Building an ML Pipeline with Kubeflow: From Development to Production
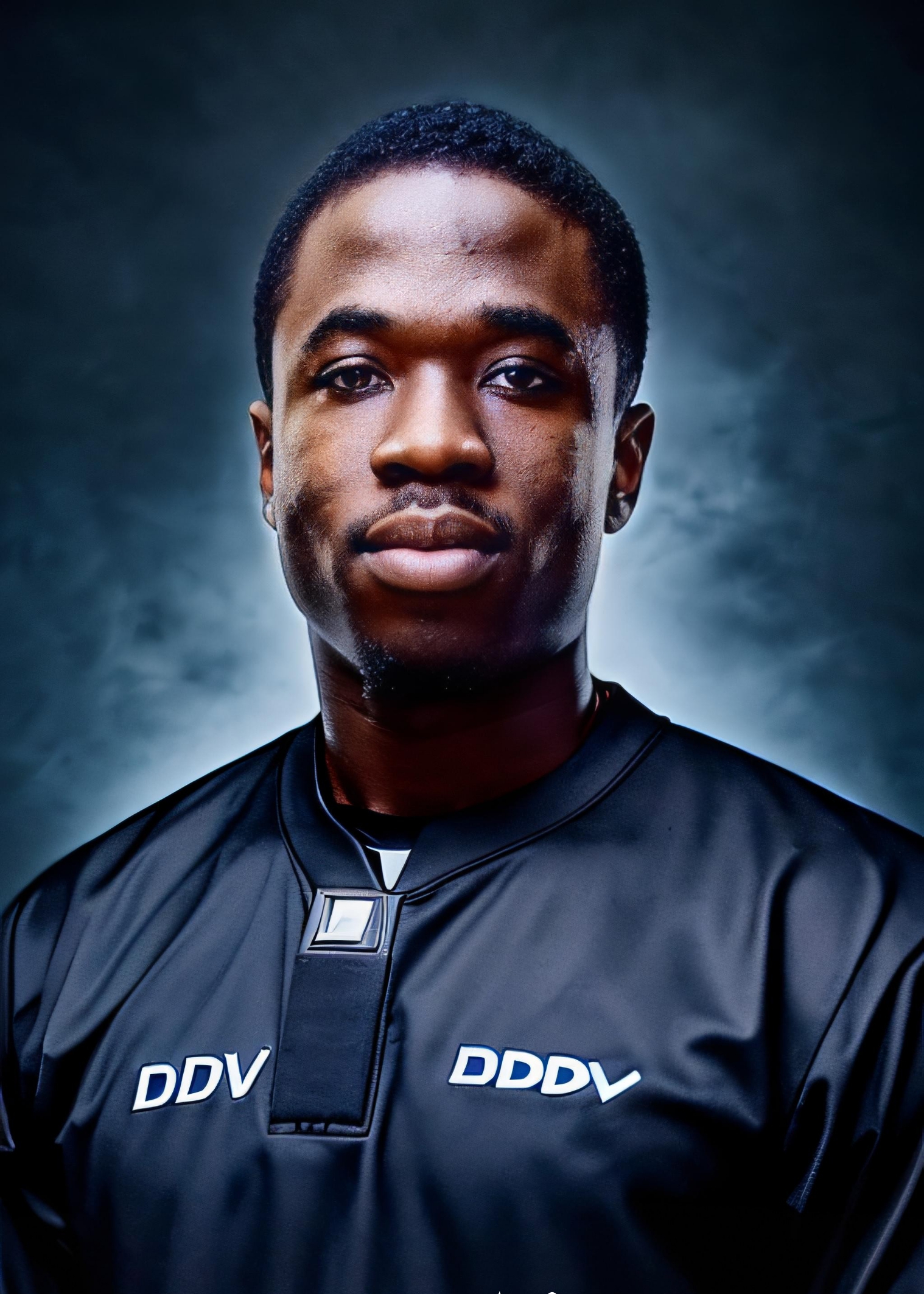
Table of contents
- Introduction
- Prerequisites
- Understanding Kubeflow Architecture
- Core Components
- Component Interaction
- Setting Up Your Development Environment
- Installing Kubeflow
- Configuring Authentication
- Setting Up Persistent Storage
- Creating Your First ML Pipeline
- Working with Kubeflow Notebooks
- Creating Custom Notebook Servers
- Managing Dependencies
- Building Production-Ready Training Workflows
- Component Definition
- Error Handling and Retries
- Model Serving and Deployment
- Setting up KFServing
- Implementing Canary Deployments
- Pipeline Monitoring and Logging
- Metrics Collection
- Logging Configuration
- Production Best Practices
- Resource Optimization
- Pipeline Optimization and Scaling
