Part 2: Enhancing Exponential Backoff with React Query for Efficient API Handling
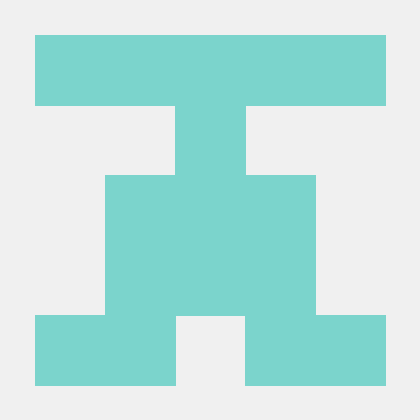
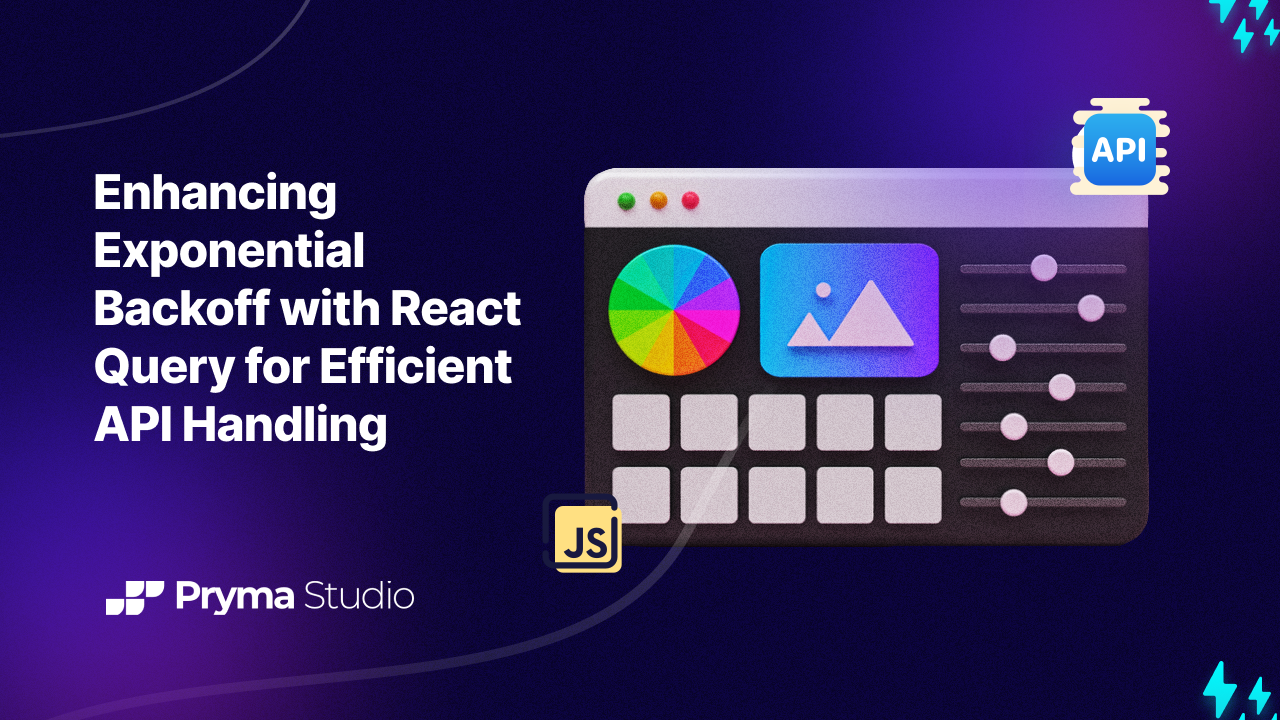
In this second part of our series, we will integrate the queueRequest
function with React Query. By combining these, you'll be able to manage data fetching more efficiently while benefiting from React Query’s advanced caching, synchronization, and state management.
Recap from Part 1
In Part 1, we implemented exponential backoff logic using the queueRequest
function. This function queues API requests and retries them with increasing delays when rate limits are encountered or requests fail. It ensures that even during high traffic or rate limiting, your API requests are handled gracefully.
The code for queueRequest
ensures that requests are managed in sequence:
export function queueRequest(url: string, options: RequestInit): Promise<any> {
return new Promise((resolve, reject) => {
requestQueue.push({ url, options, resolve, reject });
processQueue();
});
}
Integrating the queueRequest
function with React Query allows you to manage data fetching in a more efficient and resilient way, including retries and queuing, while benefiting from React Query's caching and synchronization capabilities.
Here’s how you can combine the two.
