Understanding Time in Go

Table of contents
- The Basics:
- Adjusting the Time Zone: Using the TZ Environment Variable
- Formatting Time in Go:
- Working with Time Zones Programmatically
- Manipulating Time: Adding and Subtracting Durations
- Calculating Time Differences: Using time.Since() and time.Until()
- Getting More from Time.Now()
- Time Zones: Going Beyond LoadLocation
- Working with Time Durations: More than just time.Second
- Much more: Timer and Ticker
- Time Zones: Handling DST and Leap Seconds
- Optimizing for Performance: Benchmarking with time
- Best Practices for Working with Time in Go
- 1. Avoid Frequent Calls to time.Now() in Performance-Critical Code
- 2. Be Mindful of Time Zones and Location
- 3. Use Round() and Truncate() for consistent Time Calculations
- 4. Use time.Duration Constants for better readability
- 5. Handle Time Parsing and Formatting with care
- 6. Consider Ticker and Timer for Scheduled Tasks
- 7. Ensure using Location when parsing Historical Dates
- 8. Avoid Using Zero Values of time.Time for Meaningful Checks
- 9. Avoid Time Arithmetic with time.Time Directly; Use Durations
- Wrapping Up
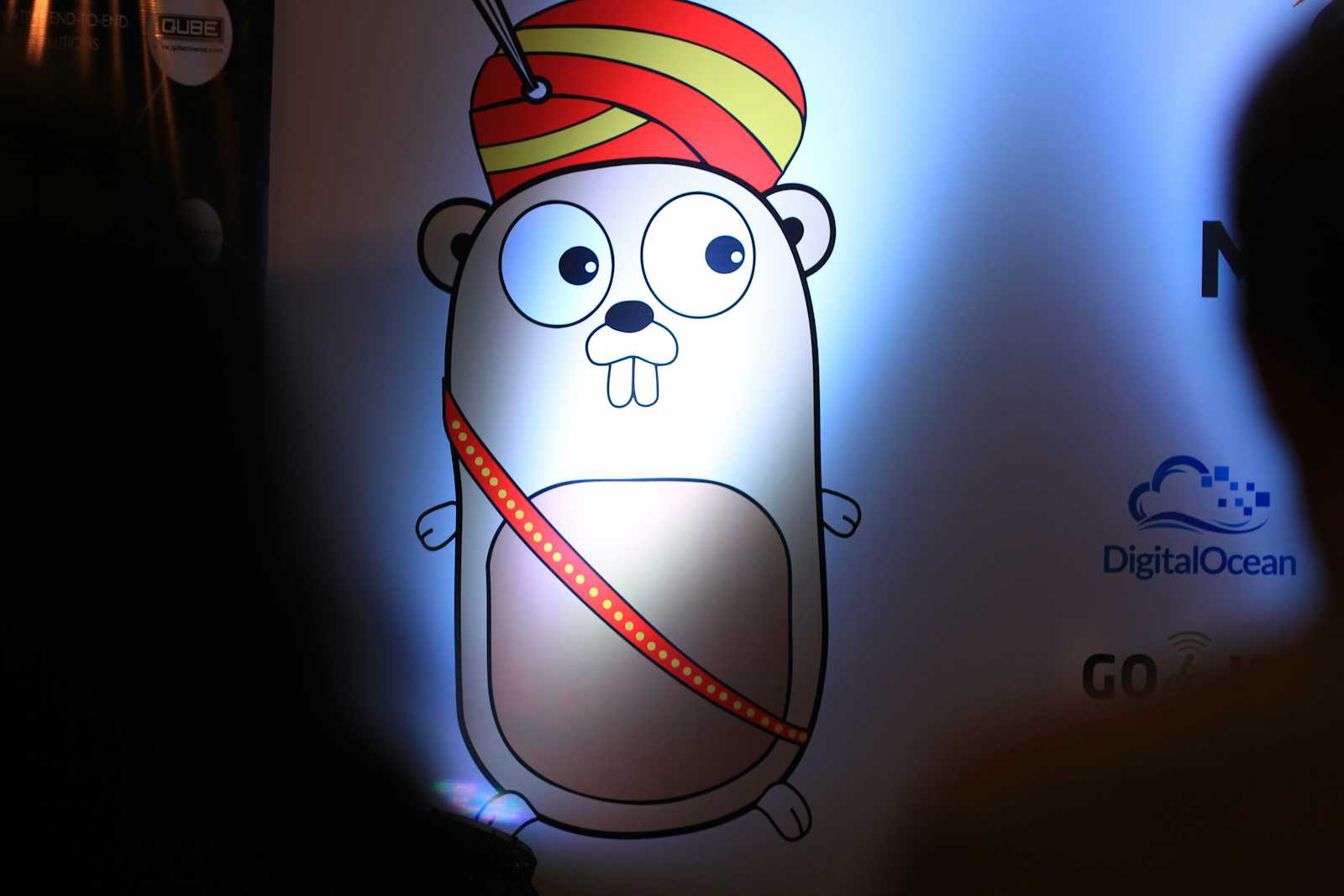
Working with time
in Go may be bit tricky at first, especially when dealing with different time zones, formatting, or time manipulation. In this blog, I'll try to cover most of the ins and outs of using Go’s time
package, focusing on practical ways to handle time.Time
, time.Now()
, and more advanced tasks like working with time zones and formatting and best practices in managing Time.
The Basics:
In Go, if you want to get the current time, time.Now()
is the straightforward way to go. It returns a time.Time
object that represents the current local time according to your system settings. Here’s a simple example:
package main
import (
"fmt"
"time"
)
func main() {
currentTime := time.Now()
fmt.Println("Current time:", currentTime)
}
When you run this code, the output might look something like:
Current time: 2024-10-28 06:49:16.59797 +0530 IST m=+0.000111210
This result shows the date, the time with fractional seconds, and the time zone offset from UTC (+0530
), as well as the time zone abbreviation (IST
for Indian Standard Time, in this example). Depending on your local settings, this will reflect the appropriate time zone.
Adjusting the Time Zone: Using the TZ
Environment Variable
If you need to test your code in different time zones, there's a quick way to do it using the TZ
