Mastering JavaScript Scope: Understanding Context in Your Code
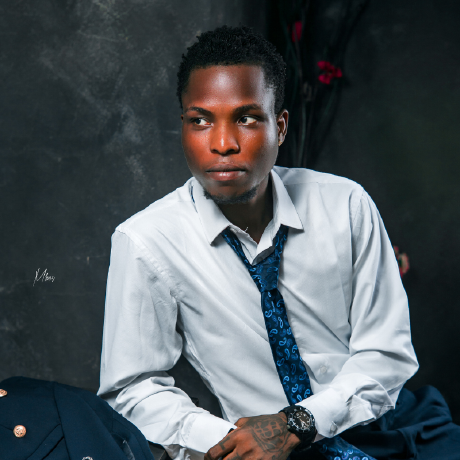
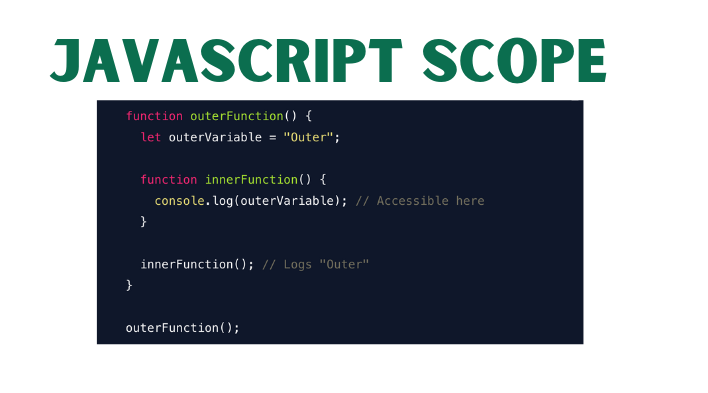
In JavaScript, scope determines the accessibility of variables and functions at different parts of your code. Mastering scope is essential for writing clean, bug-free JavaScript code and ensuring variables and functions behave as expected. Let’s dive into scope, its types, how to detect it, and why it's beneficial.
What is Scope in JavaScript?
Scope is the current context of execution in which values and expressions are “visible” or can be referenced. Think of it as a boundary for where variables and functions are accessible in your code. Scopes in JavaScript help in organizing your code and ensuring that each variable is used where intended.
Types of Scope in JavaScript
Global Scope
Definition: Variables or functions declared outside of any function or block reside in the global scope, making them accessible from anywhere in your code.
Example:
let globalVar = "I am global!"; function showGlobal() { console.log(globalVar); // Accessible here } showGlobal(); // Logs "I am global!"
Function Scope
Definition: Variables declared within a function are only accessible inside that function.
Example:
function myFunction() { let functionScoped = "Only inside this function"; console.log(functionScoped); // Accessible here } myFunction(); // Logs "Only inside this function" // console.log(functionScoped); // Error: functionScoped is not defined
Block Scope
Definition: Variables declared with
let
orconst
inside a block (i.e.,{}
) are only accessible within that block.Example:
{ let blockScoped = "Only inside this block"; console.log(blockScoped); // Accessible here } // console.log(blockScoped); // Error: blockScoped is not defined
Lexical Scope
Definition: JavaScript follows lexical scoping, meaning that inner functions have access to variables defined in their outer functions.
Example:
function outerFunction() { let outerVariable = "Outer"; function innerFunction() { console.log(outerVariable); // Accessible here } innerFunction(); // Logs "Outer" } outerFunction();
Detecting Scope in Your Code
Understanding the scope of a variable or function can help prevent unexpected behaviors:
Look at the Declaration Location: A variable’s scope is usually determined by where it is declared.
Use Console Logs: Logging variables at different points in your code can clarify their accessibility.
Use Strict Mode:
use strict;
helps by preventing undeclared variables from polluting the global scope.
Syntax Overview
Global Scope: Declared outside functions and blocks.
Function Scope: Declared with
var
,let
, orconst
inside functions.Block Scope: Declared with
let
orconst
inside{}
.Lexical Scope: Accessed based on where functions are defined.
Benefits of Understanding Scope
Prevents Variable Collision: Helps avoid situations where variable names conflict across different parts of code.
Improves Code Readability: Knowing the limits of each variable’s scope makes code easier to follow.
Enhances Memory Efficiency: Variables limited in scope are discarded once they are no longer needed.
Allows Modular Code: Scoping variables to functions or blocks makes it easier to write modular, reusable code.
Key Tips Before Using Scope in JavaScript
Use
let
andconst
: Preferlet
andconst
overvar
for block scoping and better control.Avoid Polluting the Global Scope: Minimize global variables to prevent unintended interactions.
Understand Closure: When a function remembers variables from its outer scope, it creates a closure, which can lead to memory retention.
Think Modular: Keep functions small and encapsulated to leverage scope effectively.
Example of Scope in Practice
let globalVar = "Global";
function outer() {
let outerVar = "Outer";
function inner() {
let innerVar = "Inner";
console.log(globalVar); // Accessible
console.log(outerVar); // Accessible
console.log(innerVar); // Accessible
}
inner();
// console.log(innerVar); // Error: innerVar is not defined
}
outer();
In this example:
globalVar
is accessible everywhere.
outerVar
is accessible withinouter
andinner
.
innerVar
is accessible only withininner
.
Wrapping Up
Mastering scope allows you to write organized, predictable code. By using the right scope types, you can control where variables are accessible, leading to efficient memory use and better modularity in code design.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
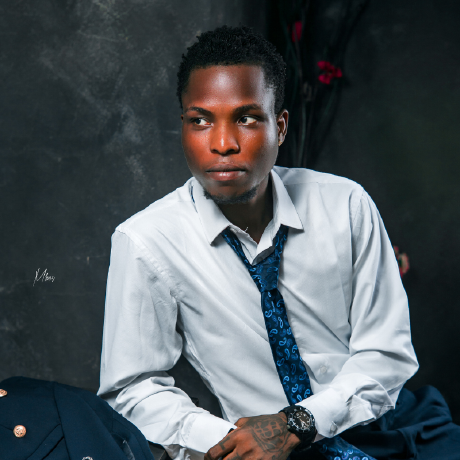
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com