Makefiles Explained: Boosting Efficiency in Software Development
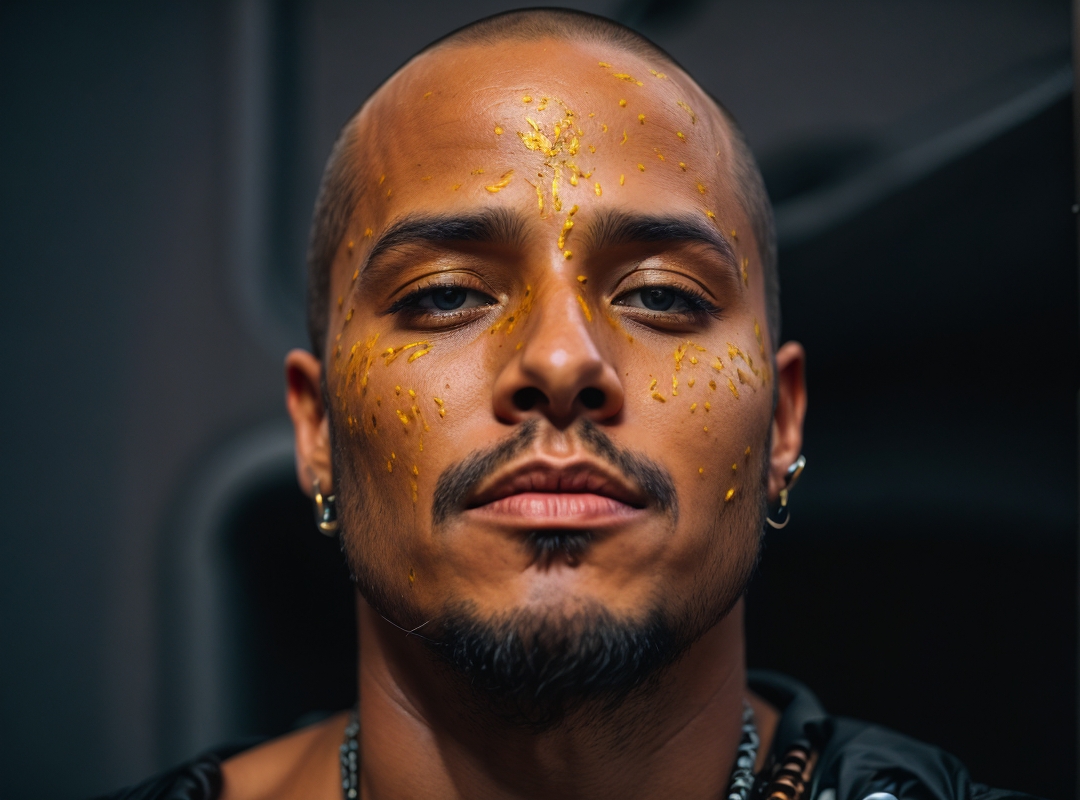
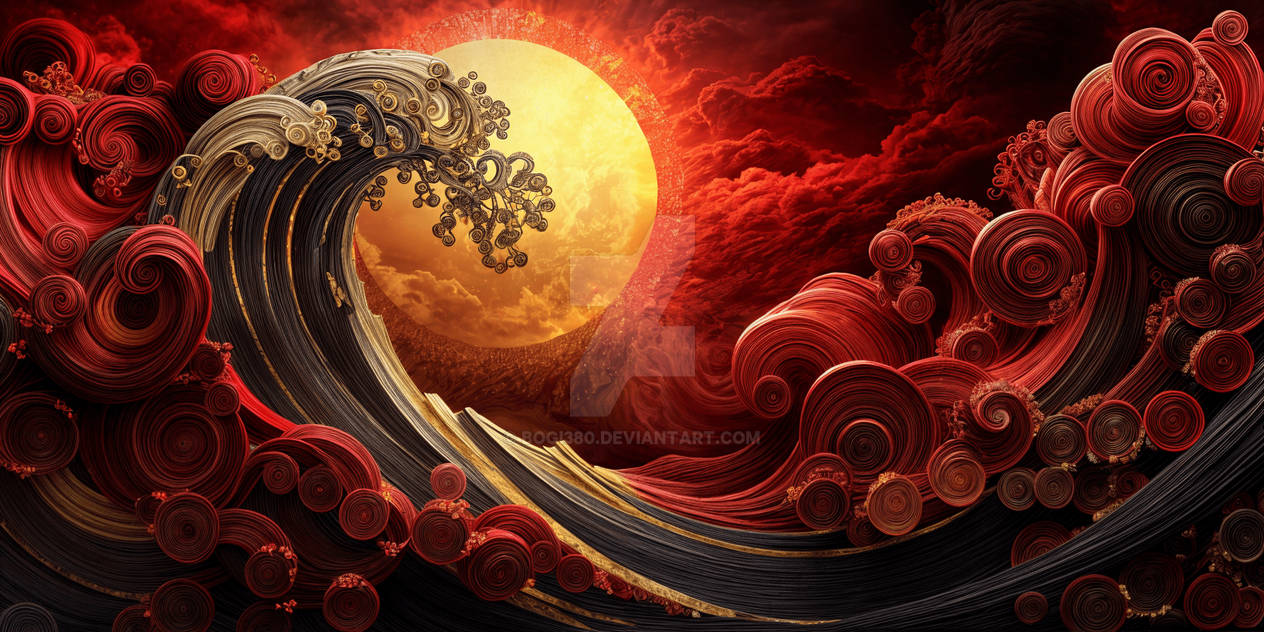
In the world of software development, efficiency and organization are key to delivering robust applications. One of the most powerful tools at a developer's disposal is the Makefile. A Makefile is more than just a text file—it's a systematic approach to automating and managing the build process of a project. In this blog post, we'll explore the intricacies of Makefiles, how they operate, and why they're essential for developers working in compiled languages like C and C++.
What is a Makefile?
At its core, a Makefile is a special type of file used to define a set of instructions for the make
utility. It streamlines the compilation and building process by outlining rules that dictate how the various source code files should be processed to create final executable binaries or libraries.
Key Components of a Makefile
A Makefile consists of several key components, including:
Targets: The name representing the final product, such as an executable or a library. It can also refer to actions, like
clean
, which removes temporary files.Dependencies: These are the files that are necessary to build the target. If a source file changes, its corresponding target will be rebuilt during the next
make
invocation.Rules: Each rule defines how to convert dependencies into a target. It typically includes the target name, its dependencies, and a series of commands needed to build it.
Variables: Variables allow the reuse of values throughout the Makefile, making it easier to modify configurations such as compiler commands or flags without changing them in multiple places.