- Distributed Training Implementation
- GPU Utilization
- Integration with External Tools
- Setting up CI/CD Pipeline
- Model Registry Integration
- Troubleshooting and Maintenance
- Common Issues and Solutions
- Case Study: End-to-End Implementation
- Real-World Scenario: Customer Churn Prediction
- Future Considerations
- Emerging Trends
- Additional Resources
- Official Documentation
- Community Resources
- Related Projects
- Conclusion
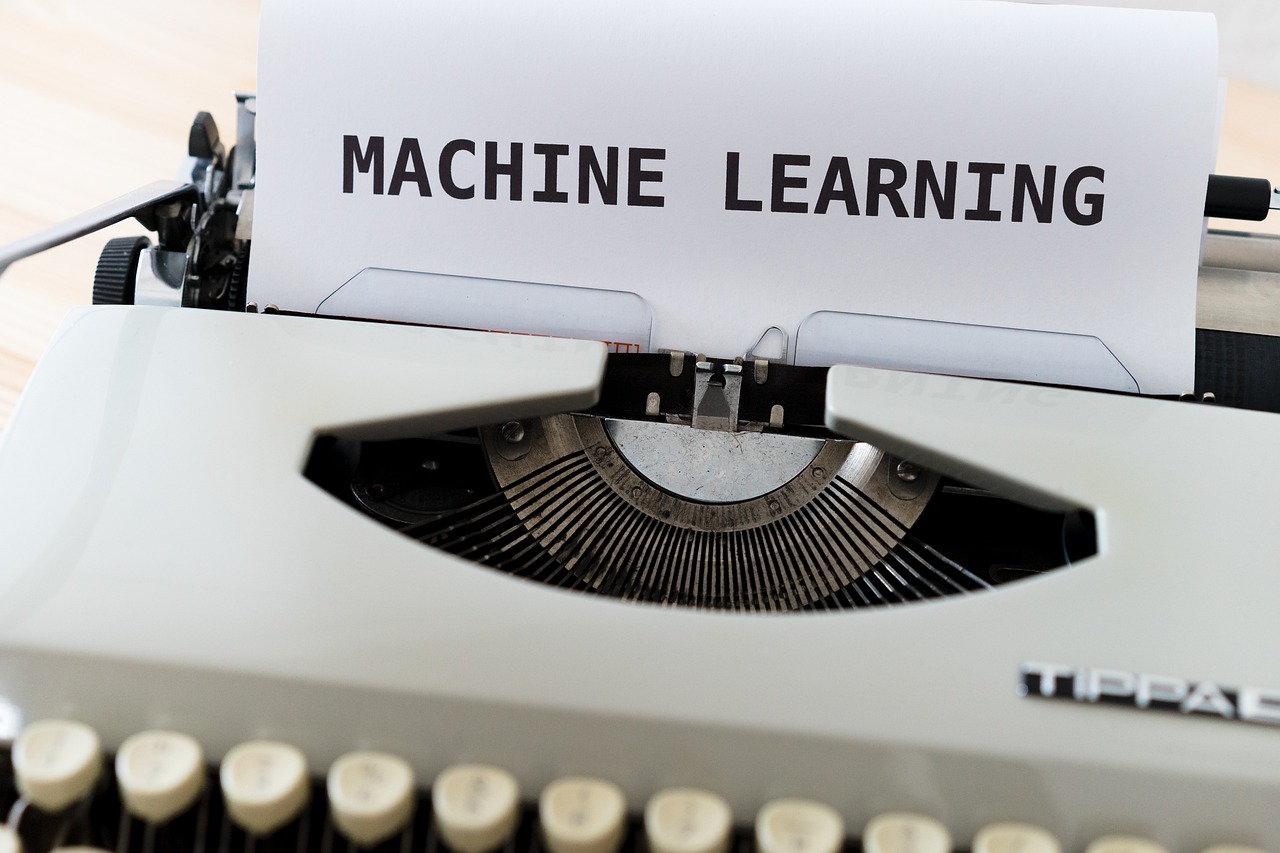
Machine Learning (ML) workloads in production require robust, scalable, and maintainable pipelines. Kubeflow provides a comprehensive solution for deploying ML workflows on Kubernetes, enabling data scientists and ML engineers to focus on model development while leveraging the scalability and reliability of cloud-native infrastructure.
