Object-Oriented Programming (OOP): An In-Depth Guide

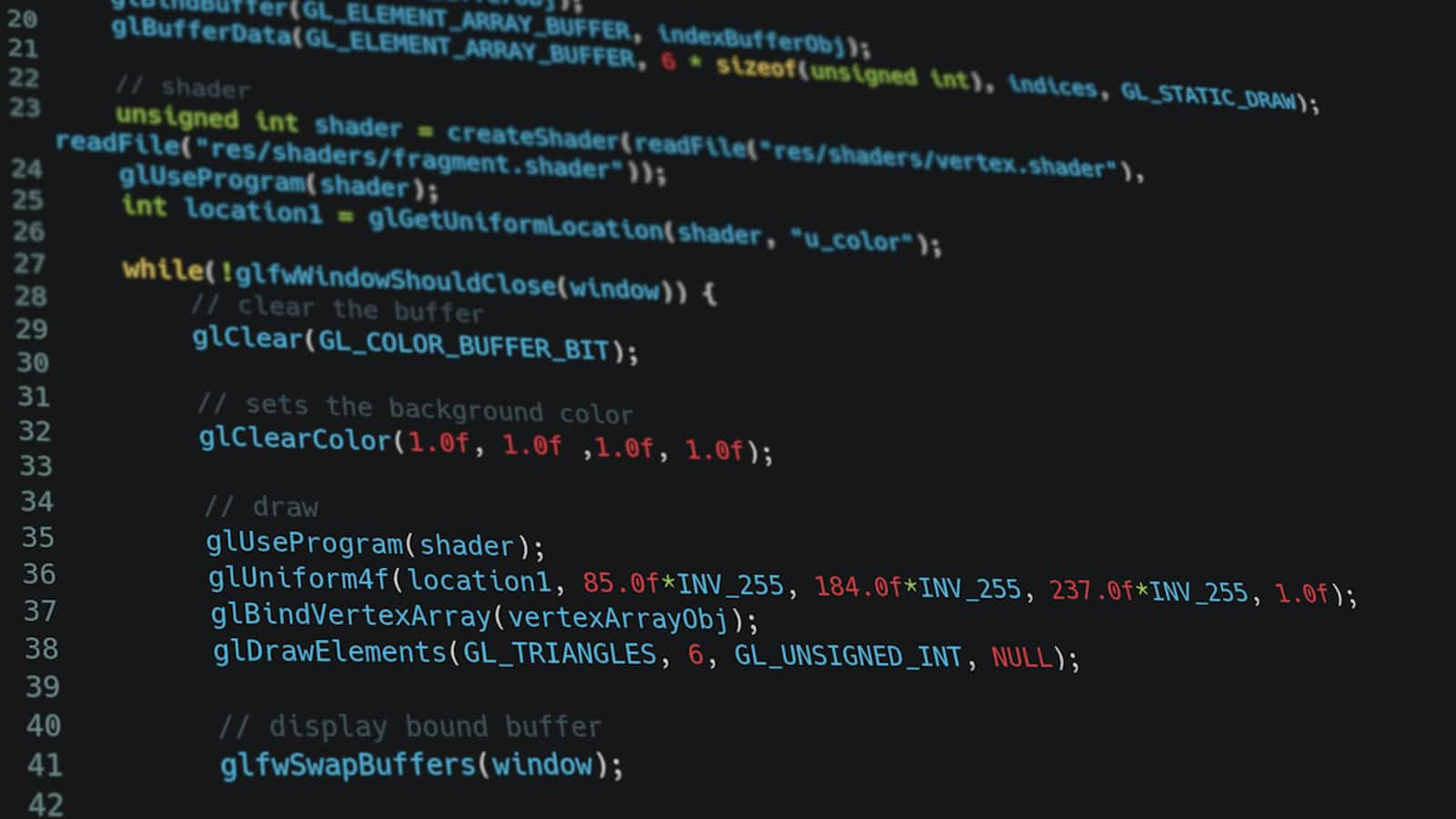
Introduction
Object-Oriented Programming (OOP) is a programming paradigm centered around objects rather than actions. It allows developers to model real-world entities using classes and objects, promoting code reusability, modularity, and maintainability. OOP is a fundamental concept in software development and is widely used in languages like Python, Java, C++, and more.
Table of Contents
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm based on the concept of "objects", which can contain data and code: data in the form of fields (attributes or properties), and code in the form of procedures (methods).
OOP focuses on:
Encapsulating data and functions together in objects.
Reusing code through inheritance and composition.
Interacting with objects through well-defined interfaces.
Key Concepts of OOP
Classes and Objects
Class: A blueprint for creating objects. Defines attributes and methods.
Object: An instance of a class. Represents a specific entity with state and behavior.
