Web Performance Testing: Tools and Techniques for Core Web Vitals
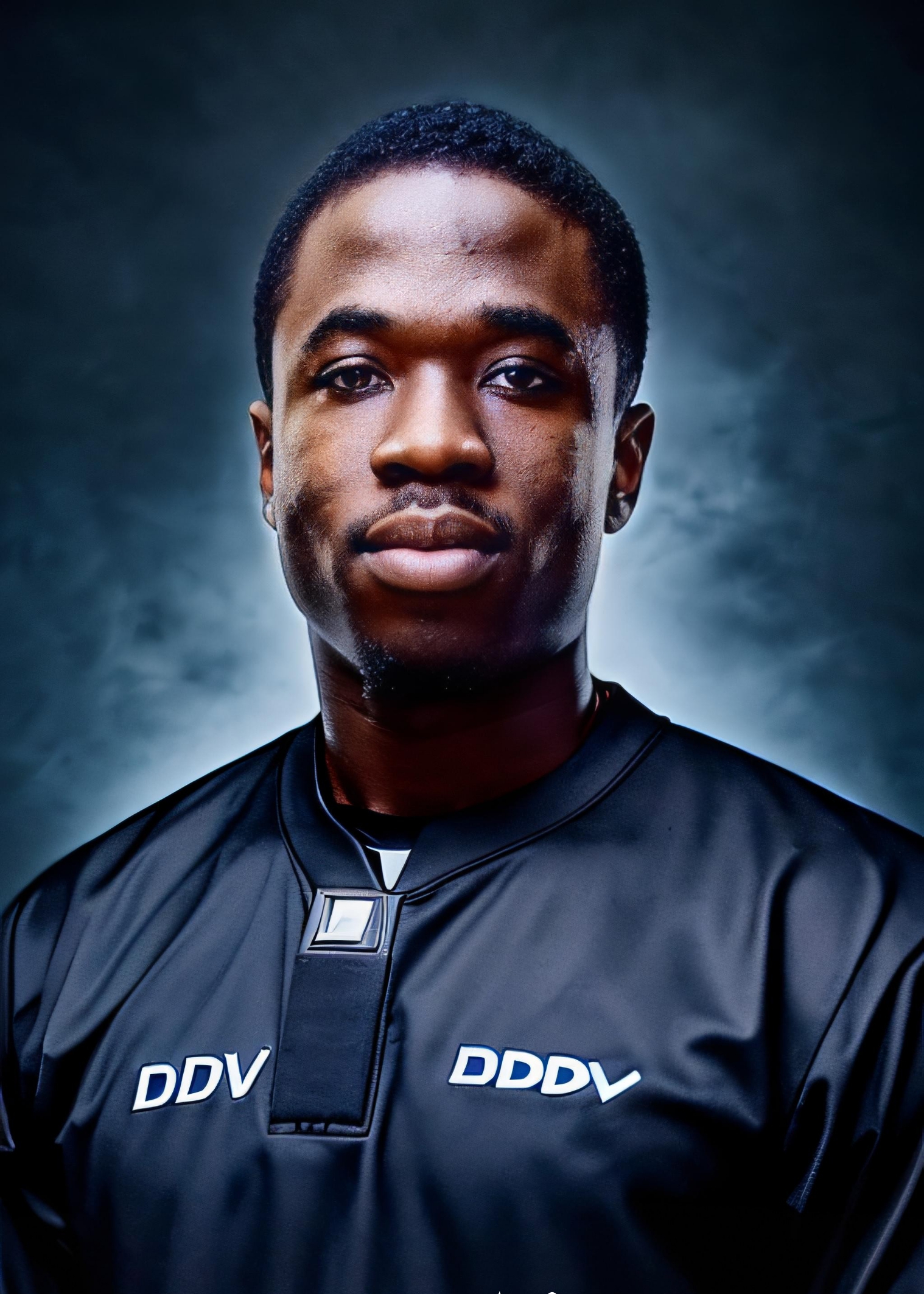
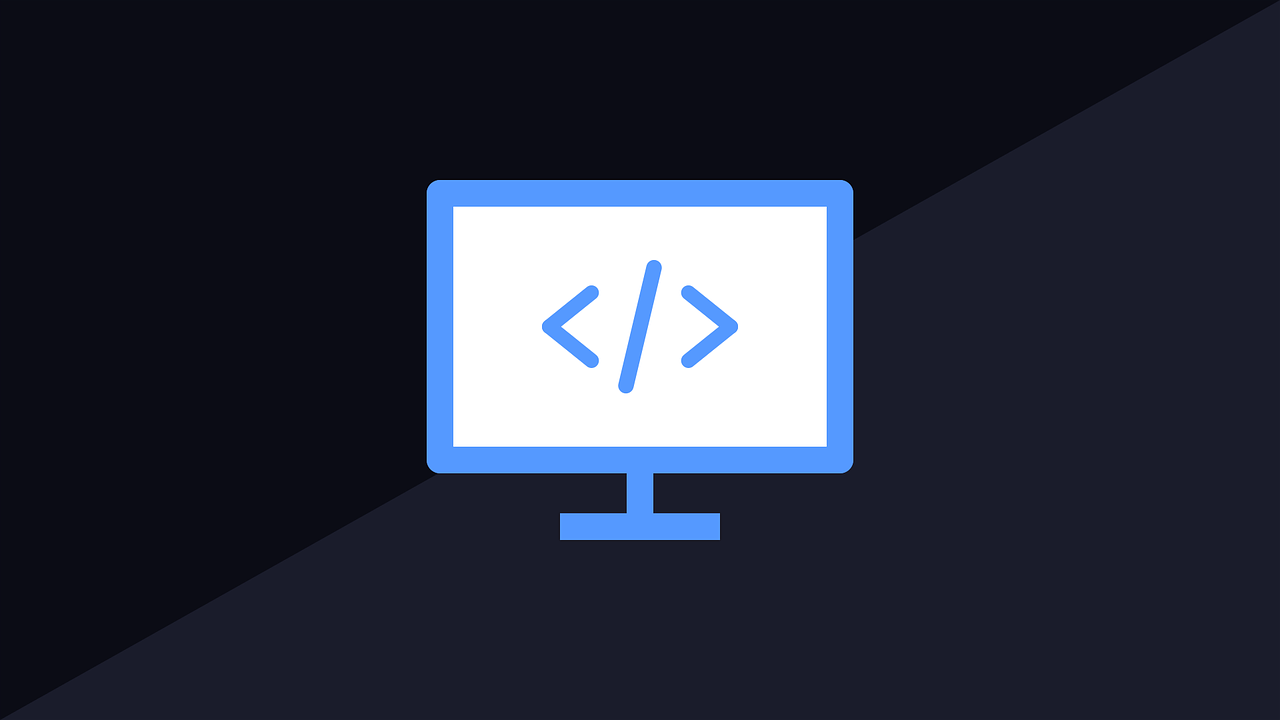
Introduction
In today’s digital landscape, web performance has become a critical factor in user retention, engagement, and conversion rates. A fast, smooth user experience is more than a luxury—it’s a necessity. Google’s Core Web Vitals (CWV) are at the forefront of measuring and improving web performance. They focus on aspects of speed, responsiveness, and stability that are crucial to a user’s experience.
This article serves as a comprehensive guide to understanding each Core Web Vital, how to effectively test them using popular tools like Lighthouse and WebPageTest, and strategies to improve them with code examples. Whether you’re a developer looking to boost performance or a product owner aiming to improve user satisfaction, this guide has you covered.
Understanding Core Web Vitals
What Are Core Web Vitals?
Core Web Vitals are a set of metrics defined by Google that represent key aspects of user experience: loading, interactivity, and visual stability. These metrics are not just about website speed but about how quickly a site becomes useful and stable to the end user. Each metric provides a measurable threshold that can be used to gauge user experience and optimize it accordingly.
