Day 8: Understanding JavaScript Events – Enhancing Interactivity
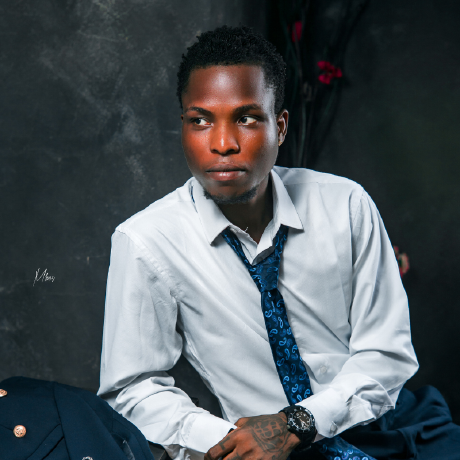
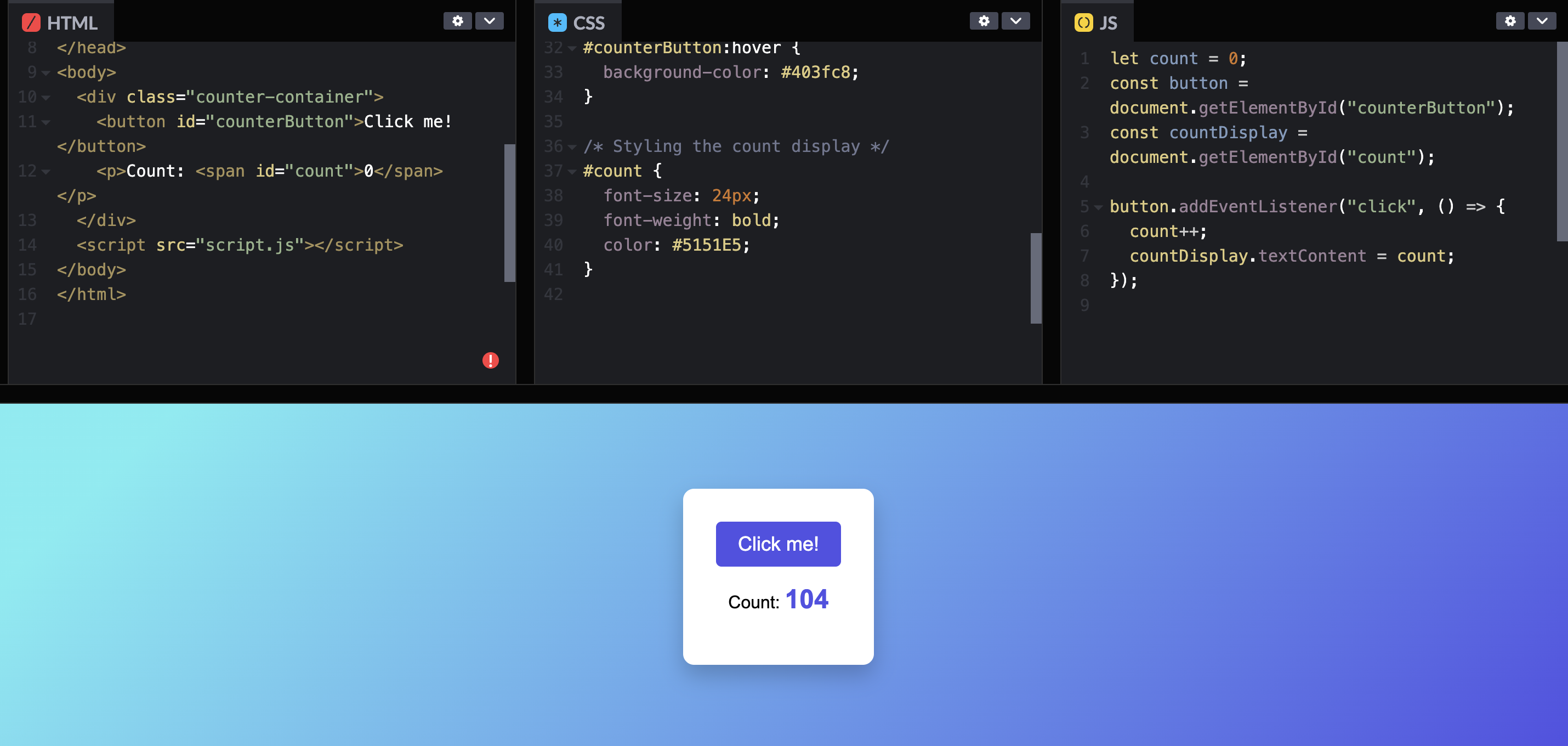
JavaScript events are at the heart of making web applications interactive, allowing us to respond to user actions like clicks, key presses, mouse movements, and more. In this post, we’ll cover what events are, why they’re essential, different types of events, and how to use them effectively.
