Error Handling in Node.js: Types and Examples intervew guide

Table of contents
- Mastering Error Handling in Node.js: A Complete Guide with Interview Insights. Learn how to handle errors like a pro and ace your Node.js interviews
- Understanding Error Handling Basics
- Types of Error Handling in Node.js
- Best Practices and Common Patterns
- Interview Questions and Answers
- Q1: What are the different ways to handle errors in Node.js?
- 1. Try-Catch Blocks for Synchronous Code
- 2. Error-First Callbacks for Asynchronous Operations
- 3. Promise Chains with .catch()
- 4. Async/Await with Try-Catch Blocks
- Q2: How would you handle errors in Promise chains?
- Q3: What's the difference between operational and programmer errors?
- 1. Operational Errors
- 2. Programmer Errors
- Best Practices for Handling Errors
- Handling Operational vs. Programmer Errors in Practice
- Summary
- Advanced Error Handling Techniques
- Conclusion
- Resources for Further Learning
- Table of Contents
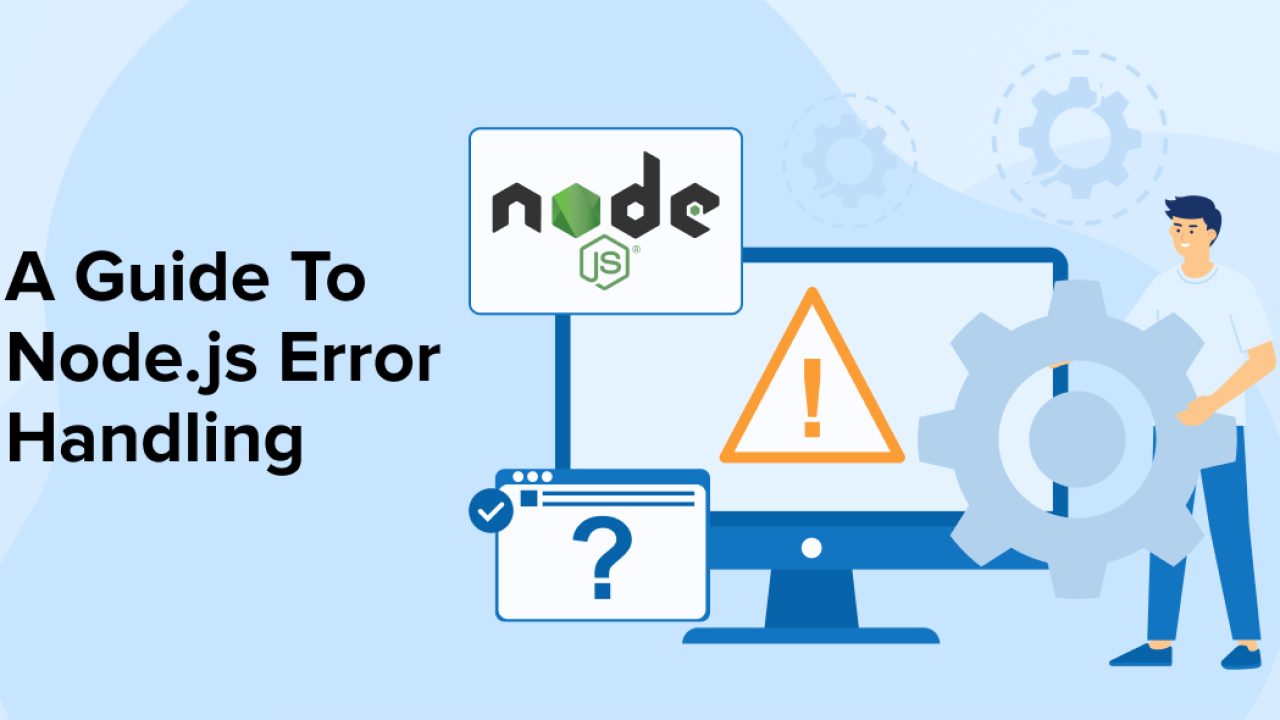
Mastering Error Handling in Node.js: A Complete Guide with Interview Insights. Learn how to handle errors like a pro and ace your Node.js interviews
As a Node.js developer, understanding error handling is crucial for building robust applications. In this comprehensive guide, we'll explore different error handling techniques, see practical examples, and prepare for common interview questions. Whether you're preparing for an interview or looking to improve your Node.js skills, this article has you covered.
