Understanding SQL Server Isolation Levels
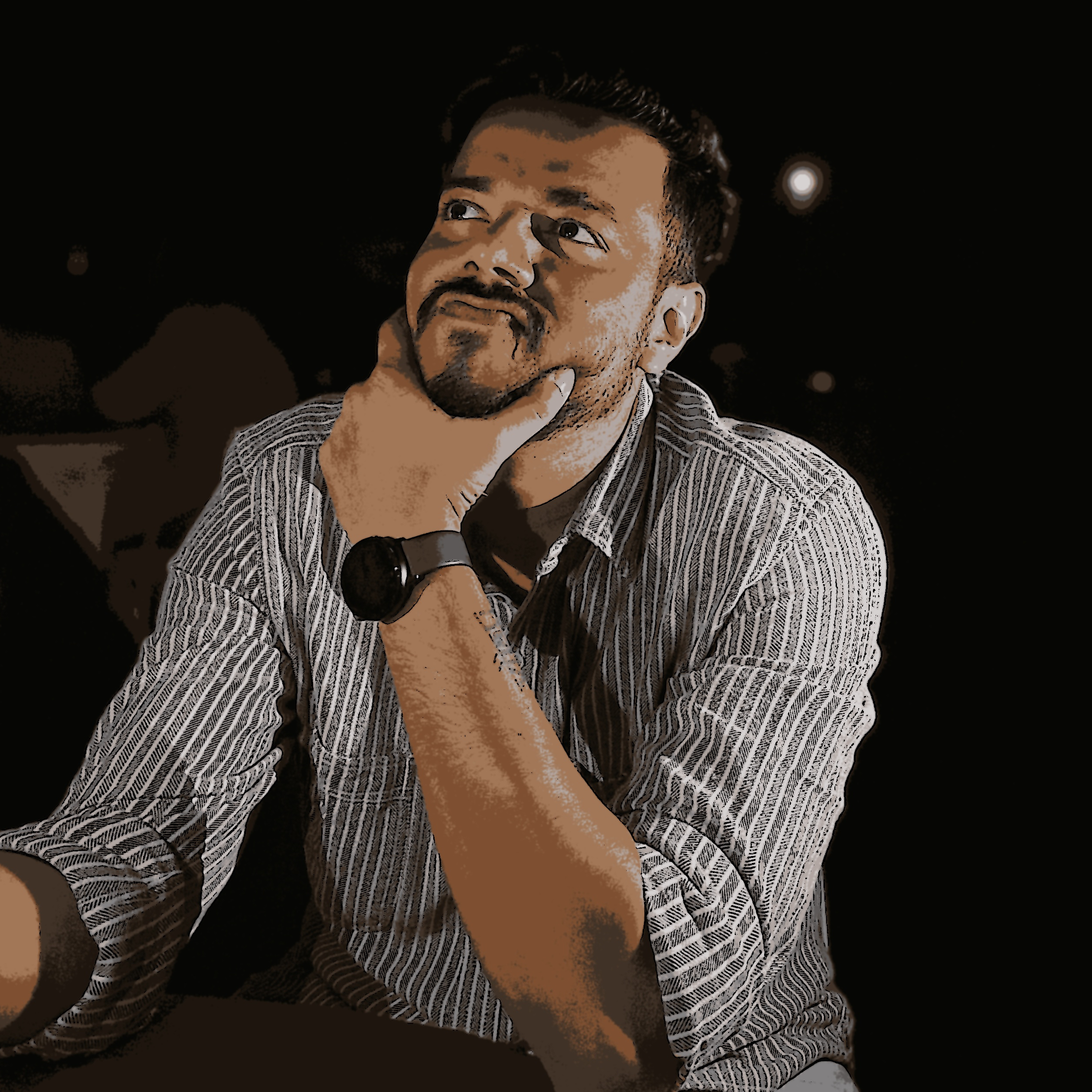
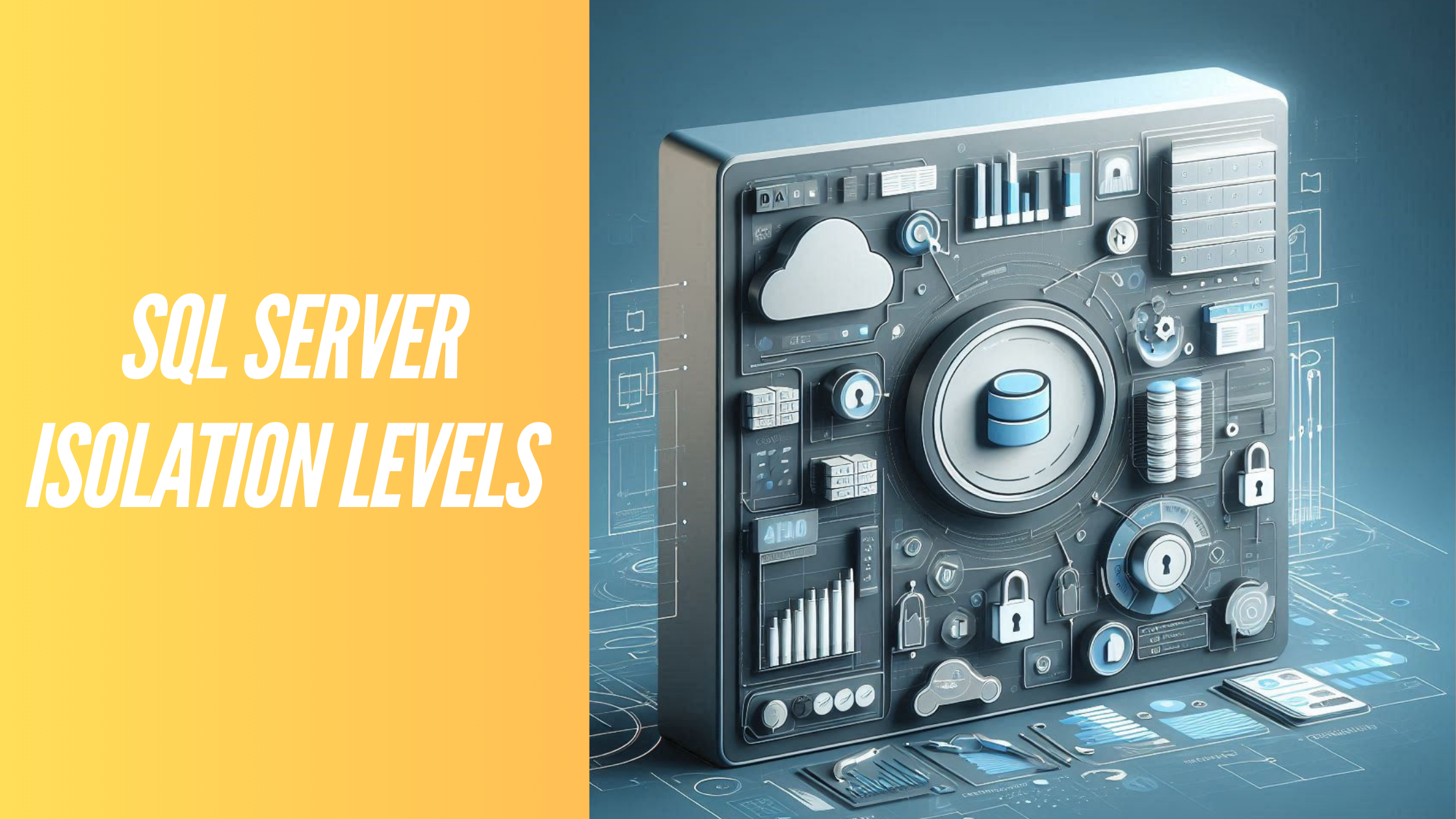
Database transactions are essential for keeping data accurate in systems where many processes run at the same time. SQL Server offers several isolation levels to balance consistency, concurrency, and performance. In this comprehensive guide, we’ll explore each isolation level, its behaviours, use cases, trade-offs, and how to choose the right one.