Introduction
Kubeflow is an open-source ML platform designed to simplify the deployment of ML workflows on Kubernetes. It provides a complete toolkit for developing, training, and deploying ML models at scale. This guide will walk you through building production-ready ML pipelines using Kubeflow, from initial setup to deployment and maintenance.
Prerequisites
Working knowledge of Kubernetes
Basic understanding of ML concepts
Familiarity with Python
Access to a Kubernetes cluster (v1.21+)
kubectl CLI tool installed
Python 3.8+
Understanding Kubeflow Architecture
Kubeflow's architecture is built on a modular design principle, allowing teams to use components independently or as a complete platform.
Core Components
Kubeflow Central Dashboard
Single entry point for all Kubeflow components
Web-based UI for managing ML workflows
Integration with all platform services
Pipeline Platform
Orchestrates end-to-end ML workflows
Manages pipeline execution and scheduling
Handles artifact management and versioning
Notebook Servers
JupyterHub-based development environment
Supports custom images and configurations
Integrated with version control systems
Training Operators
TensorFlow training (TFJob)
PyTorch training (PyTorchJob)
MXNet training (MXNetJob)
XGBoost training (XGBoostJob)
Component Interaction
apiVersion: kubeflow.org/v1
kind: TFJob
metadata:
name: mnist-training
spec:
tfReplicaSpecs:
Worker:
replicas: 3
template:
spec:
containers:
- name: tensorflow
image: tensorflow/tensorflow:2.8.0
command:
- "python"
- "/opt/model/train.py"
Setting Up Your Development Environment
Installing Kubeflow
- Using the official distribution:
# Add the Kubeflow repository
export PIPELINE_VERSION=1.8.5
kubectl apply -k "github.com/kubeflow/pipelines/manifests/kustomize/cluster-scoped-resources?ref=$PIPELINE_VERSION"
kubectl wait --for condition=established --timeout=60s crd/applications.app.k8s.io
kubectl apply -k "github.com/kubeflow/pipelines/manifests/kustomize/env/platform-agnostic-pns?ref=$PIPELINE_VERSION"
- Verify the installation:
kubectl get pods -n kubeflow
Expected output:
NAME READY STATUS RESTARTS AGE
ml-pipeline-persistenceagent-84f6d87478-8w4cc 1/1 Running 0 3m
ml-pipeline-scheduledworkflow-6c978b6b85-vxvhk 1/1 Running 0 3m
ml-pipeline-viewer-crd-6db65ccc4-mk6lm 1/1 Running 0 3m
ml-pipeline-visualizationserver-66f4f8d86f-qxm4c 1/1 Running 0 3m
Configuring Authentication
Set up basic authentication using Kubernetes RBAC:
apiVersion: v1
kind: ServiceAccount
metadata:
name: pipeline-runner
namespace: kubeflow
---
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: pipeline-runner-role
namespace: kubeflow
rules:
- apiGroups: [""]
resources: ["pods", "pods/log"]
verbs: ["get", "list", "watch", "create", "delete"]
Setting Up Persistent Storage
Configure a PersistentVolumeClaim for pipeline artifacts:
apiVersion: v1
kind: PersistentVolumeClaim
metadata:
name: pipeline-artifacts
namespace: kubeflow
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 10Gi
Creating Your First ML Pipeline
Let's create a simple pipeline that includes data preprocessing, training, and model evaluation stages.
