Building a Scalable API Documentation Strategy
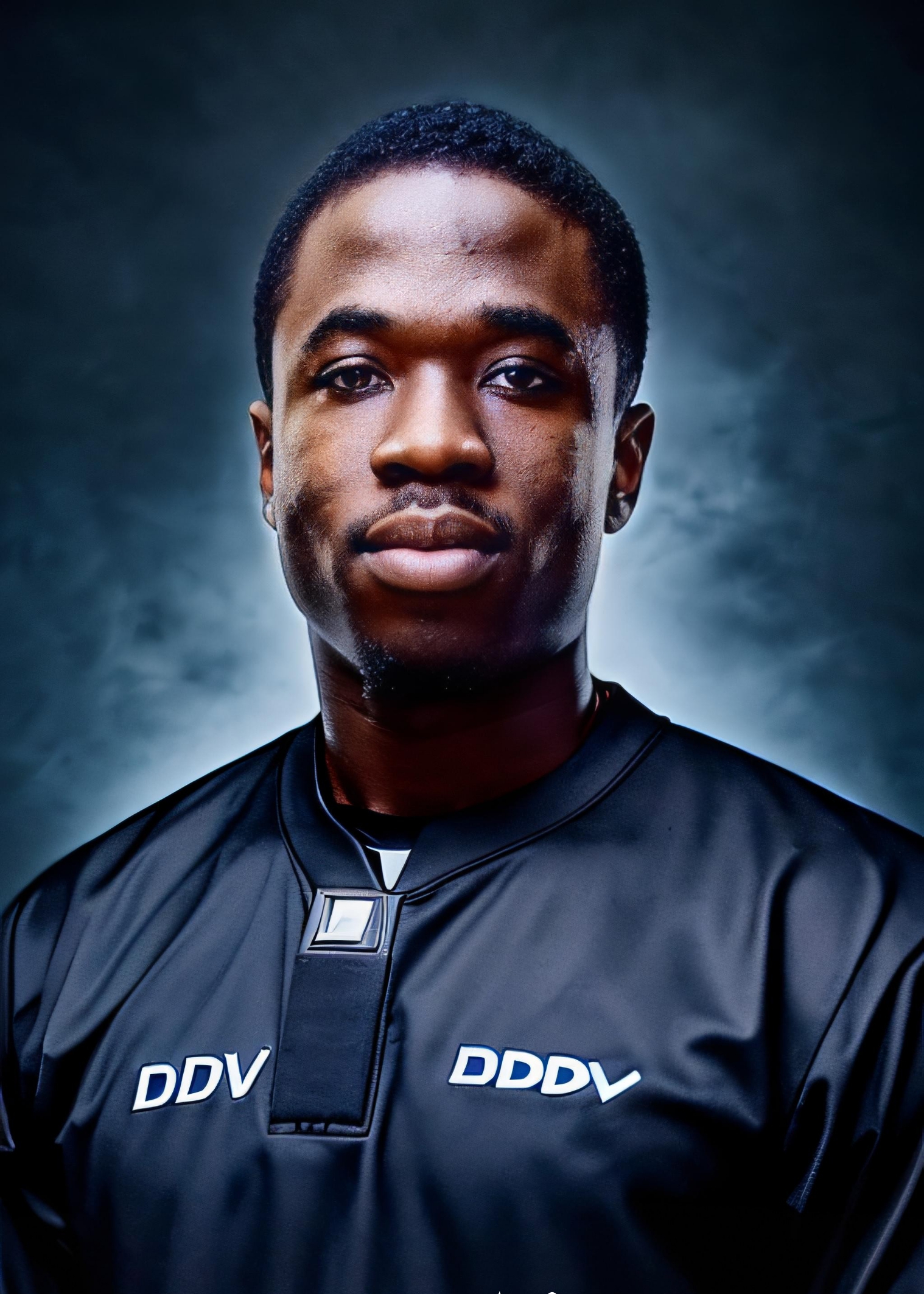
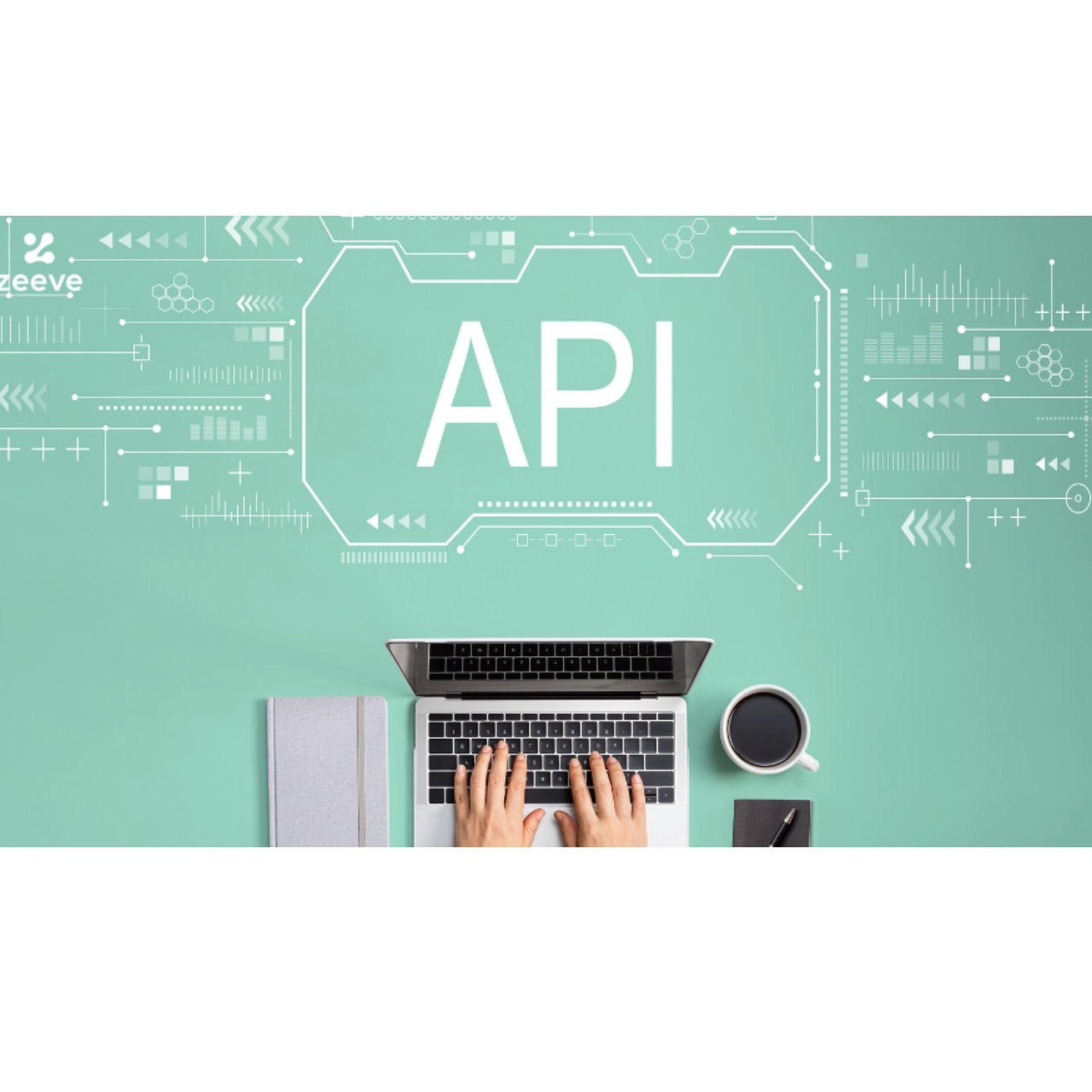
In today's API-driven world, documentation is no longer just a technical requirement—it's a crucial business asset that can make or break your developer adoption rates. Well-structured API documentation serves as the first point of contact between your service and potential integrators, acting as both a technical guide and a powerful marketing tool.