Understanding Error Handling Basics
Before diving into specific techniques, let's understand why error handling is crucial in Node.js applications:
Prevents application crashes
Improves user experience
Facilitates debugging
Ensures data integrity
Maintains application reliability
Types of Errors in Node.js
// 1. Standard JavaScript Errors
new Error('Generic error')
new TypeError('Wrong type')
new ReferenceError('Variable not defined')
new SyntaxError('Invalid syntax')
// 2. Custom Errors
class DatabaseError extends Error {
constructor(message) {
super(message);
this.name = 'DatabaseError';
}
}
Types of Error Handling in Node.js
1. Synchronous Error Handling
const fs = require('fs');
function readConfigSync() {
try {
const config = fs.readFileSync('config.json', 'utf8');
return JSON.parse(config);
} catch (error) {
if (error.code === 'ENOENT') {
console.error('Config file not found');
return {};
}
throw error; // Re-throw unexpected errors
}
}
// Usage with proper error handling
try {
const config = readConfigSync();
console.log('Config loaded:', config);
} catch (error) {
console.error('Failed to load config:', error.message);
}
Pros:
Simple to understand and implement
Clear code flow
Good for synchronous operations
Cons:
Blocks the event loop
Not suitable for asynchronous operations
Can impact performance in high-load scenarios
2. Asynchronous Error Handling with Callbacks
const fs = require('fs');
function readConfig(callback) {
fs.readFile('config.json', 'utf8', (error, data) => {
if (error) {
if (error.code === 'ENOENT') {
return callback(null, {});
}
return callback(error);
}
try {
const config = JSON.parse(data);
callback(null, config);
} catch (parseError) {
callback(new Error('Invalid JSON in config file'));
}
});
}
// Usage
readConfig((error, config) => {
if (error) {
console.error('Config error:', error.message);
return;
}
console.log('Config loaded:', config);
});
Pros:
Non-blocking
Traditional Node.js pattern
Good for handling asynchronous operations
Cons:
Can lead to callback hell
Error handling can be verbose
Complex error propagation
3. Promise-Based Error Handling
const fs = require('fs').promises;
function readConfig() {
return fs.readFile('config.json', 'utf8')
.then(data => JSON.parse(data))
.catch(error => {
if (error.code === 'ENOENT') {
return {};
}
throw error;
});
}
// Usage
readConfig()
.then(config => {
console.log('Config loaded:', config);
})
.catch(error => {
console.error('Failed to load config:', error.message);
});
Pros:
Cleaner than callbacks
Better error propagation
Supports chaining
Cons:
Requires understanding of Promise concepts
Can still be verbose for complex operations
Potential for unhandled rejections
4. Async/Await Error Handling
const fs = require('fs').promises;
async function readConfig() {
try {
const data = await fs.readFile('config.json', 'utf8');
return JSON.parse(data);
} catch (error) {
if (error.code === 'ENOENT') {
return {};
}
throw error;
}
}
// Usage
async function init() {
try {
const config = await readConfig();
console.log('Config loaded:', config);
} catch (error) {
console.error('Failed to load config:', error.message);
}
}
init();
Pros:
Clean and readable syntax
Easier error handling
Similar to synchronous code
Better stack traces
Cons:
Requires async function context
Can lead to unnecessary try/catch blocks
Potential for forgetting await
Best Practices and Common Patterns
1. Custom Error Classes
class ApplicationError extends Error {
constructor(message, status = 500) {
super(message);
this.name = 'ApplicationError';
this.status = status;
}
}
class ValidationError extends ApplicationError {
constructor(message) {
super(message, 400);
this.name = 'ValidationError';
}
}
// Usage
function validateUser(user) {
if (!user.email) {
throw new ValidationError('Email is required');
}
}
2. Global Error Handler
process.on('uncaughtException', (error) => {
console.error('Uncaught Exception:', error);
// Perform cleanup and exit
process.exit(1);
});
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection at:', promise, 'reason:', reason);
// Handle the error or exit
});
Interview Questions and Answers
Q1: What are the different ways to handle errors in Node.js?