Comments: Comments in Makefiles start with the
#
symbol, allowing developers to annotate their code without affecting functionality.
How Does the make
Utility Work?
The make
utility reads the Makefile and processes the rules based on file dependencies. When you run a command like make all
or make clean
, the utility checks which source files have changed and determines the minimum work required to bring the target files up to date. This intelligent tracking of dependencies helps save time and avoids unnecessary recompilation.
When Should You Use a Makefile?
Makefiles are particularly useful in several scenarios:
Large Projects: In larger codebases with multiple source files, a Makefile organizes the build process, saving time that would otherwise be spent manually compiling each file.
Complex Dependencies: When your project has interdependent modules or files, a Makefile makes it easier to manage these relationships, ensuring that files are built in the correct order.
Automated Build Processes: For projects that require automated builds, Makefiles standardize the procedures, reducing human error and speeding up the development cycle.
Building Blocks of a Makefile
Understanding Rules
Rules are the foundation of a Makefile. A typical rule will look like this:
target: dependencies
commands to build target
The rule specifies how to construct the target and when to consider it out of date. For example, if a source file changes, any target that relies on that source file will be marked for recompilation.
Explicit vs. Implicit Rules
When defining rules, developers can use explicit rules that lay out every detail or implicit rules that allow make
to infer how to accomplish simple tasks based on filename patterns.
