Building and Testing a Node.js API with MongoDB Atlas and Postman
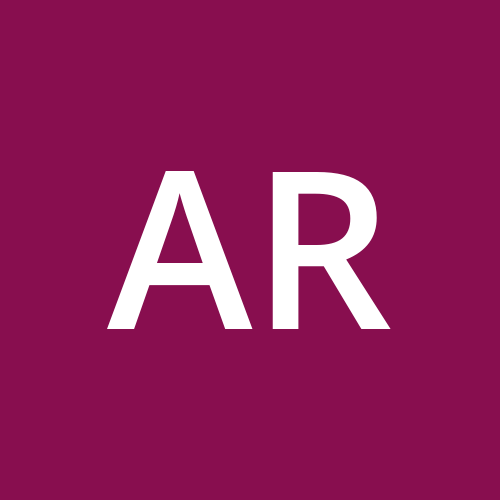
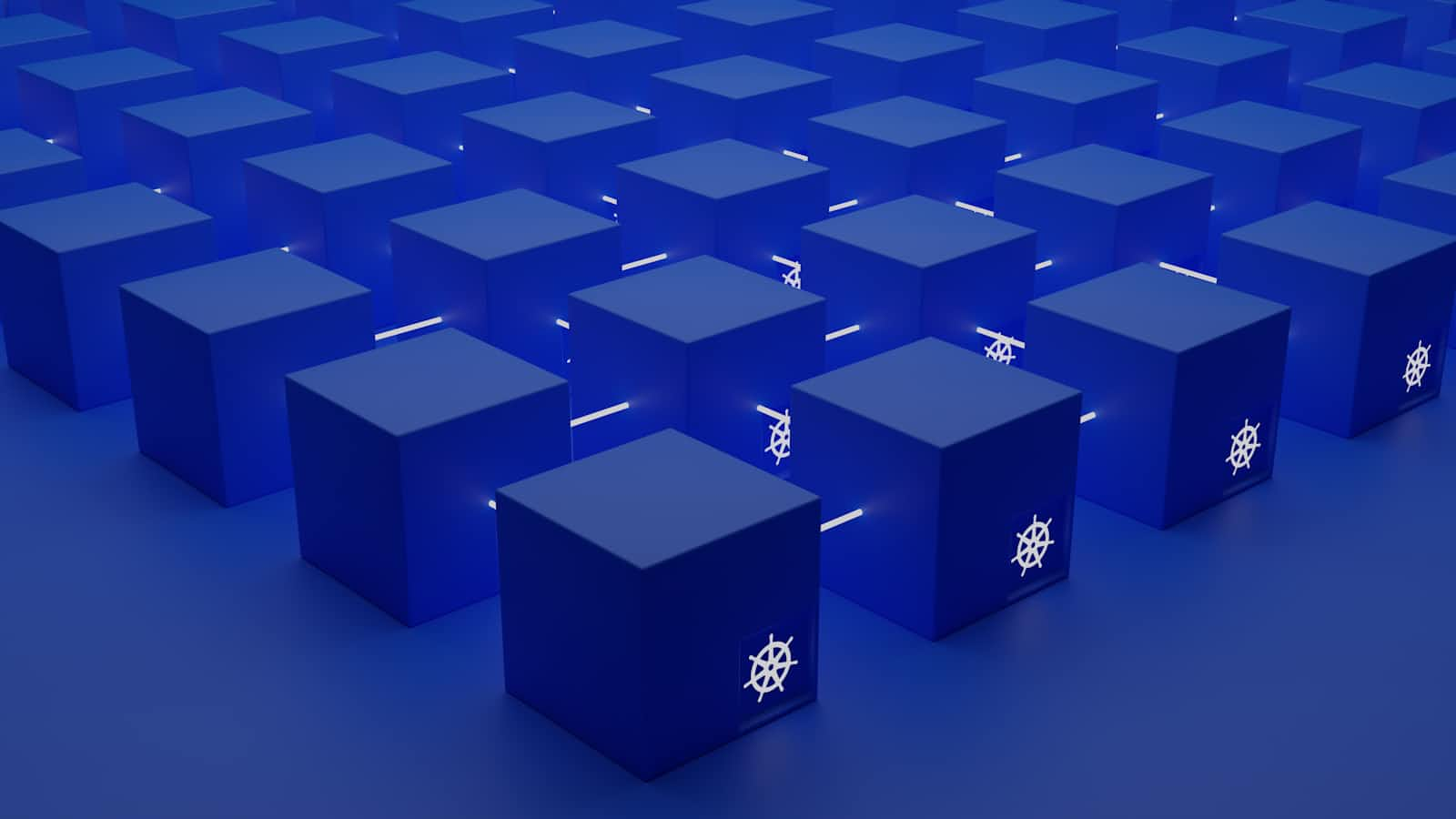
Introduction
Hey, have you ever worked with Node.js and needed a reliable place to store data? Sometimes, you want a quick, easy way to save and retrieve information without dealing with complicated databases. That’s where MongoDB Atlas comes in—a fully managed cloud version of MongoDB that works perfectly with JavaScript and Node.js, saving you the hassle of installing and managing MongoDB on your local machine.
The cool thing about MongoDB is that it lets you store data as JSON-like objects, making it super flexible and easy to work with. And to make it even simpler, we can use Mongoose—a powerful library that acts as a bridge between our Node.js app and MongoDB. Mongoose takes care of the database connection and lets us interact with MongoDB easily using JavaScript.
In this post, I’ll walk you through how to connect a Node.js app to MongoDB Atlas using Mongoose. We’ll set up the connection and cover some basic CRUD operations, so by the end, you’ll be ready to add MongoDB to your own projects.
Prerequisites
Before we jump into the code, let me make sure we’re on the same page with a few things. To follow along, here’s what you need:
Node.js installed on your computer. If you don’t have it yet, no worries! You can grab it from the official site here. Just install it, and you're good to go.
MongoDB Atlas account: MongoDB Atlas is the cloud version of MongoDB, and it’s free to use at a basic level. We’ll set up a cluster and get a connection string shortly. You won’t need to install MongoDB on your computer since we’ll be using MongoDB Atlas, a cloud-based version of MongoDB.