Introduction
Transaction isolation levels are a key part of the ACID properties (Atomicity, Consistency, Isolation, Durability) that ensure database reliability. Isolation determines how transactions interact with each other when they read or change data at the same time. SQL Server offers the following isolation levels:
READ UNCOMMITTED
READ COMMITTED (default)
REPEATABLE READ
SNAPSHOT
SERIALIZABLE
These levels manage the occurrence of dirty reads, non-repeatable reads, and phantom reads, which are common issues in concurrent transactions. Let’s explore each level, its behaviour, and use cases, with practical examples.
A phantom read occurs when a transaction reads a set of rows that satisfy a specific search condition and then later when the same transaction repeats the same read with the same conditions, it finds a different set of rows. This happens because other transactions are allowed to insert new rows or delete existing rows that match the search criteria of the first transaction's queries.
READ UNCOMMITTED
This level essentially removes all isolation guarantees and lets transactions read data that hasn't been committed yet. Think of it like reading a document while someone else is still typing it – you might see their half-finished work before they're done or even if they eventually discard their changes.
While this provides the fastest performance since there's no overhead of locking, it can lead to serious data inconsistencies and is rarely used in practice except for specific reporting scenarios where approximate results are acceptable.
-- Transaction 1
BEGIN TRANSACTION
UPDATE Products SET Price = Price + 10 WHERE ProductId = 1;
-- Not committed yet
-- Transaction 2 (different session)
SET TRANSACTION ISOLATION LEVEL READ UNCOMMITTED;
SELECT Price FROM Products WHERE ProductId = 1;
-- Reads the modified (uncommitted) price
Transaction 1's UPDATE places an exclusive lock on the row
Transaction 2's SELECT doesn't request any locks (no shared locks)
Transaction 2 reads the data immediately despite exclusive lock
Transaction 2 sees uncommitted changes (dirty reads)
If Transaction 1 rolls back, Transaction 2 has read invalid data
Multiple SELECTs in Transaction 2 might see different data as other transactions modify it
Key Characteristics
Allows dirty reads (uncommitted data)
No shared locks are acquired, meaning minimal blocking
Provides the highest performance among all isolation levels
Use Cases
Monitoring queries or approximate aggregates where precision isn’t critical
Reporting queries that can tolerate temporary inconsistencies
Long-running processes like ETL jobs, where consistency is secondary
Drawbacks
Reads may reflect uncommitted changes that are later rolled back
Risk of incorrect business decisions based on temporary data
Not suitable for financial transactions or critical operations
This behavior is similar to using the WITH (NOLOCK)
hint. Be cautious when using it, as it can miss rows during index rebuilds or page splits.
READ COMMITTED (Default)
This isolation level prevents dirty reads while maintaining a balance between consistency and performance. Provides a basic guarantee that you'll only read data that has been properly committed by other transactions.
It's like waiting for someone to finish writing their paragraph before you read it. However, it only holds locks briefly while reading the data, which means if you read the same data twice in the same transaction, you might get different results if another transaction modified it in between.