environment variable in the terminal. This can be helpful when you need to simulate different local times without changing any code. For example, to set the time zone to "Asia/Kolkata," you can do the following:
TZ="Asia/Kolkata" go run main.go
With this setting, time.Now()
will reflect the current time in Kolkata. This can save time when you’re testing time zone-dependent features, as it allows you to see how your code behaves across different time zones without changing your machine's actual settings.
Formatting Time in Go:
Go’s time formatting might seem strange at first—why use this seemingly random date, Mon Jan 2 15:04:05 MST 2006
, as the reference for formatting? The choice is actually quite deliberate and serves a practical purpose.
This reference date is memorable because each number represents a specific time component, thats sequential. Subtly funny, isn’t it? Hehe.
01
represents the month (January)02
represents the day03
,04
, and05
represent the time in 24-hour format (03:04:05)2006
represents the yearMST
stands for Mountain Standard Time, giving a time zone example, that’s7
hours backwards from UTC.
The idea is that developers can use this reference date to build custom time formats by substituting these components with their desired layout. Instead of memorizing arbitrary codes like "yyyy-MM-dd," you just match the component to the position in this reference. It may seem unconventional, but it’s consistent with Go's approach to keeping things simple and explicit.
For instance, to format the current time as "YYYY-MM-DD HH:MM:SS," you would write:
fmt.Println(currentTime.Format("2006-01-02 15:04:05 MST"))
The output might be:
2022-11-06 14:32:58 IST
The formatting style may seem unconventional at first, but it becomes straightforward once you get used to it.
Working with Time Zones Programmatically
While setting the TZ
environment variable is useful for testing, you may need to work with different time zones in your code. This can be done with time.LoadLocation()
and Time.In()
to set a specific time zone:
loc, err := time.LoadLocation("America/New_York")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
newYorkTime := time.Now().In(loc)
fmt.Println("New York Time:", newYorkTime)
Here, LoadLocation
fetches the time zone information for "America/New_York," and Time.In()
converts the current time to that time zone. This approach is useful for applications where users in different regions need to see the local time for their location.
