Data Encryption in Android: A Comprehensive Guide [PART 1]
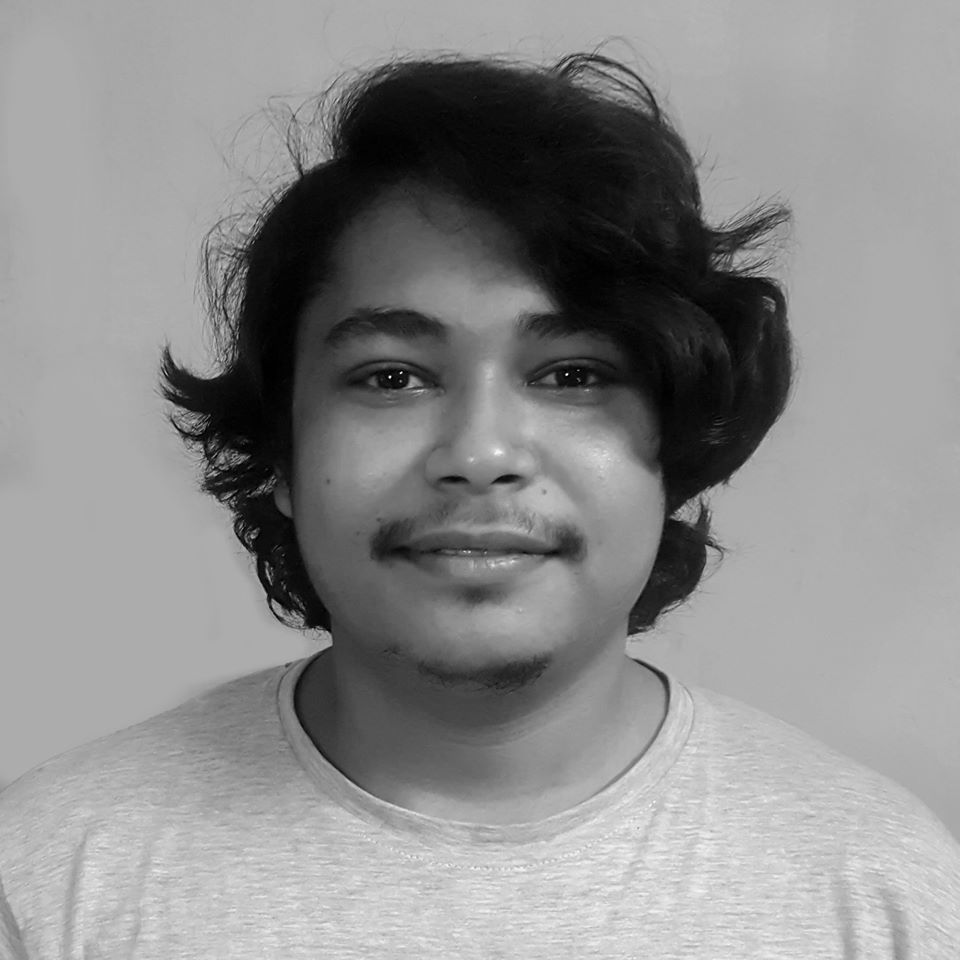
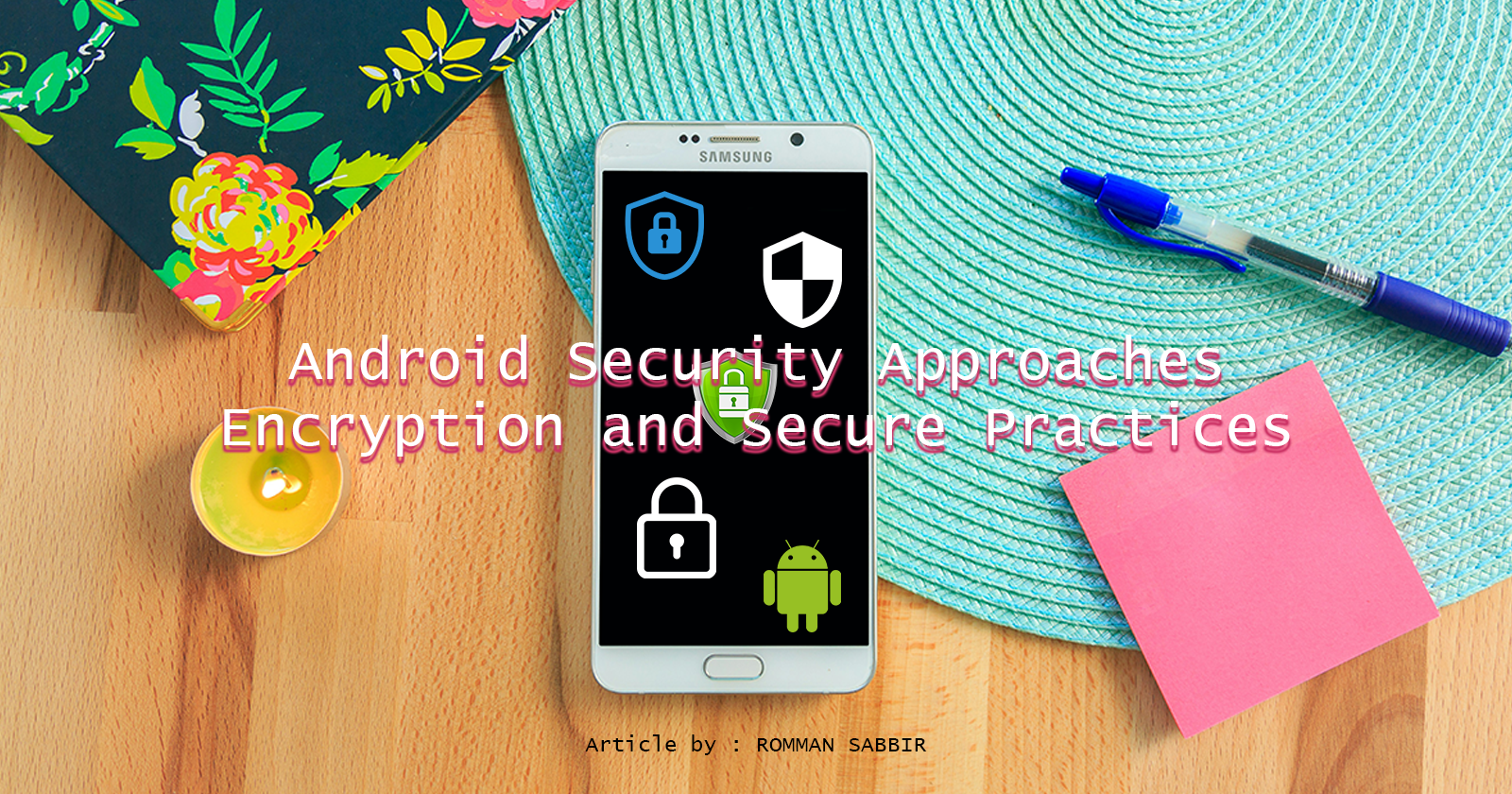
Data encryption is a fundamental part of securing mobile applications, particularly those handling sensitive information like user credentials, tokens, or files. Android provides several encryption techniques and APIs that developers can implement to ensure the security of data both at rest and in transit. In this article, we will explore different encryption methods available in Android, including AES, RSA, the KeyStore API, File-Based Encryption (FBE), and Network Security Configuration. We will provide explanations, real-world use cases, and Kotlin code snippets to help you implement these techniques in your Android applications.
AES (Advanced Encryption Standard)
AES is a symmetric encryption algorithm widely used to encrypt sensitive data such as user credentials, tokens, or files. Being a symmetric encryption method, it uses the same key for both encryption and decryption.
Use Case: Encrypting User Credentials
When your app needs to store sensitive information, such as a user's password or API token, AES is a strong choice for encrypting this data before storing it locally.
Kotlin Example: Encrypting Data Using AES