-- Transaction 1
BEGIN TRANSACTION
UPDATE Accounts SET Balance = Balance - 100 WHERE AccountId = 1;
WAITFOR DELAY '00:00:10'; -- Simulate processing
COMMIT;
-- Transaction 2 (different session)
SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
SELECT Balance FROM Accounts WHERE AccountId = 1;
-- Waits until Transaction 1 commits
Transaction 1's UPDATE places an exclusive lock on the row
Transaction 2's SELECT requests a shared lock to read the data
The shared lock is incompatible with Transaction 1's exclusive lock
Transaction 2 waits (blocks) until Transaction 1 either commits or rolls back
No dirty reads are allowed - Transaction 2 will only see committed data
Key Characteristics
Prevents dirty reads
Holds shared locks only during the read operation
Allows non-repeatable reads and phantom reads
-- Transaction 1
SET TRANSACTION ISOLATION LEVEL READ COMMITTED;
BEGIN TRANSACTION
SELECT Price FROM Products WHERE ProductId = 1; -- returns 10
-- Transaction 2 (different session)
UPDATE Products SET Price = 15 WHERE ProductId = 1;
COMMIT;
-- Back to Transaction 1
SELECT Price FROM Products WHERE ProductId = 1; -- Returns 15
-- Same query returns different results within the same transaction
Use Cases
OLTP systems and web applications where immediate consistency isn't essential
General-purpose workloads where blocking must be minimized
Drawbacks
Non-repeatable reads can occur
Blocking transactions can impact performance in high-concurrency environments
Recommendation: Bloom Filters for efficient database lookups
REPEATABLE READ
This level ensures consistent reads within a transaction, preventing both dirty and non-repeatable reads. When you read data at this level, SQL Server maintains locks on that data until your transaction completes, ensuring that if you read the same data again, you'll get the same results.
It's like putting a "do not disturb" sign on the paragraphs you're reading so nobody can change them until you're done. While this provides stronger consistency, it comes at the cost of reduced concurrency since other transactions must wait longer to modify the locked data.
-- Transaction 1
SET TRANSACTION ISOLATION LEVEL REPEATABLE READ;
BEGIN TRANSACTION
UPDATE Employees SET Salary = 60000 WHERE EmployeeId = 1;
-- Not committed
-- Transaction 2 (different session)
SELECT * FROM Employees WHERE EmployeeId = 1;
WAITFOR DELAY '00:00:10'
-- Even if someone else updates and commits a new value (70000)
-- This will still show the same value as first SELECT (60000)
SELECT * FROM Employees WHERE EmployeeId = 1;
-- However, new rows can appear (phantom reads)
SELECT * FROM Employees WHERE Salary > 55000;
-- Might show different number of rows if someone INSERTs
-- Blocked until Transaction 1 commits
-- Back to Transaction 1
SELECT Price FROM Products WHERE ProductId = 1; -- Same value as first select
COMMIT;
Transaction 1's UPDATE places an exclusive lock on the row
Transaction 2's SELECT requests a shared lock that's held until end of transaction
Transaction 2 waits for Transaction 1 to commit/rollback
Once Transaction 2 gets its shared lock, no other transaction can modify that data
Subsequent SELECTs in Transaction 2 are guaranteed to see the same data
However, new rows can be inserted that match the WHERE clause (phantom reads)
Key Characteristics
Prevents dirty reads and non-repeatable reads
Holds shared locks until the transaction completes
Can still encounter phantom reads
-- Transaction 1
SET TRANSACTION ISOLATION LEVEL REPEATABLE READ;
BEGIN TRANSACTION
SELECT * FROM Products WHERE Price BETWEEN 10 AND 20;
-- Transaction 2 (different TRANSACTION)
INSERT INTO Products (ProductId, Price) VALUES (999, 15);
COMMIT;
-- Back to Transaction 1
SELECT * FROM Products WHERE Price BETWEEN 10 AND 20;
-- New row appears (phantom)
Use Cases
Financial calculations requiring consistent reads across multiple queries
Complex reports or analytics needing accurate intermediate data
Drawbacks
Increases lock duration, reducing concurrency
Higher chance of deadlocks
Snapshot
SNAPSHOT isolation provides a consistent point-in-time view of data by using row versioning. Instead of using locks, it maintains versions of the data, allowing each transaction to see a consistent view of the database as it existed when the transaction began.
