Docker Compose & Yaml
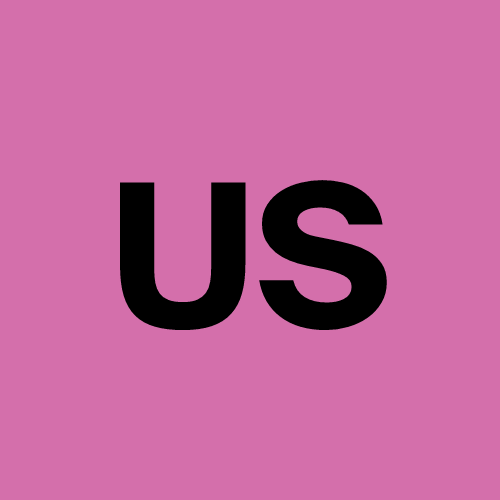
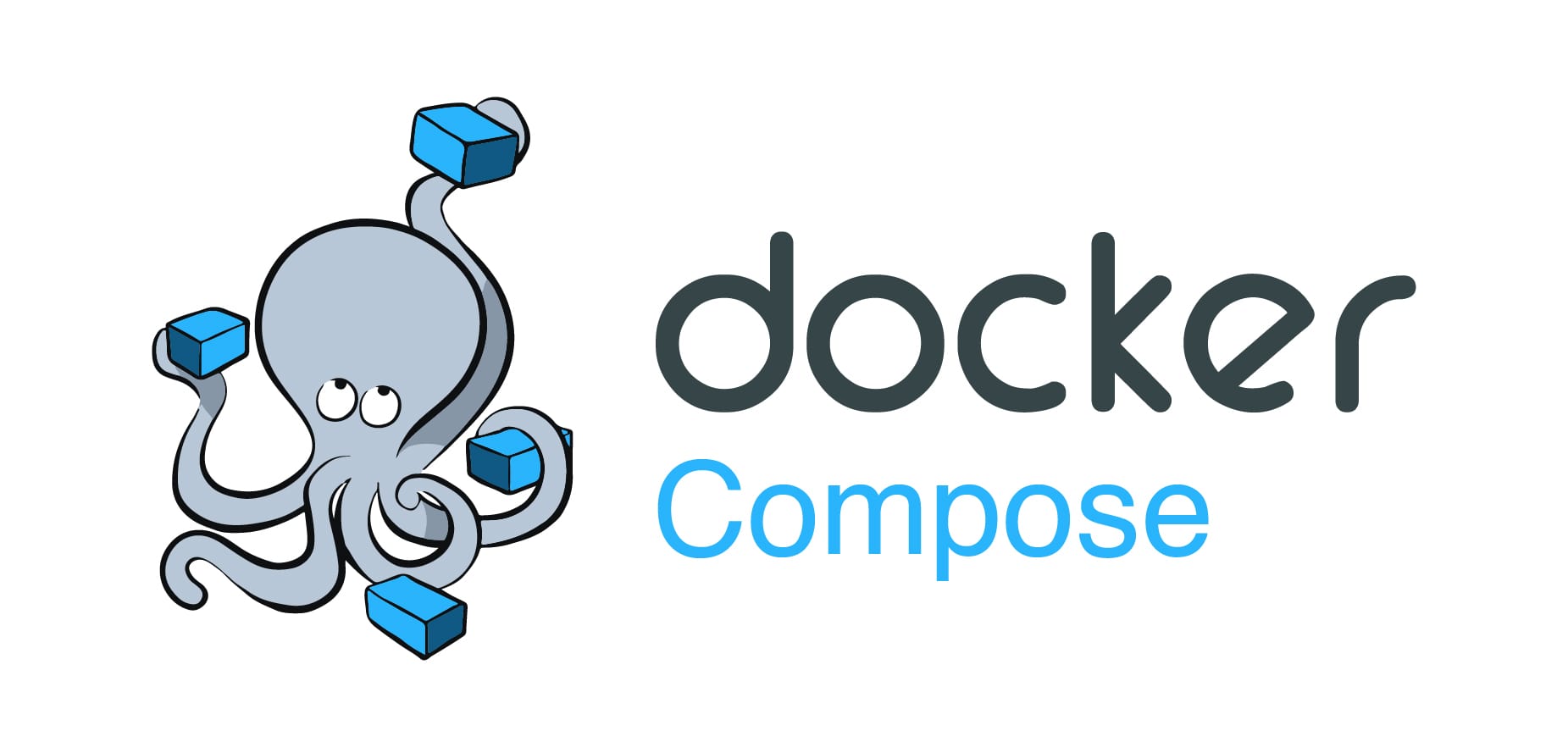
Docker Compose
Docker Compose is a tool that allows you to define and manage multi-container Docker applications using a simple YAML file called docker-compose.yml. With Docker Compose, you can:
Define Services: Specify the various services that make up your application (e.g., web server, database, cache).
