AI-Powered X Bot: Automatic Translation and Reposting
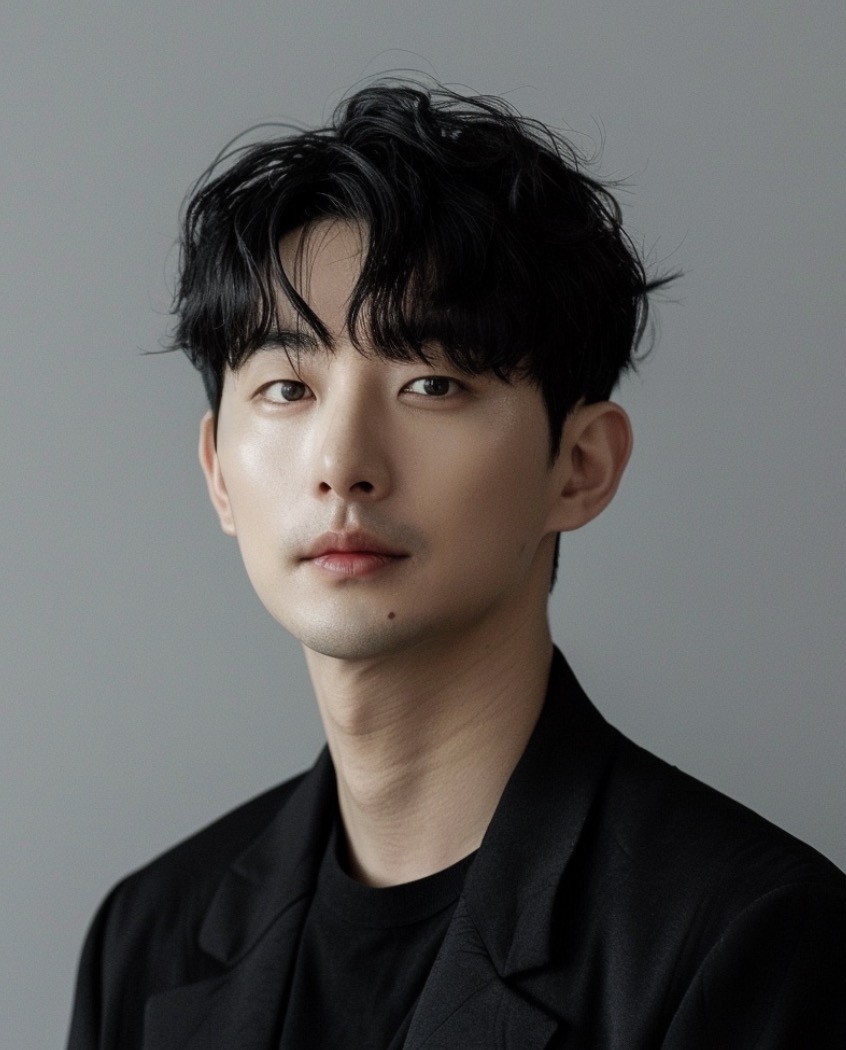
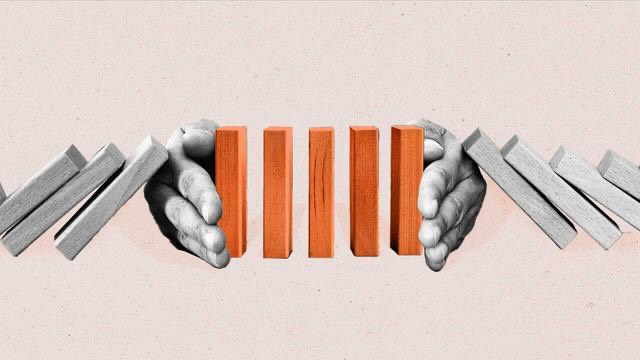
To create an AI agent that monitors specific Twitter accounts, translates their tweets using the OpenAI API, and reposts them along with any media to your own Twitter account, follow the step-by-step guide below.