Encapsulation
Encapsulation: Bundling data (attributes) and methods that operate on the data into a single unit (class).
Purpose: Protect the integrity of the data and prevent unauthorized access.
Abstraction
Abstraction: Hiding complex implementation details and exposing only the necessary features.
Purpose: Simplify interactions with complex systems.
Inheritance
Inheritance: Mechanism by which one class (child or subclass) can inherit attributes and methods from another class (parent or superclass).
Purpose: Promote code reusability and hierarchical classifications.
Polymorphism
Polymorphism: Ability of objects of different classes to be treated as objects of a common superclass.
Purpose: Allow for methods to use objects of different types interchangeably.
Detailed Examples and Code
Let's explore each concept with detailed examples in Python.
Classes and Objects Example
Defining a Class and Creating Objects
class Car:
# Class attribute
wheels = 4
# Constructor method
def __init__(self, make, model, year):
# Instance attributes
self.make = make
self.model = model
self.year = year
# Instance method
def start_engine(self):
print(f"The {self.make} {self.model} engine has started.")
# Creating objects (instances) of the Car class
car1 = Car("Toyota", "Corolla", 2020)
car2 = Car("Honda", "Civic", 2019)
# Accessing attributes and methods
print(car1.make) # Output: Toyota
print(car2.wheels) # Output: 4
car1.start_engine() # Output: The Toyota Corolla engine has started.
Explanation:
Class Definition:
class Car:
Constructor:
def __init__(self, make, model, year):
initializes instance attributes.Instance Attributes:
self.make
,self.model
,self.year
.Class Attribute:
wheels
is shared by all instances.Instance Method:
start_engine
operates on an instance.Creating Objects:
car1
andcar2
are instances ofCar
.
Encapsulation Example
Using Access Modifiers to Protect Data
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self.__balance = balance # Private attribute
# Public method to deposit money
def deposit(self, amount):
if amount > 0:
self.__balance += amount
print(f"Deposited ${amount}. New balance: ${self.__balance}")
else:
print("Invalid amount.")
# Public method to withdraw money
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
print(f"Withdrew ${amount}. New balance: ${self.__balance}")
else:
print("Insufficient funds or invalid amount.")
# Public method to get balance (read-only)
def get_balance(self):
return self.__balance
# Creating an instance
account = BankAccount("123456789", 1000)
# Using public methods to interact with private data
account.deposit(500) # Output: Deposited $500. New balance: $1500
account.withdraw(200) # Output: Withdrew $200. New balance: $1300
print(account.get_balance()) # Output: 1300
# Attempting to access private attribute directly
print(account.__balance) # AttributeError: 'BankAccount' object has no attribute '__balance'
Explanation:
Private Attribute:
__balance
is intended to be private.Public Methods:
deposit
,withdraw
,get_balance
provide controlled access.Encapsulation: Internal state (
__balance
) is protected from direct external modification.
Abstraction Example
Using Abstract Classes and Methods
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
def sleep(self):
print("Sleeping...")
class Dog(Animal):
def make_sound(self):
print("Bark!")
class Cat(Animal):
def make_sound(self):
print("Meow!")
# animal = Animal() # Cannot instantiate abstract class
dog = Dog()
cat = Cat()
dog.make_sound() # Output: Bark!
cat.make_sound() # Output: Meow!
dog.sleep() # Output: Sleeping!
Explanation:
Abstract Base Class:
Animal
is an abstract class with an abstract methodmake_sound
.Abstract Method:
@abstractmethod
decorator indicates that subclasses must implement this method.Abstraction: Hides implementation details;
Animal
defines an interface.Cannot Instantiate: You cannot create an instance of an abstract class.
Inheritance Example
Creating a Class Hierarchy
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def work(self):
print(f"{self.name} is working.")
class Manager(Employee):
def __init__(self, name, salary, department):
super().__init__(name, salary) # Call the parent class constructor
self.department = department
def work(self):
print(f"{self.name} is managing the {self.department} department.")
class Developer(Employee):
def __init__(self, name, salary, programming_language):
super().__init__(name, salary)
self.programming_language = programming_language
def work(self):
print(f"{self.name} is coding in {self.programming_language}.")
# Creating instances
manager = Manager("Alice", 90000, "Sales")
developer = Developer("Bob", 80000, "Python")
# Accessing methods
manager.work() # Output: Alice is managing the Sales department.
developer.work() # Output: Bob is coding in Python.
Explanation:
Parent Class:
Employee
is the base class.Child Classes:
Manager
andDeveloper
inherit fromEmployee
.Overriding Methods: Child classes override the
work
method.Using
super()
: Calls the constructor of the parent class.
