Flutter State Management: A Comprehensive Guide
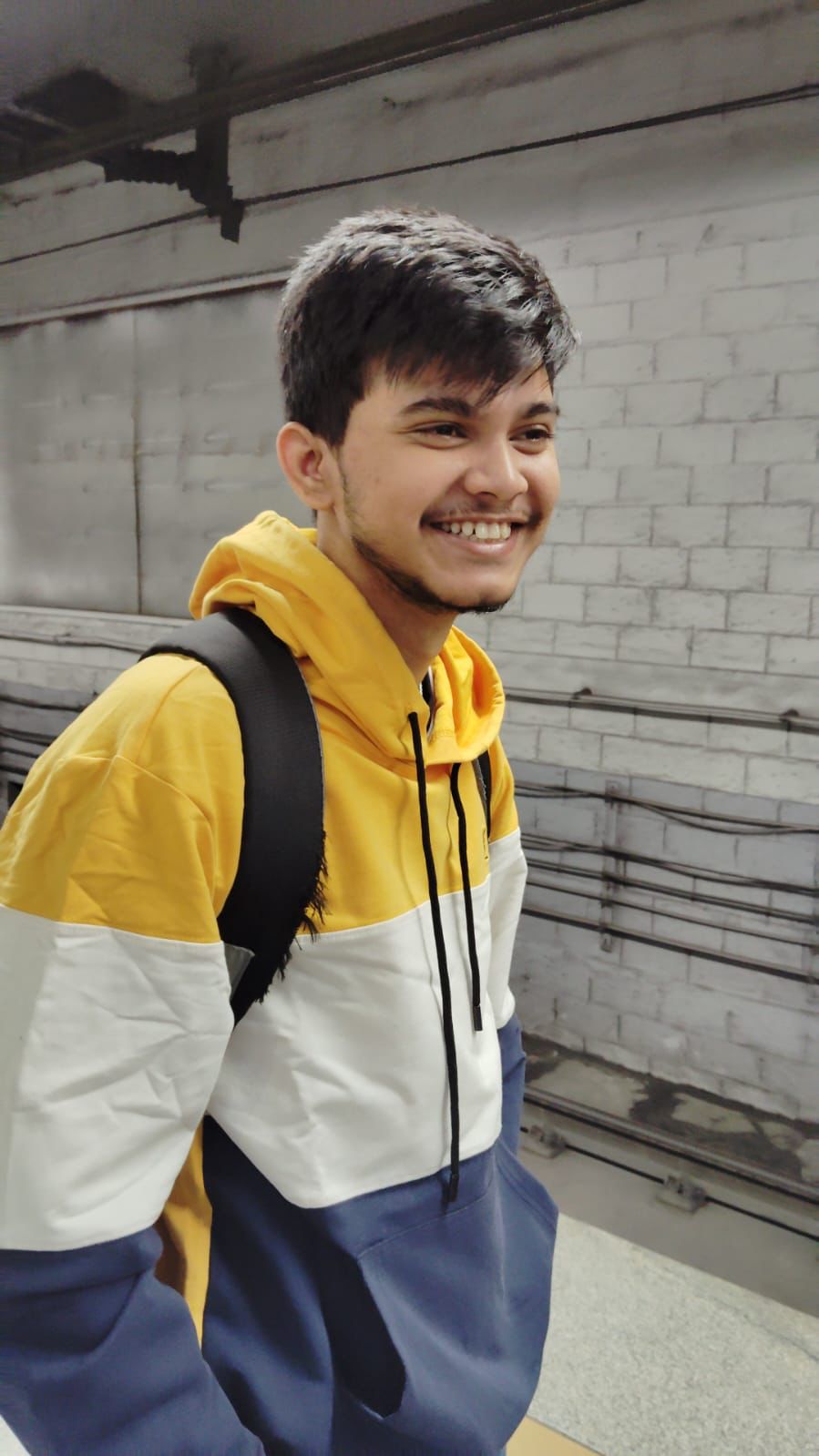
Table of contents
- Flutter State Management: A Comprehensive Guide
- Understanding State in Flutter
- 1. setState() - The Basic Approach
- 2. Provider - The Community Favorite
- 3. Bloc - For Complex Applications
- 4. Riverpod - The Evolution of Provider
- 5. GetX - The All-in-One Solution
- Choosing the Right Solution
- Best Practices
- Conclusion

Flutter State Management: A Comprehensive Guide
State management is one of the most crucial aspects of building Flutter applications. It's the backbone that determines how your app handles data flow and UI updates. In this guide, we'll explore different state management approaches in Flutter and help you choose the right one for your project.
