The Option Pattern: A Functional Programming Solution in C#
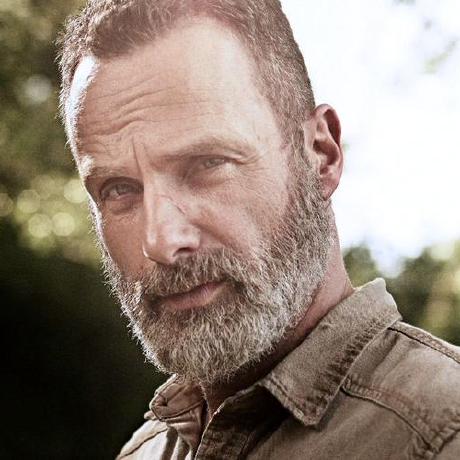
Introduction
Throughout my extensive career developing software with Microsoft technologies, I have faced various challenges. However, the most complex one has consistently been modernizing legacy systems with problematic codebases.
Often, these legacy codebases come with significant drawbacks:
Lack of documentation
Missing integration tests
Absence of unit tests
Poor null reference handling
These issues make long-term maintenance particularly challenging. The situation becomes even more complicated when dealing with objects that may or may not be null, leading to hard-to-resolve problems that could be avoided with better null-handling approaches in C#.
While C# has made considerable improvements by introducing features like the null-conditional operator (?
) and null-checking patterns (is null
), I believe these enhancements, while helpful, are not comprehensive enough for robust null handling.
My experience with functional programming has shown me how the Option/Maybe pattern can make code more reliable. I would love to see more C# developers and those working with other languages adopt these practices. Although these patterns aren't yet mainstream, they significantly contribute to code maintainability and reliability.
For these reasons, I've decided to explain what the Option pattern is, its brief history, origins, and how to effectively implement it in C#. This guide aims to introduce developers to a more robust way of handling nullable values, drawing from the best practices of functional programming.
Historical Origins
The Option pattern (also known as Maybe) has deep roots in functional programming:
First formally introduced in Haskell (as
Maybe
) in the late 1980sInspired by mathematical category theory and functional programming research
Widely adopted by ML-family languages and modern functional languages
The Billion Dollar Mistake
Tony Hoare, who invented null references in ALGOL W (1965), later famously called it his "billion-dollar mistake":
"This has led to innumerable errors, vulnerabilities, and system crashes, which have probably caused a billion dollars of pain and damage in the last forty years."
- Tony Hoare (2009)
The Cost of Null References
Research has demonstrated the significant impact of null-related issues:
Microsoft's studies found that 35-40% of bugs in production code were related to null reference exceptions
Amazon's research revealed similar patterns in their systems
Null-related bugs remain among the most common and costly issues in software development
Design Philosophy
The Option pattern represents a paradigm shift towards:
Explicit and Safer Programming
Forces developers to handle potential absence of values
Makes edge cases visible at the type level
Functional Programming Principles
Promotes immutability
Encourages pure functions
Ensures explicit data flow
Key Benefits
1. Type-Safe Null Handling
// Traditional approach (prone to null reference exceptions)
string GetUserName(int id) {
var user = repository.GetUser(id);
return user?.Name; // Might return null
}
// Option pattern approach
Option<string> GetUserName(int id) {
return repository.GetUser(id)
.Map(user => user.Name); // Explicitly handles absence
}
2. Explicit Error Handling
public class Option<T>
{
public T Value { get; }
public bool HasValue { get; }
// Pattern matching support
public TResult Match<TResult>(
Func<T, TResult> some,
Func<TResult> none) =>
HasValue ? some(Value) : none();
}
3. Composability
// Chaining operations that might fail
Option<Order> GetLatestOrder(int userId) =>
GetUser(userId)
.Bind(user => user.GetOrders())
.Bind(orders => orders.LastOrDefault());
Conclusion
The Option pattern effectively addresses several critical issues:
1. Null/Undefined Handling
Provides type-safe alternatives to null
Prevents runtime null reference exceptions
Makes potential absence of values explicit in the type system
2. Error Case Management
Forces explicit handling of success and failure cases
Improves code reliability and maintainability
Reduces bug surface area
3. Operation Composition
Enables clean chaining of operations that might fail
Supports functional programming patterns
Simplifies complex workflows with potential failure points
Closing Thoughts
Thank you for reading this article to the end. I will be publishing more content about utilizing C# in a functional programming style in the coming weeks. This series will explore how we can leverage functional programming concepts to write more robust and maintainable C# code.
Stay tuned for more articles where we'll dive deeper into functional programming patterns and their practical applications in C#.
Until next time!
Subscribe to my newsletter
Read articles from Rick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
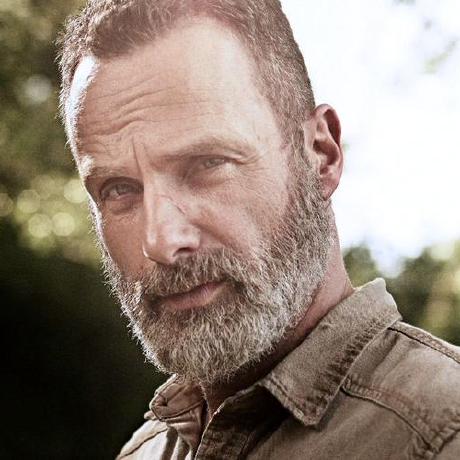
Rick
Rick
15+ years of experience having fun building apps with .NET I began my professional career in 2006, using Microsoft technologies where C# and Windows Forms and WPF were the first technologies I started working with during that time. I had the opportunity to actively participate in the Windows ecosystem as an MVP and Windows 8/Windows Phone application developer from 2013-2018. Throughout my career, I have used Azure as my default cloud platform and have primarily worked with technologies like ASP.NET Core for multiple companies globally across the US, UK, Korea, Japan, and Latin America. I have extensive experience with frameworks such as: ASP.NET Core Microsoft Orleans WPF UWP React with TypeScript Reactive Extensions Blazor I am an entrepreneur, speaker, and love traveling the world. I created this blog to share my experience with new generations and to publish all the technical resources that I had been writing privately, now made public as a contribution to enrich the ecosystem in which I have developed my career.