1. Setup the queueRequest
Function
Ensure you have your queueRequest
function implemented as described previously.
export function queueRequest(url: string, options: RequestInit): Promise<any> {
return new Promise((resolve, reject) => {
requestQueue.push({ url, options, resolve, reject });
processQueue();
});
}
2. Install React Query
If you haven’t already, install React Query:
npm install @tanstack/react-query
3. Create a Fetch Function for React Query
You can create a fetcher function that utilizes queueRequest
for use with React Query. This will handle the queuing and retries.
const fetchWithQueue = async (url: string): Promise<any> => {
const options: RequestInit = {
method: "GET",
};
const data = await queueRequest(url, options);
return data;
};
Here, fetchWithQueue
wraps the queueRequest
function, allowing us to manage retries and exponential backoff for our API calls.
4. Use React Query in Your Component
Now, you can use the useQuery
hook from React Query and pass in the fetchWithQueue
function to handle your API requests. React Query will handle caching, refetching, and invalidation.
Here’s an example component that fetches and displays data using the queueRequest
logic.
import React from 'react';
import { useQuery } from '@tanstack/react-query';
import { fetchWithQueue } from './queueRequest'; // Import your fetch function
const fetchPostData = async () => {
const url = 'https://jsonplaceholder.typicode.com/posts/1';
return fetchWithQueue(url); // Use the queueRequest wrapper
};
export const PostComponent = () => {
// Use React Query's useQuery hook to fetch the data
const { data, error, isLoading, isError } = useQuery(
['postData'], // Unique query key
fetchPostData
);
if (isLoading) return <div>Loading...</div>;
if (isError) return <div>Error: {error?.message}</div>;
return (
<div>
<h1>{data.title}</h1>
<p>{data.body}</p>
</div>
);
};
In this component:
useQuery
: This hook handles the API request. The first argument is a unique query key (postData
), and the second is the fetch function (fetchPostData
).State management: React Query handles the request state (
isLoading
,isError
,data
) and automatically updates the UI.
5. Handling Multiple Queued Requests in React Query
For handling multiple requests, you can pass in different URLs and React Query will queue them using queueRequest
. We’ll create a component that fetches data for multiple posts using the queueRequest
logic.
const fetchPostById = async (postId: number) => {
const url = `https://jsonplaceholder.typicode.com/posts/${postId}`;
return fetchWithQueue(url);
};
export const MultiplePostsComponent = () => {
const postIds = [1, 2, 3];
// Map through postIds and fetch each using useQuery
return (
<div>
{postIds.map((postId) => (
<Post key={postId} postId={postId} />
))}
</div>
);
};
const Post = ({ postId }: { postId: number }) => {
const { data, error, isLoading } = useQuery(
['post', postId], // Unique query key based on post ID
() => fetchPostById(postId)
);
if (isLoading) return <div>Loading Post {postId}...</div>;
if (error) return <div>Error loading post {postId}: {error.message}</div>;
return (
<div>
<h2>{data.title}</h2>
<p>{data.body}</p>
</div>
);
};
In this setup:
We map through an array of post IDs and fetch each post using its unique query key (
['post', postId]
). EachPost
component calls thefetchPostById
function, which in turn usesqueueRequest
to manage retries and backoff.React Query manages each post's loading and error state individually.
Key Benefits of Using React Query with queueRequest
:
Automatic Caching: React Query caches the result and avoids unnecessary network requests.
Error Handling: Both
queueRequest
and React Query handle errors. React Query retries the request in case of failure by default, but in this setup, exponential backoff and retries are handled byqueueRequest
.Query Keys: With React Query, each request has a unique query key, allowing for easy caching, refetching, and invalidation of specific data.
Loading States: React Query provides built-in loading and error states, which simplifies the UI.
Conclusion
By integrating queueRequest
with React Query, you combine the power of request queuing with React Query's caching and data synchronization, creating a robust data-fetching mechanism with exponential backoff, retries, and error handling. This setup is perfect for managing API rate limits and controlling the flow of requests in a React application.
Subscribe to my newsletter
Read articles from Abdulwasiu Abdulmuize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
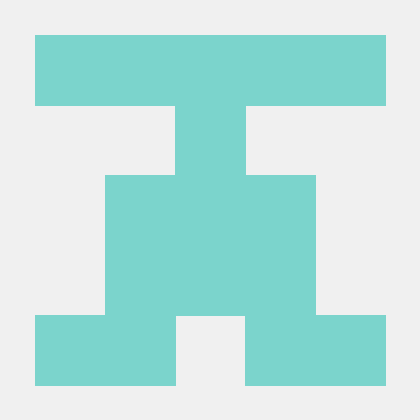