@Recover Method in Spring Boot's Retry Mechanism
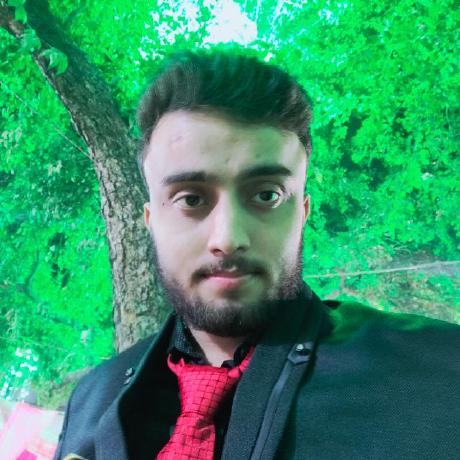
Introduction
In microservices architecture, handling transient failures gracefully is crucial for building resilient applications. Spring Boot’s retry mechanism provides a robust way to automatically retry failed operations. While retrying is helpful, there are situations where all retry attempts fail. This is where the @Recover
annotation comes into play, offering a fallback mechanism for graceful degradation.
What is @Recover?
The @Recover
annotation in Spring Boot defines a fallback method that gets executed when all retry attempts are exhausted. It acts as a safety net, allowing you to handle failures gracefully rather than letting exceptions propagate up the call stack.
Implementation Details
Basic Setup
You can include the Spring Retry dependency using either Maven or Gradle:
Maven Configuration (pom.xml):
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
Gradle Configuration (build.gradle):
dependencies {
implementation 'org.springframework.retry:spring-retry'
implementation 'org.springframework.boot:spring-boot-starter-aop'
}
Gradle Configuration (build.gradle.kts):
dependencies {
implementation("org.springframework.retry:spring-retry")
implementation("org.springframework.boot:spring-boot-starter-aop")
}
Enable retry functionality in your application:
@EnableRetry
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Using @Recover
Here’s a typical implementation pattern:
@Service
public class PaymentService {
@Retryable(
value = PaymentException.class,
maxAttempts = 3,
backoff = @Backoff(delay = 1000)
)
public String processPayment(String orderId, double amount) {
// Payment processing logic that might fail
throw new PaymentException("Payment gateway timeout");
}
@Recover
public String recoverPayment(PaymentException e, String orderId, double amount) {
// Fallback logic
return "Payment failed after retries. Using alternative payment gateway for order: " + orderId;
}
}
Key Features and ConsiderationsGradle Configuration (build.gradle.kts):
dependencies {
implementation("org.springframework.retry:spring-retry")
implementation("org.springframework.boot:spring-boot-starter-aop")
}
Enable retry functionality in your application:
@EnableRetry
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Using @Recover
Here’s a typical implementation pattern:
@Service
public class PaymentService {
@Retryable(
value = PaymentException.class,
maxAttempts = 3,
backoff = @Backoff(delay = 1000)
)
public String processPayment(String orderId, double amount) {
// Payment processing logic that might fail
throw new PaymentException("Payment gateway timeout");
}
@Recover
public String recoverPayment(PaymentException e, String orderId, double amount) {
// Fallback logic
return "Payment failed after retries. Using alternative payment gateway for order: " + orderId;
}
}
Key Features and Considerations
1. Method Signature Matching
The @Recover
method must:
Have the same return type as the
@Retryable
methodTake the exception type as its first parameter
Include all parameters from the retryable method in the same order
2. Multiple Recovery Methods
You can define multiple recovery methods for different exception types:
@Recover
public String recoverPayment(PaymentException e, String orderId, double amount) {
// Handle payment-specific exceptions
return "Payment recovery logic";
}
@Recover
public String recoverPayment(NetworkException e, String orderId, double amount) {
// Handle network-specific exceptions
return "Network recovery logic";
}
3. Exception Hierarchy
Spring Retry matches the most specific exception handler first, following the exception hierarchy:
@Recover
public String recoverPayment(Exception e, String orderId, double amount) {
// Generic fallback
return "Generic recovery logic";
}
Best Practices
- Logging: Always log the exception details in your recover method for debugging:
@Recover
public String recoverPayment(PaymentException e, String orderId, double amount) {
log.error("Payment failed after all retries. Order: {}, Error: {}", orderId, e.getMessage());
// Recovery logic
}
2. Monitoring: Add metrics to track recovery invocations:
@Recover
public String recoverPayment(PaymentException e, String orderId, double amount) {
meterRegistry.counter("payment.recovery.invocations").increment();
// Recovery logic
}
3. Circuit Breaking: Consider combining with circuit breakers:
@CircuitBreaker(name = "payment")
@Retryable(value = PaymentException.class)
public String processPayment(String orderId, double amount) {
// Payment logic
}
3. Configuration Properties:
You can also configure retry properties in your application.properties/application.yml file:
spring:
retry:
max-attempts: 3
initial-interval: 1000
multiplier: 2.0
max-interval: 5000
Or with Gradle-specific configuration in build.gradle:
springBoot {
buildInfo()
}
configurations {
all {
exclude group: 'org.springframework.boot', module: 'spring-boot-starter-logging'
}
}
Limitations and Considerations
Recovery methods only work with Spring AOP proxies
They don’t support async operations out of the box
The method must be called from another Spring bean
Recovery methods should be idempotent
Common Issues and Solutions
Version Compatibility
When using Spring Boot 3.x, ensure you’re using compatible versions:
// For Gradle
ext {
springBootVersion = '3.x.x'
springRetryVersion = '2.x.x'
}
Transaction Management
When using with transactions:
@Service
@Transactional
public class PaymentService {
@Retryable(value = PaymentException.class)
@Transactional(propagation = Propagation.REQUIRES_NEW)
public String processPayment(String orderId, double amount) {
// Payment logic
}
}
Conclusion
The @Recover
annotation is a powerful feature in Spring Boot's retry mechanism that helps build resilient applications. By providing fallback mechanisms, it ensures graceful degradation when operations fail despite retries. When implemented correctly with proper logging and monitoring, it becomes an essential tool in building robust microservices.
Remember that while recovery methods provide a safety net, they should not become a crutch for poor error handling. Always analyze and address the root cause of failures while using recovery methods as part of your overall resilience strategy.
For optimal implementation, ensure you’ve properly configured your build system (Maven or Gradle) and included all necessary dependencies. Regular testing of both the retry and recovery mechanisms is essential to maintain system reliability.
Subscribe to my newsletter
Read articles from Harsh Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
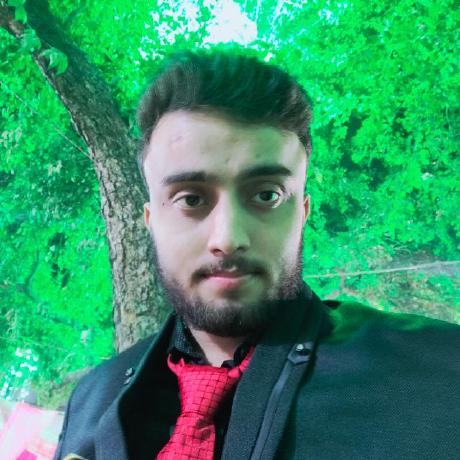