Answer: "In Node.js, there are four main approaches to handle errors:
Try-catch blocks for synchronous code
Error-first callbacks for asynchronous operations
Promise chains with .catch()
Async/await with try-catch blocks
Let me demonstrate with a practical example..."
Here's how the different ways to handle errors in Node.js can be implemented with code examples for each method:
1. Try-Catch Blocks for Synchronous Code
In synchronous code, you can use a try-catch
block to handle errors. If an error is thrown, it will be caught in the catch
block.
javascriptCopy codefunction divideNumbers(a, b) {
try {
if (b === 0) throw new Error("Division by zero is not allowed!");
return a / b;
} catch (error) {
console.error("Error:", error.message);
}
}
console.log(divideNumbers(10, 2)); // Output: 5
console.log(divideNumbers(10, 0)); // Output: Error: Division by zero is not allowed!
2. Error-First Callbacks for Asynchronous Operations
In Node.js, it's common to use error-first callbacks. The first parameter of the callback is reserved for an error, if any occurs.
javascriptCopy codeconst fs = require('fs');
// Error-first callback example
fs.readFile('nonexistent-file.txt', 'utf8', (err, data) => {
if (err) {
console.error("Error reading file:", err.message);
return;
}
console.log("File content:", data);
});
3. Promise Chains with .catch()
Promises provide a cleaner way to handle errors asynchronously. You can use the .catch()
method at the end of a promise chain to handle any errors.
javascriptCopy codeconst fs = require('fs').promises;
// Using Promises with .catch()
fs.readFile('nonexistent-file.txt', 'utf8')
.then(data => {
console.log("File content:", data);
})
.catch(err => {
console.error("Error reading file:", err.message);
});
4. Async/Await with Try-Catch Blocks
The async/await syntax allows you to handle asynchronous code similarly to synchronous code using try-catch
blocks.
javascriptCopy codeconst fs = require('fs').promises;
async function readFileAsync() {
try {
const data = await fs.readFile('nonexistent-file.txt', 'utf8');
console.log("File content:", data);
} catch (error) {
console.error("Error reading file:", error.message);
}
}
readFileAsync();
Each of these methods has its strengths and should be used according to the context in which the error may occur (synchronous vs. asynchronous, callback-based vs. promise-based).
Q2: How would you handle errors in Promise chains?
Answer: "When working with Promise chains, I handle errors in multiple ways:
Using .catch() at the end of the chain
Using .catch() in the middle for specific error recovery
Combining with async/await when needed
Here's a practical example..."
function processData() {
return fetchData()
.then(validate)
.then(transform)
.catch(error => {
if (error.name === 'ValidationError') {
return handleValidationError(error);
}
throw error;
})
.then(save)
.catch(error => {
console.error('Processing failed:', error);
throw error;
});
}
Q3: What's the difference between operational and programmer errors?
Answer: "There are two main categories of errors in Node.js:
Operational Errors:
Expected errors during normal operation
Examples: file not found, network timeout
Should be handled gracefully
Programmer Errors:
Bugs in the code
Examples: trying to read undefined, type errors
Should crash and restart the application
Let me show you how to handle each type..."