Detailed Breakdown of Each Core Web Vital Metric
1. Largest Contentful Paint (LCP)
Definition: LCP measures the loading performance of a page by timing the render of the largest image or text block visible within the user’s viewport. It tells us when the page content becomes readable or interactive, making it one of the first impressions of speed.
Ideal Benchmark: To provide a good user experience, aim for an LCP of less than 2.5 seconds.
Importance: A fast LCP means users quickly see meaningful content on the screen, reducing perceived load time and enhancing user engagement.
2. First Input Delay (FID)
Definition: FID assesses the interactivity of a web page by measuring the delay between a user’s first interaction (such as a click or tap) and the browser’s response. This is especially relevant for interactive sites, like e-commerce or single-page applications, where delays can directly impact usability.
Ideal Benchmark: For an optimal experience, aim for an FID of less than 100 milliseconds.
Importance: Quick responses to user input ensure users do not experience frustrating lags, keeping them engaged and reducing the chance of abandonment.
3. Cumulative Layout Shift (CLS)
Definition: CLS measures the visual stability of a page by quantifying unexpected shifts in layout that happen while content is loading. This metric tracks any changes that cause users to lose context or, worse, click on the wrong elements.
Ideal Benchmark: A good user experience typically requires a CLS score of less than 0.1.
Importance: Visual stability is essential for accessibility and usability, as unexpected shifts can lead to user frustration, errors, and a negative impression of the site’s quality.
Comparing Popular Web Performance Testing Tools
Effective testing is key to identifying performance bottlenecks related to Core Web Vitals. Here’s a comparison of some of the most popular testing tools that can help you measure, analyze, and improve CWV scores.
Overview of Popular Tools
Several tools on the market provide insights into Core Web Vitals, each with its strengths. Below are some of the most effective tools used by developers:
1. Lighthouse
Overview and Purpose: Lighthouse is a Google tool available in Chrome DevTools. It provides an automated analysis of page quality with metrics for performance, accessibility, SEO, and more.
Key Features: Lighthouse measures all three Core Web Vitals (LCP, FID, CLS) and provides suggestions for improvement in each area.
Pros and Cons:
Pros: Easy to use, directly integrated into Chrome, offers clear suggestions.
Cons: Limited in simulating real-world conditions, as tests are run in a controlled environment.
2. WebPageTest
Overview and Purpose: WebPageTest is an open-source web performance tool that allows for highly configurable testing scenarios, including testing from multiple geographic locations and browsers.
Key Features: The tool provides granular reports, video capture of loading processes, and the ability to simulate various connection speeds.
Pros and Cons:
Pros: Highly customizable, allows in-depth testing with detailed metrics.
Cons: More complex interface and configuration compared to other tools, with a steeper learning curve.
3. PageSpeed Insights
Overview and Purpose: PageSpeed Insights is a free tool by Google that integrates Lighthouse metrics with real-world field data.
Key Features: Measures Core Web Vitals on both mobile and desktop, offering straightforward guidance and scoring for quick improvements.
Pros and Cons:
Pros: Simple, accessible, and integrates with Chrome DevTools via Lighthouse.
Cons: Limited customization options, focuses mainly on the front-end experience.
Optimization Strategies for Each Core Web Vital
Once we understand how to measure Core Web Vitals, the next step is to improve them. Below, we’ll cover specific techniques and code examples for optimizing each metric, enhancing loading performance, interactivity, and visual stability.
Optimization for Largest Contentful Paint (LCP)
A fast LCP is crucial because it allows users to quickly see meaningful content. Here are some strategies to improve LCP, along with code examples.
Defer Non-Critical JavaScript
Loading non-essential JavaScript only after the page has fully loaded can significantly improve LCP by prioritizing critical resources.
Code Example:
<script src="non-critical.js" defer></script>
Use Preloading for Critical Assets
Preloading assets like the main hero image or large font files helps the browser load these resources earlier, improving LCP by speeding up the display of main content.