What Are Events in JavaScript?
Events are actions or occurrences, such as a mouse click or keyboard press, that can be detected by JavaScript, triggering a response. They allow us to create dynamic, interactive user experiences by letting our code "listen" to user actions and react accordingly.
Why Are Events Important?
Events enable us to add interactivity to our websites, essential for building responsive applications. Developers can enhance the user experience by mastering events with real-time feedback and interactions.
Key Event Types in JavaScript
There are various events, each with its unique role and use. Here are some fundamental event types you’ll encounter:
Mouse Events
click: Triggered when an element is clicked.
dblclick: Triggered on double-clicking an element.
mouseover: Activated when the mouse hovers over an element.
mouseout: Triggered when the mouse leaves an element.
mousemove: Tracks the mouse's movement over an element.
const button = document.querySelector("button");
button.addEventListener("click", () => {
alert("Button clicked!");
});
Keyboard Events
keydown: Fired when a key is pressed down.
keyup: Fired when a key is released.
keypress: Triggered when a key is pressed and held down (note: deprecated but may still be used in some contexts).
document.addEventListener("keydown", (event) => { if (event.key === "Enter") { console.log("Enter key pressed!"); } });
Form Events
submit: Triggered when a form is submitted.
change: Activated when an input value changes.
focus and blur: Occur when an element gains or loses focus, respectively.
const form = document.querySelector("form"); form.addEventListener("submit", (event) => { event.preventDefault(); console.log("Form submitted!"); });
Window Events
load: Fired when the entire page loads.
resize: Triggered when the window is resized.
scroll: Fired during scrolling.
window.addEventListener("load", () => {
console.log("Page fully loaded");
});
How to Add Event Listeners in JavaScript
Event listeners are functions that wait for a specified event to occur and then execute a block of code. You can use the addEventListener
method to attach event listeners to HTML elements.
const button = document.querySelector("button");
button.addEventListener("click", () => {
alert("Button clicked!");
});
Removing Event Listeners
Sometimes, you may want to remove an event listener after it’s no longer needed. This can improve performance and prevent unnecessary code execution.
function handleClick() {
alert("Button clicked!");
}
button.addEventListener("click", handleClick);
button.removeEventListener("click", handleClick);
Preventing Default Behavior
Certain elements, like forms, have default actions associated with them (e.g., submitting a form or navigating to another page). To prevent these actions, use event.preventDefault()
.
document.querySelector("form").addEventListener("submit", (event) => {
event.preventDefault();
alert("Form submission prevented!");
});
Event Delegation
Event delegation is a powerful technique that allows you to add a single event listener to a parent element rather than multiple listeners to individual child elements. This approach enhances performance, especially with dynamically created elements.
document.querySelector("ul").addEventListener("click", (event) => {
if (event.target.tagName === "LI") {
alert(`Item clicked: ${event.target.textContent}`);
}
});
Interactive Example: Button Counter
Try out this simple counter to see event listeners in action! Click the button to increase the count.
Wrapping Up
Events are essential for creating dynamic and interactive web experiences. By understanding different types of events and how to handle them, you can significantly improve the user experience of your applications.
Additional Resources
Handling Events in JavaScript
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
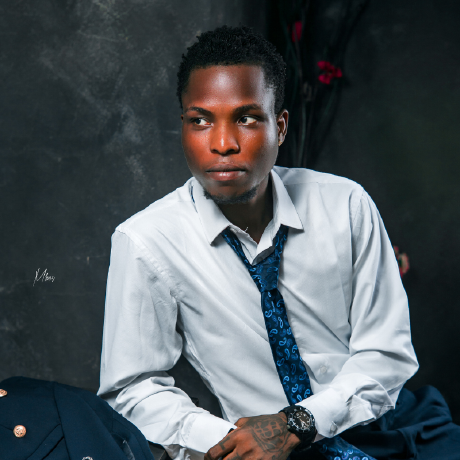
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com