Here's how to handle both Operational Errors and Programmer Errors in Node.js:
1. Operational Errors
Operational errors are expected errors that can happen during normal operation. These errors are often due to factors outside of your code’s control, such as a missing file, a network issue, or a database timeout. They should be handled gracefully, so your application can recover or provide a meaningful response to the user.
Handling Operational Errors
Below are examples of handling operational errors:
javascriptCopy codeconst fs = require('fs');
// Handling an operational error: file not found
fs.readFile('nonexistent-file.txt', 'utf8', (err, data) => {
if (err) {
// This is an operational error
console.error("Operational Error: File not found. Details:", err.message);
// Handle the error gracefully, perhaps by showing a message or default content
return;
}
console.log("File content:", data);
});
In this example:
The error of a file not being found is anticipated.
The code handles it gracefully by logging an appropriate message instead of crashing the application.
Another example using promises:
javascriptCopy codeconst fs = require('fs').promises;
async function readFileAsync() {
try {
const data = await fs.readFile('nonexistent-file.txt', 'utf8');
console.log("File content:", data);
} catch (error) {
// Handling an operational error
console.error("Operational Error: Could not read file. Details:", error.message);
}
}
readFileAsync();
2. Programmer Errors
Programmer errors are bugs in your code. These errors usually happen due to a coding mistake, such as trying to access an undefined variable or using an incorrect function. Unlike operational errors, programmer errors are unexpected and indicate that there’s a problem in your code logic. These errors should typically cause the application to crash and restart, rather than being handled at runtime.
Handling Programmer Errors
In Node.js, it's often recommended to let the application crash for programmer errors so you can fix the underlying issue rather than masking the error. Here’s an example:
javascriptCopy code// Simulating a programmer error: Trying to access a property of undefined
function simulateProgrammerError() {
try {
let user;
console.log(user.name); // This will throw a TypeError
} catch (error) {
// This is a programmer error
console.error("Programmer Error: Something is wrong in the code. Details:", error.message);
// Throw the error to let the application crash and restart
throw error;
}
}
simulateProgrammerError();
In this example:
A
TypeError
occurs because the code tries to access a property ofundefined
.The error is caught, but the application is allowed to crash by re-throwing the error so that it can be fixed during development.
Best Practices for Handling Errors
Operational Errors: These should be handled gracefully using mechanisms like error-first callbacks,
.catch()
in Promises, ortry-catch
blocks inasync/await
. It’s crucial to log the error details for troubleshooting.Programmer Errors: These should not be caught unless you’re logging them for debugging purposes. It’s better to let the application crash and restart, especially in production environments. Use tools like Node.js Cluster, PM2, or Docker to handle restarts when a crash happens.
Handling Operational vs. Programmer Errors in Practice
Here’s how you might structure a real-world Node.js application to handle both types:
javascriptCopy codeconst fs = require('fs').promises;
// Example function for reading a file, catching operational errors
async function readUserFile(fileName) {
try {
const data = await fs.readFile(fileName, 'utf8');
console.log("User file content:", data);
} catch (error) {
// Operational error handling
if (error.code === 'ENOENT') {
console.error("Operational Error: File not found -", fileName);
} else {
console.error("Operational Error:", error.message);
}
}
}
// Simulating a function with a potential programmer error
function processUserData(userData) {
if (!userData) {
// This is a programmer error; let it crash
throw new Error("Programmer Error: User data is undefined!");
}
// ... process the userData
}
// Using the functions
readUserFile('users.json'); // Operational error handling example
processUserData(undefined); // Programmer error example (causes crash)
Summary
Operational Errors: Handle them gracefully so your app can continue running. Use error messages to notify users of the issue or provide fallback content.
Programmer Errors: These indicate bugs. Don't mask them—let the app crash, fix the issue, and deploy a new version.
By understanding the difference between operational and programmer errors, you can build a more robust Node.js application, ensuring that unexpected situations are handled correctly while also quickly identifying and fixing bugs during development.