Basic JavaScript knowledge. Since we’re dealing with Node.js, it's helpful to know your way around variables, functions, and how to run JavaScript in the terminal.
And finally, a terminal or command line. We’ll be running commands and scripts, so being comfortable using the terminal will make things easier.
That's it! Once you have those ready, we can start building. Trust me, it's going to be a smooth ride.
Setting Up the Project
Alright, now that we’ve got the basics covered, let’s dive into setting up our project. We’ll create a simple Node.js app that connects to MongoDB using Mongoose. Don’t worry, I’ll walk you through each step, and it’s easier than it sounds.
Step 1: Create a New Project
First things first, let’s create a folder for our project. You can name it whatever you like, but let’s keep it simple. Open your terminal (or command line) and run this:
mkdir mongo-demo
cd mongo-demo
This creates a new directory called mongo-demo
and navigates inside it. Easy, right?
Step 2: Initialize a New Node.js Project
Next, we need to initialize the project with Node.js. This will create a package.json
file to track our dependencies (like Mongoose). Just run:
npm init -y
That -y
flag just skips the questions and uses default values. It’s like hitting “yes” to everything. You should now see a package.json
file in your folder.
Step 3: Install Dependencies
Now we need to install some packages that we’ll be using. We’ll need:
express for setting up a basic server,
mongoose to interact with MongoDB, and
dotenv to manage environment variables (like our MongoDB connection string).