Introduction
Quality API documentation directly impacts developer experience, integration success rates, and ultimately, your API's adoption. Organizations investing in comprehensive documentation strategies report:
34% faster integration times
48% reduction in support tickets
63% higher developer satisfaction rates
Let's explore how to build a documentation strategy that scales with your API's growth and meets the evolving needs of your developer community.
Fundamentals of API Documentation
Documentation Types
Your documentation ecosystem should include multiple types of content, each serving a specific purpose:
Reference Documentation
Comprehensive API specifications
Detailed parameter descriptions
Response schemas and examples
Error codes and handling procedures
Tutorials and Guides
Getting started guides
Authentication tutorials
Common use case implementations
Best practices and optimization tips
Code Samples
Language-specific examples
Complete integration scenarios
SDK implementation guides
Common patterns and solutions
API Changelog
Version updates and changes
Deprecation notices
Migration guides
Breaking change alerts
Documentation Standards
Adhering to established standards ensures consistency and maintainability:
OpenAPI (Swagger) Specification The OpenAPI specification has become the de facto standard for RESTful API documentation. It provides:
Machine-readable API descriptions
Automated documentation generation
Interactive API testing capabilities
Code generation tools
JSON/RAML Standards
Structured format for API definitions
Clear hierarchy of resources
Reusable components and patterns
Tool-friendly documentation format
Core Components
Authentication Documentation
Security documentation requires special attention as it's often the first hurdle developers face:
Authorization Methods
{
"auth_types": {
"oauth2": {
"flow": "authorization_code",
"authorizationUrl": "https://api.example.com/oauth/authorize",
"tokenUrl": "https://api.example.com/oauth/token",
"scopes": {
"read": "Read access to protected resources",
"write": "Write access to protected resources"
}
}
}
}
Token Management
Token acquisition flows
Refresh token procedures
Token storage best practices
Security considerations
Endpoints and Resources
Organize your endpoints documentation logically:
GET /api/v1/users
- Returns a list of users
- Supports pagination
- Optional filtering parameters
Parameters:
- page (integer): Page number for pagination
- limit (integer): Number of results per page
- filter (string): Filter criteria for users
Rate Limiting
Clear rate limiting documentation prevents integration issues:
Rate Limits:
- 1000 requests per hour for authenticated users
- 100 requests per hour for unauthenticated users
- Burst limits: 100 requests per minute
Interactive Elements
API Playground/Console
Provide an interactive environment for testing:
// Example of interactive console code
const response = await fetch('https://api.example.com/v1/users', {
headers: {
'Authorization': 'Bearer YOUR_TOKEN',
'Content-Type': 'application/json'