1. Set Up Twitter API Access
a. Create a Twitter Developer Account:
Visit the Twitter Developer Portal and sign up for a developer account.
Apply for the appropriate level of access. Note that Twitter's API access policies may require you to subscribe to a paid tier for certain functionalities.
b. Create a Project and App:
Once approved, create a new project and app within the developer portal.
Generate your API Key, API Secret Key, Access Token, and Access Token Secret.
Securely store these credentials; never expose them in public code repositories.
2. Set Up OpenAI API Access
a. Sign Up for OpenAI API:
Visit the OpenAI website and sign up for API access.
Obtain your API key from the OpenAI dashboard.
Keep your API key confidential.
3. Set Up Your Development Environment
a. Choose a Programming Language:
- We'll use Python due to its robust libraries for both Twitter and OpenAI APIs.
b. Install Required Libraries:
pip install tweepy
pip install openai
pip install requests
tweepy
: For interacting with the Twitter API.openai
: For accessing OpenAI's API.requests
: For handling HTTP requests (downloading media).
4. Authenticate with the APIs
a. Twitter Authentication:
import tweepy
# Twitter API credentials
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_API_SECRET'
access_token = 'YOUR_ACCESS_TOKEN'
access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET'
# Authenticate to Twitter
auth = tweepy.OAuth1UserHandler(api_key, api_secret, access_token, access_token_secret)
api = tweepy.API(auth)
b. OpenAI Authentication:
import openai
# OpenAI API key
openai.api_key = 'YOUR_OPENAI_API_KEY'
5. Monitor Specific Twitter Accounts
a. Get User IDs:
You need the user IDs (not usernames) of the accounts you want to monitor.
Use the following to retrieve user IDs:
usernames = ['username1', 'username2'] # Replace with actual usernames
user_ids = []
for username in usernames:
user = api.get_user(screen_name=username)
user_ids.append(str(user.id))
b. Set Up a Stream Listener:
class MyStreamListener(tweepy.StreamListener):
def on_status(self, status):
process_tweet(status)
def on_error(self, status_code):
if status_code == 420:
# Disconnects the stream to avoid being rate limited
return False
# Initialize and start the stream
myStreamListener = MyStreamListener()
myStream = tweepy.Stream(auth=api.auth, listener=myStreamListener)
myStream.filter(follow=user_ids)
6. Process Incoming Tweets
import os
import requests
def process_tweet(status):
try:
# Skip retweets
if hasattr(status, 'retweeted_status'):
return
text = status.text
media_files = []
# Check for media
if 'media' in status.entities:
for media in status.entities['media']:
media_url = media['media_url_https']
filename = download_media(media_url)
media_files.append(filename)
# Translate text
translated_text = translate_text(text)
# Post tweet
post_tweet(translated_text, media_files)
except Exception as e:
print(f"Error processing tweet: {e}")
7. Download Media from Tweets
def download_media(url):
filename = url.split('/')[-1]
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as f:
f.write(response.content)
return filename
else:
print(f"Failed to download media: {url}")
return None
8. Translate the Tweet Text
def translate_text(text, target_language='English'):
prompt = f"Translate the following text to {target_language}:\n\n{text}"
response = openai.Completion.create(
engine="text-davinci-003", # or 'gpt-3.5-turbo' if available
prompt=prompt,
max_tokens=500,
temperature=0.5,
)
translated_text = response.choices[0].text.strip()
return translated_text
9. Repost the Translated Tweet with Media
def post_tweet(text, media_files):
media_ids = []
for file in media_files:
if file:
res = api.media_upload(file)
media_ids.append(res.media_id)
os.remove(file) # Clean up after uploading
if media_ids:
api.update_status(status=text, media_ids=media_ids)
else:
api.update_status(status=text)
10. Error Handling and Logging
Wrap your main functions with try-except blocks.
Implement logging to monitor your agent's activities.
import logging
logging.basicConfig(level=logging.INFO)
def process_tweet(status):
try:
# Existing code...
except Exception as e:
logging.error(f"Error processing tweet: {e}")
11. Deployment Considerations
a. Running the Agent Continuously:
Deploy your script on a server or cloud service (e.g., AWS, Google Cloud, Heroku).