Code Example:
<link rel="preload" href="hero-image.jpg" as="image">
Best Practices for LCP:
Optimize server response times with techniques like caching and CDNs (Content Delivery Networks).
Compress and optimize images to reduce file sizes.
Minimize CSS and JavaScript blocking resources that could delay the initial rendering of the page.
Optimization for First Input Delay (FID)
A low FID ensures users can interact with your site without delay. Here’s how to improve it:
Minimize JavaScript Execution Time
Reducing JavaScript load can prevent long tasks from blocking the main thread and improve FID by allowing the page to respond faster to user input.
Code Example:
<script src="large-script.js" async></script>
Code-Splitting Using Webpack
Code-splitting breaks down large bundles into smaller, manageable pieces, so the browser loads only what’s needed initially. This strategy can improve FID by reducing load times.
Code Example (Webpack):
// Webpack configuration for splitting optimization: { splitChunks: { chunks: 'all', }, }
Best Practices for FID:
Use web workers to offload heavy computations away from the main thread.
Reduce the number of third-party scripts, as they often contribute to increased latency.
Break down heavy JavaScript files and load them asynchronously to prevent blocking interactions.
Optimization for Cumulative Layout Shift (CLS)
A stable layout improves CLS by preventing unexpected movements. Here’s how to avoid content shifts with specific techniques:
Specify Dimensions for Images and Videos
By defining width and height for images, videos, and iframes, you can ensure that space is allocated for these elements before they load, reducing layout shifts.
Code Example:
<img src="banner.jpg" width="600" height="400" alt="Banner Image">
Avoid Inserting New Content Above Existing Content
Reserve space in the layout for content like ads or dynamically loaded elements to avoid shifts as the page loads.
Code Example:
.ad-slot { height: 200px; width: 300px; }
Best Practices for CLS:
Set static space for ad slots, banners, and other dynamic content.
Preload fonts to prevent sudden shifts due to late font loading.
Avoid sudden transitions or animations that can impact layout stability.
Troubleshooting Core Web Vitals with a Decision Tree
To streamline the troubleshooting process, here’s a decision tree that guides you in identifying and resolving issues based on each Core Web Vital metric.
Troubleshooting Decision Tree for Core Web Vitals
1. Largest Contentful Paint (LCP)
Issue: LCP over 2.5 seconds
Check Server Response Time: If the server response is slow, consider using a CDN and enabling caching.
Review Image Optimization: Ensure images are optimized, compressed, and delivered in appropriate formats.
Analyze CSS and JavaScript Loading: Identify and defer non-essential JavaScript and CSS.
2. First Input Delay (FID)
Issue: FID over 100 milliseconds
Review Main-Thread Blocking Tasks: If tasks take longer than 50 ms, consider breaking them down or offloading heavy computations to web workers.
Analyze Third-Party Scripts: Remove or defer third-party scripts if they’re blocking the main thread.
Check JavaScript Execution Time: Reduce JavaScript execution time through code-splitting and lazy loading.
3. Cumulative Layout Shift (CLS)
Issue: CLS over 0.1
Review Image and Media Dimensions: Ensure dimensions are specified for all media elements to prevent layout shifts.
Analyze Font Loading: Use
font-display: swap
to reduce layout shifts due to delayed font rendering.Verify Layout Stability: Avoid inserting new content at the top of the page during load, and define static spaces for ads or dynamic elements.
Conclusion
Optimizing Core Web Vitals is crucial for providing a fast, interactive, and visually stable experience. With the help of tools like Lighthouse, WebPageTest, and PageSpeed Insights, you can identify bottlenecks in LCP, FID, and CLS. By implementing the optimization strategies discussed, you’ll see improvements not only in performance metrics but also in user engagement, retention, and overall satisfaction.