import kfp
from kfp import dsl
@dsl.pipeline(
name='Simple ML Pipeline',
description='A simple ML pipeline for demonstration'
)
def ml_pipeline():
preprocess_op = dsl.ContainerOp(
name='Preprocess Data',
image='preprocessor:latest',
command=['python', 'preprocess.py'],
file_outputs={
'processed_data': '/output/processed_data.csv'
}
)
train_op = dsl.ContainerOp(
name='Train Model',
image='trainer:latest',
command=['python', 'train.py'],
arguments=[
'--data', preprocess_op.outputs['processed_data']
],
file_outputs={
'model': '/output/model.h5'
}
)
evaluate_op = dsl.ContainerOp(
name='Evaluate Model',
image='evaluator:latest',
command=['python', 'evaluate.py'],
arguments=[
'--model', train_op.outputs['model']
]
)
# Compile the pipeline
kfp.compiler.Compiler().compile(ml_pipeline, 'pipeline.yaml')
Working with Kubeflow Notebooks
Kubeflow Notebooks provide an interactive development environment that integrates seamlessly with your ML pipeline development workflow.
Creating Custom Notebook Servers
- From the Kubeflow Dashboard, create a new notebook server:
apiVersion: kubeflow.org/v1
kind: Notebook
metadata:
name: ml-notebook
namespace: kubeflow
spec:
template:
spec:
containers:
- name: notebook
image: gcr.io/kubeflow-images-public/tensorflow-2.8.0-notebook-cpu:latest
resources:
limits:
cpu: "4"
memory: 8Gi
requests:
cpu: "1"
memory: 2Gi
volumeMounts:
- name: workspace
mountPath: /home/jovyan
volumes:
- name: workspace
persistentVolumeClaim:
claimName: workspace-pvc
Managing Dependencies
Create a custom Dockerfile for your notebook environment:
FROM gcr.io/kubeflow-images-public/tensorflow-2.8.0-notebook-cpu:latest
# Install additional Python packages
COPY requirements.txt /tmp/
RUN pip install -r /tmp/requirements.txt
# Install custom kernels or tools
RUN conda install -y scikit-learn pandas numpy matplotlib
# Add custom configurations
COPY jupyter_notebook_config.py /etc/jupyter/
Building Production-Ready Training Workflows
Component Definition
Create reusable components for your pipeline:
from kfp.dsl import component
@component(
base_image='python:3.8',
packages_to_install=['pandas', 'scikit-learn']
)
def data_preprocessing(
input_data_path: str,
output_data_path: str
):
"""Preprocess input data and save the result."""
import pandas as pd
from sklearn.preprocessing import StandardScaler
# Load and preprocess data
df = pd.read_csv(input_data_path)
scaler = StandardScaler()
df_scaled = pd.DataFrame(
scaler.fit_transform(df),
columns=df.columns
)
# Save processed data
df_scaled.to_csv(output_data_path, index=False)
return output_data_path
@component(
base_image='python:3.8',
packages_to_install=['tensorflow']
)
def model_training(
processed_data_path: str,
model_path: str,
epochs: int = 10
):
"""Train a model on the preprocessed data."""