Use process managers like
supervisor
orsystemd
to keep the script running.
b. Secure Storage of Credentials:
Use environment variables or a configuration management tool.
For example, in Linux:
export TWITTER_API_KEY='your_api_key'
export OPENAI_API_KEY='your_openai_api_key'
- In your script:
import os
api_key = os.getenv('TWITTER_API_KEY')
openai.api_key = os.getenv('OPENAI_API_KEY')
12. Compliance and Best Practices
a. Twitter's Terms of Service:
Ensure you have permission to repost content.
Attribution may be required; consider mentioning the original author.
b. OpenAI's Usage Policies:
Comply with OpenAI's API policies.
Do not use the API to generate disallowed content.
c. Rate Limits:
Monitor and respect rate limits for both Twitter and OpenAI APIs.
Implement backoff strategies or limit the frequency of API calls.
13. Full Example Script
Below is the consolidated script incorporating all the steps:
import tweepy
import openai
import requests
import os
import logging
# Set up logging
logging.basicConfig(level=logging.INFO)
# Twitter API credentials from environment variables
api_key = os.getenv('TWITTER_API_KEY')
api_secret = os.getenv('TWITTER_API_SECRET')
access_token = os.getenv('TWITTER_ACCESS_TOKEN')
access_token_secret = os.getenv('TWITTER_ACCESS_TOKEN_SECRET')
# OpenAI API key
openai.api_key = os.getenv('OPENAI_API_KEY')
# Authenticate to Twitter
auth = tweepy.OAuth1UserHandler(api_key, api_secret, access_token, access_token_secret)
api = tweepy.API(auth)
# User IDs to monitor
usernames = ['username1', 'username2'] # Replace with actual usernames
user_ids = []
for username in usernames:
user = api.get_user(screen_name=username)
user_ids.append(str(user.id))
def download_media(url):
filename = url.split('/')[-1]
response = requests.get(url)
if response.status_code == 200:
with open(filename, 'wb') as f:
f.write(response.content)
return filename
else:
logging.error(f"Failed to download media: {url}")
return None
def translate_text(text, target_language='English'):
prompt = f"Translate the following text to {target_language}:\n\n{text}"
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=500,
temperature=0.5,
)
translated_text = response.choices[0].text.strip()
return translated_text
def post_tweet(text, media_files):
media_ids = []
for file in media_files:
if file:
res = api.media_upload(file)
media_ids.append(res.media_id)
os.remove(file)
if media_ids:
api.update_status(status=text, media_ids=media_ids)
else:
api.update_status(status=text)
def process_tweet(status):
try:
# Skip retweets and replies
if hasattr(status, 'retweeted_status') or status.in_reply_to_status_id:
return
text = status.text
media_files = []
# Check for media
if 'media' in status.entities:
for media in status.entities['media']:
media_url = media['media_url_https']
filename = download_media(media_url)
if filename:
media_files.append(filename)
# Translate text
translated_text = translate_text(text)
# Post tweet
post_tweet(translated_text, media_files)
logging.info(f"Reposted tweet from {status.user.screen_name}")
except Exception as e:
logging.error(f"Error processing tweet: {e}")
class MyStreamListener(tweepy.StreamListener):
def on_status(self, status):
process_tweet(status)
def on_error(self, status_code):
logging.error(f"Stream error: {status_code}")
if status_code == 420:
# Disconnects the stream
return False
myStreamListener = MyStreamListener()
myStream = tweepy.Stream(auth=api.auth, listener=myStreamListener)
logging.info("Starting Twitter stream...")
myStream.filter(follow=user_ids)
14. Testing Your Agent
Run the script in a controlled environment first.
Monitor the output and logs to ensure it's working as expected.
Test edge cases, such as tweets without media or tweets in different languages.
15. Enhancements and Additional Features
Language Detection: Implement language detection to handle tweets in various languages.
Error Notifications: Set up email or messaging alerts for critical errors.
