Docker Volume And Docker Network .
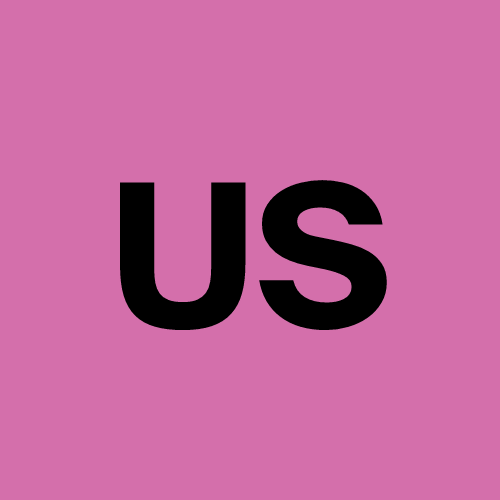
Table of contents
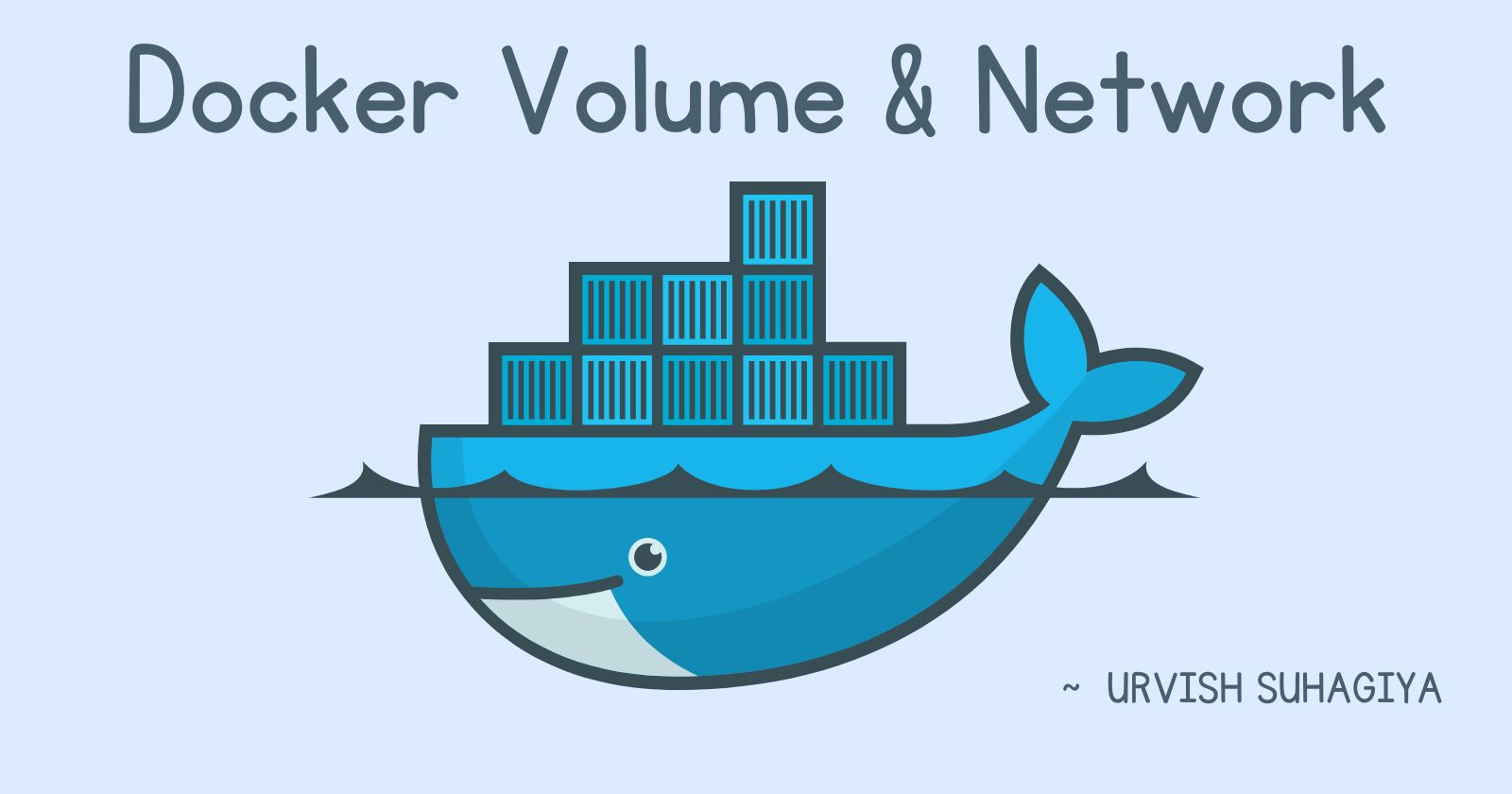
Docker Volumes :
Example Scenario
Imagine you have a web application that saves user uploads. You don't want these uploads to disappear every time you update or restart your container. By using a volume, you can ensure that the uploaded files persist.