Manipulating Time: Adding and Subtracting Durations
In Go, time.Time
objects are immutable, which means you can’t modify them directly. To add or subtract time, you create a new instance using methods like Add()
:
oneHourLater := time.Now().Add(time.Hour)
fmt.Println("One hour later:", oneHourLater)
You can subtract time by adding a negative duration:
oneHourAgo := time.Now().Add(-time.Hour)
fmt.Println("One hour ago:", oneHourAgo)
Go supports various durations (time.Minute
, time.Second
, etc.), making it convenient to manipulate time accurately.
Calculating Time Differences: Using time.Since()
and time.Until()
To determine the difference between two times, you can use time.Since()
for elapsed time or time.Until()
for future events. Both return a time.Duration
that can be expressed in hours, minutes, or seconds.
start := time.Now()
// some process...
duration := time.Since(start)
fmt.Println("Duration taken:", duration)
And for counting down to an event:
eventTime := time.Date(2022, time.December, 25, 0, 0, 0, 0, time.Local)
timeLeft := time.Until(eventTime)
fmt.Println("Time until Christmas:", timeLeft)
Using time.Duration
helps make time calculations straightforward, whether you need precision to the second or are working with longer intervals.
Getting More from Time.Now()
We all know Time.Now()
gives the current time, but did you know you can access finer details about it? The time.Time
struct has several fields and methods that can be quite handy:
Nanosecond Precision: Go’s
time
package allows you to work with time at nanosecond precision. If you need high accuracy (think benchmarking or profiling), you can access the exact nanosecond part usingTime.Nanosecond()
:currentTime := time.Now() fmt.Println("Nanoseconds:", currentTime.Nanosecond())
This can be useful for tasks where timing accuracy is critical, such as performance testing.
Unix Timestamp Shortcuts: While
Unix()
andUnixNano()
are commonly used to get seconds and nanoseconds since the Unix epoch, there's alsoTime.Round()
andTime.Truncate()
that help round off or truncate a time to a specific unit. For example, if you want to get the nearest minute:roundedTime := time.Now().Round(time.Minute) fmt.Println("Rounded to the nearest minute:", roundedTime)
These methods are handy for applications like logging, where timestamps need to be uniform.
Time Zones: Going Beyond LoadLocation
We’ve seen how to use time.LoadLocation()
to change the time zone programmatically. But there are more nuanced ways to handle time zones and locations in Go:
Fixed Zones for Specific Offsets: If you need to work with a fixed offset from UTC (regardless of daylight saving time), you can create a
FixedZone
:fixedZone := time.FixedZone("IST", 5.5*60*60) // 5.5 hours offset from UTC fixedTime := time.Now().In(fixedZone) fmt.Println("Fixed Zone Time:", fixedTime)
This can be useful for scenarios like dealing with historical data, where time zones need to be consistent even if they used to observe daylight saving time.
Parsing Time with Specific Time Zones: When parsing time from a string, you can explicitly specify the location:
loc, _ := time.LoadLocation("Asia/Kolkata") timeInKolkata, err := time.ParseInLocation("2006-01-02 15:04", "2024-10-28 14:00", loc) if err != nil { fmt.Println("Error parsing time:", err) return } fmt.Println("Parsed Time in Kolkata:", timeInKolkata)
Using
ParseInLocation
makes sure that the parsed time takes into account the specified time zone, which can be crucial for applications where accurate time interpretation is needed across regions.
Working with Time Durations: More than just time.Second
Most Go developers know about basic durations like time.Second
or time.Minute
. But there’s more you can do with durations that can make your code cleaner and more expressive:
Creating Custom Durations: Go allows you to define durations directly using time units. For instance:
fiveAndHalfHours := 5*time.Hour + 30*time.Minute fmt.Println("Five and a half hours:", fiveAndHalfHours)
This syntax is great for complex time calculations, allowing you to avoid having to do conversions manually.
Sleep with Precision: While
time.Sleep()
is typically used with whole durations liketime.Second
, it can also accept finer durations:time.Sleep(100 * time.Millisecond) fmt.Println("Slept for 100 milliseconds")
This can be used for tasks like rate-limiting API requests or implementing retry logic with exponential backoff.
