How JavaScript Handles Concurrent Requests Despite Being Single-Threaded: The Magic Behind the Scenes

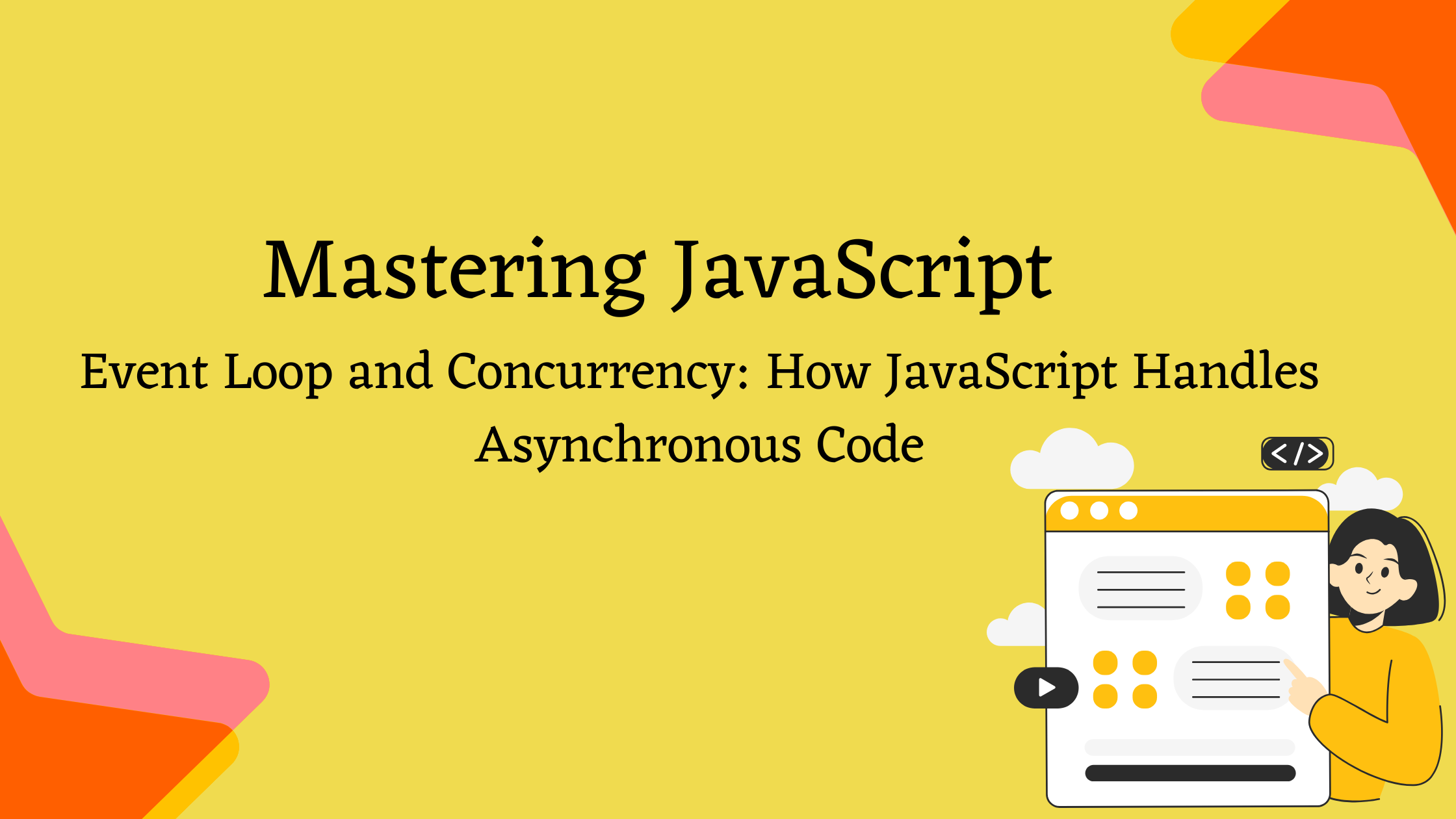
Introduction
JavaScript is known as a single-threaded language, meaning it can only execute one task at a time. However, despite this limitation, JavaScript can handle multiple asynchronous operations efficiently, such as fetching data from an API or managing millions of requests on a server. How does it achieve this? The secret lies in the Event Loop and Concurrency model.
In this article, we’ll break down what the event loop is, how JavaScript handles asynchronous code, and why it matters, especially in production environments like Node.js, where millions of requests need to be managed without slowing down.
Where Does the Event Loop Come From?