Explicit Rules: These rules are explicitly defined, detailing exactly how a target is built, such as:
prog: main.c helper.c gcc -Wall -o prog main.c helper.c
Implicit Rules: These are predefined by the
make
system, which allows a concise Makefile by utilizing patterns. For instance:%.o: %.c gcc -c $< -o $@
In this case, the %
acts as a wildcard, matching any file name with a .c
extension and compiling it into a corresponding .o
object file.
Variables: Harnessing Flexibility
Variables in Makefiles simplify the configuration process. The syntax for defining a variable is straightforward:
VARIABLE_NAME = value
For instance:
CC = gcc
CFLAGS = -Wall
When utilized, variables are referenced with the $
symbol, as follows:
prog: main.c helper.c
$(CC) $(CFLAGS) -o prog main.c helper.c
This approach not only enhances clarity but also facilitates updates; if the compiler or flags need to change, only one line needs to be adjusted.
Default Variables
Makefiles also include default variables that ensure compatibility across environments. Common default variables include:
CC
: Designates the compiler (typicallygcc
).CFLAGS
: Compiler flags for warnings and optimization.LDLIBS
: Libraries to be linked.RM
: The command to remove files (typicallyrm -f
).
Effective Use of the All Rule
A well-structured Makefile should ideally have an "all" rule that compiles all necessary components of your project. This is especially important in projects with multiple source files, as it allows for efficient incremental builds. Here’s how to construct an "all" target that recompiles only the updated source files:
all: $(OBJS)
$(OBJS): %.o: %.c
$(CC) $(CFLAGS) -o $@ $<
In the above example, the all
target depends on the object files, which get created from their corresponding source files. The $(OBJS)
variable can be set to a list of all your object files, allowing make
to intelligently rebuild only those files that have changed since the last compilation.
Pattern Substitution in Makefiles
Pattern substitution can further streamline your Makefile, especially when you have to deal with files that share common attributes or extensions. This allows for dynamic referencing and management of file names.
Here are a few examples of how pattern substitution can be applied:
Changing Extensions:
FILES = file1.c file2.c file3.c OBJ = $(FILES:.c=.o)
In this case, the
.c
extension of each file is replaced with.o
, generating a list of object filenames.Adding Prefixes:
FILES = main.c helper.c OBJS = $(FILES:%=obj/%)
Here, the
obj/
prefix is added to each filename inFILES
, creating a variableOBJS
that refers to the object files.Removing Prefixes:
ALL_FILES = src/main.c src/utils.c BASE_FILES = $(ALL_FILES:src/%=%)
This removes the
src/
prefix from each file inALL_FILES
, simplifying the filenames without losing context.
Leveraging Makefile Comments
While Makefiles can appear dense due to their syntax, comments serve as a valuable tool for documentation. Comments help developers, especially those new to a project, understand the purpose behind various rules and variables. Use comments generously to explain complex commands or the intent behind certain rules:
# Compiler and flags
CC = gcc
CFLAGS = -Wall
# Main target
prog: main.c helper.c
$(CC) $(CFLAGS) -o prog main.c helper.c
Advantages of Using a Makefile
The application of Makefiles offers several distinct advantages in software development:
Efficiency: Makefiles reduce the unnecessary recompilation of files, thereby saving time during the build process. Changes to a few files will only trigger recompilation of those files, rather than the entire codebase.
Clarity and Maintenance: The structured format of Makefiles makes it easier for developers to understand the build process and maintain the project. It's a clear declaration of what depends on what.
Cross-Platform Compatibility: Makefiles can be configured to handle differing environments and compilation requirements. This can lead to a greatly reduced setup time for new developers joining the project.
Automated Builds: With a Makefile, builds can be easily automated in scripts or continuous integration systems. This leads to smoother development cycles and faster testing.
Final Thoughts
In conclusion, mastering Makefiles is an essential skill for software developers, particularly those working with C, C++, or similar languages. A well-designed Makefile acts as both a roadmap and a toolkit, enabling developers to navigate complex build processes efficiently.