import tensorflow as tf
import pandas as pd
# Implementation details...
Error Handling and Retries
Implement robust error handling in your pipeline:
@dsl.pipeline(
name='Production ML Pipeline',
description='Production-ready ML pipeline with error handling'
)
def production_pipeline():
with dsl.ExitHandler(exit_op=cleanup_op()):
preprocess = data_preprocessing(
input_data_path='gs://your-bucket/data.csv'
).set_retry(
num_retries=3,
backoff_duration='30s',
backoff_factor=2.0
)
train = model_training(
processed_data_path=preprocess.output,
epochs=10
).add_node_selector_constraint(
'cloud.google.com/gke-accelerator', 'nvidia-tesla-k80'
).set_retry(num_retries=2)
Model Serving and Deployment
Setting up KFServing
Deploy your trained model using KFServing:
apiVersion: serving.kubeflow.org/v1beta1
kind: InferenceService
metadata:
name: tensorflow-model
namespace: kubeflow
spec:
predictor:
tensorflow:
storageUri: "gs://your-bucket/model"
resources:
limits:
cpu: "4"
memory: 8Gi
requests:
cpu: "1"
memory: 2Gi
Implementing Canary Deployments
Create a canary deployment strategy:
apiVersion: serving.kubeflow.org/v1beta1
kind: InferenceService
metadata:
name: model-canary
namespace: kubeflow
spec:
predictor:
canaryTrafficPercent: 20
tensorflow:
storageUri: "gs://your-bucket/model-v2"
Pipeline Monitoring and Logging
Metrics Collection
Implement custom metrics using Prometheus:
from prometheus_client import Counter, Histogram
prediction_counter = Counter(
'model_predictions_total',
'Total number of predictions made',
['model_version']
)
prediction_latency = Histogram(
'prediction_latency_seconds',
'Time spent processing prediction'
)
@prediction_latency.time()
def predict(input_data):
result = model.predict(input_data)
prediction_counter.labels(model_version='v1').inc()
return result
Logging Configuration
Set up structured logging:
import logging
import json
logger = logging.getLogger('ml_pipeline')
def setup_logging():
handler = logging.StreamHandler()
handler.setFormatter(
logging.Formatter(
'%(asctime)s - %(name)s - %(levelname)s - %(message)s'
)
)
logger.addHandler(handler)
logger.setLevel(logging.INFO)
def log_metrics(metrics):
logger.info(json.dumps({
'event_type': 'metrics',
'metrics': metrics
}))
Production Best Practices
Resource Optimization
Right-sizing Resources
resources: limits: cpu: "4" memory: 8Gi nvidia.com/gpu: "1" requests: cpu: "2" memory: 4Gi
Autoscaling Configuration
apiVersion: autoscaling/v2beta1 kind: HorizontalPodAutoscaler metadata: name: model-hpa spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: model-deployment minReplicas: 2 maxReplicas: 10 metrics: - type: Resource resource: name: cpu targetAverageUtilization: 70
Pipeline Optimization and Scaling
Distributed Training Implementation
Implement distributed training using TensorFlow:
@dsl.pipeline(
name='Distributed Training Pipeline',
description='Multi-worker distributed training'
)
def distributed_training_pipeline():
worker_count = 4
with dsl.ParallelFor([i for i in range(worker_count)]) as worker:
train = dsl.ContainerOp(
name=f'worker-{worker}',
image='tensorflow/tensorflow:2.8.0',
command=['python', '/opt/train.py'],
arguments=[
'--worker_id', worker,
'--worker_count', worker_count
]
)
train.set_gpu_limit(1)
GPU Utilization
Configure GPU-aware scheduling:
apiVersion: scheduling.k8s.io/v1
kind: PriorityClass
metadata:
name: gpu-priority
value: 1000000
globalDefault: false
description: "Priority class for GPU workloads"
---
apiVersion: batch/v1
kind: Job
metadata:
name: gpu-training
spec:
template:
spec:
priorityClassName: gpu-priority
containers:
- name: training
image: training-image:latest
resources:
limits:
nvidia.com/gpu: 1
env:
- name: CUDA_VISIBLE_DEVICES
value: "0"
Integration with External Tools