It's like making a personal copy of the document for each reader at the moment they start reading. This provides strong consistency without blocking other transactions, but requires additional storage space to maintain the different versions.
-- Initial state
-- EmployeeId = 1, Salary = 50000
-- TRANSACTION 1
BEGIN TRANSACTION
-- Takes a snapshot of the database at this point
-- Read initial value
SELECT * FROM Employees WHERE EmployeeId = 1; -- Shows 50000
WAITFOR DELAY '00:00:10'
-- TRANSACTION 2 (during TRANSACTION 1's delay)
BEGIN TRANSACTION
UPDATE Employees SET Salary = 60000 WHERE EmployeeId = 1;
COMMIT
-- Back to TRANSACTION 1
-- Still sees old value (50000) despite committed update in TRANSACTION 2
SELECT * FROM Employees WHERE EmployeeId = 1; -- Shows 50000
-- If TRANSACTION 1 tries to update same row
UPDATE Employees SET Salary = 55000 WHERE EmployeeId = 1;
-- Will fail with update conflict error
COMMIT
Key Characteristics
Uses tempdb to store row versions
Avoids blocking between readers and writers
Prevents dirty reads, non-repeatable reads, and phantom reads
Use Cases
Reporting applications requiring consistent views
Long-running queries that shouldn't block writers
Drawbacks
Increased tempdb usage due to row versioning
Update conflicts can occur, requiring error handling in applications
Recommendation: Sharding and Partitioning in Relational Databases
SERIALIZABLE
This is the strictest isolation level, ensuring that transactions behave as if they were executed sequentially. It not only locks the data you read but also prevents other transactions from inserting new rows that would match your query criteria.
Imagine not just protecting the paragraphs you're reading, but also preventing anyone from adding new paragraphs in between them. This provides complete isolation but can significantly impact performance as transactions must wait for each other more frequently.
-- TRANSACTION 1
BEGIN TRANSACTION
-- Places range locks
SELECT * FROM Employees WHERE Salary BETWEEN 50000 AND 70000;
WAITFOR DELAY '00:00:10'
-- Nobody can INSERT/UPDATE/DELETE within this range until transaction completes
SELECT * FROM Employees WHERE Salary BETWEEN 50000 AND 70000;
-- Guaranteed to return same results
COMMIT
-- TRANSACTION 2
-- These will block until TRANSACTION 1's transaction completes
INSERT INTO Employees (EmployeeId, Salary) VALUES (2, 65000);
UPDATE Employees SET Salary = 52000 WHERE EmployeeId = 1;
Key Characteristics
Prevents all concurrency issues, including phantom reads
Uses range locks to block inserts within a query’s range
Ensures maximum consistency at the cost of performance
Use Cases
Financial systems requiring strict consistency
Regulatory compliance scenarios
Critical operations where data integrity is non-negotiable
Drawbacks
Significantly reduces concurrency
Higher chance of blocking and deadlocks
Isolation Levels Compared: Finding the Right Fit for Your Needs
Isolation Level | Dirty Reads | Non-repeatable Reads | Phantom Reads | Locking Strategy | Use Cases |
Read Uncommitted | Yes | Yes | Yes | None | Monitoring, approximate aggregates |
Read Committed | No | Yes | Yes | Shared locks (short) | OLTP systems, web apps |
Repeatable Read | No | No | Yes | Shared locks (long) | Financial calculations |
Snapshot | No | No | No | Row versioning | Reporting, long-running queries |
Serializable | No | No | No | Range locks | Critical transactions |
Conclusion
In conclusion, understanding SQL Server isolation levels is essential for optimizing database performance and ensuring data consistency in concurrent environments. Each isolation level offers a different balance between consistency and concurrency, with specific use cases and trade-offs.
By carefully selecting the appropriate isolation level, you can enhance the efficiency and reliability of your database operations. It's important to thoroughly test and monitor your system to find the right balance that meets your application's requirements, ensuring both data integrity and optimal performance.