import java.security.SecureRandom
import javax.crypto.Cipher
import javax.crypto.KeyGenerator
import javax.crypto.SecretKey
import javax.crypto.spec.GCMParameterSpec
// Generate AES key
fun generateAESKey(): SecretKey {
val keyGen = KeyGenerator.getInstance("AES")
keyGen.init(256) // 256-bit AES key
return keyGen.generateKey()
}
// Encrypt data using AES
fun encryptData(data: ByteArray, secretKey: SecretKey): ByteArray {
val cipher = Cipher.getInstance("AES/GCM/NoPadding")
val iv = ByteArray(12) // GCM recommended IV length is 12 bytes
SecureRandom().nextBytes(iv) // Generate random IV
val gcmSpec = GCMParameterSpec(128, iv)
cipher.init(Cipher.ENCRYPT_MODE, secretKey, gcmSpec)
return iv + cipher.doFinal(data)
}
// Decrypt data using AES
fun decryptData(encryptedData: ByteArray, secretKey: SecretKey): ByteArray {
val iv = encryptedData.copyOfRange(0, 12)
val cipherData = encryptedData.copyOfRange(12, encryptedData.size)
val cipher = Cipher.getInstance("AES/GCM/NoPadding")
val gcmSpec = GCMParameterSpec(128, iv)
cipher.init(Cipher.DECRYPT_MODE, secretKey, gcmSpec)
return cipher.doFinal(cipherData)
}
In this example, GCM mode (Galois/Counter Mode) is used, which is a secure mode for AES encryption that also provides integrity by generating an authentication tag.