What is a Docker Volume?
A Docker Volume is like a special storage space that you can use to save data generated by your Docker containers. Think of it as a separate hard drive that you attach to your container. This storage is outside of the container itself, so even if you delete the container, the data in the volume remains safe.
Why Use Docker Volumes?
Persistence: Data stored in a volume persists even after the container is deleted.
Sharing: Multiple containers can access the same volume, allowing them to share data.
Ease of Backup: Volumes can be easily backed up and restored.
Separation of Concerns: Keeps your container image lightweight by separating data storage from the application.
How to Use Docker Volumes?
- Creating a Volume:
docker volume create my_volume
- Attaching a Volume to a Container:
docker run -d --name my_container --mount source=my_volume,target=/app busybox
- Viewing Volumes:
docker volume ls
- Removing a Volume:
docker volume rm my_volume
Docker Networks :
Example Scenario
Imagine you have a multi-tier application with a web server container and a database container. You want the web server to communicate with the database. By creating a network, you can connect both containers to this network, allowing them to talk to each other securely.
What is a Docker Network?
A Docker Network allows multiple Docker containers to communicate with each other. It's like setting up a local network where all your containers can talk to each other securely and efficiently.
Why Use Docker Networks?
Container Communication: Enables containers to discover and communicate with each other.
Isolation: Provides isolation between containers. Containers on different networks cannot communicate unless you connect them.
Flexibility: You can create multiple networks and attach containers to one or more networks as needed.
How to Use Docker Networks?
Creating a Network:
docker network create my_network
- Running a Container on a Network:
docker run -d --name my_container --network my_network busybox
- Viewing Networks:
docker network ls
- Inspecting a Network:
docker network inspect my_network
- Removing a Network:
docker network rm my_network
Combining Docker Volumes and Networks
Here's a practical example using Docker Compose to bring it all together:
- Create a New Directory for Docker Compose:
mkdir docker-setup
cd docker-setup
- Create the docker-compose.yml File:
touch docker-compose.yml
- Add the Docker Compose Configuration:
docker-compose.yml
Example:
version: '3'
services:
db:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: example
ports:
- "3306:3306"
web:
image: nginx:latest
deploy:
replicas: 3
endpoint_mode: dnsrr
ports:
- "8081:80"
- "8082:80"
- "8083:80"
Steps to Run:
Managing the Docker Containers
- Start the Multi-Container Application:
docker-compose up -d
- Scale the Web Service:
docker-compose up -d --scale web=3
- View the Status of Containers:
docker-compose ps
- View Logs of the Web Service:
docker-compose logs web
- Stop and Remove Containers, Networks, and Volumes:
docker-compose down
Example for Docker Volumes Sharing
- Create a Named Volume:
docker volume create shared-data
- Run the First Container:
docker run -d --name container1 --mount source=shared-data,target=/data busybox sh -c "while true; do echo 'Hello from container1' > /data/file.txt; sleep 1; done"
- Run the Second Container:
docker run -d --name container2 --mount source=shared-data,target=/data busybox sh -c "while true; do cat /data/file.txt; sleep 1; done"
- Verify Data Sharing:
docker exec container2 cat /data/file.txt
- List All Volumes:
docker volume ls
- Remove the Volume:
docker volume rm shared-data
By understanding and using Docker Volumes and Docker Networks, we can create flexible, scalable, and persistent applications that leverage the full power of Docker.
Subscribe to my newsletter
Read articles from Urvish Suhagiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
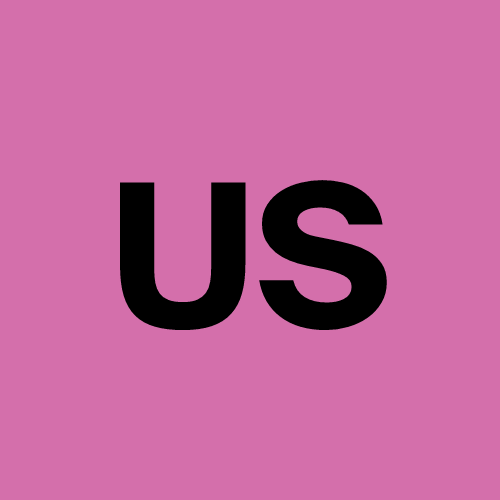
Urvish Suhagiya
Urvish Suhagiya
Exploring the world of DevOps ๐.