Subscribe to my newsletter
Read articles from Rajat Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
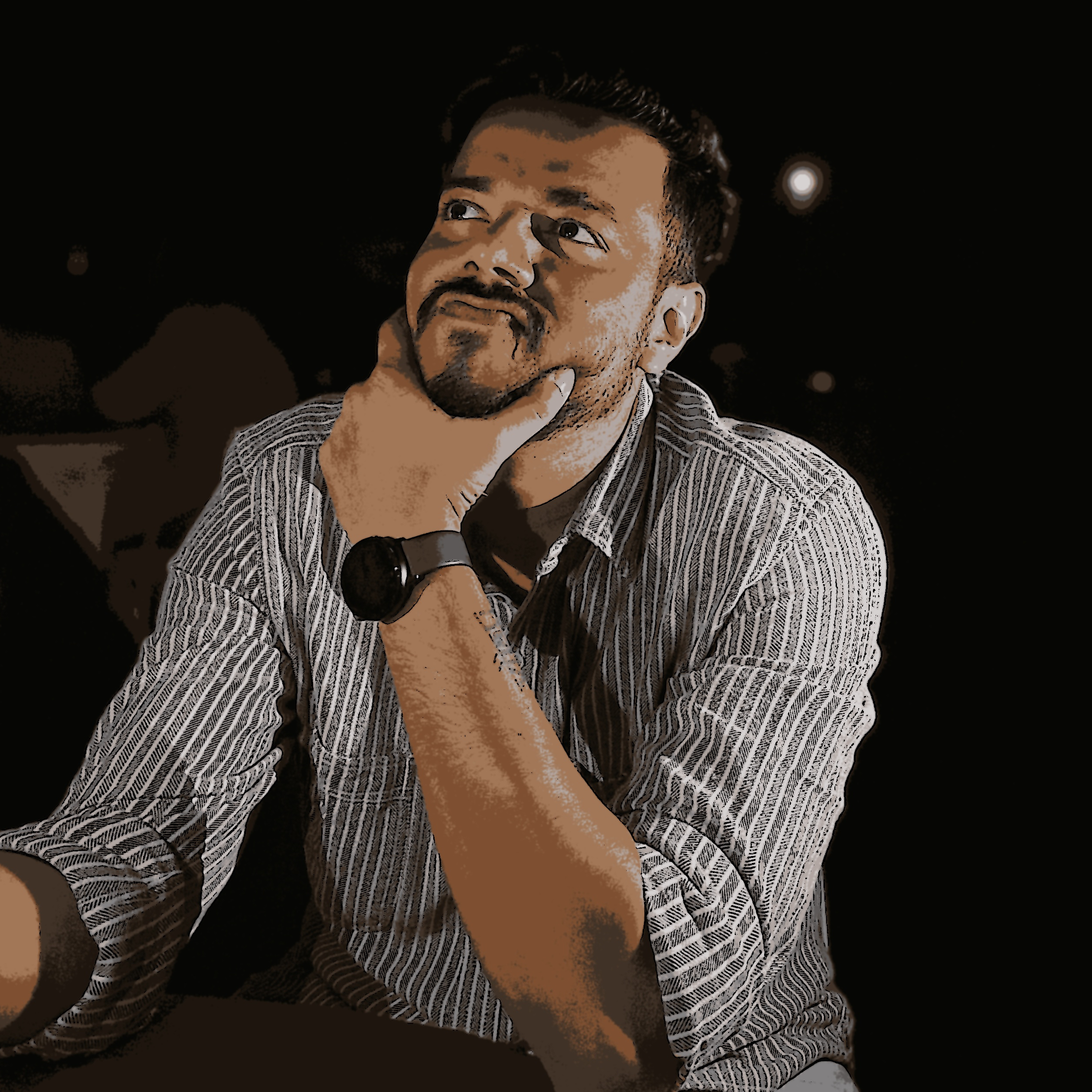