Go ahead and install them by running this command:
npm install express mongoose dotenv
Boom! You’ve got all the tools we need. 🎉
Step 4: Create a Basic App File
Let’s create our main file, where all the magic will happen. In the mongo-demo
folder, create a new file called app.js
:
touch app.js
Setting Up MongoDB Atlas
Create a MongoDB Atlas Cluster
Sign up for MongoDB Atlas: Head over to MongoDB Atlas and create a free account if you don’t have one already.
Create a new project: In your Atlas dashboard, create a new project, and then click on Build a Cluster.
Choose a free cluster: Select the Free Tier option, choose your cloud provider, and pick a region close to you.
Configure Access:
Add a new database user and set a username and password (make sure to remember these).
Go to Network Access and whitelist your current IP address or allow access from all IP addresses if you’re testing from multiple locations.
Get the connection string: Once your cluster is set up, click Connect, choose Connect your application, select the latest version of Node.js, and copy the connection string provided.
Store Your MongoDB URI in a .env
File
Create a new file called .env
in the root of your project and add your MongoDB URI. Replace <username>
and <password>
with the credentials you created in MongoDB Atlas, <cluster-url>
with the generated cluster URL, and <database-name>
with your chosen database name (e.g., myDatabase
). This connection string tells Mongoose where to find your MongoDB instance on Atlas.
MONGO_URI=mongodb+srv://<username>:<password>@your-cluster-url.mongodb.net/<database-name>?retryWrites=true&w=majority
Now, let’s write some basic code in app.js
to set up our server and connect to MongoDB. Copy this into your app.js
file:
const express = require('express');
const mongoose = require('mongoose');
const dotenv = require('dotenv');
dotenv.config();
const app = express();
const port = 3000;
// Connect to MongoDB Atlas
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => console.log('Connected to MongoDB Atlas...'))
.catch(err => console.error('Could not connect to MongoDB Atlas...', err));
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Let me break this down for you:
We’re using
express
to create a simple server.mongoose
is what we’ll use to connect to MongoDB.dotenv
is used to load our environment variables (like the MongoDB URI).
Finally, let’s fire up the server and make sure everything is working. In your terminal, run:
node app.js
If everything is set up correctly, you should see:
Connected to MongoDB Atlas...
Server is running on port 3000
And that’s it! 🎉 We’ve just set up a basic Node.js project and connected it to MongoDB using Mongoose. Pretty smooth, right? Let’s move on to creating some data!
Creating a Basic Schema and Model
Alright, now that we’ve got the server up and running, it’s time to dive into the fun part— creating data. MongoDB is a NoSQL database, which means it stores data in a more flexible, JSON-like format. But with Mongoose, we can define clear schemas for our data to keep things structured.
So, let’s create a schema and model for something simple, like a User.
What is a Schema?
A schema is basically a blueprint for how your data should look. It defines the structure of the documents in a MongoDB collection. In our case, we’ll create a User
schema, where each user will have properties like name
, email
, and age
.
Step 1: Creating the User Model
In your project, create a folder called
models
. Inside that folder, create a file calledUser.js
. This is where we’ll define our schema.Now, let’s create the user schema inside
User.js
. Add this code:
const mongoose = require('mongoose');
// Define the User schema
const userSchema = new mongoose.Schema({
name: {
type: String,
required: true
},
email: {
type: String,
required: true,
unique: true
},
age: {
type: Number,
default: 18
}
});
// Create the User model
const User = mongoose.model('User', userSchema);
module.exports = User;
What’s Happening Here?
We’re using Mongoose to define our
User
schema. Each user will have three fields:name
(a required string),email
(a required and unique string), andage
(a number with a default value of 18).
The schema defines the structure, and the model is how we interact with the database. The
User
model will allow us to create, read, update, and delete users from the MongoDB collection.
Step 2: Using the User Model
Now that we’ve created our User
model, let’s put it to use. We’ll write some simple routes to create and fetch users from the database.