JavaScript’s concurrency model is based on the event loop, which originates from the language’s design to be non-blocking. When JavaScript was first developed to run in browsers, it was meant to handle tasks like user interactions, network requests, and rendering the page without freezing or slowing down.
Single-threaded execution means that JavaScript can only perform one operation at a time. But real-world applications need to do many things concurrently, like loading data from a server while still allowing the user to interact with the page. This is where the event loop and asynchronous operations come in.
Is It Possible to Write Asynchronous Code in JavaScript? How?
Yes, you can write asynchronous code in JavaScript, and there are several ways to do it:
Callbacks: The most basic form of handling asynchronous operations. A callback is a function passed to another function, which gets executed after an operation is completed.
Example:
setTimeout(() => { console.log("This runs after 2 seconds"); }, 2000);
Promises: Promises provide a cleaner way to handle asynchronous code. Instead of passing callbacks, a promise returns a result (either resolved or rejected) that you can chain with
.then()
and.catch()
.Example:
fetch("https://api.example.com/data") .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
Async/Await: Introduced in ES6,
async
andawait
syntax allows you to write asynchronous code that looks and behaves like synchronous code, making it easier to read and manage.Example:
async function fetchData() { try { const response = await fetch("https://api.example.com/data"); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } } fetchData();
How Does Node.js Handle Millions of Requests Despite Being Single-Threaded?
Node.js, built on Chrome’s V8 JavaScript engine, extends JavaScript’s event-driven model to server-side applications. Despite being single-threaded, Node.js can handle millions of requests efficiently. This is thanks to a few key features:
Non-blocking I/O operations: In Node.js, most I/O operations (like reading a file or querying a database) are non-blocking. This means Node.js can initiate these operations and continue executing other code without waiting for the I/O operation to finish.
The Event Loop: The event loop in Node.js plays a vital role in managing asynchronous operations. Here's a simplified breakdown of how it works:
When an asynchronous operation is initiated (like a network request), it is offloaded to the system’s kernel or a worker thread.
Node.js then continues executing other tasks while the asynchronous operation is in progress.
Once the operation is complete, the result is placed in the callback queue.
The event loop checks if the call stack is empty, and if so, it processes the callback queue, executing the associated callbacks or promises.
This non-blocking behavior allows Node.js to handle numerous requests at once, even though it’s single-threaded.
- Thread Pool (libuv): While JavaScript itself is single-threaded, Node.js uses a library called libuv to manage a thread pool for tasks that cannot be offloaded to the kernel (like file system operations). These tasks are executed in the background, and their results are returned to the event loop when complete.
Production Use Case: Handling Millions of Requests
Let’s consider a production use case: You’re running a server in Node.js that handles millions of concurrent requests, such as an API for an e-commerce platform during a sale. How does Node.js manage this massive load?
Non-blocking Operations: Requests that involve I/O (such as reading from a database, sending an email, or logging a user in) are initiated and then offloaded to either the kernel or the thread pool. This allows Node.js to continue accepting new requests without being blocked by ongoing I/O operations.
Efficient Resource Usage: Because of Node.js’s non-blocking nature, the system resources (like CPU and memory) are used efficiently. Instead of allocating one thread per request, Node.js uses a single thread to handle multiple requests concurrently.
Scaling: In production environments, Node.js applications can scale both horizontally and vertically. For instance, you can run multiple instances of your Node.js server using tools like PM2 or Node Cluster to take advantage of multi-core processors. This further enhances Node.js’s ability to handle millions of requests.
Real-Time Applications: For applications like chat systems or live notifications, Node.js is highly suited due to its event-driven architecture. The event loop ensures that real-time updates are pushed to users without delay, even under heavy load.
Conclusion
JavaScript’s event loop and concurrency model may seem limiting at first glance, but they provide an elegant solution to handling asynchronous operations efficiently. Understanding how the event loop works and how Node.js manages millions of requests on a single thread helps developers write scalable, performant applications.
By embracing non-blocking operations, using async/await, and leveraging the power of the event loop, you can build applications that handle large numbers of concurrent users without breaking a sweat.
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
An experienced professional with 5.5+ years in the industry, adept at collaborating effectively with developers across various domains to ensure timely and successful project deliveries. Proficient in Android/Flutter development and currently excelling as a backend developer specializing in Node.js. I bring a strong enthusiasm for learning new frameworks, paired with a quick-learning mindset and a passion for writing bug-free, optimized code. I am always ready to adapt to and learn cloud technologies, ensuring continuous growth and improvement. I actively contribute to communities by writing insightful articles on my blog and am seeking support from you all to create more valuable content and tutorials like this.