Advanced Error Handling Techniques
1. Error Middleware (Express.js)
const express = require('express');
const app = express();
// Error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
if (err instanceof ValidationError) {
return res.status(400).json({
error: err.message
});
}
res.status(500).json({
error: 'Something went wrong!'
});
});
2. Error Recovery Patterns
async function retryOperation(operation, maxAttempts = 3) {
for (let attempt = 1; attempt <= maxAttempts; attempt++) {
try {
return await operation();
} catch (error) {
if (attempt === maxAttempts) throw error;
console.log(`Attempt ${attempt} failed, retrying...`);
await new Promise(resolve => setTimeout(resolve, 1000 * attempt));
}
}
}
// Usage
await retryOperation(async () => {
return await fetchDataFromAPI();
});
Conclusion
Error handling is a critical skill for Node.js developers. By understanding and implementing proper error handling techniques, you can build more reliable applications and handle interviews with confidence. Remember:
Always handle both synchronous and asynchronous errors appropriately
Use custom error classes for better error management
Implement global error handlers for uncaught errors
Choose the right error handling pattern based on your use case
Practice explaining your error handling approach for interviews
Resources for Further Learning
Node.js Official Documentation
Error Handling Best Practices
Express.js Error Handling Guide
JavaScript Error Handling Patterns
Table of Contents
Connect with me
If you have any questions about my projects or want to discuss potential collaboration opportunities, please don't hesitate to connect with me. You can reach me through the following channels:
Email: bodheeshvc.developer@gmail.com
LinkedIn: www.linkedin.com/in/bodheeshvc
GitHub: https://github.com/BODHEESH
Youtube: https://www.youtube.com/@BodhiTechTalks
Medium: https://medium.com/@bodheeshvc.developer
Twitter: https://x.com/Bodheesh_
I'm always happy to connect with other professionals in the tech industry and discuss ways to work together. Feel free to reach out and let's see how we can help each other grow and succeed!
What error handling patterns do you use in your Node.js applications? Share your experiences and best practices in the comments below!
Subscribe to my newsletter
Read articles from Bodheesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bodheesh
Bodheesh
🚀 Bodheesh V C | Software Engineer | Tech Blogger | Vlogger | Learning Enthusiast | Node.js Developer | MERN Stack Advocate As a backend developer with over 2.5 years of experience, I’m passionate about building efficient, scalable systems that drive impactful results. Specializing in Node.js and the MERN stack, I’ve had the opportunity to work on a wide array of projects—from creating seamless APIs and real-time applications to implementing effective codes that enhance performance and scalability. I believe in simplicity and maintainability, striving to write clean, well-documented code that not only solves the problem at hand but also sets the foundation for future growth. My experience spans a variety of domains including backend architecture, database management, API development and cloud integrations. Beyond coding, I have a deep interest in sharing knowledge with the developer community. I actively contribute to blogs and social platforms, simplifying complex concepts around system design, DSA (Data Structures and Algorithms), and interview preparation, so that developers at all levels can benefit. My tech blogs, interview guides, and insights into best coding practices are geared toward helping others grow and succeed in their software development journey. I am also a vlogger, and I’ve created content around tech trends, tutorials, and tools to inspire others to pursue their passion for technology. As a continuous learner, I’m constantly exploring emerging technologies, such as AI-driven development, and finding ways to incorporate these advancements into real-world applications. My enthusiasm for coding, combined with my commitment to lifelong learning, keeps me motivated and excited for the future of software development. What I do: Backend development, specializing in Node.js and MERN stack Creating scalable, high-performance Applications Building RESTful APIs and microservices Writing tech blogs on Node.js, system design, and coding best practices Preparing developers for interviews with practical guidance on DSA and problem-solving Advocating for clean code and maintainable architectures Sharing knowledge through tutorials, blogs, and videos Whether it’s collaborating on an exciting project, learning from fellow developers, or simply discussing the latest in tech, I’m always eager to connect and grow together with the community. Let’s build something great!