Setting up CI/CD Pipeline
Example GitHub Actions workflow:
name: ML Pipeline CI/CD
on:
push:
branches: [main]
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Configure Kubeflow
run: |
echo "${{ secrets.KUBECONFIG }}" > kubeconfig.yaml
export KUBECONFIG=kubeconfig.yaml
- name: Compile Pipeline
run: |
pip install kfp
python pipeline/compile.py
- name: Deploy Pipeline
run: |
python pipeline/deploy.py \
--pipeline-package pipeline.yaml \
--experiment-name production
Model Registry Integration
from mlflow import MlflowClient
from kfp import components
def register_model(
model_uri: str,
model_name: str,
registry_uri: str
):
client = MlflowClient(registry_uri)
# Register model
result = client.create_registered_model(model_name)
# Create new version
version = client.create_model_version(
name=model_name,
source=model_uri,
run_id=None
)
return version.version
register_op = components.create_component_from_func(
func=register_model,
base_image='python:3.8',
packages_to_install=['mlflow']
)
Troubleshooting and Maintenance
Common Issues and Solutions
- Pipeline Failures
def diagnose_pipeline_failure(run_id: str):
client = kfp.Client()
run = client.get_run(run_id)
if run.status.error:
print(f"Pipeline failed with error: {run.status.error}")
# Get failed steps
failed_steps = [
step for step in run.status.nodes
if step.status.state == 'FAILED'
]
for step in failed_steps:
print(f"\nFailed step: {step.name}")
print(f"Error message: {step.error}")
# Get logs
logs = client.get_pod_logs(
step.pod_name,
step.namespace
)
print(f"Logs:\n{logs}")
- Resource Issues
#!/bin/bash
function check_resource_usage() {
echo "Checking node resource usage..."
kubectl top nodes
echo "\nChecking pod resource usage..."
kubectl top pods -n kubeflow
echo "\nChecking pending pods..."
kubectl get pods -n kubeflow | grep Pending
echo "\nChecking failed pods..."
kubectl get pods -n kubeflow | grep Failed
}
Case Study: End-to-End Implementation
Real-World Scenario: Customer Churn Prediction
from kfp import dsl
from kfp.components import create_component_from_func
def data_ingestion(data_path: str) -> str:
"""Ingest customer data from various sources."""
# Implementation details...
def feature_engineering(
raw_data_path: str,
output_path: str
):
"""Create features for churn prediction."""
# Implementation details...
def model_training(
feature_path: str,
model_path: str
):
"""Train churn prediction model."""
# Implementation details...
@dsl.pipeline(
name='Customer Churn Pipeline',
description='End-to-end customer churn prediction'
)
def churn_pipeline():
ingest = data_ingestion(
data_path='gs://customer-data/raw'
)
features = feature_engineering(
raw_data_path=ingest.output,
output_path='gs://customer-data/features'
)
train = model_training(
feature_path=features.output,
model_path='gs://models/churn'
)
# Deploy model
deploy = model_deployment(
model_path=train.output,
deployment_name='churn-predictor'
)
Future Considerations
Emerging Trends
AutoML Integration
Integration with tools like Katib for hyperparameter tuning
Automated feature selection
Neural architecture search
Federated Learning
Cross-silo training
Privacy-preserving ML
Edge deployment
MLOps Evolution
Increased automation
Enhanced monitoring
Improved governance
Additional Resources
Official Documentation
Community Resources
GitHub repositories
Slack channels
User groups
Conference talks
Related Projects
TensorFlow Extended (TFX)
MLflow
Feast (Feature Store)
Seldon Core
Conclusion
Building ML pipelines with Kubeflow provides a robust foundation for deploying machine learning workloads at scale. By following the best practices and patterns outlined in this guide, you can create maintainable, scalable, and production-ready ML systems.