Polymorphism Example
Using Polymorphism with Methods
class Shape:
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
import math
return math.pi * self.radius ** 2
# Function that takes any Shape and calculates the area
def calculate_area(shape):
print(f"The area is {shape.area()}")
# Creating instances
rectangle = Rectangle(5, 10)
circle = Circle(7)
# Using polymorphism
calculate_area(rectangle) # Output: The area is 50
calculate_area(circle) # Output: The area is 153.93804002589985
Explanation:
Polymorphism: The
calculate_area
function works with any object that has anarea
method.Common Interface: Both
Rectangle
andCircle
inherit fromShape
and implementarea
.Interchangeability: Different shapes can be used interchangeably in the function.
Additional OOP Concepts
Composition
Composition: A class can be composed of one or more objects of other classes.
"Has-a" Relationship: Indicates that an object contains or is composed of other objects.
Example:
class Engine:
def start(self):
print("Engine started.")
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
self.engine = Engine() # Car has an Engine
def start_car(self):
self.engine.start()
print(f"The {self.make} {self.model} is ready to go.")
car = Car("Ford", "Mustang")
car.start_car()
# Output:
# Engine started.
# The Ford Mustang is ready to go.
Explanation:
Composition:
Car
is composed of anEngine
.Encapsulation of Components:
Engine
is a part ofCar
.
Aggregation
Aggregation: Similar to composition but with a weaker relationship.
Objects can exist independently: The contained objects can exist without the container.
Example:
class Employee:
def __init__(self, name):
self.name = name
class Department:
def __init__(self, name, employees):
self.name = name
self.employees = employees # Department aggregates Employees
def get_employees(self):
for emp in self.employees:
print(emp.name)
# Employees can exist independently
emp1 = Employee("Alice")
emp2 = Employee("Bob")
department = Department("IT", [emp1, emp2])
department.get_employees()
# Output:
# Alice
# Bob
Explanation:
Aggregation:
Department
aggregatesEmployee
instances.Independent Existence:
Employee
instances exist outsideDepartment
.
Association
Association: A general term for a relationship between classes.
Objects can interact with each other: No ownership implied.
Example:
class Teacher:
def __init__(self, name):
self.name = name
def teach(self, student):
print(f"{self.name} is teaching {student.name}.")
class Student:
def __init__(self, name):
self.name = name
teacher = Teacher("Mr. Smith")
student = Student("John")
teacher.teach(student) # Output: Mr. Smith is teaching John.
Explanation:
Association:
Teacher
andStudent
are associated through theteach
method.No Ownership: Neither class owns the other.
Benefits of OOP
Modularity: Code is organized into separate objects.
Reusability: Classes can be reused across programs.
Extensibility: Easy to add new features through inheritance and polymorphism.
Maintainability: Encapsulation allows for code changes with minimal impact.
Conclusion
Object-Oriented Programming is a powerful paradigm that models software design around data (objects) and behaviors (methods). By understanding and applying OOP principles such as encapsulation, abstraction, inheritance, and polymorphism, developers can create flexible, modular, and reusable code.
Quick Revision Notes
Class: Blueprint for creating objects.
Object: Instance of a class.
Encapsulation: Bundling data and methods; protecting internal state.
Abstraction: Hiding complex details; exposing essential features.
Inheritance: One class inherits from another; promotes code reusability.
Polymorphism: Objects of different classes can be treated as objects of a common superclass.
Composition: "Has-a" relationship; objects composed of other objects.
Aggregation: Weak "has-a" relationship; objects can exist independently.
Association: General relationship between classes; objects interact without ownership.
Practice Exercise:
Create a Class Hierarchy for Employees:
Define a base class
Employee
with attributesname
andsalary
.Define methods
work()
anddisplay_info()
.Create subclasses
FullTimeEmployee
andPartTimeEmployee
that inherit fromEmployee
.Override the
work()
method in each subclass.Instantiate objects and demonstrate polymorphism.
Solution:
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def work(self):
print(f"{self.name} is working.")
def display_info(self):
print(f"Name: {self.name}, Salary: {self.salary}")
class FullTimeEmployee(Employee):
def work(self):
print(f"{self.name} is working full-time.")
class PartTimeEmployee(Employee):
def work(self):
print(f"{self.name} is working part-time.")
# Instances
emp_full = FullTimeEmployee("Alice", 60000)
emp_part = PartTimeEmployee("Bob", 30000)
# Polymorphism
for emp in (emp_full, emp_part):
emp.work()
emp.display_info()
# Output:
# Alice is working full-time.
# Name: Alice, Salary: 60000
# Bob is working part-time.
# Name: Bob, Salary: 30000
Additional Resources:
Python OOP Tutorial: Real Python - Object-Oriented Programming (OOP)
Official Python Documentation: Classes - Python Docs
Subscribe to my newsletter
Read articles from Sai Prasanna Maharana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
