Building a Better React Application with Repository and Adapter Design Patterns

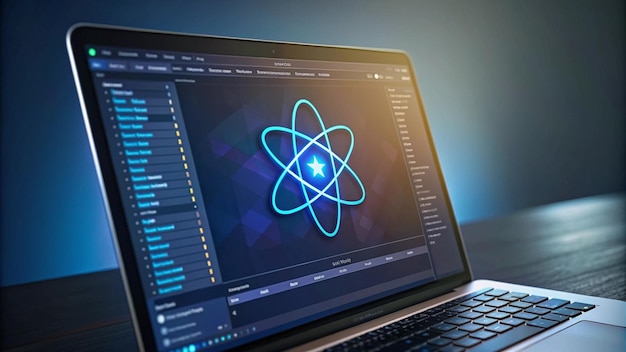
In modern web development, React applications development requires efficient data handling and smooth communication between components and external systems. Two popular design patterns that help achieve this are the Repository and Adapter patterns. By implementing these patterns, developers can streamline data management, improve code organization, and enhance overall application performance. This article explores how to leverage these patterns to build a scalable and robust React application.
Understanding the Design Patterns
1. Repository Pattern
The Repository Pattern is a structural pattern that provides an abstraction over data storage, acting as a bridge between the application and data sources. It centralizes data access logic, which means all data-related operations (CRUD) are handled through a single interface. This approach simplifies code management, especially in larger applications.
Benefits of the Repository Pattern:
Centralizes data access, enhancing maintainability.
Promotes separation of concerns, making code reusable.
Simplifies testing by allowing easy mocking of data access.
2. Adapter Pattern
The Adapter Pattern is another structural pattern that allows incompatible interfaces to work together. In React applications, the Adapter pattern can translate data from one format to another, ensuring that data from different sources fits seamlessly into the app’s components. By serving as a translator, it helps manage inconsistencies and keeps components isolated from data transformation logic.
Benefits of the Adapter Pattern:
Enables compatibility between mismatched data formats.
Keeps components decoupled from data structure specifics.
Improves code readability and maintainability.
Setting Up the Project
To get started with implementing these patterns, we’ll create a React application. For this example, assume we are using data from a REST API.
Initialize the project:
npx create-react-app react-design-patterns cd react-design-patterns npm install axios
Project dependencies: We’ll use
axios
for API requests, but feel free to replace it with any preferred method for fetching data.
Structuring the Project
A well-organized folder structure is crucial for maintaining scalability. Here’s an example of how you could structure the project:
src
│
├── components # Contains reusable components
│ ├── UserComponent.js
│
├── repositories # Contains repositories for data access
│ ├── UserRepository.js
│
├── adapters # Contains adapters to standardize data format
│ ├── UserAdapter.js
│
└── App.js # Main application component
This structure helps keep components, repositories, and adapters isolated, making the project easy to maintain and scale.
Implementing the Repository
The repository acts as a single source of truth for data-related operations, such as fetching, creating, updating, and deleting data.
Example Code for UserRepository.js
:
import axios from 'axios';
class UserRepository {
constructor() {
this.apiURL = 'https://api.example.com/users';
}
async fetchUsers() {
try {
const response = await axios.get(this.apiURL);
return response.data;
} catch (error) {
console.error('Error fetching users:', error);
throw error;
}
}
async fetchUserById(id) {
try {
const response = await axios.get(`${this.apiURL}/${id}`);
return response.data;
} catch (error) {
console.error('Error fetching user by ID:', error);
throw error;
}
}
}
export default new UserRepository();
In this repository, we define methods to fetch users and fetch a user by ID. This encapsulation allows us to manage all API interactions from a single location.
Implementing the Adapter
The adapter pattern helps convert data formats from the repository to the format required by the components.
Example Code for UserAdapter.js
:
class UserAdapter {
static adaptUser(data) {
return {
id: data.user_id,
name: data.user_name,
email: data.user_email,
isActive: data.is_active,
};
}
static adaptUserList(dataList) {
return dataList.map(data => this.adaptUser(data));
}
}
export default UserAdapter;
In this example, the adapter translates API data properties (user_id
, user_name
, etc.) into a format that the application expects (id
, name
, etc.). This way, the data transformations are isolated from components, making the code cleaner and easier to maintain.
Using the Repository and Adapter in a Component
Once the repository and adapter are set up, we can use them within a component to retrieve and display data.
Example Code for UserComponent.js
:
import React, { useEffect, useState } from 'react';
import UserRepository from '../repositories/UserRepository';
import UserAdapter from '../adapters/UserAdapter';
const UserComponent = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const rawUserData = await UserRepository.fetchUsers();
const adaptedUserData = UserAdapter.adaptUserList(rawUserData);
setUsers(adaptedUserData);
} catch (error) {
console.error('Error fetching and adapting user data:', error);
}
};
fetchData();
}, []);
return (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>
{user.name} - {user.email}
</li>
))}
</ul>
</div>
);
};
export default UserComponent;
Here, we’re using both the repository to fetch data and the adapter to convert it to the desired format before setting it in the component state. This keeps our component free from data-fetching logic and data transformation details.
Putting It All Together
Now that the Repository and Adapter patterns are implemented, our component code is streamlined. We’ve separated data access logic and data transformation logic, making it easier to manage and test. Here’s a recap of how everything fits:
Repository: Handles API requests and centralizes data fetching.
Adapter: Transforms data into a standardized format for the components.
Component: Displays data without handling fetching or transformation.
This modular approach enhances code readability, maintainability, and flexibility, making it easier to add new data sources or modify the data structure as needed.
Conclusion
Using the Repository and Adapter design patterns in a React application promotes a clean architecture and simplifies complex data flows. The Repository pattern centralizes data access, while the Adapter pattern standardizes data formats, reducing component dependencies and enhancing testability. This approach is equally beneficial for mobile application development, where efficient data management and consistent data formats are essential for a seamless user experience. By implementing these patterns, you can build scalable, maintainable React applications that handle data more effectively, setting up a solid foundation for future growth.
Subscribe to my newsletter
Read articles from AddWeb Solution directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

AddWeb Solution
AddWeb Solution
Outcome-driven IT development, consulting, and outsourcing company, specializing in Web/Mobile App Development. For the past 10+ years, we have thrived by ‘adding’ value to the ‘web’ world with our timely and quality ‘solutions’. Our IT solutions help startups grow, improve the reach of medium-sized businesses, and help larger ventures make deeper connections. AddWeb Solution is consistently sloping upwards, providing flawless solutions, timely deliveries, and boosting overall productivity by ensuring maximum ROI. We are really proud of building great products for world-class brands. We are a professional and friendly team with experience ranging from 2 to 16 years. With more than 500+ successful projects delivered, we are ready to take it to the next height.