Much more: Timer
and Ticker
When you need to perform tasks at regular intervals, time.Ticker
and time.Timer
can come in handy:
Ticker
: Repeated Actions: ATicker
can trigger events at regular intervals. This is useful for cases like refreshing a dashboard or polling a service:ticker := time.NewTicker(2 * time.Second) go func() { for t := range ticker.C { fmt.Println("Tick at:", t) } }() time.Sleep(10 * time.Second) ticker.Stop() fmt.Println("Ticker stopped")
In the above example, we stop the ticker after 10 seconds to prevent it from running indefinitely.
Timer
: One-Off Delays: Unlike aTicker
, aTimer
triggers a single event after a specified delay. It’s useful for setting timeouts:timer := time.NewTimer(5 * time.Second) <-timer.C fmt.Println("Timer expired after 5 seconds")
You can also reset a timer if you need to extend the duration before it expires.
Time Zones: Handling DST and Leap Seconds
Most developers don’t often deal with daylight saving time (DST) or leap seconds directly, but there are cases where it can be important:
Automatic DST Handling: Go automatically adjusts for DST based on the system time zone database. If you’re working with locations that observe DST,
time.LoadLocation()
will adjust times accordingly.Handling Leap Seconds: While leap seconds are rare, they do exist. Go’s
time
package accommodates leap seconds in itstime.Parse()
andtime.Date
()
methods, though they are normalized to the nearest second. If you’re working in domains like astronomy or GPS systems, you might need to keep this in mind.
Optimizing for Performance: Benchmarking with time
Lastly, if you're into performance tuning, Go's time
package has some useful tools:
Measuring Code Execution Time: The
time.Since()
function is a straightforward way to measure how long a block of code takes to execute:start := time.Now() // some code you want to benchmark duration := time.Since(start) fmt.Println("Execution time:", duration)
This is a basic form of benchmarking and can be expanded into more sophisticated performance monitoring if needed.
Best Practices for Working with Time in Go
Handling time can be complex, but following some best practices can help you avoid common pitfalls and make your code more robust. Here are some key guidelines to keep in mind when using Go's time
package.
1. Avoid Frequent Calls to time.Now()
in Performance-Critical Code
While time.Now()
is straightforward to use, it involves a system call to fetch the current time, which can be relatively expensive when called frequently. In performance-sensitive code, such as loops or hot code paths, it’s better to compute the current time once and reuse the value.
Instead of:
for i := 0; i < 1000; i++ {
fmt.Println("Current time:", time.Now())
}
Do this:
now := time.Now()
for i := 0; i < 1000; i++ {
fmt.Println("Current time:", now)
}
By caching the time value, you reduce the overhead of repeatedly querying the system clock, making your code more efficient.
2. Be Mindful of Time Zones and Location
When dealing with different time zones, always make sure to set the Location
explicitly if your application is not purely local. The default time.Now()
uses the local system time zone, but you can convert a time to a specific location using Time.In()
.
Example:
loc, err := time.LoadLocation("UTC")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
utcTime := time.Now().In(loc)
fmt.Println("Current time in UTC:", utcTime)
Explicitly setting the Location
helps avoid ambiguity, especially when dealing with timestamps that may need to be converted or displayed across different regions.
3. Use Round()
and Truncate()
for consistent Time Calculations
If you need to align times to a specific unit, such as rounding to the nearest minute or truncating to the start of the hour, use Round()
or Truncate()
. These functions make time calculations more predictable and can help avoid inconsistencies caused by fractional seconds.
Example:
roundedTime := time.Now().Round(time.Minute)
fmt.Println("Rounded to the nearest minute:", roundedTime)
truncatedTime := time.Now().Truncate(time.Hour)
fmt.Println("Truncated to the start of the hour:", truncatedTime)
Using these methods ensures that time values are aligned to a consistent boundary, which can be useful when comparing timestamps or scheduling tasks.
4. Use time.Duration
Constants for better readability
Instead of manually calculating the number of seconds, minutes, or hours for time durations, use the built-in constants like time.Second
, time.Minute
, and time.Hour
. This improves readability and helps avoid mistakes when specifying time intervals.