User Interface: Create a simple interface to add or remove accounts to monitor.
Database Integration: Store processed tweets to avoid duplicates.
16. Important Considerations
Legal and Ethical Compliance: Always ensure that your actions comply with legal requirements and ethical standards.
Privacy: Do not store or share sensitive user data.
Attribution: Consider giving credit to original authors when reposting content.
Scalability: If monitoring multiple accounts or handling high tweet volumes, optimize your code for scalability.
By following these steps, you'll have an AI agent capable of monitoring specified Twitter accounts, translating their tweets, and reposting them along with any media to your own account. Remember to keep your API keys secure and to respect the terms of service of both Twitter and OpenAI.
Subscribe to my newsletter
Read articles from Ewan Mak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
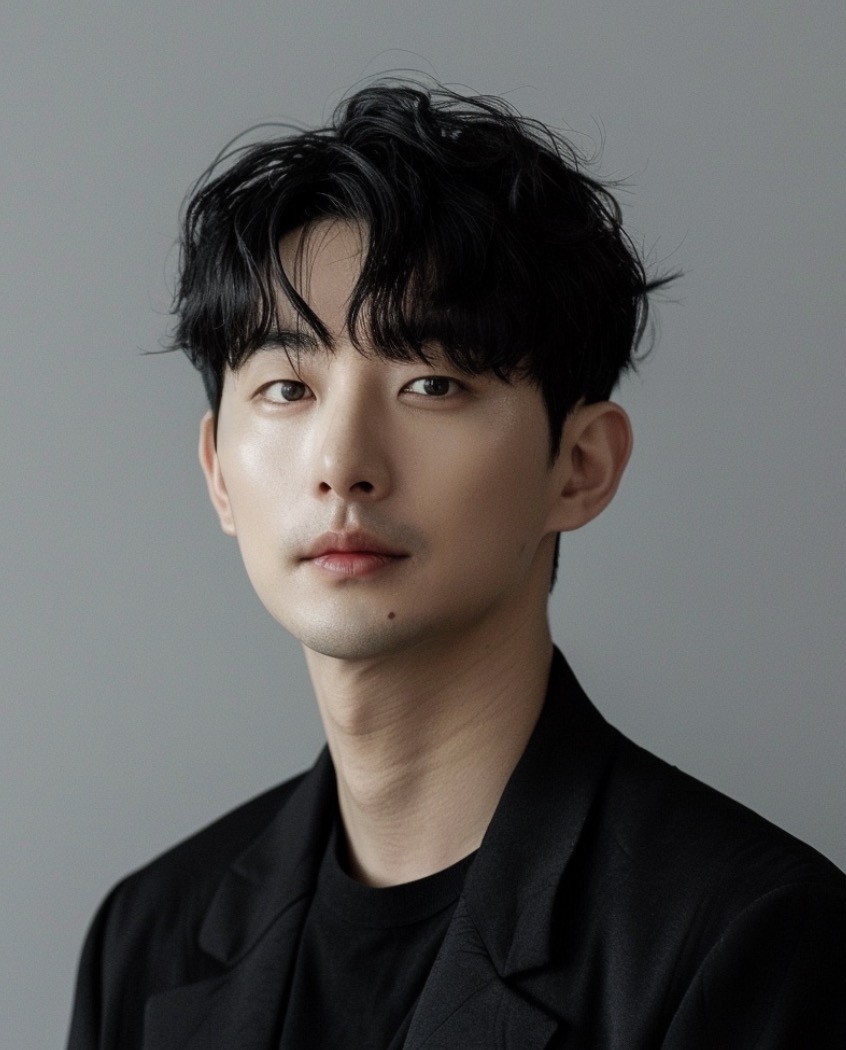
Ewan Mak
Ewan Mak
Crafting seamless user experiences with a passion for headless CMS, Vercel deployments, and Cloudflare optimization. I'm a Full Stack Developer with expertise in building modern web applications that are blazing fast, secure, and scalable. Let's connect and discuss how I can help you elevate your next project!