Remember, web performance optimization is an ongoing process. Regularly testing and fine-tuning your site ensures it consistently meets Core Web Vitals thresholds, delivering an excellent experience for every visitor.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
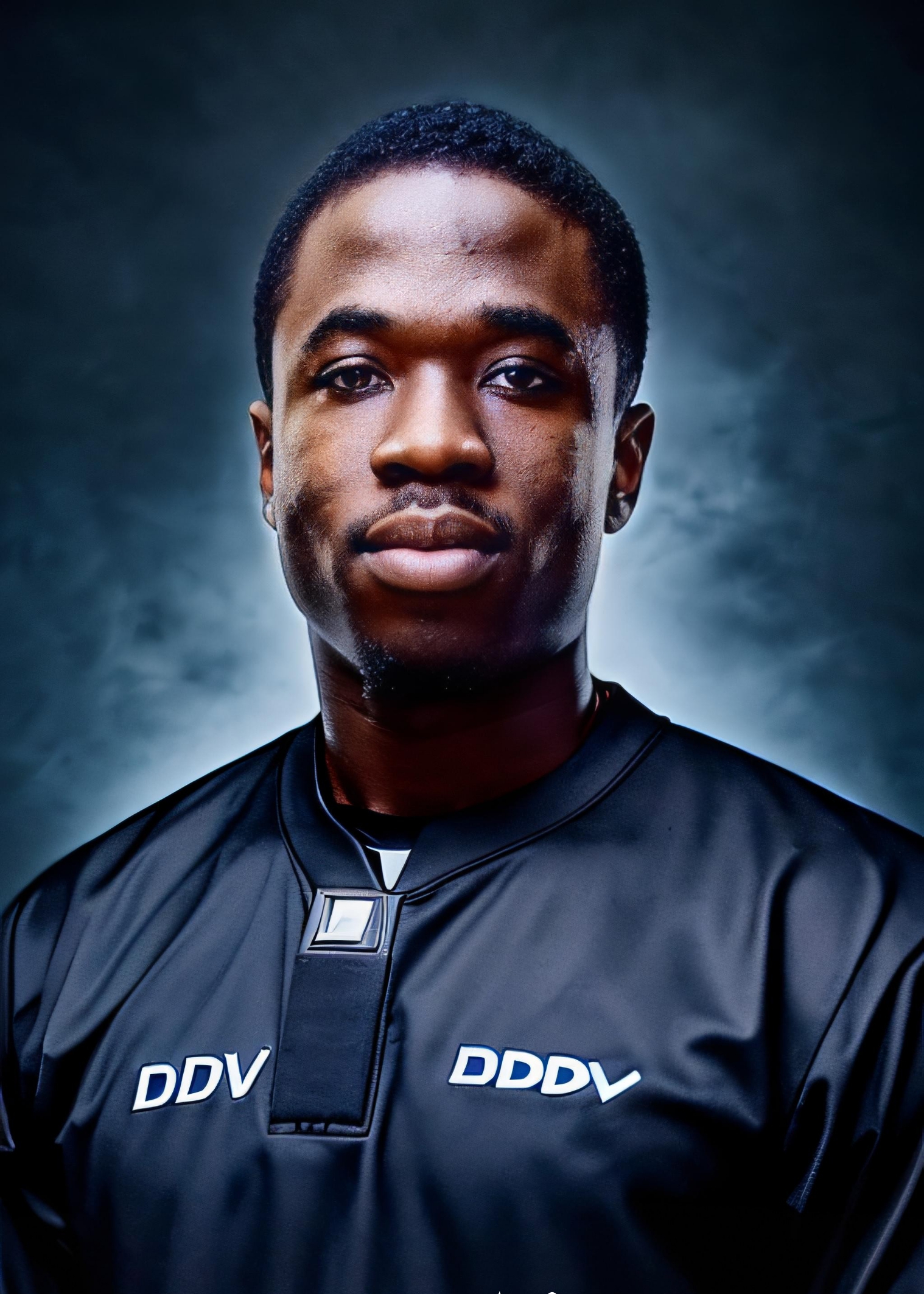
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.