Understanding State in Flutter
Before diving into specific solutions, let's understand what "state" means in Flutter:
Ephemeral State (Local State): Temporary state that belongs to a single widget
App State (Global State): Data that needs to be shared across multiple widgets
Server State: Data that comes from external sources like APIs
1. setState() - The Basic Approach
setState()
is Flutter's built-in solution for handling local state:
class CounterWidget extends StatefulWidget {
@override
_CounterWidgetState createState() => _CounterWidgetState();
}
class _CounterWidgetState extends State<CounterWidget> {
int count = 0;
void increment() {
setState(() {
count++;
});
}
// ... rest of the implementation
}
Best for:
Simple widgets
Local state management
Prototypes and small apps
Limitations:
Not suitable for sharing state between widgets
Can lead to unnecessary rebuilds
Difficult to manage in larger applications
2. Provider - The Community Favorite
Provider is a wrapper around InheritedWidget, making it easier to manage and share state:
// Define a ChangeNotifier
class Counter with ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
// Use in widget tree
ChangeNotifierProvider(
create: (_) => Counter(),
child: MyApp(),
);
// Consume the state
Consumer<Counter>(
builder: (context, counter, child) {
return Text('${counter.count}');
},
);
Best for:
Medium-sized applications
Sharing state between widgets
Reactive state management
3. Bloc - For Complex Applications
BLoC (Business Logic Component) separates business logic from UI:
// Event
abstract class CounterEvent {}
class IncrementEvent extends CounterEvent {}
// State
class CounterState {
final int count;
CounterState(this.count);
}
// Bloc
class CounterBloc extends Bloc<CounterEvent, CounterState> {
CounterBloc() : super(CounterState(0)) {
on<IncrementEvent>((event, emit) {
emit(CounterState(state.count + 1));
});
}
}
Best for:
Large applications
Complex state management
Teams with clear architecture preferences
4. Riverpod - The Evolution of Provider
Riverpod offers compile-time safety and better dependency management:
// Define a provider
final counterProvider = StateNotifierProvider<Counter, int>((ref) {
return Counter();
});
class Counter extends StateNotifier<int> {
Counter() : super(0);
void increment() => state++;
}
// Use in widgets
Consumer(
builder: (context, ref, child) {
final count = ref.watch(counterProvider);
return Text('$count');
},
);
Best for:
Type-safe state management
Complex dependency graphs
Modern Flutter applications
5. GetX - The All-in-One Solution
GetX provides state management, routing, and dependency injection:
class Controller extends GetxController {
var count = 0.obs;
void increment() => count++;
}
// In widget
GetX<Controller>(
builder: (controller) {
return Text('${controller.count}');
},
);
Best for:
Rapid development
All-in-one solution needs
Simpler learning curve
Choosing the Right Solution
When selecting a state management solution, consider:
Project Size:
Small projects: setState or Provider
Medium projects: Provider or Riverpod
Large projects: Bloc or Riverpod
Team Experience:
New to Flutter: Provider
Experienced team: Bloc or Riverpod
Need quick results: GetX
Project Requirements:
Simple data flow: Provider
Complex business logic: Bloc
Type safety priority: Riverpod
Best Practices
Keep It Simple:
Don't over-engineer for small applications
Start with simpler solutions and migrate when needed
Consistency:
Stick to one primary state management solution
Document your state management patterns
Testing:
Choose solutions that make testing easier
Write unit tests for your state logic
Performance:
Monitor widget rebuilds
Use selective state updates when possible
Conclusion
There's no one-size-fits-all solution for state management in Flutter. The best approach depends on your specific needs, team size, and project complexity. Start with simpler solutions and evolve as your needs grow. Remember that good architecture is about making maintenance easier and code more readable, not about using the most complex solution available.