As you create your own Makefiles, remember the importance of organization, clarity, and efficiency. Experiment with both explicit and implicit rules, make robust use of variables, and understand when to leverage pattern substitution to keep your Makefile concise and manageable.
Investing the time to become proficient with Makefiles will streamline your development process and empower you to deliver high-quality software faster and with less hassle. So, roll up your sleeves, dive into the world of Makefiles, and experience the benefits that come with automated build processes!
Resources for Further Exploration
To deepen your understanding of Makefiles, consider some of the following resources:
GNU Make Manual: The authoritative source for understanding all features of
make
.Makefile Tutorial: Online tutorials that guide you through advanced Makefile features.
Embrace Makefiles, and you’ll find that they simplify your projects and workflows in significant ways. Happy coding!
Subscribe to my newsletter
Read articles from Younis Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
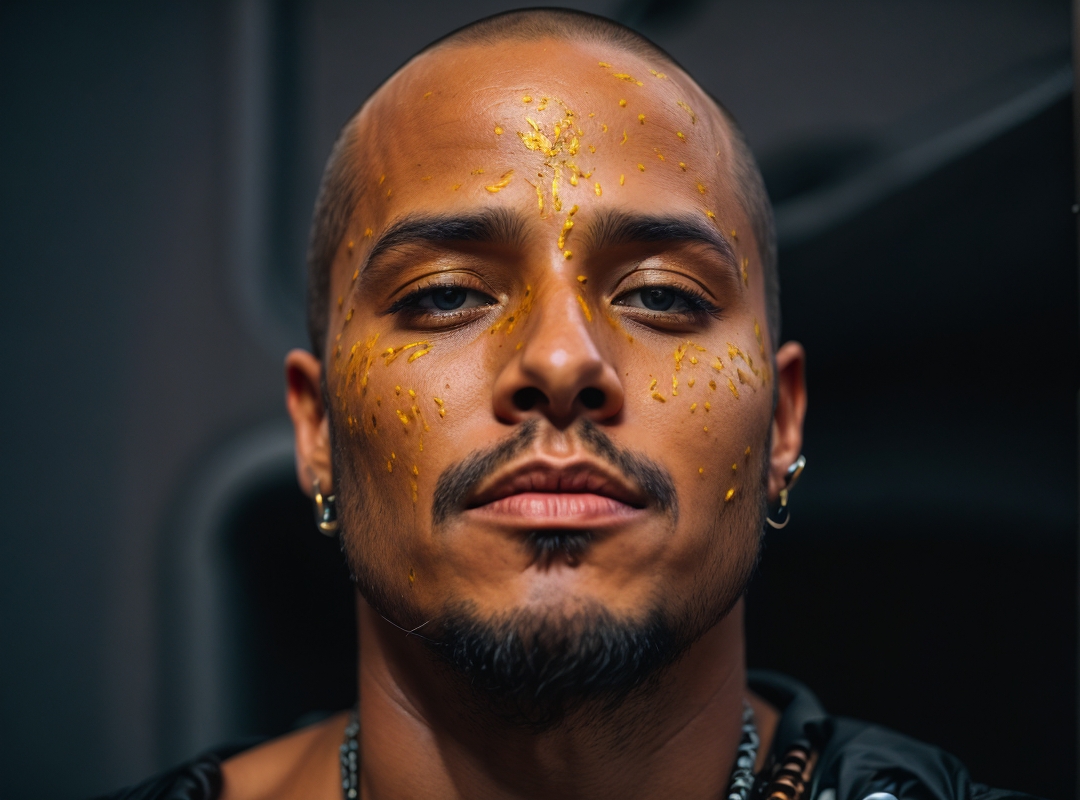
Younis Ahmed
Younis Ahmed
Hey there! 👋 Developer by day, Graphic Designer by night, and Software Engineer Student in between. 🌟 Join me on this journey as I explore the world of coding, design, and everything tech-related. 🖥️✨ #DeveloperLife #GraphicDesignPassion #TechJourney