}
});
const users = await response.json();
console.log(users);
Sample Applications
Offer complete, working examples:
# Python SDK implementation example
from example_api import Client
client = Client(api_key='YOUR_API_KEY')
# List all users with pagination
users = client.users.list(
page=1,
limit=10,
filter='active=true'
)
for user in users:
print(f"User ID: {user.id}, Name: {user.name}")
Version Control and Management
API Versioning Strategies
Choose and document a clear versioning strategy:
URL Versioning
https://api.example.com/v1/resources https://api.example.com/v2/resources
Header Versioning
Accept: application/vnd.example.v1+json Accept: application/vnd.example.v2+json
Change Management
Document your change management process:
Breaking Changes:
- Announced 6 months in advance
- Supported for 12 months after deprecation
- Migration guides provided
- Test environment available
Documentation Infrastructure
Documentation Platforms
Choosing the right documentation platform is crucial for long-term maintainability:
Static Site Generators
Jekyll/Hugo for high-performance static sites
MkDocs for Markdown-based documentation
Sphinx for complex technical documentation
Example mkdocs.yml
configuration:
site_name: API Documentation
theme:
name: material
features:
- navigation.tabs
- search.suggest
plugins:
- search
- mermaid
nav:
- Home: index.md
- API Reference: reference.md
- Guides:
- Authentication: guides/auth.md
- Rate Limiting: guides/rate-limits.md
Automation
Implement automation to maintain documentation accuracy:
API Specification Generation
// Example of automated OpenAPI spec generation
const swaggerJsdoc = require('swagger-jsdoc');
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'API Documentation',
version: '1.0.0',
},
},
apis: ['./routes/*.js'],
};
const specs = swaggerJsdoc(options);
Documentation Testing
# Example of automated doc testing
def test_endpoint_documentation():
schema = get_api_schema('/users')
assert 'get' in schema
assert 'parameters' in schema['get']
assert 'responses' in schema['get']
Maintenance and Updates
Review Process
Establish a systematic review workflow:
Technical Accuracy Checks
Weekly automated endpoint testing
Monthly manual review of examples
Quarterly deep-dive technical audits
User Feedback Integration
Feedback Loop: 1. Collect feedback (Github issues, support tickets) 2. Prioritize changes (impact vs. effort matrix) 3. Implement updates 4. Verify with user testing
Quality Assurance
Implement comprehensive QA processes:
Documentation Testing Checklist
[ ] All code examples are tested and working
[ ] Links are valid and pointing to correct resources
[ ] Authentication examples include all supported methods
[ ] Response examples match current API responses
[ ] Rate limit information is accurate
[ ] Deprecated features are clearly marked
Developer Experience
Navigation and Search
Optimize for developer efficiency:
<!-- Example of enhanced search implementation -->
<div class="doc-search">
<input
type="search"
placeholder="Search API docs..."