RSA (Rivest-Shamir-Adleman)
RSA is an asymmetric encryption algorithm commonly used for secure data transmission, such as key exchange or signing sensitive information. Unlike AES, RSA uses a pair of keys: a public key for encryption and a private key for decryption.
Use Case: Secure Key Exchange
In Android applications, RSA is often used in combination with AES. RSA can encrypt the AES key itself, which is then securely exchanged between the client and server, while the data is encrypted using the faster AES algorithm.
Kotlin Example: RSA Encryption and Decryption
import java.security.KeyPairGenerator
import java.security.PrivateKey
import java.security.PublicKey
import javax.crypto.Cipher
// Generate RSA key pair
fun generateRSAKeyPair(): Pair<PublicKey, PrivateKey> {
val keyPairGenerator = KeyPairGenerator.getInstance("RSA")
keyPairGenerator.initialize(2048) // 2048-bit RSA key
val keyPair = keyPairGenerator.generateKeyPair()
return Pair(keyPair.public, keyPair.private)
}
// Encrypt data using RSA public key
fun encryptDataWithRSA(data: ByteArray, publicKey: PublicKey): ByteArray {
val cipher = Cipher.getInstance("RSA/ECB/OAEPWithSHA-256AndMGF1Padding")
cipher.init(Cipher.ENCRYPT_MODE, publicKey)
return cipher.doFinal(data)
}
// Decrypt data using RSA private key
fun decryptDataWithRSA(encryptedData: ByteArray, privateKey: PrivateKey): ByteArray {
val cipher = Cipher.getInstance("RSA/ECB/OAEPWithSHA-256AndMGF1Padding")
cipher.init(Cipher.DECRYPT_MODE, privateKey)
return cipher.doFinal(encryptedData)
}
Here, OAEP (Optimal Asymmetric Encryption Padding) is used for RSA encryption, which is recommended for preventing attacks such as padding oracle attacks.
KeyStore API
The Android KeyStore system allows you to securely generate and store cryptographic keys. The keys are stored in a hardware-backed or software-only keystore, depending on the device. This API ensures that the keys are not accessible by any unauthorized process or application.
Use Case: Storing Encryption Keys Securely
You can use the KeyStore to store AES or RSA keys, ensuring that even if the device is compromised, the keys remain protected.
Kotlin Example: Using Android KeyStore
import android.security.keystore.KeyGenParameterSpec
import android.security.keystore.KeyProperties
import java.security.KeyStore
import javax.crypto.KeyGenerator
import javax.crypto.SecretKey
// Generate and store AES key in KeyStore
fun generateAndStoreKey(): SecretKey {
val keyGenerator = KeyGenerator.getInstance(KeyProperties.KEY_ALGORITHM_AES, "AndroidKeyStore")
val keyGenParameterSpec = KeyGenParameterSpec.Builder(
"MyKeyAlias",
KeyProperties.PURPOSE_ENCRYPT or KeyProperties.PURPOSE_DECRYPT
)
.setBlockModes(KeyProperties.BLOCK_MODE_GCM)
.setEncryptionPaddings(KeyProperties.ENCRYPTION_PADDING_NONE)
.build()
keyGenerator.init(keyGenParameterSpec)
return keyGenerator.generateKey()
}
// Retrieve the AES key from KeyStore
fun getKeyFromKeyStore(): SecretKey? {
val keyStore = KeyStore.getInstance("AndroidKeyStore")
keyStore.load(null)
return keyStore.getKey("MyKeyAlias", null) as SecretKey?
}
By storing keys in the Android KeyStore, you ensure that they are isolated from the application’s memory and more secure from external threats.
File-Based Encryption (FBE)
File-Based Encryption (FBE) is an Android feature that encrypts files on a per-user basis. This means that individual files are encrypted with different keys, adding an extra layer of security. FBE ensures that files are inaccessible until the user is authenticated.
Use Case: Protecting User Files
If your app deals with sensitive files like medical records, documents, or images, using FBE ensures that files remain encrypted even when the device is locked or not in use.
Implementation in Android
File-based encryption is automatically enabled in newer Android devices, and developers only need to ensure their apps support FBE by using standard storage APIs.
// Example of writing encrypted data to storage using Android Storage APIs
val encryptedData = encryptData("Sensitive File Data".toByteArray(), secretKey)
applicationContext.openFileOutput("encrypted_file", Context.MODE_PRIVATE).use {
it.write(encryptedData)
}
With FBE, you don’t have to manage encryption and decryption directly. Android handles it based on user authentication states.