Go back to your app.js
file and update it like this:
const express = require('express');
const mongoose = require('mongoose');
const dotenv = require('dotenv');
const User = require('./models/User'); // Import the User model
dotenv.config();
const app = express();
const port = 3000;
app.use(express.json()); // Middleware to parse JSON requests
// Connect to MongoDB
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => console.log('Connected to MongoDB Atlas...'))
.catch(err => console.error('Could not connect to MongoDB Atlas...', err));
// POST: Create a new user
app.post('/users', async (req, res) => {
try {
const user = new User(req.body);
await user.save();
res.status(201).send(user);
} catch (err) {
res.status(400).send(err);
}
});
// GET: Fetch all users
app.get('/users', async (req, res) => {
try {
const users = await User.find();
res.send(users);
} catch (err) {
res.status(500).send(err);
}
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
What’s Happening Now?
We’ve added two routes to our app:
POST
/users
: This allows us to create a new user by sending user data in the request body.GET
/users
: This retrieves all users from the MongoDB collection.
We’re using
express.json()
middleware to automatically parse incoming JSON requests.The User model is used in both routes to interact with the MongoDB collection. In the POST route, we create and save a new user, and in the GET route, we retrieve all users from the collection.
You can also check if the operation was successful by going to MongoDB Atlas → Clusters → Browse Collections. On the left side, you should see your database name, and when you click on it, you should see your collections. Currently, there is only one collection called users
.
In MongoDB, collections are similar to tables in relational databases. When you create a Mongoose model without specifying a collection name, Mongoose automatically generates a collection name based on the model name by converting it to lowercase and pluralizing it. This is exactly what happened to the User
model in the ./models/User
file.
Basic CRUD Operations
Alright, now that we’ve created a user and fetched all users from the database, let's complete the picture by adding the remaining CRUD operations. In case you’re not familiar, CRUD stands for Create, Read, Update, and Delete—basically the four essential operations you’ll be using with databases.
We’ve already covered Create and Read, so now let’s dive into Update and Delete.
Update a User
Imagine you’ve created a user but now want to update their info—maybe they changed their email or you got their age wrong. We can handle this using a PUT request. Let’s add a PUT route in your app.js
to update a user by their ID:
// PUT: Update a user by ID
app.put('/users/:id', async (req, res) => {
try {
const user = await User.findByIdAndUpdate(req.params.id, req.body, { new: true, runValidators: true });
if (!user) {
return res.status(404).send('User not found');
}
res.send(user);
} catch (err) {
res.status(400).send(err);
}
});
Breaking it Down:
findByIdAndUpdate: Mongoose’s method to find a user by their unique ID and update their info.
We’re passing
{ new: true }
so that Mongoose returns the updated document (by default, it returns the original).{ runValidators: true }
ensures that Mongoose rechecks the schema's validation rules before saving the updates.If the user isn’t found, we return a
404
status code.
Delete a User
Next, let’s say you need to remove a user from the database. We can do this using a DELETE request. Add a DELETE route in app.js
:
// DELETE: Delete a user by ID
app.delete('/users/:id', async (req, res) => {
try {
const user = await User.findByIdAndDelete(req.params.id);
if (!user) {
return res.status(404).send('User not found');
}
res.send('User deleted');
} catch (err) {
res.status(500).send(err);
}
});
Breaking it Down:
findByIdAndDelete: Mongoose method to find a user by their ID and delete them from the database.
If the user isn’t found, we return a
404
response.If the deletion is successful, we send a simple "User deleted" message.
Full CRUD Example Recap:
Here’s a quick summary of all the routes we’ve created so far:
Create a User (POST):
/users
Fetch All Users (GET):
/users
Update a User (PUT):
/users/:id
Delete a User (DELETE):
/users/:id
And just like that, you've got a full CRUD API up and running! 🎉
Testing the API with Postman
Now that our API is fully set up, the next step is to test it and make sure everything works as expected. One of the easiest ways to test APIs is by using a tool called Postman. If you haven't used it before, Postman is a free, user-friendly tool that lets you make HTTP requests (GET, POST, PUT, DELETE) and see the responses from your API.