Instead of:
time.Sleep(5000 * time.Millisecond)
Do this:
time.Sleep(5 * time.Second)
Using the time.Duration
constants makes it clear what the intended duration is, which makes the code easier to understand and maintain.
5. Handle Time Parsing and Formatting with care
Repeating again, Go's time parsing and formatting use a reference date (Mon Jan 2 15:04:05 MST 2006
). When working with different date and time formats, always use this reference to define your desired layout. It may seem unusual at first, but it’s consistent with Go's philosophy of keeping things explicit.
Example:
parsedTime, err := time.Parse("2006-01-02 15:04:05", "2024-10-28 14:32:05")
if err != nil {
fmt.Println("Error parsing time:", err)
return
}
fmt.Println("Parsed time:", parsedTime)
Familiarize yourself with the reference layout to avoid errors when parsing or formatting times, especially when working with various date formats.
6. Consider Ticker
and Timer
for Scheduled Tasks
Again, when you need to execute tasks at regular intervals or set timeouts, use time.Ticker
or time.Timer
. These are more reliable and easier to manage than using a loop with manual time calculations.
Ticker Example:
ticker := time.NewTicker(1 * time.Second)
defer ticker.Stop()
for range ticker.C {
fmt.Println("Tick at:", time.Now())
}
Tickers and timers help keep time-based operations precise without worrying about timing drift that could occur with manual calculations.
7. Ensure using Location
when parsing Historical Dates
The Location
type is more than just a time zone label; it also contains rules for calculating offsets, including daylight saving time adjustments. When parsing historical dates, always load the appropriate Location
to ensure accurate time calculations.
Example:
loc, err := time.LoadLocation("America/New_York")
if err != nil {
fmt.Println("Error loading location:", err)
return
}
historicTime, err := time.ParseInLocation("2006-01-02 15:04:05", "2015-03-08 02:30:00", loc)
if err != nil {
fmt.Println("Error parsing time:", err)
return
}
fmt.Println("Historical time in New York:", historicTime)
By using Location
when parsing dates, you account for historical time changes like daylight saving time, making your time calculations accurate.
8. Avoid Using Zero Values of time.Time
for Meaningful Checks
In Go, the zero value of time.Time
(time.Time{}
) represents the time "0001-01-01 00:00:00 UTC." While it can be used for checking if a time is uninitialized, avoid using it to signify meaningful dates, as it can cause confusion or lead to subtle bugs.
Instead, use explicit checks or pointers to indicate whether a time is set.
Example:
var t time.Time
if t.IsZero() {
fmt.Println("Time is not initialized")
}
Using IsZero()
provides a clear way to verify if a time value has been set, without relying on comparisons to magic values.
9. Avoid Time Arithmetic with time.Time
Directly; Use Durations
When working with time.Time
, avoid direct arithmetic. Instead, use methods like Add()
, Sub()
, and AddDate()
to modify time values safely.
Instead of:
newTime := time.Now() + 5*time.Hour // This wont work
Do this:
newTime := time.Now().Add(5 * time.Hour)
fmt.Println("New time:", newTime)
Using these methods ensures that calculations are type-safe and work consistently across different Go versions.
Wrapping Up
Thats it for now on Go's time.Time
package. Happy coding! Let Time be on your side. :)
Subscribe to my newsletter
Read articles from Ashwin Gopalsamy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ashwin Gopalsamy
Ashwin Gopalsamy
Product-first engineer, blogger and open-source contributor with around 4 years of experience in software development, cloud-native architecture and distributed systems. I build fintech products that process millions of transactions daily and drive substantial revenue. My expertise spans designing, architecting and deploying scalable software, focusing on the business under the code. I collaborate closely with engineers, product owners, and guilds, known for my clear communication and team-centric approach in dynamic environments. Colleagues appreciate my adaptability, openness and focus on diverse, meaningful contributions. Beyond coding, I’m recognized for my documentation, ownership and presentation skills, which drive clarity and engagement across teams. Bilingual in English and Deustch, I bridge cross-functional teams across geographies, ensuring smooth, efficient communication. I’m always open to new opportunities for connection and collaboration. Let’s connect and explore ways to create together.