Network Security Configuration
The Network Security Configuration in Android allows you to customize your app’s network security settings, such as enforcing HTTPS connections and defining policies for certificate pinning. This ensures that your app communicates over secure channels.
Use Case: Enforcing Secure Network Communication
Many Android apps communicate with backend servers. Using HTTPS ensures that data transmitted between the app and server is encrypted, preventing eavesdropping or data tampering.
XML Configuration for HTTPS Enforcement
To enforce HTTPS in your app, you can use a network security configuration file:
<!-- res/xml/network_security_config.xml -->
<network-security-config>
<domain-config cleartextTrafficPermitted="false">
<domain includeSubdomains="true">example.com</domain>
</domain-config>
</network-security-config>
Then, specify this configuration in your AndroidManifest.xml:
<application
android:networkSecurityConfig="@xml/network_security_config"
... >
</application>
By setting cleartextTrafficPermitted="false"
, you prevent your app from sending data over insecure HTTP.
Conclusion
Encrypting data is essential for building secure Android applications. Whether it’s encrypting sensitive data with AES, securely exchanging keys with RSA, or using the KeyStore API to store cryptographic keys, these encryption methods protect your app from various security threats. Implementing File-Based Encryption (FBE) ensures that files are encrypted at rest, and enforcing secure network communication with Network Security Configuration protects data in transit.
By leveraging these encryption techniques and tools, you can build Android apps that not only meet security best practices but also safeguard user data against potential attacks.
That's it for today. Happy Coding...
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
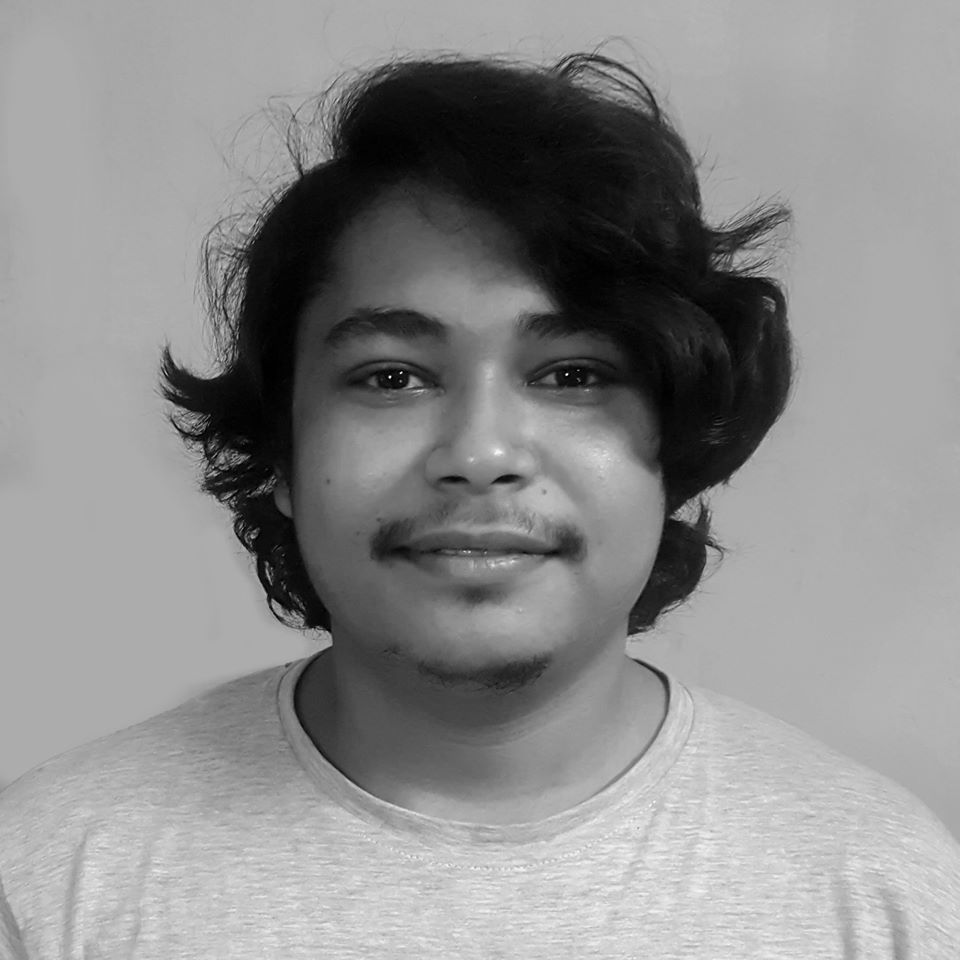
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.