The Flutter ecosystem continues to evolve, and new state management solutions may emerge. The key is to understand the fundamentals and choose a solution that balances complexity with functionality for your specific use case.
Subscribe to my newsletter
Read articles from Satyam Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
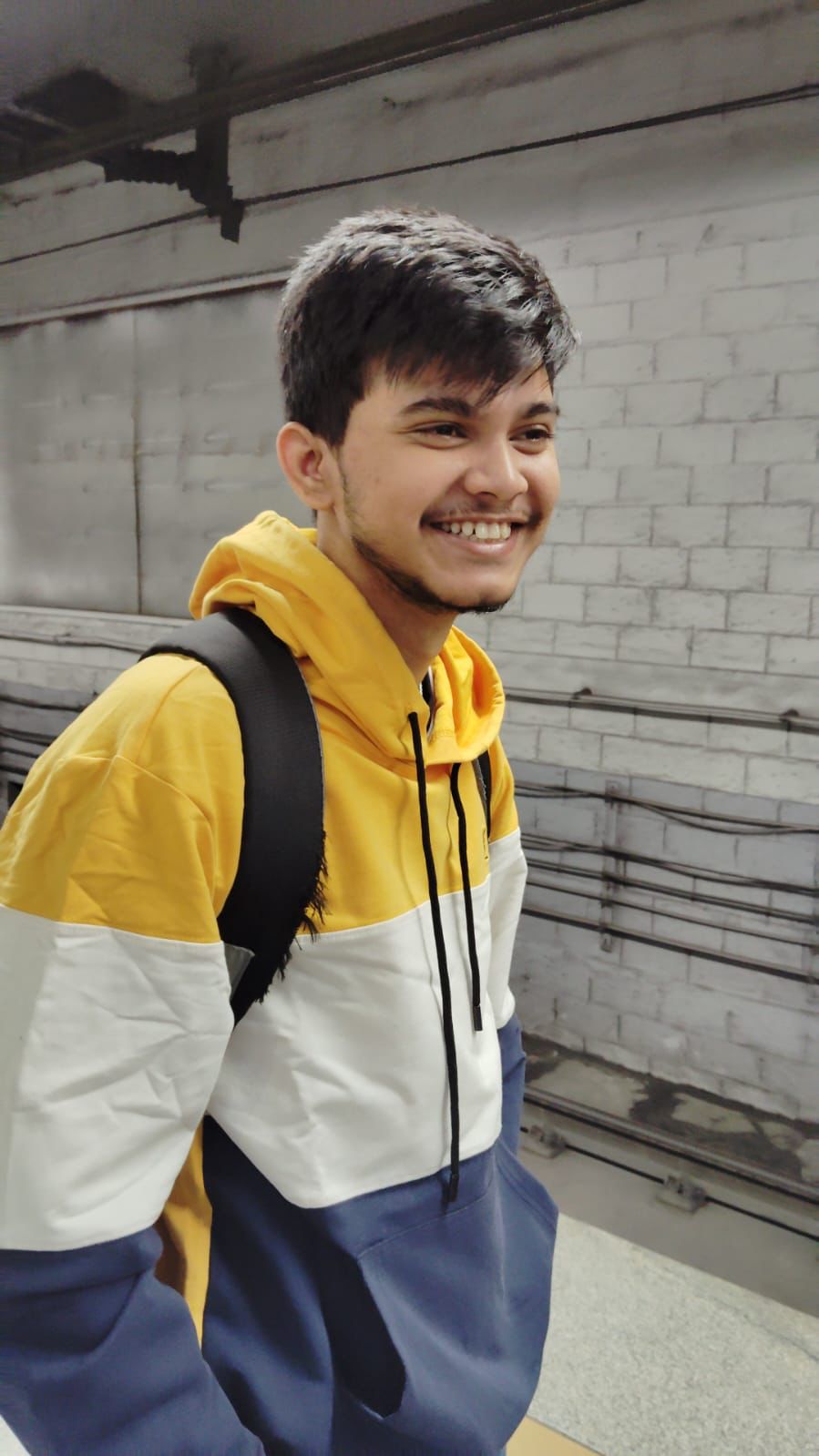
Satyam Jha
Satyam Jha
Hey , I am Satyam, I am a Flutter Developer experience over 3 years and good experience of figma.