data-search-index="/api/search.json"
data-instant-results="true"
>
<div class="search-results" aria-live="polite"></div>
</div>
Accessibility Features
Ensure documentation is accessible to all developers:
Mobile Responsiveness
/* Example of responsive documentation styles */ .documentation-container { max-width: 1200px; margin: 0 auto; padding: 20px; } @media (max-width: 768px) { .documentation-container { padding: 10px; font-size: 14px; } pre { max-width: 100%; overflow-x: auto; } }
Offline Access
Progressive Web App implementation
Downloadable PDF versions
Cached documentation updates
Metrics and Improvement
Usage Analytics
Track key documentation metrics:
// Example of documentation analytics tracking
const docMetrics = {
trackPageView: (page) => {
analytics.track({
event: 'doc_page_view',
properties: {
page,
timeSpent: calculateTimeSpent(),
scrollDepth: calculateScrollDepth(),
searchTerms: getSearchHistory()
}
});
}
};
Key Metrics to Monitor:
Page views per section
Time spent per page
Search query patterns
Failed searches
Feedback ratings
Support ticket correlation
Continuous Improvement
Implement a continuous improvement cycle:
Regular User Testing
Monthly user interviews
Quarterly usability studies
A/B testing of new features
Documentation Health Metrics
Monthly Documentation Health Check: - Coverage: 98% of API endpoints documented - Accuracy: 99% of examples verified working - Freshness: 95% of content updated in last 6 months - User Satisfaction: 4.5/5 average rating
Best Practices and Guidelines
Writing Style
Maintain consistent documentation style:
Style Guide Highlights:
Use active voice
Keep sentences concise
Include code examples for all concepts
Maintain consistent terminology
Avoid jargon and abbreviations
Include error scenarios and edge cases
Design Patterns
Follow established documentation design patterns:
Visual Hierarchy
Clear headings and subheadings
Consistent spacing and formatting
Color-coded elements for different content types
Prominent call-outs for important information
Code Formatting
// Example of consistent code formatting { "status": "success", "data": { "user": { "id": "123", "name": "John Doe", "email": "john@example.com" } }, "meta": { "requestId": "abc-123", "timestamp": "2024-10-28T12:00:00Z" } }
Conclusion
Building a scalable API documentation strategy requires careful planning, consistent execution, and continuous improvement. Remember these key takeaways:
Start with a solid foundation of standards and tools
Automate wherever possible to maintain accuracy
Focus on developer experience and accessibility
Measure and iterate based on user feedback
Maintain consistency in style and formatting
Regular updates and maintenance are crucial
By following these guidelines and continuously adapting to your users' needs, you can build documentation that not only serves its purpose but becomes a competitive advantage for your API.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
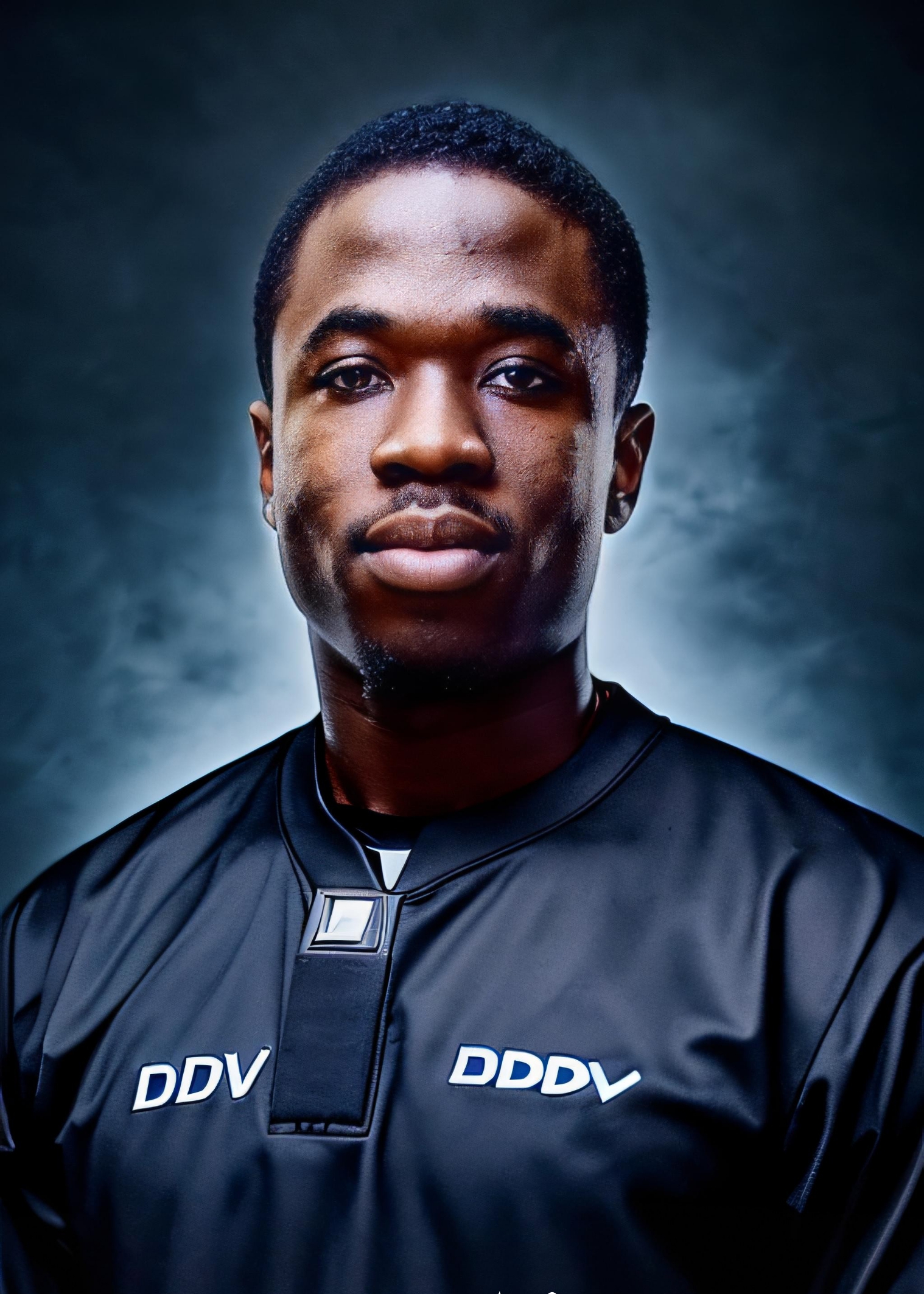
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.