How to Use React's Context API to Make State Management Easy

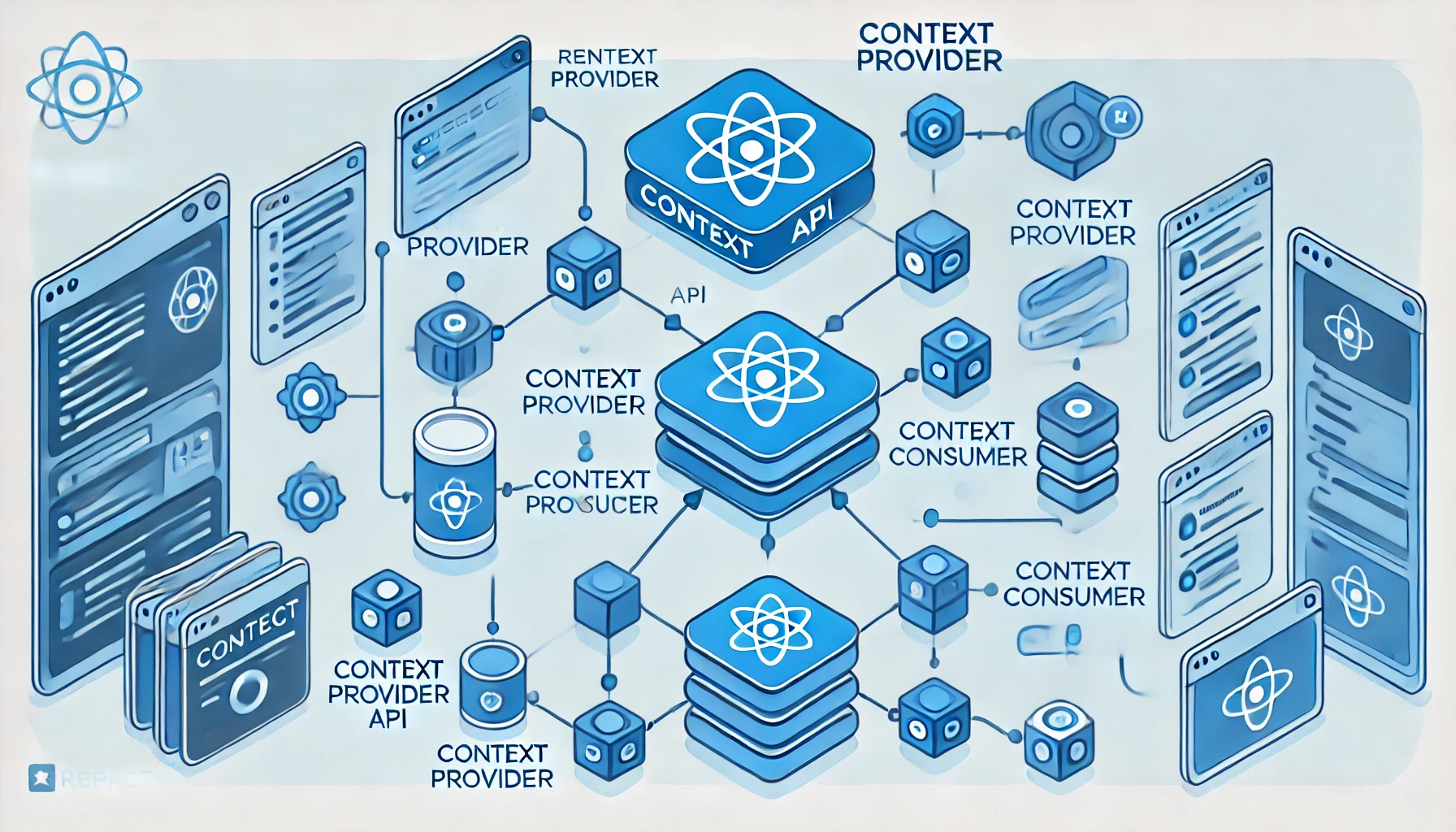
When developing React applications, managing data between components is one of the most common challenges. Typically, "props drilling" โ the process of passing props from a parent component down to deeply nested child components โ can make our code cumbersome and difficult to maintain. This is where React's Context API steps in, making our work easier by allowing us to share data across multiple components without the hassle of prop drilling.
What is React's Context API?
Introduced in React 16.3, the Context API is a tool for creating a centralized "context" that components can subscribe to, bypassing the need to pass props down manually through each level of the component tree. Think of it like a global storage system for your application, where any component can access specific data it needs directly.
In simple terms, Context API acts as a manager for "global" data โ that is, data that many components need access to, such as user authentication status, theme settings, or preferred language.
When Should You Use Context?
While Context can be a powerful tool, it's best used selectively. Overusing it, especially for data that isn't widely shared or doesn't change frequently, can lead to complex and less efficient code. Here are a few scenarios where Context API is a great fit:
Authentication: Store user login status or user profile information accessible to many parts of the app.
Theme and Language Settings: Share themes or language preferences throughout the application.
Shopping Cart in E-Commerce: Track a user's cart items across various sections in a shopping application.
For smaller, isolated states that only one or two components need, using regular props or local component state is often more efficient.
How Does the Context API Work?
The Context API involves three main steps: creating a context, providing the context, and consuming the context.
Create a Context
You start by creating a context usingReact.createContext()
. This function returns a Context object that holds data you want to share.const UserContext = React.createContext();
Provide Context to Components
Next, use aProvider
component to make the context data available to the component tree. TheProvider
wraps the components that need access to the data and accepts avalue
prop, which contains the data to be shared.<UserContext.Provider value={user}> <MyComponent /> </UserContext.Provider>
Consume the Context
To access the context data in a child component, use theuseContext
hook. This hook allows components to read the context directly without needing to pass it down through props.import { useContext } from 'react'; const MyComponent = () => { const user = useContext(UserContext); return <div>Hello, {user.name}!</div>; };
Benefits of Using Context API
Efficiency in Data Flow: By avoiding prop drilling, you can manage data flow more effectively, especially in large applications with deep component hierarchies.
Improved Readability: Context makes the codebase more readable and maintainable by centralizing the management of commonly used data.
Better Flexibility with Hooks: The combination of Context and hooks, like
useContext
, allows for a much more modular and functional approach, making it easy to adjust your data management patterns as the app grows.
Limitations of the Context API
While Context is a convenient tool, it has some limitations:
Performance Considerations: Every time the context value changes, all components consuming that context re-render, potentially impacting performance. This can be mitigated by splitting up contexts for different parts of your app or using
React.memo
oruseMemo
.Debugging Complexity: Since Context can alter values across various components without explicit prop passing, debugging can become slightly harder in large applications.
Subscribe to my newsletter
Read articles from Pavani Pampana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pavani Pampana
Pavani Pampana
๐ Full Stack Developer | Problem Solver | Tech Enthusiast ๐ With 1 year of hands-on experience in full stack development, I specialize in building responsive, scalable, and efficient web applications. Passionate about both frontend and backend technologies, I love solving complex problems and constantly seek out new learning opportunities. ๐ง Skills: React.js, Python, Django, HTML, CSS, MySQL, and more! I am now actively looking for a new role where I can apply my expertise, contribute innovative solutions, and help drive the success of a forward-thinking organization. Let's build something impactful together!