Step 1: Install Postman
If you don’t have Postman installed, go ahead and download it from the official website. Once you’ve got it set up, you’re ready to start testing!
Step 2: Testing Each Endpoint
Let’s walk through testing each endpoint we’ve created, using Postman to send requests and check the results.
Create a User (POST Request)
Endpoint:
http://localhost:3000/users
Method: POST
Body: Select
raw
andJSON
as the format, and input something like this:
{
"name": "John Doe",
"email": "john@example.com",
"age": 25
}
- Expected Response: You should get back a response with the user object you just created, including a unique
_id
:
{
"_id": "6123abc456def7890gh12345",
"name": "John Doe",
"email": "john@example.com",
"age": 25,
"__v": 0
}
Fetch All Users (GET Request)
Endpoint:
http://localhost:3000/users
Method: GET
Expected Response: You should get an array of all users in your MongoDB collection:
[
{
"_id": "6123abc456def7890gh12345",
"name": "John Doe",
"email": "john@example.com",
"age": 25,
"__v": 0
}
]
Update a User (PUT Request)
Endpoint:
http://localhost:3000/users/:id
(Replace:id
with the user's actual_id
, e.g.,http://localhost:3000/users/6123abc456def7890gh12345
)Method: PUT
Body: Update the user's information in the JSON format, like this:
{
"name": "Jane Doe",
"email": "jane@example.com",
"age": 30
}
- Expected Response: You should get back the updated user object:
{
"_id": "6123abc456def7890gh12345",
"name": "Jane Doe",
"email": "jane@example.com",
"age": 30,
"__v": 0
}
Delete a User (DELETE Request)
Endpoint:
http://localhost:3000/users/:id
(Again, replace:id
with the actual user ID).Method: DELETE
Expected Response: You should get a message confirming the user was deleted, such as:
"User deleted"
Step 3: Debugging with Postman
If something doesn’t seem to work, don’t worry - Postman makes it easy to debug:
Response Codes: Pay attention to HTTP status codes in Postman (like 200, 400, or 500). If you get a
400 Bad Request
or500 Internal Server Error
, it might be due to incorrect input or issues with the server.Error Messages: Check the response body for any error messages that can help you track down the problem.
Conclusion
In this guide, we’ve taken a step-by-step approach to build a simple Node.js application connected to MongoDB Atlas using Mongoose. Along the way, we covered:
Setting up a Node.js project and establishing a connection to MongoDB Atlas.
Defining a schema and creating a model for structured data.
Performing CRUD operations (Create, Read, Update, Delete) on user data.
Testing our API with Postman to ensure everything works as expected.
With this foundation, you're now equipped to expand this project in many ways. You can add more complex data models, implement user authentication (like JWT tokens), or even deploy your app to a service like Heroku or Vercel. Since MongoDB Atlas is cloud-based, deploying your Node.js app is simpler as the database is already hosted and managed separately.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
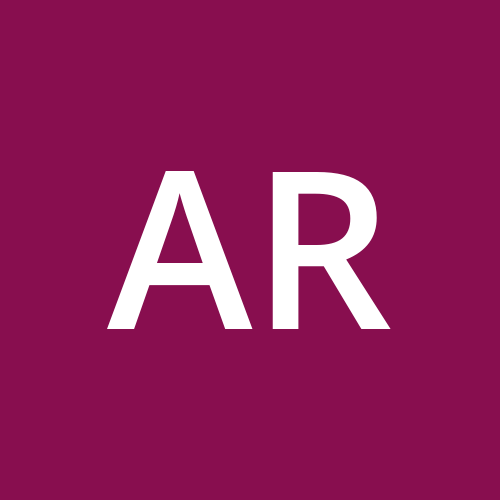