Key takeaways:
Start with a solid architecture
Implement proper monitoring and logging
Follow production best practices
Plan for scaling and maintenance
Stay updated with the ecosystem
Remember that building production ML pipelines is an iterative process. Start small, test thoroughly, and scale gradually based on your needs.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
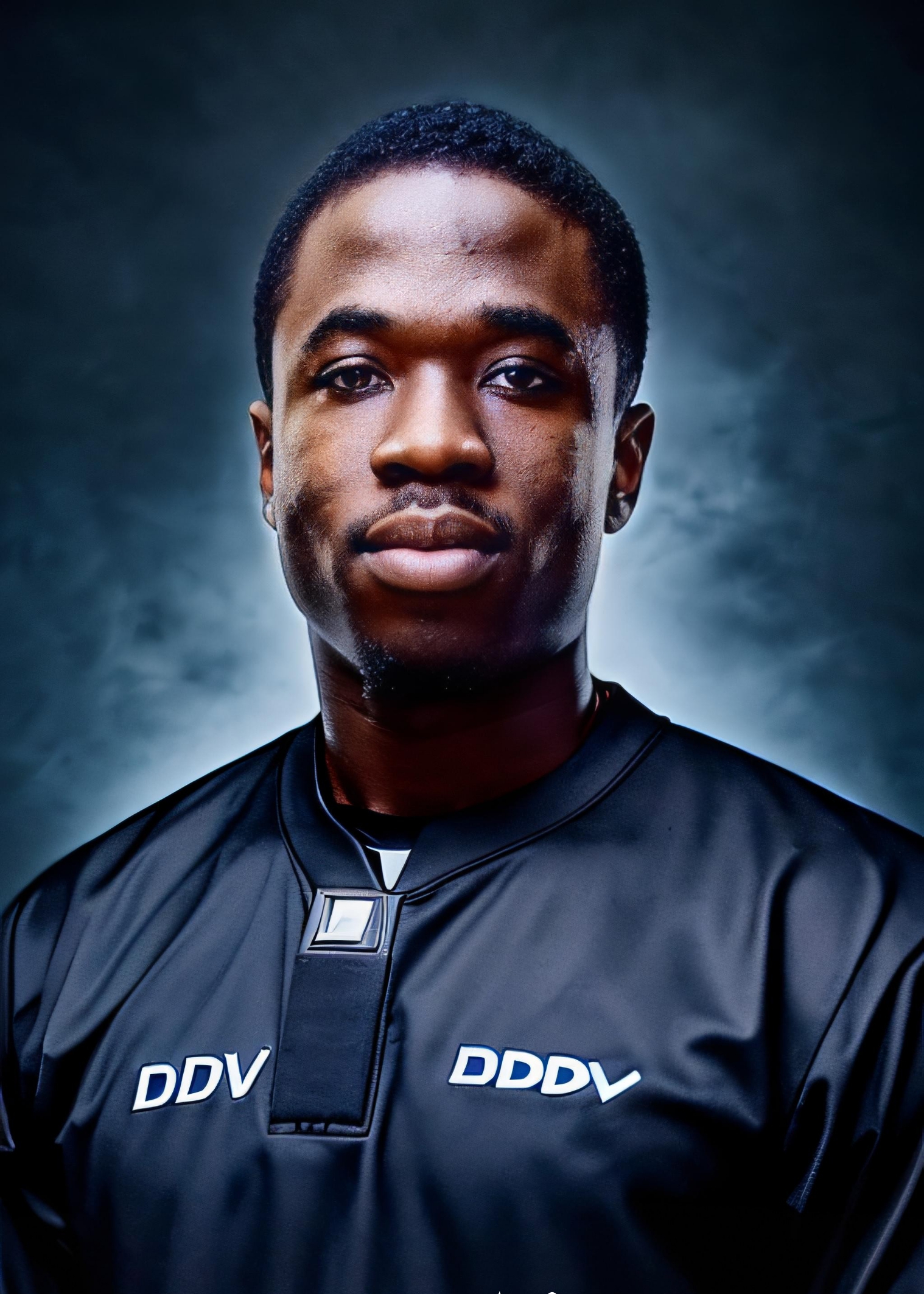
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.