Configure Services: Set up how each service should run, including settings like ports, volumes, and environment variables.
Manage Multi-Container Applications: Use a single command to start and stop all services, simplifying the orchestration of complex applications.
Basic Commands:
docker-compose up: Starts the application and creates containers for each service defined in the docker-compose.yml.
docker-compose down: Stops and removes all containers defined in the docker-compose.yml.
docker-compose logs: Displays the logs from all containers in the application.
What is YAML?
YAML (YAML Ain’t Markup Language) is a human-readable data serialization language often used for configuration files. It is designed to be simple and easy to read, making it a popular choice for developers.
Key Features of YAML:
Human-Readable: Easy to read and write, making it suitable for configuration files.
Hierarchical Structure: Supports nested data structures, allowing for complex configurations.
Data Serialization: Ideal for representing data structures such as lists and dictionaries.
File Extensions: YAML files typically have a .yml or .yaml extension.
We will create a project with :
A Flask application that serves a simple web page.
A Redis database that the Flask app will use for storing and retrieving data.
Docker Compose to orchestrate these services.
Step-by-Step Solution
Step 1: Set Up Project Directory
- Create a project directory:
mkdir flask-redis-docker
cd flask-redis-docker
- Create subdirectories for the Flask app:
mkdir web
Step 2: Create Flask Application
- Create a file named app.py in the web directory:
# web/app.py
from werkzeug.urls import url_quote_plus as url_quote
from flask import Flask, jsonify
import redis
import os
app = Flask(__name__)
redis_host = os.environ.get('REDIS_HOST', 'redis')
r = redis.Redis(host=redis_host, port=6379, db=0)
@app.route('/')
def index():
visits = r.incr('counter')
return jsonify({'message': f'Hello, you are visitor number {visits}'})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
- Create a requirements.txt file in the web directory:
Flask==2.2.2
redis==4.4.0
werkzeug==2.2.2
Step 3: Create a Dockerfile for Flask App
- Create a Dockerfile in the web directory:
# web/Dockerfile
FROM python:3.9
WORKDIR /app
COPY requirements.txt requirements.txt
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Step 4: Create Docker Compose File
- Create a docker-compose.yml file in the root directory:
version: '3.8'
services:
web:
build:
context: ./web
ports:
- "5000:5000"
environment:
- REDIS_HOST=redis
depends_on:
- redis
redis:
image: redis:alpine
ports:
- "6379:6379"
Step 5: Build and Run the Application
- Navigate to the root of the project directory:
cd flask-redis-docker
- Run the following command to build and start the services:
docker-compose up --build
Step 6: Access the Application
- Open your web browser and go to:
http://localhost:5000
- You should see a JSON response like:
{"message": "Hello, you are visitor number 1"}
Step 7: Manage the Containers
- To stop the containers:
docker-compose down
- To view the logs:
docker-compose logs
- To start the containers again:
docker-compose up
Step 8: Remove the Containers
- To remove all containers defined in the docker-compose.yml:
docker-compose down
Subscribe to my newsletter
Read articles from Urvish Suhagiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
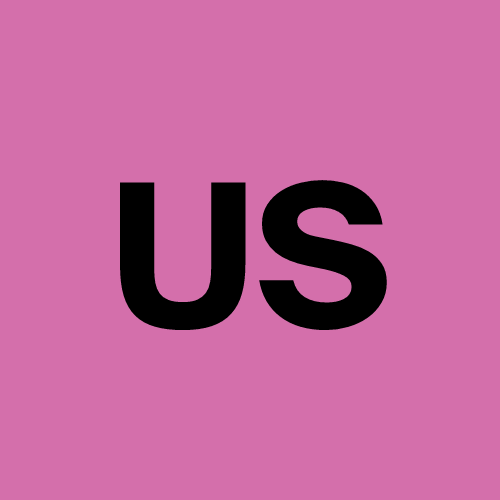
Urvish Suhagiya
Urvish Suhagiya
Exploring the world of DevOps 🌐.