGPT while grappling with the concept of asyncHandler
from the backend series by Hitesh Choudhary. As I faced challenges in understanding this important aspect of error handling in asynchronous programming, I documented our discussions to clarify my thoughts and insights.
What are Higher-Order Functions?
A higher-order function is a function that either takes one or more functions as arguments, returns a function, or both. This capability allows for more abstract and powerful programming constructs. Higher-order functions can help to create reusable, clean, and manageable code.
Example 1: map
The map
function is a classic example of a higher-order function. It takes an array and a function as arguments, applies that function to each element of the array, and returns a new array with the results.
const numbers = [1, 2, 3, 4];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8]
In this example, map
takes an anonymous function num => num * 2
, which is executed for each element in the numbers
array, resulting in a new array of doubled values.
Example 2: setTimeout
The setTimeout
function is another example of a higher-order function. It takes a callback function and a delay (in milliseconds) as arguments, executing the callback after the specified delay.
console.log("Start");
setTimeout(() => {
console.log("Executed after 2 seconds");
}, 2000);
console.log("End");
In this example, the message “Executed after 2 seconds” will be logged after a delay of 2 seconds, while “Start” and “End” are logged immediately.
The Challenge of Understanding asyncHandler
While learning about higher-order functions, I encountered challenges in understanding the asyncHandler
function used in Express.js applications. The asyncHandler
is designed to simplify error handling for asynchronous route handlers. Here’s a breakdown of how it works:
The asyncHandler
Function
The skeleton of the asyncHandler
function looks like this:
const asyncHandler = (requestHandler) => {
return (req, res, next) => {
// Logic goes here
};
};
*********************
const asyncHandler = (requestHandler) => {
return (req, res, next) => {
Promise.resolve(requestHandler(req, res, next)).catch((err) => next(err));
};
};
Breaking Down the Syntax
Higher-Order Function:
asyncHandler
itself is a higher-order function. It takes another function, referred to asrequestHandler
, as its argument.This means that
asyncHandler
expects you to pass in a function that handles an incoming request (like an Express route handler).
Returning a New Function:
The function returns a new function, which is the actual middleware function that Express will use.
This returned function has access to three parameters:
req
,res
, andnext
, which are standard in Express middleware.
Parameters:
req
: The request object representing the incoming HTTP request.res
: The response object used to send a response back to the client.next
: A callback function that, when called, passes control to the next middleware in the stack.
Handling the Request:
Inside the returned function,
Promise.resolve(requestHandler(req, res, next))
is used. This line does a couple of things:It calls the
requestHandler
function, passing it thereq
,res
, andnext
parameters.The
requestHandler
can be an asynchronous function, returning a promise.Promise.resolve()
ensures that even ifrequestHandler
is synchronous and does not return a promise, it will still be treated as a promise.
Error Handling:
The
.catch((err) => next(err))
part is crucial:If any error occurs within the
requestHandler
(whether it's synchronous or asynchronous), it will be caught by this catch block.The error is then passed to the next middleware in the stack by calling
next(err)
, allowing centralized error handling in Express.
Example Usage
Here's how you would typically use asyncHandler
in an Express route:
app.get('/user/:id', asyncHandler(async (req, res, next) => {
const userId = req.params.id;
const user = await User.findById(userId);
if (!user) {
return res.status(404).json({ success: false, message: 'User not found' });
}
res.json({ success: true, user });
}));
Flow of Execution
Define a Route: When you define a route in your Express application, you specify what should happen when a request hits that route. Typically, this involves processing the request, possibly fetching data, and sending a response.
Wrap the Route Handler: By wrapping your route handler with the
asyncHandler
, you're ensuring that any errors that occur during the execution of that handler will be caught and passed to the next error-handling middleware in your Express application.Parameters (
req
,res
,next
): The function thatasyncHandler
returns will have the standard Express middleware parameters:
Executing Code With and Without asyncHandler
Without asyncHandler
In traditional route handling without asyncHandler
, you would have to manage errors using try-catch blocks. Here's an example:
app.get('/user/:id', async (req, res, next) => {
try {
const userId = req.params.id;
const user = await User.findById(userId);
if (!user) {
return res.status(404).json({ success: false, message: 'User not found' });
}
res.json({ success: true, user });
} catch (error) {
next(error); // Pass the error to the next middleware
}
});
With asyncHandler
Using asyncHandler
, the code becomes cleaner and more readable:
app.get('/user/:id', asyncHandler(async (req, res, next) => {
const userId = req.params.id;
const user = await User.findById(userId);
if (!user) {
return res.status(404).json({ success: false, message: 'User not found' });
}
res.json({ success: true, user });
}));
Conclusion
Higher-order functions are a fundamental concept in JavaScript that can significantly enhance your coding capabilities. Functions like map
and setTimeout
exemplify the power of higher-order functions in creating concise and effective code.
Understanding how to work with higher-order functions, such as asyncHandler
, can take some practice, but mastering them can lead to more maintainable and readable code in your applications. Don't hesitate to break down complex functions into simpler parts to grasp their functionality fully.
Happy coding!
Subscribe to my newsletter
Read articles from Tanay Nagde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
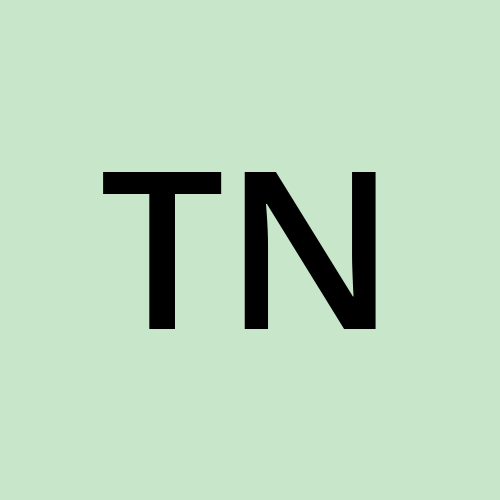